What Is a Do While Statement in JavaScript and How Does It Work?
### Introduction
In the world of programming, control flow is a fundamental concept that dictates how a program executes its instructions. Among the various control flow structures available in JavaScript, the `do while` statement stands out for its unique approach to looping. Unlike other loop constructs, the `do while` statement guarantees that the block of code within it will execute at least once, regardless of the condition that follows. This feature makes it particularly useful in scenarios where an initial action is necessary before any condition can be evaluated.
As we delve deeper into the `do while` statement, we will explore its syntax, functionality, and practical applications. This looping mechanism not only enhances the versatility of your code but also allows for more intuitive programming in certain situations. By understanding how to effectively implement the `do while` statement, you can write cleaner, more efficient JavaScript that responds dynamically to user inputs and other conditions.
Join us as we unpack the intricacies of the `do while` statement, providing you with the knowledge to leverage this powerful tool in your coding arsenal. Whether you are a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, this exploration will equip you with the insights needed to enhance your JavaScript programming capabilities.
Understanding the Do While Statement in JavaScript
The `do while` statement in JavaScript is a type of loop that executes a block of code at least once before checking a specified condition. This is particularly useful when the initial execution of code is necessary irrespective of the condition’s truth value. The syntax for the `do while` statement is as follows:
javascript
do {
// code block to be executed
} while (condition);
In this structure, the code inside the `do` block will run first, followed by a check of the `condition`. If the condition evaluates to `true`, the loop will continue to execute; if it evaluates to “, the loop will terminate.
Key Characteristics of the Do While Statement
- Guaranteed Execution: The most significant feature of a `do while` loop is that it guarantees at least one execution of the code block, regardless of the condition.
- Post-Condition Check: The condition is evaluated after the execution of the code block, distinguishing it from the regular `while` loop where the condition is evaluated before the code block executes.
- Incremental Updates: It is common to include an update to the condition variable within the loop to eventually terminate the loop.
Example of a Do While Loop
To illustrate the `do while` statement, consider the following example where we want to prompt a user to enter a positive number:
javascript
let number;
do {
number = prompt(“Please enter a positive number:”);
} while (number <= 0);
In this example, the loop will continue prompting the user until they enter a positive number. The prompt will be displayed at least once, regardless of the initial input.
Comparison with Other Loop Constructs
The `do while` loop can be compared with other looping constructs such as the `while` loop and `for` loop. Below is a summary of their differences:
Loop Type | Execution Guarantee | Condition Check |
---|---|---|
Do While | At least once | After execution |
While | Zero or more times | Before execution |
For | Zero or more times | Before execution |
Best Practices for Using Do While Loops
When implementing `do while` loops in JavaScript, consider the following best practices:
- Initialize Variables: Ensure that all variables used in the condition are initialized before entering the loop.
- Modify Condition Variables: Update any variables involved in the loop’s condition within the loop to avoid infinite loops.
- Keep Conditions Simple: Use straightforward conditions to improve readability and maintainability.
- Consider Alternatives: If the logic does not require guaranteed execution, evaluate if a `while` or `for` loop may be more appropriate.
By adhering to these guidelines, developers can effectively utilize `do while` statements in their JavaScript programs, ensuring clarity and correctness in control flow.
Understanding the `do while` Statement in JavaScript
The `do while` statement is a control flow structure that executes a block of code at least once before evaluating a specified condition. This guarantees that the code inside the block runs before the condition is tested. The syntax of the `do while` loop is as follows:
javascript
do {
// Code to be executed
} while (condition);
This structure is particularly useful when the initial execution of the loop is required, regardless of whether the condition holds true.
Key Features of the `do while` Statement
- Guaranteed Execution: The block of code within the `do` statement is executed at least once, making it ideal for scenarios where the initial execution is necessary.
- Condition Evaluation: The condition is evaluated after the block of code is executed. If the condition is true, the loop iterates again; if , the loop terminates.
- Usage Scenarios: Commonly used for user input validation, menu systems, and situations where the user must be prompted for input at least once.
