How Can I Use Excel VBA to Check If a Cell in a Range Is Empty?
In the world of Excel, efficiency is paramount, and mastering VBA (Visual Basic for Applications) can significantly elevate your spreadsheet game. Whether you’re automating repetitive tasks, creating complex data analyses, or simply enhancing the functionality of your worksheets, knowing how to manipulate and evaluate cell contents is essential. One common challenge that many users face is determining whether a specific cell within a defined range is empty. This seemingly simple task can have profound implications for data integrity, error handling, and overall workflow optimization. In this article, we will delve into the methods and best practices for checking if a cell in a range is empty using VBA, empowering you to streamline your Excel projects.
When working with ranges in Excel, it’s crucial to understand how to assess the state of individual cells. A cell may appear empty but could contain invisible characters, formulas, or formatting that affect your data processing. VBA provides powerful tools to navigate these intricacies, enabling you to create robust scripts that can handle various scenarios. By learning how to check for empty cells effectively, you can prevent errors in your calculations, ensure accurate data entry, and enhance the user experience of your spreadsheets.
Moreover, the ability to identify empty cells can pave the way for more advanced functionalities, such as conditional formatting, data validation
Using VBA to Check for Empty Cells in a Range
To determine if a cell within a specified range is empty using Excel VBA, you can utilize the `IsEmpty` function. This function checks whether a specific cell or range contains any data. If it does not, `IsEmpty` returns `True`, indicating that the cell is empty.
Here’s a basic example of how to implement this in your VBA code:
“`vba
Sub CheckEmptyCells()
Dim rng As Range
Dim cell As Range
Dim emptyCells As String
Set rng = ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10″) ‘ Define the range
For Each cell In rng
If IsEmpty(cell) Then
emptyCells = emptyCells & cell.Address & ” ” ‘ Collect addresses of empty cells
End If
Next cell
If emptyCells <> “” Then
MsgBox “The following cells are empty: ” & emptyCells
Else
MsgBox “No empty cells found.”
End If
End Sub
“`
In this example, the code iterates through each cell in the defined range (`A1:A10`). If it finds an empty cell, it collects the cell’s address and displays a message box listing all empty cells.
Handling Multiple Conditions
In some cases, you may want to check for multiple conditions or handle different types of “emptiness,” such as cells containing only spaces. You can extend the logic by using the `Trim` function alongside `IsEmpty`. Here’s an example of how to do this:
“`vba
Sub CheckEmptyOrWhitespaceCells()
Dim rng As Range
Dim cell As Range
Dim emptyCells As String
Set rng = ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10”)
For Each cell In rng
If IsEmpty(cell) Or Trim(cell.Value) = “” Then
emptyCells = emptyCells & cell.Address & ” ”
End If
Next cell
If emptyCells <> “” Then
MsgBox “The following cells are empty or contain only whitespace: ” & emptyCells
Else
MsgBox “No empty or whitespace-only cells found.”
End If
End Sub
“`
This code snippet checks for both truly empty cells and those that appear empty but contain whitespace, providing a more thorough validation of the cell contents.
Table of Empty Cell Checks
Function | Description |
---|---|
`IsEmpty(cell)` | Returns `True` if the cell is truly empty. |
`Trim(cell.Value)` | Removes spaces and checks if the cell is empty after trimming. |
Best Practices
When working with cell checks in Excel VBA, consider the following best practices:
- Always define the range explicitly to avoid unintended results.
- Use `Option Explicit` at the top of your module to require variable declaration, which helps prevent errors.
- Handle potential errors gracefully using error handling techniques to enhance code robustness.
- Consider the performance impact when dealing with large ranges; minimize the number of read/write operations on the Excel sheet.
Using VBA to Check If a Cell in a Range Is Empty
In Excel VBA, determining whether a specific cell in a defined range is empty can be accomplished through various methods. The `IsEmpty` function and direct comparison with an empty string are two common approaches.
Method 1: Using the IsEmpty Function
The `IsEmpty` function checks if a variable has been initialized or if a cell is devoid of any content. This function is particularly useful when iterating through a range of cells.
“`vba
Dim cell As Range
Dim rng As Range
Set rng = ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10”)
For Each cell In rng
If IsEmpty(cell) Then
‘ Your code here for empty cell
End If
Next cell
“`
Method 2: Direct Comparison
Another approach is to directly compare the cell’s value to an empty string. This method is straightforward and can be easily implemented in loops or conditional statements.
“`vba
Dim cell As Range
Dim rng As Range
Set rng = ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10”)
For Each cell In rng
If cell.Value = “” Then
‘ Your code here for empty cell
End If
Next cell
“`
Checking Multiple Cells
When working with multiple cells, it’s often necessary to check each cell’s status and perform actions accordingly. The following code snippet demonstrates how to count the number of empty cells in a specified range.
