How Can I Retrieve User Group Membership Using PowerShell?
In today’s digital landscape, managing user access and permissions is crucial for maintaining security and efficiency within organizations. As systems grow more complex, IT professionals often find themselves needing to quickly ascertain user group memberships to ensure that the right individuals have the appropriate access to resources. Enter PowerShell, a powerful scripting language and command-line shell that simplifies the management of Windows environments. With its robust capabilities, PowerShell provides an efficient way to retrieve user group memberships, making it an indispensable tool for system administrators and IT specialists.
Understanding how to get user group membership through PowerShell can streamline administrative tasks and enhance security protocols. Whether you’re troubleshooting access issues, auditing permissions, or preparing for compliance checks, knowing how to efficiently pull user group data is essential. PowerShell’s versatility allows you to query Active Directory, local user accounts, and more, providing a comprehensive view of user access rights across your organization.
As we delve deeper into the topic, we’ll explore the various methods and commands available in PowerShell for retrieving user group memberships. From simple queries to more complex scripts, you’ll discover how to harness the full potential of PowerShell to manage user access effectively. Whether you’re a seasoned IT professional or a newcomer to PowerShell, this guide will equip you with the knowledge you need to navigate user group management with confidence.
Using PowerShell to Retrieve User Group Membership
To get user group membership in PowerShell, you can utilize the `Get-ADUser` cmdlet, which is part of the Active Directory module. This cmdlet allows you to query user properties, including group memberships.
Here’s a basic example of how to retrieve a user’s group memberships:
“`powershell
Get-ADUser -Identity “username” -Properties MemberOf | Select-Object -ExpandProperty MemberOf
“`
In this command:
- `-Identity` specifies the username of the account you wish to query.
- `-Properties MemberOf` retrieves the groups that the user is a member of.
- `Select-Object -ExpandProperty MemberOf` outputs the group names directly.
If you’re working in an environment where you need to check multiple users, you can loop through a list of usernames using a script:
“`powershell
$usernames = @(“user1”, “user2”, “user3″)
foreach ($username in $usernames) {
Get-ADUser -Identity $username -Properties MemberOf |
Select-Object Name, @{Name=”Groups”;Expression={($_.MemberOf -join “, “)}}
}
“`
This script will output a list of users along with their corresponding groups in a more readable format.
Exporting Group Membership Information
For reporting purposes, you may want to export the group membership information to a CSV file. This can be done easily by modifying the previous script:
“`powershell
$usernames = @(“user1”, “user2”, “user3″)
$results = foreach ($username in $usernames) {
Get-ADUser -Identity $username -Properties MemberOf |
Select-Object Name, @{Name=”Groups”;Expression={($_.MemberOf -join “, “)}}
}
$results | Export-Csv -Path “UserGroupMembership.csv” -NoTypeInformation
“`
The above code collects the user names and their groups into a variable `$results`, which is then exported to a CSV file named `UserGroupMembership.csv`.
Understanding Group Types
In Active Directory, users can belong to different types of groups, each serving distinct purposes. Here’s a brief overview of the main types:
Group Type | Description |
---|---|
Security Group | Used to assign permissions to shared resources. |
Distribution Group | Used for email distribution lists; does not have security settings. |
Domain Local Group | Used to assign permissions within a single domain. |
Global Group | Used to group users within the same domain; can be assigned permissions in other domains. |
Universal Group | Can contain users from any domain in a forest and can be assigned permissions across the entire forest. |
Understanding these group types is essential when managing user permissions and access within an Active Directory environment. By leveraging PowerShell, administrators can efficiently monitor and manage user memberships across various groups, ensuring compliance with security policies and enhancing organizational efficiency.
Retrieving User Group Membership in PowerShell
To retrieve a user’s group membership in PowerShell, you can utilize the `Get-ADUser` cmdlet in conjunction with the `Get-ADPrincipalGroupMembership` cmdlet. This allows for a straightforward approach to extract information regarding the groups to which a user belongs.
Using Get-ADUser and Get-ADPrincipalGroupMembership
The following command retrieves the group memberships for a specific user:
“`powershell
Get-ADUser -Identity “username” | Get-ADPrincipalGroupMembership | Select-Object Name
“`
In this command:
- `Get-ADUser -Identity “username”`: Retrieves the user object based on the specified username.
- `Get-ADPrincipalGroupMembership`: Fetches all groups that the user is a member of.
- `Select-Object Name`: Outputs only the names of the groups.
Ensure that you replace `”username”` with the actual username of the user whose group memberships you wish to query.
Filtering Group Memberships
If you need to filter the retrieved groups based on specific criteria, you can use the `Where-Object` cmdlet. For example, to find only security groups, you can modify the command as follows:
“`powershell
Get-ADUser -Identity “username” | Get-ADPrincipalGroupMembership | Where-Object {$_.GroupCategory -eq ‘Security’} | Select-Object Name
“`
This command filters the groups to include only those categorized as security groups.
