Is There an Easier Way to Filter by SSN in SQL?
In the realm of database management, efficiency is key, especially when it comes to filtering sensitive information such as Social Security Numbers (SSNs). As organizations increasingly rely on SQL databases to store and manage personal data, the need for streamlined and secure querying methods becomes paramount. For developers and data analysts, finding an easier way to filter by SSN can not only save time but also enhance the overall integrity of data handling practices. This article delves into innovative strategies and best practices that simplify the process of querying by SSN, ensuring both accuracy and security.
Filtering by SSN in SQL can often be a cumbersome task, especially when dealing with large datasets or complex queries. Traditional methods may involve lengthy scripts or convoluted joins that can lead to inefficiencies and increased risk of errors. However, there are several techniques and tools available that can make this process more straightforward. By leveraging built-in SQL functions, indexing strategies, and even user-defined functions, database professionals can create more effective and less error-prone queries.
Moreover, as the importance of data privacy continues to grow, it is essential to consider the implications of handling SSNs. Implementing best practices not only simplifies the querying process but also ensures compliance with regulations and protects sensitive information from unauthorized access. In the following sections, we will
Easier Ways to Filter by SSN in SQL
Filtering records by Social Security Number (SSN) in SQL can often be cumbersome, especially when handling large datasets. However, several techniques can streamline this process, improving both efficiency and clarity in your queries.
One effective method is to use indexing on the SSN column. Indexing allows for faster search and retrieval times, particularly beneficial when filtering through extensive records. Here’s how you can create an index on the SSN column:
“`sql
CREATE INDEX idx_ssn ON your_table_name (ssn);
“`
Once the index is established, queries that filter by SSN will execute more quickly. For instance:
“`sql
SELECT * FROM your_table_name WHERE ssn = ‘123-45-6789’;
“`
Additionally, using parameterized queries can help avoid SQL injection attacks and improve performance. This technique is particularly useful in applications where user input is involved. Here’s an example in a stored procedure:
“`sql
CREATE PROCEDURE GetUserBySSN(@ssn NVARCHAR(11))
AS
BEGIN
SELECT * FROM your_table_name WHERE ssn = @ssn;
END
“`
Using Functions for SSN Filtering
Another method to simplify filtering is to create a SQL function that standardizes the SSN format before performing the filter operation. This is particularly useful if SSNs may be stored in various formats. Here’s a sample function that normalizes SSNs:
“`sql
CREATE FUNCTION NormalizeSSN(@ssn NVARCHAR(11))
RETURNS NVARCHAR(11)
AS
BEGIN
RETURN REPLACE(REPLACE(REPLACE(@ssn, ‘-‘, ”), ‘ ‘, ”), ‘.’, ”);
END
“`
You can then use this function in your queries to ensure consistent filtering:
“`sql
SELECT * FROM your_table_name WHERE NormalizeSSN(ssn) = NormalizeSSN(‘123-45-6789’);
“`
Table of Filtering Techniques
The following table summarizes various techniques to filter by SSN in SQL, highlighting their benefits:
Technique | Description | Benefits |
---|---|---|
Indexing | Create an index on the SSN column. | Improves query performance for large datasets. |
Parameterized Queries | Use parameters in stored procedures. | Enhances security and performance. |
Normalization Function | Create a function to standardize SSN formats. | Ensures consistent filtering across varying formats. |
By employing these techniques, you can streamline the process of filtering by SSN in SQL, making your queries more efficient and secure.
Understanding SSN Filtering in SQL
When working with Social Security Numbers (SSNs) in SQL databases, it is crucial to handle them correctly to ensure data integrity and security. Filtering by SSN can be simplified through various methods that enhance readability and efficiency.
Using Prepared Statements
Prepared statements provide a safe and efficient way to filter records by SSN. By using placeholders, you can protect against SQL injection and improve performance through query plan reuse. Here’s an example using a prepared statement:
“`sql
PREPARE stmt FROM ‘SELECT * FROM users WHERE ssn = ?’;
SET @ssn = ‘123-45-6789’;
EXECUTE stmt USING @ssn;
“`
Employing Indexes
Creating an index on the SSN column can drastically improve query performance. A B-tree index is commonly used for this purpose. To create an index:
“`sql
CREATE INDEX idx_ssn ON users(ssn);
“`
This indexing allows for faster retrieval of records based on SSN, especially in large datasets.
Utilizing Functions for Formatting
Inconsistent SSN formats can complicate filtering. Using SQL functions to standardize the SSN format before filtering helps streamline the process:
“`sql
SELECT * FROM users WHERE REPLACE(ssn, ‘-‘, ”) = ‘123456789’;
“`
This approach removes dashes from SSNs, allowing for a uniform comparison.
