How Do You Determine the Canvas Size Unit in Fabric.js?
In the world of web development, creating dynamic and interactive graphics is becoming increasingly essential. One powerful tool that has emerged to simplify this process is Fabric.js, a robust JavaScript library that provides an intuitive way to work with HTML5 canvas elements. Whether you’re designing a custom image editor, an interactive game, or a data visualization tool, understanding how to manipulate the canvas size and its units is crucial for achieving the desired results. This article will delve into the intricacies of canvas size in Fabric.js, exploring how different units can affect your designs and enhance user experience.
When working with Fabric.js, the canvas size is not just a matter of pixels; it encompasses various units that can significantly impact the layout and functionality of your project. The library allows developers to define the dimensions of the canvas in multiple ways, providing flexibility to cater to different design needs. From setting fixed pixel values to utilizing responsive units, understanding these options can help you create a more adaptable and visually appealing interface.
Moreover, the choice of canvas size units can influence how elements are rendered and interact with user inputs. By grasping the nuances of canvas dimensions and their implications, developers can optimize performance and ensure that their applications look great across a range of devices and screen sizes. As we explore the different
Understanding Canvas Size Units in Fabric.js
When working with Fabric.js, it is crucial to understand how canvas size is defined and managed, as it can significantly impact the rendering of objects and overall performance. Fabric.js utilizes a coordinate system based on pixels, which is the standard unit of measurement for web graphics. This approach ensures that the canvas behaves consistently across different devices and screen resolutions.
Setting Canvas Size
The canvas size in Fabric.js can be set using two primary methods: through the HTML `
- HTML Attributes: When creating a canvas element in HTML, you can specify the width and height directly.
“`html
“`
- Fabric.js API: You can also define the canvas size when initializing the Fabric.js canvas.
“`javascript
var canvas = new fabric.Canvas(‘c’, {
width: 800,
height: 600
});
“`
Setting the canvas size correctly is essential for ensuring that all objects are rendered as expected and that the canvas behaves properly during interactions.
Responsive Canvas Design
For applications that need to adapt to various screen sizes, implementing a responsive design is vital. Fabric.js provides tools to handle resizing, allowing developers to maintain the aspect ratio or completely adjust the layout.
- Maintaining Aspect Ratio: To ensure that the canvas resizes while keeping its aspect ratio, developers can listen to window resize events and adjust the canvas size accordingly.
- Scaling Objects: When resizing the canvas, it may be necessary to scale the objects on the canvas to fit the new dimensions. This can be achieved using the `scaleToWidth` or `scaleToHeight` methods.
Canvas Size Properties
The Fabric.js canvas object has specific properties that can be accessed and modified to control the canvas’s dimensions and behavior.
Property | Description |
---|---|
width |
Defines the width of the canvas in pixels. |
height |
Defines the height of the canvas in pixels. |
viewportTransform |
Allows transformation of the viewport for zooming and panning. |
setDimensions() |
Method to set the width and height of the canvas dynamically. |
By understanding these properties, developers can manipulate the canvas effectively to achieve desired outcomes in their applications.
Conclusion on Canvas Size Management
Effective management of canvas size in Fabric.js is essential for creating visually appealing and functional web applications. By utilizing the available methods and properties, developers can ensure optimal performance and user experience. Properly setting the canvas size, adapting it for responsiveness, and leveraging the properties provided by Fabric.js are foundational skills for any developer working with this powerful library.
Understanding Fabric.js Canvas Size Units
In Fabric.js, the canvas size can be defined using various units, which directly influence how objects are rendered and displayed. Understanding these units is essential for creating responsive and visually appealing designs.
Canvas Size Configuration
When initializing a Fabric.js canvas, you can set its width and height using pixel values or percentages. Here are the common configurations:
- Pixels: The most straightforward unit. Defining the canvas in pixels is standard practice.
- Percentages: Useful for responsive designs, allowing the canvas to adjust based on the parent container’s size.
Setting Canvas Size in Fabric.js
The canvas size can be set during initialization or modified later. Below are examples illustrating both methods:
“`javascript
// Initialize canvas with specific size
const canvas = new fabric.Canvas(‘canvasId’, {
width: 800,
height: 600,
});
// Change canvas size later
canvas.setWidth(500);
canvas.setHeight(400);
“`
Units and Scaling
Fabric.js handles scaling based on the specified units. Here’s a breakdown of how scaling interacts with canvas size:
- Default Units: Fabric.js defaults to pixels. All measurements (width, height, object dimensions) are pixel-based unless specified otherwise.
- Scaling Factors: When scaling the canvas, ensure that the aspect ratio is maintained to avoid distortion of objects.
