How Can You Resolve the ‘Error: Pulling is Not Possible Because You Have Unmerged Files’ Issue?
In the world of version control, Git stands as a powerful ally for developers, enabling seamless collaboration and efficient code management. However, even the most seasoned programmers can encounter roadblocks that disrupt their workflow. One such obstacle is the dreaded error message: “error: pulling is not possible because you have unmerged files.” This cryptic notification can leave users scratching their heads, unsure of how to proceed. Understanding the implications of this error is crucial for maintaining a smooth development process and ensuring that your project remains on track.
When you see this error, it signifies that Git has detected conflicts in your files that need to be resolved before you can proceed with pulling updates from a remote repository. Unmerged files typically arise during a merge operation, where changes from different branches clash, creating ambiguity about which version should prevail. This situation not only halts your ability to sync with the latest changes but also highlights the importance of conflict resolution in collaborative environments.
Navigating through the intricacies of Git can be daunting, especially when faced with unmerged files. However, with a clear understanding of how to identify and resolve these conflicts, you can swiftly restore harmony to your codebase. In the following sections, we will delve deeper into the causes of this error, effective strategies for conflict resolution
Understanding Unmerged Files in Git
When you encounter the error message “error: pulling is not possible because you have unmerged files,” it indicates that your local Git repository has unresolved merge conflicts. A merge conflict arises when Git is unable to automatically reconcile differences between branches. This situation typically happens during a `git pull` operation, where changes from a remote branch are merged into your local branch.
Causes of Unmerged Files
Unmerged files can occur due to various reasons, including:
- Concurrent changes: Multiple contributors modifying the same lines in a file.
- Branch divergence: Significant differences between the branches being merged.
- Incorrect conflict resolution: Merging changes without properly resolving conflicts.
Identifying Unmerged Files
You can identify unmerged files by executing the following command:
“`bash
git status
“`
This command will list all files that are in a conflict state, typically marked as “both modified.”
Resolving Merge Conflicts
To resolve merge conflicts and eliminate the unmerged files, follow these steps:
- Open the conflicted files: Look for the conflict markers (<<<<<<<, =======, >>>>>>>) that Git inserts into the files.
- Edit the files: Decide how to merge the changes. You can keep your changes, accept incoming changes, or combine both.
- Remove conflict markers: After editing, ensure all conflict markers are deleted.
- Stage the resolved files: Use the command below to add the resolved files to the staging area.
“`bash
git add
“`
- Commit the changes: Finalize the resolution by committing your changes.
“`bash
git commit -m “Resolved merge conflicts”
“`
Example of Conflict Resolution
Here’s a simplified example of a merge conflict:
“`plaintext
<<<<<<< HEAD
Your changes here.
=======
Incoming changes here.
>>>>>>> branch-name
“`
You can resolve it by editing the section to reflect the desired final outcome:
“`plaintext
Your changes here, plus the incoming changes.
“`
Preventing Future Conflicts
To minimize the occurrence of unmerged files, consider the following best practices:
- Regularly pull changes from the remote repository to keep your local branch updated.
- Communicate with team members about significant changes to shared files.
- Break changes into smaller commits to reduce the likelihood of conflicts.
Summary of Commands
Command | Purpose |
---|---|
`git status` | Check the status of the repository |
`git add |
Stage resolved files |
`git commit -m “message”` | Commit your changes after resolution |
By following these steps and best practices, you can effectively manage unmerged files in Git and streamline your development workflow.
Understanding the Error Message
The error message “error: pulling is not possible because you have unmerged files” typically occurs in Git when there are unresolved merge conflicts in your repository. This situation prevents you from performing a pull operation, which is essential for syncing your local repository with the remote one. Understanding the components of this error can help you resolve it efficiently.
- Unmerged files: These are files that Git was unable to merge due to conflicts between your local changes and the changes in the remote repository.
- Pull operation: This process involves fetching changes from a remote repository and merging them with your local branch. If conflicts exist, Git halts the operation to prevent loss of data.
Identifying Unmerged Files
To address the error, first identify which files are unmerged. Use the following Git command:
“`bash
git status
“`
This command will display the status of your working directory and indicate which files have merge conflicts.
- Look for lines that start with “both modified,” “added by them,” or “deleted by them.” These indicate the files that require your attention.
Resolving Merge Conflicts
Once you have identified the unmerged files, you need to resolve the conflicts. Here’s a structured approach:
- Open each conflicting file: Use a code editor to examine the files listed as unmerged.
