How Can I Use DevExtreme to Implement a Confirmation Dialog with a Text Field?
In today’s fast-paced digital landscape, user experience is paramount, and developers are constantly seeking innovative ways to engage users effectively. One such tool that has gained traction is the DevExtreme library, renowned for its robust UI components that streamline application development. Among these components, the confirm dialog stands out as a vital feature, allowing developers to prompt users for confirmation before executing critical actions. But what if you could elevate this functionality by incorporating a text field into the confirm dialog? This article explores the exciting possibilities of using a text field within the DevExtreme confirm dialog, enhancing interactivity and user input in your applications.
Overview of DevExtreme Confirm Dialogs
The DevExtreme confirm dialog is a versatile component that provides a straightforward way to solicit user confirmation. Traditionally, it presents users with a simple message and options to accept or cancel an action. However, integrating a text field into this dialog transforms it into a more dynamic tool, enabling users to provide additional context or information before proceeding. This enhancement not only improves user engagement but also allows developers to gather valuable input directly from the user interface.
By leveraging the capabilities of DevExtreme, developers can customize the confirm dialog to suit their application’s specific needs. The addition of a text field opens up a realm of possibilities, from gathering feedback to
Implementation of DevExtreme Confirm with Text Field
To enhance user interaction within your web application, integrating a confirm dialog with a text field using DevExtreme can be a powerful tool. This allows users to input additional information before confirming an action, providing a more dynamic and engaging experience.
Creating a Basic Confirm Dialog
To create a confirm dialog with a text field, you first need to set up a basic DevExtreme confirm dialog. Here’s how you can do that:
- Ensure you have included the necessary DevExtreme libraries in your project.
- Use the `DevExpress.ui.dialog.confirm` method to create a dialog.
Here’s a basic example:
“`javascript
function showConfirmDialog() {
DevExpress.ui.dialog.confirm(“Please enter your details:”, “Confirmation”).done(function (result) {
if (result) {
// Handle confirmation
}
});
}
“`
This code snippet initializes a confirmation dialog with a message. However, we will extend this to include a text field.
Integrating a Text Field
To include a text input field in the confirm dialog, you need to manipulate the dialog’s content. You can achieve this by using the `content` option to insert HTML elements directly.
“`javascript
function showConfirmWithInput() {
const inputHtml = ‘‘;
DevExpress.ui.dialog.custom({
title: “Confirmation”,
messageHtml: inputHtml,
buttons: [
{ text: “Cancel”, onClick: function() { /* handle cancel */ }},
{ text: “Confirm”, onClick: function() {
const userInput = document.getElementById(“userInput”).value;
// Handle user input
}}
]
});
}
“`
In this code, we created a custom dialog that includes an input field. The `messageHtml` property allows for the inclusion of HTML, enabling you to place an input field for user interaction.
Styling the Input Field
It’s essential to ensure that the input field is styled appropriately to match your application’s design. You can achieve this by applying CSS styles. Here’s an example of basic styling:
“`css
userInput {
width: 100%;
padding: 8px;
margin-top: 10px;
border: 1px solid ccc;
border-radius: 4px;
}
“`
This CSS will make the input field more user-friendly and aesthetically pleasing.
Example of a Complete Dialog with User Input
Below is a complete example that combines all the elements discussed:
“`javascript
function showConfirmWithInput() {
const inputHtml = ‘‘;
DevExpress.ui.dialog.custom({
title: “Confirmation”,
messageHtml: inputHtml,
buttons: [
{ text: “Cancel”, onClick: function() { /* handle cancel */ }},
{ text: “Confirm”, onClick: function() {
const userInput = document.getElementById(“userInput”).value;
alert(“User input: ” + userInput); // Handle user input
}}
]
});
}
“`
Considerations for User Experience
When designing a confirm dialog with a text field, consider the following:
- Input Validation: Ensure that user input is validated to prevent errors.
- Accessibility: Make sure that the input field is accessible to all users, including those using screen readers.
- Feedback: Provide immediate feedback upon user interaction to improve usability.
Consideration | Description |
---|---|
Input Validation | Check for required fields and valid formats. |
Accessibility | Ensure compatibility with assistive technologies. |
Feedback | Notify users of successful or erroneous input. |
Integrating a confirm dialog with a text field in DevExtreme enhances user experience by allowing for more interactive and meaningful confirmations.
Implementing DevExtreme Confirm Dialog with Text Field
To enhance user interaction in your applications, integrating a confirmation dialog that includes a text field can be highly effective. This allows users to provide additional information before confirming their actions.
Configuration of DevExtreme Confirm Dialog
To create a confirmation dialog with a text field, you can utilize the `DevExpress.ui.dialog` component. This component can be customized to include a text input field alongside the standard confirmation buttons.
