How Do You Declare a Constant in Java?
In the world of programming, constants play a crucial role in maintaining the integrity and clarity of your code. When working with Java, understanding how to declare a constant is fundamental for any developer looking to write clean, efficient, and maintainable code. Constants are values that remain unchanged throughout the execution of a program, providing a reliable reference point that can enhance readability and reduce the risk of errors. Whether you’re developing a simple application or a complex system, mastering the declaration of constants in Java is a skill that can significantly elevate your coding prowess.
Declaring a constant in Java is straightforward, yet it carries significant implications for how your code behaves. By using the `final` keyword, you can create variables that cannot be reassigned once initialized, ensuring that the value remains constant throughout the program. This practice not only helps prevent accidental modifications but also communicates to other developers that certain values are intended to remain unchanged, fostering better collaboration and understanding within teams.
Moreover, constants can be declared at various levels within your Java application, from local constants within methods to class-level constants that can be accessed throughout your codebase. Utilizing constants effectively can lead to more organized and efficient code, allowing developers to make changes in one place without the risk of unintended consequences elsewhere. As we delve deeper into the specifics of
Declaring Constants in Java
In Java, constants are typically declared using the `final` keyword. A constant is a variable whose value cannot be changed once it is assigned. This is particularly useful in cases where a fixed value is needed throughout the program, promoting code clarity and preventing accidental modifications.
To declare a constant, you follow the general syntax:
“`java
final dataType CONSTANT_NAME = value;
“`
Where:
- `dataType` specifies the type of the constant (e.g., `int`, `double`, `String`).
- `CONSTANT_NAME` is a name that should be in uppercase letters by convention.
- `value` is the initial value assigned to the constant.
Example of Declaring Constants
“`java
final int MAX_USERS = 100;
final double PI = 3.14159;
final String COMPANY_NAME = “Tech Innovations”;
“`
Benefits of Using Constants
- Immutability: Once assigned, the value cannot be changed, ensuring stability.
- Readability: Using descriptive constant names makes the code easier to understand.
- Maintainability: Changes to constant values need to be made in only one place, reducing errors.
Constant Naming Conventions
- Use uppercase letters for constant names.
- Separate words with underscores for better readability.
Example of Naming Convention
“`java
final int MAX_CONNECTIONS = 50;
final String DEFAULT_COLOR = “Blue”;
“`
Using Constants in Code
Constants can be used in expressions, loops, and method parameters, making them versatile. Below is a simple example demonstrating their use in a method:
“`java
public class Application {
final static int MAX_ATTEMPTS = 3;
public static void main(String[] args) {
for (int attempt = 1; attempt <= MAX_ATTEMPTS; attempt++) {
System.out.println("Attempt number: " + attempt);
}
}
}
```
Constant Types
Constants can be categorized into various types based on their data types:
Constant Type | Example Declaration | Description |
---|---|---|
Integer | final int DAYS_IN_WEEK = 7; | Holds a fixed integer value. |
Double | final double GRAVITY = 9.81; | Holds a constant floating-point number. |
String | final String APP_NAME = “My Application”; | Holds a fixed string value. |
By utilizing constants effectively, developers can enhance the integrity of their applications, ensuring that vital values remain unchanged throughout the execution of the program.
Declaring a Constant in Java
In Java, constants are typically declared using the `final` keyword. This indicates that the value of the variable cannot be changed once it has been initialized. Constants are often used for values that are meant to remain unchanged throughout the program, such as mathematical constants, configuration settings, or fixed values.
Syntax for Declaring Constants
The basic syntax for declaring a constant in Java is as follows:
“`java
final dataType CONSTANT_NAME = value;
“`
- dataType: The type of the constant (e.g., `int`, `double`, `String`).
- CONSTANT_NAME: The name of the constant, which is conventionally written in uppercase letters with words separated by underscores.
- value: The value assigned to the constant at the time of declaration.
