Does Python Have Arrays? Unraveling the Truth Behind Python’s Data Structures
When it comes to programming languages, Python is often celebrated for its simplicity and versatility. As developers dive into the world of Python, one common question arises: “Does Python have arrays?” This inquiry opens up a broader conversation about data structures and how Python manages collections of data. Understanding the nuances of arrays and their alternatives in Python can significantly enhance your coding efficiency and effectiveness.
At first glance, the concept of arrays may seem straightforward, especially for those coming from languages like C or Java, where arrays are a fundamental part of the syntax. However, Python approaches data storage with a unique perspective that prioritizes flexibility and ease of use. While traditional arrays may not be a built-in feature of Python, the language offers powerful alternatives that serve similar purposes, allowing developers to handle collections of data in a variety of ways.
In this article, we will explore the intricacies of Python’s data structures, focusing on lists, tuples, and the array module. We’ll delve into their characteristics, advantages, and use cases, providing you with a comprehensive understanding of how to effectively manage and manipulate data in Python. Whether you’re a beginner or an experienced programmer, this exploration will equip you with the knowledge to make informed decisions about the best data structures for your projects.
Understanding Arrays in Python
While Python does not have a built-in array data type like some other programming languages, it does provide several ways to work with array-like structures. The most common alternatives are lists and the array module, as well as NumPy arrays for numerical data.
Python Lists
Python lists are one of the most versatile data structures available in the language. They can store a collection of items, which can be of different types, including integers, floats, strings, and even other lists. Lists are dynamic, meaning they can grow or shrink in size.
Key characteristics of Python lists include:
- Dynamic sizing: Lists can grow or shrink as needed.
- Heterogeneous elements: Lists can contain items of different data types.
- Mutability: Lists can be modified after creation (adding, removing, or changing elements).
Example of creating and manipulating a list:
python
my_list = [1, 2, 3, 4]
my_list.append(5) # Adding an element
my_list[0] = 0 # Modifying an element
The array Module
For situations where you specifically need array-like behavior with more constraints on data types, Python provides the `array` module. The `array` type is more efficient in terms of memory than lists when dealing with large amounts of numerical data, as it stores items in a more compact format.
Key features of the `array` module include:
- Type constraints: You must specify the type of elements the array will hold, ensuring homogeneity.
- Less overhead: Arrays consume less memory compared to lists.
Here’s how to create an array using the `array` module:
python
import array as arr
my_array = arr.array(‘i’, [1, 2, 3, 4]) # ‘i’ indicates the array will contain integers
NumPy Arrays
For more advanced numerical operations and larger datasets, the NumPy library is the preferred option in Python. NumPy arrays provide a powerful N-dimensional array object, which facilitates efficient mathematical computations.
Benefits of using NumPy arrays:
- Performance: NumPy arrays are optimized for performance, especially for large datasets.
- Multidimensional support: They support multidimensional arrays, which can be beneficial for various applications, such as data science and machine learning.
- Rich functionality: NumPy provides a wide range of mathematical functions for array operations.
Example of creating a NumPy array:
python
import numpy as np
my_numpy_array = np.array([1, 2, 3, 4])
Comparison Table
Feature | Python List | Array Module | NumPy Array |
---|---|---|---|
Data Type | Heterogeneous | Homogeneous | Homogeneous |
Memory Efficiency | Less efficient | More efficient | Highly efficient |
Performance | Slow for large datasets | Faster than lists | Fastest for numerical computations |
Dimensionality | 1D | 1D | Multi-dimensional |
In summary, while Python does not have a native array type, it offers several alternatives, each suited to different types of tasks, from simple data storage with lists to advanced numerical operations with NumPy arrays.
Understanding Arrays in Python
Python does not have a built-in array data structure in the same way that languages like C or Java do. Instead, Python provides several options that can serve similar purposes, primarily through lists and the `array` module.
Python Lists as Arrays
Lists are the most commonly used data structure for holding ordered collections of items in Python. They can contain elements of different data types, making them flexible and versatile.
- Creation: Lists are created using square brackets.
python
my_list = [1, 2, 3, ‘four’, 5.0]
- Accessing Elements: Elements are accessed using zero-based indexing.
python
first_element = my_list[0] # 1
- Common Operations:
- Appending: `my_list.append(6)`
- Removing: `my_list.remove(‘four’)`
- Slicing: `sub_list = my_list[1:4]`
Using the Array Module
For scenarios requiring a more traditional array structure, Python provides the `array` module, which offers a space-efficient representation of basic data types.