“`vba
Dim cell As Range
Dim rng As Range
Dim emptyCount As Integer
Set rng = ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10”)
emptyCount = 0
For Each cell In rng
If IsEmpty(cell) Then
emptyCount = emptyCount + 1
End If
Next cell
MsgBox “Number of empty cells: ” & emptyCount
“`
Handling Different Data Types
Cells in Excel can contain various data types, including text, numbers, and formulas. It’s important to consider these when checking for empty cells. A cell that appears empty may actually contain a formula that returns an empty string, which will not be detected by the `IsEmpty` function.
Example of Handling Formulas
If you want to check for cells that are either truly empty or contain formulas returning empty strings, use the following method:
“`vba
Dim cell As Range
Dim rng As Range
Dim emptyCount As Integer
Set rng = ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10”)
emptyCount = 0
For Each cell In rng
If IsEmpty(cell) Or cell.Value = “” Then
emptyCount = emptyCount + 1
End If
Next cell
MsgBox “Number of truly empty cells or cells with empty strings: ” & emptyCount
“`
Performance Considerations
When checking large ranges, consider using the `Application.ScreenUpdating` property to improve performance. Disabling screen updating during the execution of your VBA code can make your code run faster.
“`vba
Application.ScreenUpdating =
‘ Your code here
Application.ScreenUpdating = True
“`
This optimization can be particularly beneficial in scenarios where multiple checks and updates are performed on the worksheet.
Expert Insights on Checking for Empty Cells in Excel VBA
Dr. Emily Carter (Senior Data Analyst, Excel Solutions Inc.). “In my experience, using the IsEmpty function within a VBA script is the most efficient way to determine if a cell in a specified range is empty. This approach not only simplifies the code but also enhances performance when dealing with large datasets.”
Michael Thompson (VBA Programming Specialist, Tech Innovations). “When checking if cells in a range are empty, I recommend using a loop in conjunction with the IsEmpty function. This allows for greater flexibility in handling multiple cells and can be particularly useful when validating user input or preparing data for analysis.”
Linda Patel (Excel VBA Consultant, Data Insights Group). “For those looking to streamline their VBA code, implementing the CountA function can be a powerful alternative. It provides a quick way to assess the number of non-empty cells in a range, allowing users to easily identify if any cells are empty without iterating through each one individually.”
Frequently Asked Questions (FAQs)
How can I check if a specific cell is empty in Excel VBA?
You can use the `IsEmpty` function to check if a specific cell is empty. For example, `If IsEmpty(Range(“A1”)) Then …` will evaluate whether cell A1 is empty.
What is the method to check if any cell in a range is empty?
You can loop through the range using a `For Each` loop. For instance:
“`vba
Dim cell As Range
For Each cell In Range(“A1:A10”)
If IsEmpty(cell) Then
‘ Code to execute if the cell is empty
End If
Next cell
“`
Can I use the `CountBlank` function to check for empty cells in a range?
Yes, the `CountBlank` function can be used to count the number of empty cells in a specified range. For example, `If Application.WorksheetFunction.CountBlank(Range(“A1:A10”)) > 0 Then …` checks if there are any empty cells in the range A1:A10.
What happens if I check an entire range for emptiness?
When checking an entire range, you need to determine how you want to handle multiple empty cells. You can either count them, as mentioned with `CountBlank`, or loop through each cell to perform specific actions based on whether they are empty.
Is there a way to highlight empty cells in a range using VBA?
Yes, you can highlight empty cells by looping through the range and applying formatting. For example:
“`vba
Dim cell As Range
For Each cell In Range(“A1:A10”)
If IsEmpty(cell) Then
cell.Interior.Color = RGB(255, 0, 0) ‘ Highlights empty cells in red
End If
Next cell
“`
Can I use conditional formatting to check for empty cells without VBA?
Yes, you can use conditional formatting to visually highlight empty cells. Select the range, go to Conditional Formatting, choose “New Rule,” and use the formula `=ISBLANK(A1)` (adjust the reference as needed) to apply formatting to empty cells.
In Excel VBA, checking if a cell within a specified range is empty is a common task that can streamline data validation and processing. This can be achieved using various methods, such as the `IsEmpty` function, which directly evaluates whether a cell contains any data. Additionally, the `CountA` function can be utilized to assess the number of non-empty cells within a range, allowing for more complex evaluations of data integrity.
Utilizing loops to iterate through each cell in a range provides a flexible approach to identify empty cells. This method is particularly useful when dealing with larger datasets or when specific actions need to be taken for each empty cell found. Furthermore, employing conditional statements enables the execution of tailored responses based on the presence or absence of data, enhancing the overall functionality of the VBA script.
In summary, mastering the techniques to check for empty cells in a range using Excel VBA not only improves data management but also enhances the efficiency of automated tasks. By leveraging built-in functions and control structures, users can create robust solutions that ensure data quality and facilitate informed decision-making. This knowledge is essential for anyone looking to optimize their use of Excel for data analysis and reporting.
Author Profile
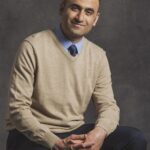
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?