How Do You Use the MM DD YYYY Date Format in SQL Server?
When it comes to managing and manipulating data in SQL Server, understanding date formats is crucial for ensuring accuracy and consistency. Among the various formats available, the `mm dd yyyy` format stands out as a common choice for representing dates in a way that is both human-readable and widely recognized. Whether you are developing a new database application, generating reports, or simply querying data, mastering this date format can significantly enhance your efficiency and effectiveness in working with temporal data.
In SQL Server, dates can be stored in various formats, but the challenge often lies in how to properly display or convert these dates to meet specific requirements. The `mm dd yyyy` format is particularly useful for applications that require a clear and straightforward representation of dates. Understanding how to manipulate and format dates in SQL Server not only helps in presenting data more clearly but also aids in avoiding common pitfalls associated with date handling, such as misinterpretation and errors in data entry.
As you delve deeper into the intricacies of date formatting in SQL Server, you will discover a range of functions and techniques that allow you to seamlessly convert and format dates to suit your needs. From basic queries to advanced formatting options, this exploration will equip you with the knowledge to effectively manage date-related data and ensure that your applications run smoothly and accurately. Get
Date Format in SQL Server: MM DD YYYY
In SQL Server, date formats can vary based on the settings of the server and the specific requirements of the application. The format `MM DD YYYY` is not a default format for SQL Server; however, it can be achieved through conversion functions and formatting options.
To convert a date to the `MM DD YYYY` format, you can use the `FORMAT` function in SQL Server. This function allows for a variety of formatting options, including the desired date format. Here’s an example of how to use it:
“`sql
SELECT FORMAT(GETDATE(), ‘MM dd yyyy’) AS FormattedDate;
“`
This query retrieves the current date and formats it as `MM DD YYYY`.
Using CONVERT for Date Formatting
Another method to format dates in SQL Server is by using the `CONVERT` function. The `CONVERT` function allows you to specify a style code that determines how the date will be formatted. For example, the style code `101` gives you the `MM/DD/YYYY` format, which can be modified to fit `MM DD YYYY` by replacing the slashes with spaces:
“`sql
SELECT REPLACE(CONVERT(VARCHAR, GETDATE(), 101), ‘/’, ‘ ‘) AS FormattedDate;
“`
This method provides flexibility for formatting dates while adhering to specific requirements.
Considerations for Date Formats
When working with date formats, several considerations should be taken into account:
- Regional Settings: The date format can vary based on the server’s regional settings. Always verify the server’s configuration before relying on a specific format.
- Data Type: Ensure that the column data type is `DATE`, `DATETIME`, or `DATETIME2` to avoid conversion errors.
- Storing Dates: It is generally advisable to store dates in their native format and convert them to the desired format only when displaying data.
Date Format Example Table
The following table illustrates different ways to format dates in SQL Server:
Method | Example Query | Output Format |
---|---|---|
FORMAT Function | SELECT FORMAT(GETDATE(), ‘MM dd yyyy’) | MM DD YYYY |
CONVERT Function | SELECT REPLACE(CONVERT(VARCHAR, GETDATE(), 101), ‘/’, ‘ ‘) | MM DD YYYY |
CAST Function | SELECT CAST(GETDATE() AS DATE) | YYYY-MM-DD |
By using these methods, you can effectively handle date formatting in SQL Server and ensure your applications present dates in a user-friendly manner.
Date Format in SQL Server: MM DD YYYY
To format dates in SQL Server as MM DD YYYY, you can utilize several built-in functions. The most common functions used for date formatting are `FORMAT()`, `CONVERT()`, and `CAST()`. Each function has its own syntax and capabilities.
Using the FORMAT() Function
The `FORMAT()` function is a versatile way to format date and time values. The syntax for formatting a date to MM DD YYYY is as follows:
“`sql
SELECT FORMAT(GETDATE(), ‘MM dd yyyy’) AS FormattedDate;
“`
- GETDATE() retrieves the current date and time.
- The format string ‘MM dd yyyy’ specifies the desired output format.
This will output the current date in the specified format.
Using the CONVERT() Function
The `CONVERT()` function is another method that allows for date formatting. The syntax can be a bit more complex, but it is widely used. Here’s how to use it for the MM DD YYYY format:
“`sql
SELECT CONVERT(VARCHAR(10), GETDATE(), 101) AS FormattedDate;
“`
- The 101 style code corresponds to the format MM/DD/YYYY. To convert it to MM DD YYYY, you can replace the slashes with spaces:
“`sql
SELECT REPLACE(CONVERT(VARCHAR(10), GETDATE(), 101), ‘/’, ‘ ‘) AS FormattedDate;
“`
This method effectively changes the slashes to spaces.
Using the CAST() Function
While the `CAST()` function does not directly format dates, you can combine it with string manipulation functions to achieve the desired format. Here’s an example:
“`sql
SELECT
RIGHT(‘0’ + CAST(MONTH(GETDATE()) AS VARCHAR(2)), 2) + ‘ ‘ +
RIGHT(‘0’ + CAST(DAY(GETDATE()) AS VARCHAR(2)), 2) + ‘ ‘ +
CAST(YEAR(GETDATE()) AS VARCHAR(4)) AS FormattedDate;
“`
This approach manually constructs the date string by:
- Extracting the month, day, and year separately.