- Importing the Module:
python
import array
- Creating an Array: Arrays require a type code to define the data type of the elements.
python
int_array = array.array(‘i’, [1, 2, 3, 4, 5]) # ‘i’ for integers
- Key Characteristics:
- Homogeneous: All elements must be of the same type.
- Type Codes: Common type codes include:
Type Code | C Type | Python Type |
---|---|---|
‘i’ | int | int |
‘f’ | float | float |
‘d’ | double | float |
NumPy Arrays
For numerical computations, the NumPy library provides a powerful array object known as `ndarray`. This is particularly useful for handling large datasets and performing mathematical operations.
- Installation:
bash
pip install numpy
- Creating a NumPy Array:
python
import numpy as np
numpy_array = np.array([1, 2, 3, 4, 5])
- Features:
- Multidimensional: Supports arrays of any dimension.
- Vectorized Operations: Allows for operations on entire arrays without explicit loops.
- Broadcasting: Facilitates arithmetic operations between arrays of different shapes.
Performance Considerations
When choosing between lists, arrays, and NumPy arrays, the decision should be based on specific use cases:
- Lists:
- Pros: Flexible, easy to use.
- Cons: Slower for numerical operations due to type heterogeneity.
- Array Module:
- Pros: More memory efficient than lists for large datasets of the same type.
- Cons: Limited to one-dimensional and homogeneous data.
- NumPy Arrays:
- Pros: Highly efficient for numerical computations, supports advanced mathematical functions.
- Cons: Requires external library and may have a steeper learning curve for beginners.
Understanding Arrays in Python: Expert Insights
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “While Python does not have a built-in array data type like some other programming languages, it offers several alternatives such as lists and the array module, which can be used for similar functionalities. For numerical computations, libraries like NumPy provide powerful array-like structures that are essential for data analysis.”
James Liu (Software Engineer, Python Development Group). “In Python, the term ‘array’ can be somewhat misleading. The native list type can serve many purposes of an array, but for performance-critical applications, developers often turn to the array module or NumPy arrays, which are optimized for numerical operations and memory efficiency.”
Sarah Thompson (Computer Science Educator, Online Learning Platform). “Understanding that Python’s lists are dynamic and can hold mixed data types is crucial for beginners. However, when it comes to numerical data, using the array module or NumPy is advisable, as they provide more functionality and efficiency, particularly for large datasets.”
Frequently Asked Questions (FAQs)
Does Python have arrays?
Python does not have a built-in array data type like some other programming languages. However, it provides several alternatives, such as lists and the array module.
What is the difference between a list and an array in Python?
A list in Python is a versatile, dynamic data structure that can hold mixed data types. An array, specifically from the array module, is more efficient for numerical data and requires all elements to be of the same type.
How do I create an array in Python?
To create an array in Python, you can use the `array` module by importing it and then initializing an array with a specified type code, such as `array(‘i’, [1, 2, 3])` for integers.
Are there libraries in Python that provide array functionality?
Yes, libraries such as NumPy and pandas provide advanced array-like data structures. NumPy offers n-dimensional arrays, which are highly efficient for numerical computations.
When should I use lists instead of arrays in Python?
Use lists when you need a flexible data structure that can hold different data types or when the size of the collection may change. Arrays are preferable for performance-critical applications involving large datasets of uniform data types.
Can I perform mathematical operations on arrays in Python?
Yes, if you use libraries like NumPy, you can perform element-wise mathematical operations on arrays efficiently. This capability is one of the key advantages of using NumPy arrays over standard Python lists.
Python does not have a built-in array data type in the same way that languages like C or Java do. Instead, Python provides a versatile and powerful data structure known as a list, which can be used similarly to arrays in other programming languages. Lists in Python can hold elements of different data types and can be dynamically resized, making them highly flexible for various programming needs.
For scenarios where performance is critical, especially with large datasets, Python offers the array module, which provides a more efficient array-like structure. However, this module is less commonly used than lists and is limited to elements of a single data type. Additionally, libraries such as NumPy provide highly optimized array implementations that are widely used in scientific computing and data analysis, offering advanced functionalities and performance enhancements over the standard list and array types.
while Python does not have traditional arrays, it provides alternative data structures such as lists and specialized libraries that fulfill similar roles. Understanding the differences and appropriate use cases for lists, arrays, and NumPy arrays is essential for effective programming in Python. This knowledge allows developers to choose the right data structure based on their specific requirements, ensuring optimal performance and ease of use.
Author Profile
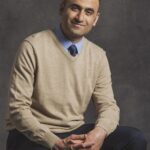
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?