Responsive Design Considerations
To create a responsive canvas, consider the following practices:
- Use CSS for Parent Containers: Set the parent container’s width and height using CSS. This allows the Fabric.js canvas to inherit those dimensions.
- Event Listeners: Add listeners to handle window resizing, adjusting the canvas size dynamically.
“`javascript
window.addEventListener(‘resize’, function() {
const newWidth = window.innerWidth * 0.8; // 80% of window width
const newHeight = window.innerHeight * 0.8; // 80% of window height
canvas.setWidth(newWidth);
canvas.setHeight(newHeight);
});
“`
Example of Canvas Size Adjustment
Here’s a simple example of creating a responsive Fabric.js canvas that adjusts to the window size:
“`html
“`
This configuration ensures that the canvas fills the entire viewport, adapting as the window size changes.
Best Practices for Canvas Size Management
To ensure optimal performance and usability, consider the following best practices:
- Consistent Unit Use: Stick to one unit type throughout your project to avoid confusion.
- Performance Monitoring: Larger canvas sizes can affect performance. Monitor and optimize object rendering.
- Testing Across Devices: Check canvas behavior on various devices to ensure a consistent user experience.
Unit Type | Description | Use Case |
---|---|---|
Pixels | Absolute size unit | Static designs where precision is key |
Percentages | Relative size unit | Responsive designs adapting to various screen sizes |
Understanding Fabric.js Canvas Size Units
Dr. Emily Carter (Senior Software Engineer, Creative Tech Solutions). “When working with Fabric.js, it’s crucial to understand that the canvas size can be defined in various units such as pixels, percentages, or even viewport units. Each unit serves different purposes depending on the design requirements and responsiveness needed for web applications.”
Michael Chen (Lead Frontend Developer, Digital Innovations Inc.). “In Fabric.js, the canvas size is typically set in pixels, which provides the most control over the rendering of graphical elements. However, utilizing relative units like percentages can enhance the adaptability of the canvas in responsive designs, allowing for a more fluid user experience across different devices.”
Sarah Thompson (UX/UI Designer, Modern Web Agency). “Choosing the right canvas size unit in Fabric.js is essential for maintaining visual consistency. While pixels are the standard, I often recommend experimenting with CSS units like ‘vw’ and ‘vh’ for a more dynamic layout that adjusts seamlessly to various screen sizes, improving overall usability.”
Frequently Asked Questions (FAQs)
What units can be used for setting the canvas size in Fabric.js?
Fabric.js primarily uses pixels as the unit for defining canvas size. However, you can also use CSS units like percentages or viewport units when styling the canvas element through CSS.
How do I set a specific width and height for a Fabric.js canvas?
You can set the width and height of a Fabric.js canvas by using the `setWidth()` and `setHeight()` methods or by specifying the `width` and `height` properties when creating a new canvas instance.
Can I use different units for canvas size in Fabric.js?
While Fabric.js uses pixels as the default unit, you can convert other units like centimeters or inches to pixels based on the screen’s DPI (dots per inch) for accurate sizing.
Does the canvas size affect the rendering of objects in Fabric.js?
Yes, the canvas size directly affects how objects are rendered. Objects will scale and position based on the canvas dimensions, so it’s important to set the canvas size appropriately to match your design requirements.
How can I make a responsive Fabric.js canvas?
To create a responsive Fabric.js canvas, you can adjust the canvas size dynamically using JavaScript based on the window size and use CSS to maintain the aspect ratio.
Is there a way to get the current size of the Fabric.js canvas?
Yes, you can retrieve the current canvas size by accessing the `width` and `height` properties of the Fabric.js canvas instance, which will return the dimensions in pixels.
In the context of Fabric.js, understanding canvas size units is crucial for effectively managing the dimensions and scaling of graphical elements. Fabric.js operates primarily in pixels, which is the default unit of measurement for the canvas. This means that when developers set the width and height of the canvas, they are specifying these dimensions in pixels. This pixel-based approach allows for precise control over the rendering and placement of objects on the canvas.
Moreover, Fabric.js provides options for scaling and transforming objects, which can be particularly useful when dealing with different screen sizes or resolutions. Developers can utilize methods such as `setWidth()` and `setHeight()` to dynamically adjust the canvas size, ensuring that the visual output remains consistent across various devices. Additionally, the library supports responsive design principles, allowing for the adjustment of canvas dimensions based on the viewport size.
Another important aspect to consider is the relationship between the canvas size and the resolution of the output. When exporting graphics or images from the canvas, developers should be mindful of the resolution settings to ensure that the final output meets the desired quality standards. Fabric.js allows for the specification of export dimensions, which can be scaled appropriately to maintain clarity and detail in the resulting images.
a solid
Author Profile
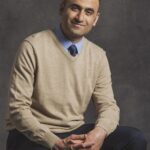
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?