- Using RIGHT() to ensure two-digit formats for month and day.
Considerations for Date Formatting
When working with date formats in SQL Server, consider the following:
- Regional Settings: SQL Server settings may affect how dates are interpreted.
- Data Types: Ensure the input date is of a compatible type (e.g., `DATE`, `DATETIME`, `DATETIME2`).
- Performance: Format conversion can impact performance; consider storing dates in a standard format and formatting them only when necessary for display.
Example Table of Date Formats
Format Type | SQL Syntax Example | Result Example |
---|---|---|
FORMAT | `FORMAT(GETDATE(), ‘MM dd yyyy’)` | 10 05 2023 |
CONVERT | `REPLACE(CONVERT(VARCHAR(10), GETDATE(), 101), ‘/’, ‘ ‘)` | 10 05 2023 |
Manual Construction | `RIGHT(‘0’ + CAST(MONTH(GETDATE()) AS VARCHAR(2)), 2) + ‘ ‘ + RIGHT(‘0’ + CAST(DAY(GETDATE()) AS VARCHAR(2)), 2) + ‘ ‘ + CAST(YEAR(GETDATE()) AS VARCHAR(4))` | 10 05 2023 |
Utilizing these functions and methods, you can efficiently format dates in SQL Server to meet your specific requirements.
Understanding Date Formats in SQL Server: Expert Insights
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When working with SQL Server, it is crucial to understand the various date formats available. The ‘mm dd yyyy’ format is not natively supported in SQL Server, as it typically uses ‘yyyy-mm-dd’ for ISO compliance. However, you can convert dates to the desired format using the FORMAT function or CONVERT function, which allows for greater flexibility in date representation.”
Michael Thompson (Senior SQL Developer, DataWise Analytics). “In SQL Server, handling date formats effectively is essential for data integrity. While ‘mm dd yyyy’ can be achieved through string manipulation, it is advisable to store dates in a standard format and convert them only when necessary for display purposes. This practice enhances performance and reduces errors in date calculations.”
Lisa Nguyen (Data Management Consultant, Insight Strategies). “The ‘mm dd yyyy’ date format can lead to confusion, especially in international contexts. SQL Server provides robust functions like FORMAT and CONVERT that can help format dates as needed. However, I recommend always using the standard ‘yyyy-mm-dd’ format for storage, as it simplifies queries and ensures compatibility across different systems.”
Frequently Asked Questions (FAQs)
What is the default date format in SQL Server?
The default date format in SQL Server is determined by the language setting of the session. Common formats include ‘YYYY-MM-DD’ for ISO format and ‘MM/DD/YYYY’ for U.S. English settings.
How can I convert a date to MM DD YYYY format in SQL Server?
You can convert a date to MM DD YYYY format using the `FORMAT` function: `FORMAT(your_date_column, ‘MM dd yyyy’)`. Alternatively, you can use `CONVERT` with style 101: `CONVERT(VARCHAR, your_date_column, 101)`.
What SQL Server function can I use to get the current date in MM DD YYYY format?
To get the current date in MM DD YYYY format, use: `SELECT FORMAT(GETDATE(), ‘MM dd yyyy’) AS CurrentDate`. This will return the current date formatted as specified.
Can I change the default date format in SQL Server?
Yes, you can change the default date format by setting the language for the session using `SET LANGUAGE` or by modifying the server’s default language settings. However, it is recommended to use explicit formatting in queries for consistency.
What happens if I insert a date in MM DD YYYY format without proper conversion?
Inserting a date in MM DD YYYY format without proper conversion may lead to errors or incorrect data being stored, depending on the server’s language and date format settings. Always ensure dates are in a recognized format.
Is it possible to store dates in MM DD YYYY format in SQL Server?
SQL Server stores dates in a binary format regardless of how they are displayed. You can format the output as MM DD YYYY when retrieving data, but the internal storage remains in a standard date format.
In SQL Server, the date format can vary based on the requirements of the application and the context in which the date is being used. The format “mm dd yyyy” is not a standard output format in SQL Server; however, it can be achieved through various methods, including the use of the CONVERT function. This function allows users to specify the desired date format by using a style code, which can convert dates into a string representation in the specified format.
It is essential to understand that the default date format in SQL Server is influenced by the server’s language and regional settings. Therefore, when working with dates, it is crucial to ensure that the format aligns with the expectations of the users or the application. Using the correct format helps prevent errors in data interpretation and enhances the clarity of date-related information.
Additionally, it is advisable to use the ISO 8601 format (YYYY-MM-DD) when storing or comparing dates in SQL Server. This format is unambiguous and avoids issues related to regional settings. When displaying dates, however, formatting functions like FORMAT or CONVERT can be utilized to present the date in a more user-friendly manner, such as “mm dd yyyy,” when necessary.
In summary,
Author Profile
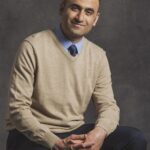
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?