Example of a `do while` Loop
Here is a practical example demonstrating a `do while` loop that prompts the user for a number until they enter a positive value:
javascript
let number;
do {
number = prompt(“Please enter a positive number:”);
} while (number <= 0);
console.log("You entered a positive number: " + number);
In this example:
- The user is prompted to enter a number.
- The loop continues until the user enters a number greater than zero.
Differences Between `while` and `do while` Loops
Feature | `while` Loop | `do while` Loop |
---|---|---|
Execution Guarantee | May not execute if condition is | Executes at least once |
Condition Check | Before executing the loop | After executing the loop |
Syntax | `while (condition) { … }` | `do { … } while (condition);` |
The choice between using a `while` and a `do while` loop depends on whether you need the loop to execute at least once.
Common Mistakes to Avoid
- Infinite Loops: Ensure that the loop condition will eventually evaluate to . If the condition remains true indefinitely, the loop will run forever.
- Data Type Mismatch: Be cautious of data types when evaluating conditions. For example, comparing a number with a string can lead to unexpected behavior.
- Uninitialized Variables: Ensure that any variables used in the condition are properly initialized before the loop begins to avoid reference errors.
By adhering to these guidelines, you can effectively implement the `do while` statement in your JavaScript code, leveraging its unique properties to suit your programming needs.
Understanding the Do While Statement in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The do while statement in JavaScript is particularly useful for scenarios where the code block needs to execute at least once before any condition is evaluated. This is crucial in applications where user input is required immediately, ensuring that the prompt is displayed before any validation checks.”
Michael Chen (JavaScript Framework Developer, CodeCraft Labs). “In my experience, the do while loop can simplify code readability in certain cases, especially when dealing with user interactions. It allows developers to clearly express the intent of executing a block of code repeatedly until a specific condition is met, which can enhance maintainability.”
Sarah Thompson (JavaScript Educator, Digital Learning Academy). “Teaching the do while statement to beginners can be pivotal in their understanding of control flow in programming. It serves as an excellent example of how loops can function differently based on their structure, contrasting it with the traditional while loop to highlight its unique behavior.”
Frequently Asked Questions (FAQs)
What is a do while statement in JavaScript?
The `do while` statement is a control flow statement that executes a block of code at least once and then repeatedly executes the block as long as a specified condition evaluates to true.
How does a do while statement differ from a while statement?
The primary difference is that a `do while` statement guarantees that the code block will execute at least once, whereas a `while` statement may not execute at all if the condition is at the outset.
What is the syntax of a do while statement in JavaScript?
The syntax is as follows:
javascript
do {
// code block to be executed
} while (condition);
Can you provide an example of a do while statement in action?
Certainly. Here’s a simple example:
javascript
let count = 0;
do {
console.log(count);
count++;
} while (count < 5);
This code will log numbers 0 through 4 to the console.
What are common use cases for a do while statement?
Common use cases include scenarios where user input is required, such as prompting a user until valid input is received, or executing a loop where the initial execution is necessary before checking the condition.
Are there any limitations or considerations when using a do while statement?
Yes, one should be cautious of infinite loops if the condition never becomes . Properly managing the condition and ensuring that the loop will eventually terminate is essential to avoid performance issues.
The “do while” statement in JavaScript is a control flow structure that allows for repeated execution of a block of code as long as a specified condition remains true. This statement is distinct from the traditional “while” loop in that it guarantees at least one execution of the code block, regardless of the condition’s initial state. The syntax consists of the “do” keyword followed by a block of code and the “while” keyword with a condition, ensuring that the loop continues until the condition evaluates to .
One of the key advantages of using a “do while” loop is its ability to handle scenarios where the execution of the code block must occur at least once before any condition checks. This characteristic makes it particularly useful in situations such as user input validation, where the program should prompt the user for input at least once before determining if the input meets certain criteria.
In summary, the “do while” statement is a powerful tool in JavaScript for managing repetitive tasks with a guaranteed initial execution. Its unique structure allows developers to create more intuitive and user-friendly applications by ensuring that critical code runs before any conditions are evaluated. Understanding the nuances of this control structure can significantly enhance a developer’s ability to write effective and efficient JavaScript code.
Author Profile
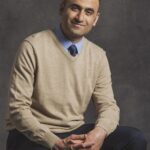
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?