Exporting Group Memberships to CSV
To export the list of group memberships to a CSV file for reporting or analysis, you can extend the command as shown below:
“`powershell
Get-ADUser -Identity “username” | Get-ADPrincipalGroupMembership | Select-Object Name | Export-Csv -Path “C:\path\to\output.csv” -NoTypeInformation
“`
In this example:
- `Export-Csv -Path “C:\path\to\output.csv”`: Specifies the file path where the CSV will be saved.
- `-NoTypeInformation`: Prevents PowerShell from including type information in the first line of the CSV.
Batch Processing for Multiple Users
To check group memberships for multiple users, you can use a loop or a pipeline with an array of usernames. For instance:
“`powershell
$usernames = @(“user1”, “user2”, “user3”)
foreach ($username in $usernames) {
Get-ADUser -Identity $username | Get-ADPrincipalGroupMembership | Select-Object @{Name=’Username’;Expression={$username}}, Name
}
“`
This will output the group memberships along with the corresponding usernames, making it easier to track which groups each user belongs to.
Example Output
The output from the above commands will typically look like this:
Username | Group Name |
---|---|
user1 | Domain Users |
user1 | Sales Group |
user2 | Domain Users |
user2 | Marketing Group |
user3 | Domain Users |
user3 | IT Support Group |
Using these methods, you can efficiently manage and review user group memberships in an Active Directory environment through PowerShell.
Expert Insights on Retrieving User Group Membership with PowerShell
Dr. Emily Carter (Senior Systems Administrator, Tech Solutions Inc.). “Utilizing PowerShell to get user group membership is an efficient way to manage permissions within an organization. The command ‘Get-ADUser -Identity username -Properties MemberOf’ provides a straightforward method to retrieve this information, making it essential for any system administrator.”
Michael Thompson (IT Security Consultant, CyberSafe Advisors). “In the realm of user management, understanding group memberships is crucial for maintaining security protocols. PowerShell’s ‘Get-ADGroupMember’ cmdlet not only lists members of a specific group but can also be combined with other commands to enhance reporting capabilities, which is vital for audits.”
Linda Zhang (PowerShell Expert and Author, Scripting Mastery). “For those looking to automate user group membership checks, leveraging PowerShell scripts can save significant time and reduce errors. Implementing a script that cycles through multiple users and retrieves their group memberships can streamline administrative tasks and improve overall efficiency.”
Frequently Asked Questions (FAQs)
How can I check a user’s group membership using PowerShell?
You can check a user’s group membership by using the command `Get-ADUser -Identity username -Properties MemberOf`. This retrieves the groups that the specified user is a member of.
What module do I need to use to get user group membership in PowerShell?
To get user group membership, you need the Active Directory module for Windows PowerShell. You can import it using the command `Import-Module ActiveDirectory`.
Can I get group membership for multiple users at once in PowerShell?
Yes, you can retrieve group membership for multiple users by using a command like `Get-ADUser -Filter * -Properties MemberOf`, which lists all users and their group memberships.
Is it possible to export user group membership information to a CSV file?
Yes, you can export user group membership information to a CSV file using the command `Get-ADUser -Filter * -Properties MemberOf | Select-Object Name, MemberOf | Export-Csv -Path “C:\Path\To\File.csv” -NoTypeInformation`.
What permissions are required to view user group memberships in PowerShell?
You need to have sufficient permissions within Active Directory to view user group memberships. Typically, being a member of the Domain Users group is sufficient, but specific permissions may vary based on your organization’s policies.
Can I filter group membership results based on specific criteria?
Yes, you can filter group membership results using the `Where-Object` cmdlet. For example, `Get-ADUser -Filter * -Properties MemberOf | Where-Object { $_.MemberOf -like “*GroupName*” }` filters users based on group names.
In summary, retrieving user group membership in PowerShell is a fundamental task for system administrators and IT professionals managing user permissions and access control. PowerShell provides a variety of cmdlets, such as `Get-ADUser` and `Get-ADGroupMember`, which facilitate the extraction of group membership information from Active Directory. These tools allow for efficient management and auditing of user roles within an organization, ensuring that access rights are appropriately assigned and maintained.
Additionally, leveraging PowerShell scripts can automate the process of checking group memberships for multiple users, thereby saving time and reducing the likelihood of human error. By utilizing filters and parameters, administrators can tailor their queries to retrieve specific information, such as nested group memberships or users belonging to particular groups. This capability enhances the overall security posture by enabling more granular control over user access.
Moreover, understanding how to effectively use PowerShell for user group membership queries not only streamlines administrative tasks but also empowers IT professionals to enforce compliance with organizational policies. Regular audits and reviews of group memberships can help identify potential security risks and ensure that users have the appropriate level of access based on their current roles and responsibilities.
Author Profile
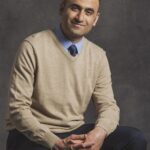
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?