Example of Constant Declaration
Here is an example demonstrating the declaration of constants in Java:
“`java
final int MAX_USERS = 100;
final double PI = 3.14159;
final String APP_NAME = “MyApplication”;
“`
In this example:
- `MAX_USERS` is an integer constant set to 100.
- `PI` is a double constant representing the value of Pi.
- `APP_NAME` is a String constant that holds the name of the application.
Best Practices for Using Constants
When declaring constants, consider the following best practices:
- Use descriptive names that convey the purpose of the constant.
- Follow naming conventions (uppercase letters and underscores).
- Group related constants together, possibly in an interface or class.
- Document the purpose and usage of constants for clarity.
Constants in Interfaces
In Java, constants can also be defined within interfaces. All fields in an interface are implicitly `public`, `static`, and `final`, making them constants by default. For example:
“`java
public interface AppConstants {
int MAX_USERS = 100;
String DEFAULT_LANGUAGE = “English”;
}
“`
This allows for easy access to constants across different classes that implement the interface.
Comparison with Variables
Feature | Constant | Variable |
---|---|---|
Declaration | `final dataType name = value;` | `dataType name = value;` |
Mutability | Immutable | Mutable |
Scope | Can be local or static | Can be local, instance, or static |
Naming | All uppercase with underscores | Camel case |
Constants play a crucial role in improving code readability and maintainability by providing meaningful names to fixed values, reducing the likelihood of errors related to hard-coded values.
Understanding Constant Declaration in Java: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Declaring a constant in Java is achieved using the `final` keyword, which ensures that the variable cannot be reassigned after its initial value is set. This is crucial for maintaining the integrity of values that should remain unchanged throughout the execution of a program.”
James Liu (Java Developer Advocate, CodeCraft). “When you declare a constant in Java, it is a best practice to also use the `static` modifier if the constant is meant to be shared across all instances of a class. This combination of `static final` allows for efficient memory usage and enhances readability in your code.”
Sarah Thompson (Lead Java Instructor, Dev Academy). “Constants in Java are typically declared in uppercase letters with underscores separating words, which follows the naming conventions of the Java community. This approach not only improves code clarity but also helps developers quickly identify constants within the codebase.”
Frequently Asked Questions (FAQs)
What is a constant in Java?
A constant in Java is a variable whose value cannot be changed once it is assigned. Constants are defined using the `final` keyword.
How do you declare a constant in Java?
To declare a constant in Java, use the `final` keyword followed by the data type, the variable name, and the value. For example: `final int MAX_VALUE = 100;`.
Can a constant be modified after declaration?
No, once a constant is declared using the `final` keyword, its value cannot be modified. Any attempt to change it will result in a compilation error.
What are the naming conventions for constants in Java?
Constants are typically named using uppercase letters with underscores separating words. For example: `MAX_LENGTH`, `DEFAULT_TIMEOUT`.
Can constants be declared at class level in Java?
Yes, constants can be declared at the class level, making them accessible to all methods within the class. They can also be declared as `static` to be shared across all instances.
Are there any performance benefits to using constants in Java?
Yes, using constants can improve performance and readability. Constants can help the compiler optimize code and prevent accidental changes to values that should remain fixed.
In Java, declaring a constant is achieved using the `final` keyword. When a variable is declared as `final`, it indicates that its value cannot be modified once it has been assigned. This characteristic is crucial for maintaining the integrity of values that should remain unchanged throughout the program, such as mathematical constants or configuration settings.
Constants in Java are typically declared using uppercase letters with underscores separating words, following the naming convention for readability. For instance, a constant representing the value of Pi can be declared as `public static final double PI = 3.14159;`. This declaration not only signifies that the value of PI is constant but also makes it accessible across the class or application if declared as `public static`.
Using constants effectively enhances code clarity and maintainability. It helps prevent accidental changes to important values, thereby reducing bugs and improving the overall robustness of the application. Moreover, utilizing constants can lead to better performance, as the Java compiler can optimize the usage of these immutable values during runtime.
Author Profile
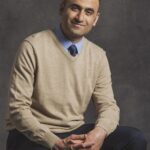
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?