Why Am I Seeing the Error: ‘Jump to Case Label’ in My Code?
In the world of programming, encountering errors is an inevitable part of the development process. One such perplexing issue that developers often face is the “error: jump to case label” message, which can halt progress and lead to frustration. This error typically arises in languages like C and C++, where the intricacies of control flow and case statements can lead to unexpected behavior. Understanding the root causes of this error is essential for programmers looking to write cleaner, more efficient code. In this article, we will delve into the nuances of this error, exploring its implications and offering insights into how to resolve it effectively.
When a programmer sees the “jump to case label” error, it usually indicates a problem with how the code is structured, particularly within switch statements. This error arises when there is an attempt to jump into a case label without proper initialization or when the flow of control does not adhere to the language’s rules. Such issues often stem from a misunderstanding of scope and variable declarations, which can lead to unintended consequences in the program’s execution.
By examining common scenarios that trigger this error, developers can gain a clearer understanding of how to avoid it in their own code. Moreover, we will discuss best practices for structuring switch statements and managing control flow, ensuring that your
Error: Jump to Case Label
The “error: jump to case label” message in C and C++ programming occurs during the compilation stage when the control flow of a program violates the language’s rules regarding the `switch` statement. This typically happens when there is an attempt to jump into a `case` label from outside its scope, such as from within a loop or a conditional statement, leading to behavior.
To understand this error better, it is important to consider the structure of a `switch` statement. A `switch` consists of multiple `case` labels, each representing a potential branch of execution depending on the value of the variable being evaluated. The syntax generally looks like this:
“`c
switch (expression) {
case constant1:
// code block
break;
case constant2:
// code block
break;
default:
// code block
}
“`
This structure enforces a well-defined path of execution. However, if a `goto` statement or other control flow mechanisms attempt to bypass this structure, the compiler raises the error.
Common Causes of the Error
Several scenarios can lead to this compilation error:
- Using `goto` Statements: Jumping directly to a `case` label from outside its scope.
- Improper Scope Handling: Defining `case` labels within a nested block or function and trying to jump to them from an outer block.
- Control Flow Constructs: Using loops or conditionals that can alter the expected flow of execution without appropriate case handling.
Example Scenarios
To illustrate the error, consider the following example:
“`c
void example() {
int x = 1;
switch (x) {
case 1:
// Do something
goto case2; // This will cause an error
case 2:
// Do something else
break;
}
}
“`
In this example, the use of `goto` to jump to `case 2` violates the structure of the `switch`, leading to the “jump to case label” error.
Best Practices to Avoid the Error
To avoid encountering this error, consider the following best practices:
- Limit Use of `goto`: Prefer structured control flow using loops and conditionals.
- Keep Case Labels Within Scope: Ensure that all `case` labels are defined within the same block of the `switch`.
- Use Functions: If complex logic is needed, refactor code into functions to maintain clarity and avoid jumping across scopes.
Error Resolution Table
Cause | Resolution |
---|---|
Using `goto` to jump to a case | Remove `goto` statements; restructure code. |
Case labels in nested blocks | Ensure all case labels are at the same level within the switch. |
Improper control flow | Redesign control flow to use loops and conditionals without jumping. |
By adhering to these guidelines, developers can maintain clean, efficient code while avoiding common pitfalls associated with the `switch` statement and its case labels.
Understanding the Error
The `error: jump to case label` occurs in C and C++ programming languages when the flow of control attempts to jump to a case label in a switch statement without meeting the necessary conditions. This error typically arises when there is a misunderstanding of how case labels operate within the scope of a switch statement.
Common Causes
Several scenarios can lead to this error:
- Improper Use of Control Flow: Attempting to use `goto` statements that jump into a switch case directly.
- Misplaced Statements: Including non-case-related statements before a case label without proper handling.
- Loop Structures: Jumping from outside a loop or function into a switch case directly.
Example of the Error
Consider the following code snippet that demonstrates the `error: jump to case label`:
“`c
include
void example(int value) {
switch (value) {
case 1:
printf(“One\n”);
break;
case 2:
printf(“Two\n”);
break;
default:
goto case 1; // This line causes the error
}
}
“`
In this example, the use of `goto` to jump to `case 1` is invalid and will trigger the error.
