How Can You Create a View on a Stored Procedure with ‘CREATE VIEW’ Syntax?
In the world of database management, efficiency and clarity are paramount. One powerful tool that can enhance these aspects is the use of views, particularly when they are created from stored procedures. The concept of creating a view that encapsulates the execution of a stored procedure, such as `exec dbo.uspstoredproceduretest`, opens up a realm of possibilities for developers and database administrators alike. This innovative approach not only streamlines the retrieval of complex data but also simplifies the interaction with underlying procedures, making it easier to integrate and manipulate data within applications.
Creating a view on a stored procedure allows users to leverage the procedural logic encapsulated within the procedure while presenting the results in a straightforward and accessible format. This method can be particularly beneficial in scenarios where data needs to be aggregated, filtered, or transformed before being presented to end-users. By abstracting the complexities of the stored procedure, developers can create a seamless experience for those querying the database, fostering better performance and maintainability.
As we delve deeper into the mechanics of creating a view based on a stored procedure, we will explore the benefits, potential pitfalls, and best practices associated with this technique. Whether you’re a seasoned database professional or a newcomer eager to enhance your SQL skills, understanding this concept will empower you to optimize your database
Understanding Views and Stored Procedures
In SQL Server, views provide a way to encapsulate complex queries and present data in a simplified format. A view can be thought of as a virtual table that is constructed from a query, offering a way to streamline the retrieval of data. Stored procedures, on the other hand, are precompiled collections of SQL statements that can perform operations such as data manipulation and retrieval.
While views are primarily used to present data, stored procedures execute logic and can include parameters, allowing for dynamic behavior. When there is a need to leverage the functionality of a stored procedure within the context of a view, one might consider the creation of a view that encapsulates the stored procedure’s execution.
Creating a View Based on a Stored Procedure
It is important to note that SQL Server does not allow direct execution of stored procedures within a view. Instead, one can use a workaround to achieve similar functionality. The typical approach involves creating a user-defined function (UDF) that wraps the stored procedure’s logic, which can then be referenced in the view.
Here is a basic outline of how you might approach this:
- Create a User-Defined Function: The function should encapsulate the logic of the stored procedure, allowing it to return a table that can be used in a view.
- Create the View: Once the function is in place, you can create a view that selects from this function.
Example
Assuming you have a stored procedure `dbo.uspstoredproceduretest`, the following is an example of creating a user-defined function and then a view.
Step 1: Create a User-Defined Function
“`sql
CREATE FUNCTION dbo.ufn_uspstoredproceduretest()
RETURNS TABLE
AS
RETURN (
EXEC dbo.uspstoredproceduretest
);
“`
Step 2: Create the View
“`sql
CREATE VIEW viewonstoredprocedure AS
SELECT * FROM dbo.ufn_uspstoredproceduretest();
“`
Key Considerations
- Performance: Using functions can sometimes lead to performance overhead, especially if the function is called multiple times in a query.
- Transaction Management: Stored procedures often handle transactions, and when wrapping them in functions, ensure that the transactional integrity is maintained.
- Return Types: The user-defined function must return a table type that aligns with the expected output of the stored procedure.
Limitations of Using Views with Stored Procedures
Limitation | Description |
---|---|
Direct Execution | You cannot directly execute a stored procedure within a view. |
Function Restrictions | User-defined functions have limitations regarding side effects. |
Performance Implications | Nested function calls can lead to slower performance. |
Using views in conjunction with stored procedures can be powerful but requires careful planning and consideration of SQL Server’s constraints. By creating a function that mirrors the stored procedure’s logic, you can effectively integrate the two while maintaining the benefits of both constructs.
Creating a View Based on a Stored Procedure
Creating a view that executes a stored procedure directly is not standard practice in SQL Server. Views are typically used to encapsulate complex queries or select statements, while stored procedures are designed for executing more complex logic and operations. However, there are workarounds to achieve similar results.
Understanding Limitations
- Views vs. Stored Procedures:
- Views are static and do not accept parameters.
- Stored procedures can include business logic and accept parameters, allowing for dynamic behavior.
- Execution Context:
- A view cannot directly execute a stored procedure. Instead, it can only reference tables or other views.
Alternative Approaches
While you cannot create a view that directly executes a stored procedure, consider the following alternatives:
- Using a Table-Valued Function:
- You can create a table-valued function that wraps the logic of your stored procedure and can be referenced in a view.
- Temporary Tables:
- You can execute the stored procedure into a temporary table and then create a view on that temporary table. However, this requires managing the lifecycle of the temporary table.
Example of Creating a Table-Valued Function
Assuming your stored procedure performs a query and returns a set of results, you can convert this logic into a table-valued function.