- Locate conflict markers: Git marks the conflicting sections in the files with:
- `<<<<<<< HEAD`: Your local changes
- `=======`: The separator between your changes and the incoming changes
- `>>>>>>> [commit-id]`: The incoming changes
- Choose how to resolve: You can:
- Keep your changes
- Accept incoming changes
- Combine both changes manually
- Remove conflict markers: After resolving the conflicts, ensure to delete the conflict markers from the file.
- Mark the file as resolved: Use the following command for each file:
“`bash
git add
“`
Completing the Merge Process
After resolving all conflicts and staging the files, you can complete the merge process:
- Commit the changes to finalize the merge:
“`bash
git commit -m “Resolved merge conflicts”
“`
- Now, you can successfully perform the pull operation:
“`bash
git pull
“`
Preventing Future Conflicts
While conflicts are sometimes inevitable, there are strategies to minimize their occurrence:
- Frequent pulls: Regularly sync your local repository with the remote to reduce the number of changes that need merging.
- Communicate with your team: Coordinate with team members on changes that might affect shared files.
- Smaller, focused changes: Break down large changes into smaller commits to simplify the merging process.
Additional Commands for Conflict Resolution
Here are some helpful Git commands that can assist in managing merge conflicts:
Command | Description |
---|---|
`git mergetool` | Opens a visual merge tool for resolving conflicts. |
`git diff` | Shows differences between commits, files, etc. |
`git log` | Displays commit history to help understand changes. |
`git stash` | Temporarily saves changes to clean your working directory. |
By following these steps and utilizing the commands provided, you will be able to effectively resolve the error and maintain a smooth workflow in your Git projects.
Resolving Unmerged Files in Version Control Systems
Dr. Emily Carter (Senior Software Engineer, Code Integrity Solutions). “The error message indicating that pulling is not possible due to unmerged files typically arises when there are conflicts in the code that need to be resolved. It is crucial to address these conflicts before attempting to pull new changes, as unresolved files can lead to further complications in the version control process.”
Michael Chen (Version Control Specialist, DevOps Insights). “When encountering the ‘unmerged files’ error, developers should first check the status of their repository using the command ‘git status’. This will provide a clear overview of the files in conflict, allowing them to resolve the issues manually or by using merge tools before proceeding with the pull operation.”
Sarah Thompson (Technical Writer, GitHub Documentation). “It is essential to understand that unmerged files indicate a need for manual intervention. Users should carefully review the changes in the conflicting files and decide how to integrate them. Once resolved, committing the changes will allow for a successful pull, ensuring that the local repository is in sync with the remote repository.”
Frequently Asked Questions (FAQs)
What does the error “pulling is not possible because you have unmerged files” mean?
This error indicates that there are unresolved merge conflicts in your local repository. Git cannot perform a pull operation until these conflicts are resolved.
How can I check for unmerged files in my Git repository?
You can check for unmerged files by running the command `git status`. This will display the files that are in conflict and need resolution.
What steps should I take to resolve unmerged files?
To resolve unmerged files, open each conflicted file, manually edit the sections marked by Git, and then save the changes. After resolving, use `git add
Can I abort the merge process if I don’t want to resolve conflicts?
Yes, you can abort the merge process by executing the command `git merge –abort`. This will revert your repository to the state before the merge began.
What happens if I ignore the unmerged files and try to pull again?
If you attempt to pull again without resolving the unmerged files, Git will return the same error message, preventing any further operations until the conflicts are addressed.
Is there a way to automate the resolution of merge conflicts?
While there are tools and strategies to assist with merge conflict resolution, such as using merge tools or strategies like `git merge -X theirs`, it is generally recommended to review conflicts manually to ensure accuracy.
The error message “pulling is not possible because you have unmerged files” typically occurs in Git when a user attempts to perform a pull operation while there are unresolved merge conflicts in the repository. This situation arises when changes from different branches or commits conflict with each other, and Git requires the user to manually resolve these conflicts before proceeding with any further operations. As a result, it is crucial for developers to address these unmerged files to maintain a clean and functional codebase.
To resolve this issue, users must first identify the files that are in a conflicted state. This can be achieved by using the command `git status`, which will list the unmerged paths. Once identified, developers can open these files and make the necessary edits to resolve the conflicts. After resolving the conflicts, it is essential to stage the changes using `git add` and then commit them with `git commit`. Only after these steps can users safely execute the pull command without encountering the same error.
In summary, the presence of unmerged files prevents Git from executing pull operations, highlighting the importance of conflict resolution in collaborative development environments. Developers must be diligent in managing merges and conflicts to ensure a smooth workflow. By understanding and addressing these issues promptly, teams can
Author Profile
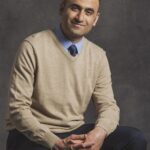
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?