How to Resolve the Error
To avoid this error, adhere to the following practices:
- Avoid Goto Statements: Refrain from using `goto` to jump into switch case labels.
- Proper Case Structure: Ensure that control flow constructs (like loops and conditionals) are properly closed before reaching a case label.
- Use Functions: When needing to reuse logic, encapsulate code in functions rather than attempting to jump between cases.
Best Practices for Switch Statements
To effectively utilize switch statements without triggering errors, consider these best practices:
- Always Use Break Statements: Ensure that each case ends with a `break` statement unless fall-through behavior is desired.
- Group Related Cases: If multiple case labels perform the same action, group them together to minimize redundancy.
- Provide a Default Case: Always include a `default` case to handle unexpected values.
Debugging Tips
When encountering this error, apply these debugging techniques:
Technique | Description |
---|---|
Code Review | Carefully review the control flow of your program. |
Simplify Logic | Break down complex switch statements into simpler parts. |
Use Compiler Flags | Enable more verbose compiler warnings to get hints about the problematic area. |
By following these guidelines and understanding the nature of the `error: jump to case label`, developers can effectively prevent and resolve this issue in their code.
Understanding the ‘Jump to Case Label’ Error in Programming
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The ‘jump to case label’ error typically arises in languages like C and C++ when a switch statement attempts to jump to a case label that is not properly defined or reachable. This often indicates a logical flaw in the code structure, necessitating a thorough review of the switch-case implementation.”
Michael Chen (Lead Developer, Tech Innovators Inc.). “In my experience, the ‘jump to case label’ error can also occur when a case label is incorrectly defined outside of the switch statement’s scope. Developers should ensure that all case labels are correctly positioned and that they adhere to the language’s syntax rules to avoid this issue.”
Sarah Thompson (Programming Language Researcher, Future Code Institute). “This error serves as a reminder of the importance of understanding control flow in programming. It highlights the necessity for developers to be vigilant about the visibility and accessibility of case labels within switch statements, as any oversight can lead to compilation errors.”
Frequently Asked Questions (FAQs)
What does the error “jump to case label” mean in C/C++?
The “jump to case label” error occurs when a non-constant expression is used in a switch statement. This error indicates that the compiler has detected an attempt to jump to a case label that is not valid due to the flow of control.
How can I resolve the “jump to case label” error?
To resolve this error, ensure that all case labels in your switch statement are constant expressions. Additionally, avoid using variables or expressions that can change value in the case labels.
Can this error occur in other programming languages?
Yes, similar errors can occur in other programming languages that support switch-case constructs. The specifics of the error message may vary, but the underlying issue of invalid control flow remains consistent.
What are common scenarios that lead to this error?
Common scenarios include using variables instead of constants in case labels, having a goto statement that jumps into a switch block, or improperly nesting switch statements.
Is there a way to prevent this error from occurring in future code?
To prevent this error, always use constant values in case labels, and carefully structure your control flow to avoid jumping into switch cases from outside the block. Regular code reviews can also help identify potential issues early.
Are there any tools that can help identify this error before compilation?
Yes, static code analysis tools can help identify potential issues, including improper use of switch-case statements. Integrated development environments (IDEs) often provide warnings or suggestions that can help catch these errors during coding.
The error message “jump to case label” typically arises in programming languages like C and C++ when a switch statement contains a jump that violates the language’s rules regarding control flow. Specifically, this error occurs when the code attempts to jump into a case label from outside the switch statement, which is not permitted. Such violations can lead to behavior, making it crucial for developers to adhere to the structured flow of control defined by the language specifications.
Understanding the cause of this error is essential for effective debugging. The most common scenario involves the use of break statements or improper use of labels within nested structures. Developers must ensure that any jumps, such as those from loops or conditionals, do not inadvertently lead into case labels. This adherence to proper control flow not only prevents compilation errors but also enhances code readability and maintainability.
To resolve the “jump to case label” error, programmers should review their switch statements and the surrounding code for any instances of improper jumps. Refactoring the code to avoid such jumps or restructuring the logic can often eliminate the error. Additionally, utilizing compiler warnings and static analysis tools can help identify potential issues before they lead to runtime problems.
Author Profile
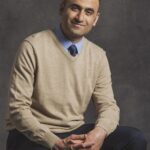
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?