“`sql
CREATE FUNCTION dbo.uspGetResults()
RETURNS TABLE
AS
RETURN
(
SELECT * FROM YourSourceTable WHERE YourConditions
);
“`
You can then create a view based on this function:
“`sql
CREATE VIEW dbo.viewOnStoredProcedure AS
SELECT * FROM dbo.uspGetResults();
“`
Using Temporary Tables with Stored Procedures
This approach involves executing the stored procedure to populate a temporary table and then creating a view based on that temporary table.
“`sql
— Step 1: Execute stored procedure into a temporary table
CREATE TABLE TempResults (Column1 INT, Column2 NVARCHAR(50));
INSERT INTO TempResults
EXEC dbo.uspStoredProcedureTest;
— Step 2: Create a view based on the temporary table
CREATE VIEW dbo.ViewOnStoredProcedure AS
SELECT * FROM TempResults;
“`
Note: This view will only be usable within the same session as the temporary table exists, as temporary tables are session-specific.
Performance Considerations
When using these alternatives, keep the following in mind:
- Performance Overheads:
- Table-valued functions can impact performance if the underlying logic is complex.
- Temporary tables may lead to increased resource usage, especially in high-concurrency environments.
- Maintenance:
- Ensure that any changes in the logic of the stored procedure are mirrored in the table-valued function to maintain consistency.
By understanding these approaches and their limitations, you can effectively work with SQL Server to achieve your desired outcomes while adhering to best practices.
Expert Insights on Creating Views from Stored Procedures
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Creating a view based on a stored procedure, such as `exec dbo.uspstoredproceduretest`, can streamline data access and enhance performance. However, it is crucial to ensure that the stored procedure returns a consistent result set, as any changes in its output structure can lead to unexpected errors in the view.”
Michael Chen (Senior SQL Developer, Data Solutions Group). “Using `CREATE VIEW` to encapsulate the execution of a stored procedure is a powerful technique. It allows for improved security and abstraction, but developers must be cautious about the implications on performance and maintainability, especially in high-transaction environments.”
Linda Martinez (Data Management Consultant, Insight Analytics). “While it is technically feasible to create a view that executes a stored procedure, it is generally advisable to evaluate whether a view or a table-valued function would be more appropriate. Views are typically more efficient for static queries, while table-valued functions can provide the flexibility needed for dynamic data retrieval.”
Frequently Asked Questions (FAQs)
What is a view in SQL Server?
A view in SQL Server is a virtual table that provides a way to present data from one or more tables. It can encapsulate complex queries, allowing users to simplify data access and enhance security by restricting access to specific rows or columns.
Can a view be created from a stored procedure?
No, a view cannot be directly created from a stored procedure in SQL Server. Views are defined using a SELECT statement, while stored procedures can contain complex logic and multiple statements, including control-of-flow constructs.
What does the command `exec dbo.uspstoredproceduretest` do?
The command `exec dbo.uspstoredproceduretest` executes a stored procedure named `uspstoredproceduretest` located in the `dbo` schema. This command runs the procedure and returns any result sets or output parameters defined within it.
Is it possible to use a stored procedure’s result set in a view?
No, a stored procedure’s result set cannot be directly used in a view. However, you can create a table-valued function that returns a result set, which can then be used in a view.
What are the benefits of using views in SQL Server?
Views provide several benefits, including simplifying complex queries, enhancing security by limiting data exposure, providing a consistent interface for data access, and enabling easier maintenance of SQL code.
How do I create a view based on a SELECT statement?
To create a view based on a SELECT statement, use the following syntax: `CREATE VIEW view_name AS SELECT column1, column2 FROM table_name;`. This defines the view and specifies the data it will present.
Creating a view that executes a stored procedure, such as the example provided with `create view viewonstoredprocedure as exec dbo.uspstoredproceduretest`, is a topic that merits careful consideration in database management. While SQL Server allows the creation of views to simplify data retrieval, it is important to note that views are generally designed to encapsulate a SELECT statement. Executing a stored procedure directly within a view is not supported, as views cannot contain procedural logic or execute commands that produce side effects.
One of the key insights from this discussion is the distinction between views and stored procedures. Views are primarily used for presenting data in a specific format or structure, allowing users to query data without needing to understand the underlying complexities. In contrast, stored procedures are designed to perform operations, including data manipulation and complex business logic. Therefore, if the goal is to encapsulate the logic of a stored procedure, it may be more appropriate to use a stored procedure directly or consider alternatives such as table-valued functions or inline table-valued functions that can be utilized in a SELECT statement.
while the intention to create a view that executes a stored procedure reflects a desire to streamline database interactions, it is essential to adhere to the limitations and intended
Author Profile
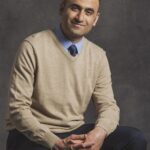
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?