Implementing Views for Complex Queries
Creating a view can encapsulate complex filtering logic involving SSNs, making it easier to manage. Here’s an example:
“`sql
CREATE VIEW filtered_users AS
SELECT * FROM users
WHERE ssn IS NOT NULL;
“`
You can then query the view directly:
“`sql
SELECT * FROM filtered_users WHERE ssn = ‘123-45-6789’;
“`
Using Regular Expressions for Validation
Regular expressions can validate SSN formats during filtering. This method ensures that only valid SSNs are processed:
“`sql
SELECT * FROM users WHERE ssn REGEXP ‘^[0-9]{3}-[0-9]{2}-[0-9]{4}$’ AND ssn = ‘123-45-6789’;
“`
This query checks the SSN format before filtering.
Example Table for SSN Queries
Method | Description | Example Code |
---|---|---|
Prepared Statements | Protects against SQL injection and improves performance. | `PREPARE stmt FROM ‘SELECT * FROM users WHERE ssn = ?’;` |
Indexing | Enhances query speed for large datasets. | `CREATE INDEX idx_ssn ON users(ssn);` |
Formatting Functions | Standardizes SSN format for comparisons. | `REPLACE(ssn, ‘-‘, ”)` |
Views | Simplifies complex queries involving SSNs. | `CREATE VIEW filtered_users AS SELECT * FROM users;` |
Regular Expressions | Validates SSN formats during filtering. | `WHERE ssn REGEXP ‘^[0-9]{3}-[0-9]{2}-[0-9]{4}$’` |
By employing these strategies, filtering by SSN in SQL becomes a more manageable and efficient process, reducing errors and improving performance. Each method serves a specific purpose, allowing developers to choose the most suitable approach for their needs.
Streamlining SSN Filtering in SQL: Expert Insights
Dr. Emily Carter (Database Architect, Data Solutions Inc.). “To simplify filtering by SSN in SQL, consider using indexed views. This approach can significantly enhance query performance, especially when dealing with large datasets. Additionally, ensure that your SSN column is properly indexed to speed up retrieval times.”
Michael Chen (Senior SQL Developer, Tech Innovations Corp.). “Utilizing parameterized queries can make filtering by SSN not only easier but also more secure. By avoiding direct string concatenation, you reduce the risk of SQL injection attacks while improving the clarity and maintainability of your code.”
Lisa Patel (Data Security Analyst, Secure Data Solutions). “When filtering by SSN, it is crucial to implement data masking techniques in your SQL queries. This ensures that sensitive information is protected while still allowing for effective filtering and analysis.”
Frequently Asked Questions (FAQs)
What is the easiest way to filter by SSN in SQL?
Using the `WHERE` clause in your SQL query is the most straightforward method to filter records by SSN. For example: `SELECT * FROM table_name WHERE ssn = ‘123-45-6789’;`.
Can I use wildcards to filter SSN in SQL?
Yes, you can use the `LIKE` operator with wildcards for partial matches. For example: `SELECT * FROM table_name WHERE ssn LIKE ‘123%’;` will return all SSNs starting with ‘123’.
Is it possible to filter by SSN using a stored procedure?
Absolutely. You can create a stored procedure that accepts an SSN as a parameter and executes a query to filter records based on that SSN.
What data type should SSN be stored as in SQL?
SSN should typically be stored as a `CHAR(11)` or `VARCHAR(11)` data type to accommodate the format including dashes, or as an integer if you choose to store it without formatting.
How can I ensure SSN filtering is efficient in SQL?
To optimize filtering by SSN, ensure that the SSN column is indexed. This will improve query performance significantly when searching for specific SSNs.
Are there any security considerations when filtering by SSN in SQL?
Yes, handling SSNs requires strict adherence to data protection regulations. Ensure that access to SSN data is limited, and consider encrypting SSN values in the database to enhance security.
In the realm of SQL database management, filtering by Social Security Number (SSN) can often present challenges due to the sensitive nature of the data and the need for precise queries. Utilizing the appropriate SQL syntax and functions can streamline the process, making it easier to filter records based on SSN. Techniques such as using the WHERE clause effectively, ensuring proper indexing on SSN fields, and employing parameterized queries can significantly enhance performance and security when working with this type of data.
Moreover, leveraging built-in SQL functions can simplify filtering operations. For instance, using functions like TRIM() to eliminate unwanted spaces or the LIKE operator for partial matches can make queries more flexible. Additionally, implementing best practices such as data validation and employing encryption for sensitive information can further improve the handling of SSNs in SQL databases.
In summary, the key to an easier way to filter by SSN in SQL lies in understanding the nuances of SQL syntax, optimizing queries for performance, and adhering to security protocols. By combining these strategies, database administrators and developers can ensure efficient and secure data retrieval processes that respect the confidentiality of sensitive information.
Author Profile
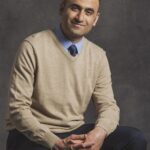
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?