“`javascript
function showConfirmDialog() {
const dialog = DevExpress.ui.dialog.custom({
title: “Confirm Action”,
messageHtml: `
`,
buttons: [
{
text: “Cancel”,
onClick: () => { dialog.hide(); }
},
{
text: “Confirm”,
onClick: () => {
const inputValue = document.getElementById(“confirmInput”).value;
handleConfirm(inputValue);
dialog.hide();
}
}
]
});
dialog.show();
}
function handleConfirm(inputValue) {
// Handle the confirmation with the provided input value
console.log(“Confirmed with input:”, inputValue);
}
“`
Key Features of the Custom Dialog
- Customizable Message: The dialog can contain HTML, allowing for rich text formatting and additional elements like inputs.
- Callback Functions: You can define actions to be executed based on user input upon confirmation.
- Dynamic Input Handling: The input value can be accessed directly in the confirmation handler for further processing.
Styling the Text Field
For better user experience, you may want to style the text input field. Applying CSS can help in aligning the input field with the overall design of your application. Here’s a basic example:
“`css
confirmInput {
width: 100%;
padding: 8px;
margin-top: 10px;
border: 1px solid ccc;
border-radius: 4px;
}
“`
Considerations for User Experience
When implementing a confirmation dialog with a text field, keep the following points in mind:
- Validation: Consider validating the input before allowing the confirmation action. This prevents errors caused by invalid or empty inputs.
- Accessibility: Ensure that the dialog is accessible. Implement keyboard navigations and screen reader support.
- Responsiveness: Design the dialog to be responsive, ensuring it displays well on various devices.
Example of Usage
Here is an example scenario where you might use this dialog:
Action | Description |
---|---|
Deleting an Item | Prompt the user to confirm the deletion and provide a reason. |
Submitting Feedback | Ask the user to confirm their feedback and allow them to clarify their comments. |
In these scenarios, the confirmation dialog not only confirms actions but also collects essential user input, enhancing functionality and improving user engagement.
Expert Insights on Implementing DevExtreme Confirm with Text Field
Maria Chen (Senior Frontend Developer, Tech Innovations Inc.). “Integrating a text field within the DevExtreme confirm dialog enhances user interactivity. It allows users to provide additional context or feedback directly related to their confirmation, making the process more intuitive and user-friendly.”
James Patel (UI/UX Designer, Creative Solutions Group). “Using a text field in the DevExtreme confirm dialog can significantly improve the user experience. It not only captures user input effectively but also allows for a more dynamic interaction, enabling users to express their thoughts or concerns before finalizing their decisions.”
Linda Gomez (Software Engineer, Digital Experience Agency). “From a technical perspective, implementing a text field in the DevExtreme confirm dialog requires careful consideration of validation and data handling. Ensuring that the input is properly sanitized and managed will prevent potential issues and enhance overall application security.”
Frequently Asked Questions (FAQs)
What is DevExtreme confirm with a text field?
DevExtreme confirm with a text field is a dialog component that prompts users for confirmation while allowing them to enter additional information through an input field. This feature enhances user interaction by collecting necessary data during confirmation processes.
How do I implement a confirm dialog with a text field in DevExtreme?
To implement a confirm dialog with a text field, you can use the `DevExpress.ui.dialog.confirm` method, providing a custom template that includes an input element. This allows you to capture user input alongside the confirmation action.
Can I customize the appearance of the confirm dialog in DevExtreme?
Yes, the appearance of the confirm dialog can be customized using CSS and by modifying the dialog options such as title, message, and buttons. You can also create a custom template for more extensive modifications.
Is it possible to validate the input field in the confirm dialog?
Yes, you can validate the input field by implementing custom validation logic before the dialog is closed. This ensures that the user provides valid input before proceeding with the confirmation action.
What events can I handle when using the confirm dialog with a text field?
You can handle various events such as `onShown`, `onHidden`, and `onButtonClick`. These events allow you to execute specific actions based on user interactions with the dialog.
Can I retrieve the input value from the text field after confirmation?
Yes, you can retrieve the input value after confirmation by accessing the input element within the dialog’s callback function. This allows you to use the entered data for further processing or actions.
DevExtreme provides a versatile confirmation dialog that can be customized to include various components, including text fields. This functionality allows developers to create more interactive and user-friendly confirmation prompts, where users can input information before confirming an action. By integrating a text field into the confirmation dialog, applications can gather essential data from users, enhancing the overall user experience and ensuring that the confirmation process is both informative and efficient.
One of the key advantages of using a confirmation dialog with a text field is the ability to collect user input directly related to the action being confirmed. For example, if a user is about to delete an item, the dialog can prompt them to provide a reason for the deletion. This not only adds a layer of accountability but also allows developers to implement logic based on the user’s input, such as logging the reason or preventing the action if certain criteria are not met.
Moreover, implementing this feature in DevExtreme is straightforward, thanks to its robust API and documentation. Developers can easily customize the dialog’s appearance and behavior, ensuring that it aligns with the overall design of their application. This flexibility allows for a seamless integration of the confirmation dialog with text fields, making it a powerful tool for enhancing user interactions within web applications.
Author Profile
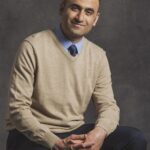
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?