How Can You Build a Script in Python? A Step-by-Step Guide for Beginners
Introduction
In an age where automation and efficiency are paramount, the ability to craft a script in Python has become an invaluable skill for both budding programmers and seasoned developers alike. Whether you’re looking to streamline repetitive tasks, analyze data, or create interactive applications, Python’s simplicity and versatility make it an ideal choice. But where do you start? Building a script may seem daunting at first, but with the right guidance, you can transform your ideas into functional code that brings your vision to life.
Creating a script in Python involves understanding the foundational elements of the language, including its syntax, data structures, and libraries. At its core, scripting is about translating your thought process into a series of logical steps that a computer can execute. This journey begins with identifying a problem or task that you want to address and then breaking it down into manageable components. By leveraging Python’s extensive ecosystem of modules, you can enhance your script’s capabilities and efficiency, allowing for more complex functionalities with less effort.
As you embark on this scripting adventure, you’ll discover the importance of planning and testing your code. Writing a script is not just about getting it to run; it’s about ensuring it runs correctly and efficiently. This article will guide you through the essential concepts and best practices for building a Python script,
Defining the Purpose of Your Script
Before writing any script in Python, it’s crucial to clearly define its purpose. Understanding what you want to achieve will guide your coding decisions and help in structuring your script effectively. Consider the following aspects:
- Target Audience: Who will use your script?
- Functionality: What specific tasks should it perform?
- Inputs and Outputs: What data will your script take in, and what will it produce?
Creating a brief outline of these points will serve as a roadmap for your development process.
Setting Up Your Development Environment
A well-configured environment is essential for efficient script development. Follow these steps to set up your Python environment:
- Install Python: Download the latest version of Python from the official website.
- Choose an IDE or Text Editor: Options include PyCharm, VSCode, or even simple text editors like Notepad++.
- Install Necessary Libraries: Use pip to install any libraries your script may need. For example:
bash
pip install requests
Here’s a simple table summarizing popular Python IDEs:
IDE | Features | Best For |
---|---|---|
PyCharm | Code completion, debugging, version control | Professional developers |
VSCode | Lightweight, customizable, built-in terminal | General-purpose programming |
Jupyter Notebook | Interactive coding, data visualization | Data science and machine learning |
Writing Your First Script
Once your environment is set up, you can start writing your script. Begin with a simple example to familiarize yourself with the syntax and structure of Python. For instance, a basic script that prints “Hello, World!” looks like this:
python
print(“Hello, World!”)
After writing your script, save it with a `.py` extension. To run the script, use the command line:
bash
python your_script.py
Implementing Functions and Modular Code
As your script grows in complexity, organizing your code becomes essential. Utilizing functions helps in breaking down tasks into manageable segments. Here’s how to define a function in Python:
python
def greet(name):
print(f”Hello, {name}!”)
You can call this function with:
python
greet(“Alice”)
Consider the following best practices for modular code:
- Keep functions focused: Each function should perform a single task.
- Use descriptive names: Function names should clearly describe their purpose.
- Document your code: Use comments and docstrings to explain complex sections.
Testing and Debugging Your Script
Testing and debugging are critical steps in the development process. Here are some techniques you can use:
- Unit Testing: Use the `unittest` module to create tests for your functions.
- Debugging: Insert print statements or use a debugger tool to trace issues in your code.
Example of a simple unit test:
python
import unittest
class TestGreetFunction(unittest.TestCase):
def test_greet(self):
self.assertEqual(greet(“Bob”), “Hello, Bob!”)
if __name__ == ‘__main__’:
unittest.main()
By following these guidelines, you can effectively build a Python script tailored to your needs while maintaining clarity and functionality throughout the development process.
Understanding the Basics of Python Scripting
To build a script in Python, it is essential to understand the fundamental components of the language. Python is known for its readability and simplicity, making it an excellent choice for scripting.
- Variables: Used to store data that can be referenced and manipulated.
- Data Types: Includes integers, floats, strings, lists, tuples, dictionaries, and sets.
- Control Structures: These include conditional statements (if, else, elif) and loops (for, while) that allow for decision-making and repeated execution of code.
- Functions: Defined blocks of code that perform specific tasks and can be reused throughout the script.
Setting Up Your Environment
Before writing a script, you must set up your development environment.
- Install Python: Download and install the latest version of Python from the official website.
- Choose an IDE/Text Editor: Options include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
- Sublime Text
- Verify Installation: Open a terminal or command prompt and type `python –version` to ensure Python is installed correctly.
Creating Your First Python Script
To create a Python script, follow these steps:
- Open your text editor or IDE.
- Create a new file: Name it with a `.py` extension (e.g., `myscript.py`).
- Write your script: Start with a simple print statement.
python
print(“Hello, World!”)
- Save the file.
Running Your Python Script
Once you have created your script, you can run it.
- Using the Command Line:
- Navigate to the directory where your script is saved.
- Type `python myscript.py` and press Enter.
- Using an IDE:
- Most IDEs have a built-in run option, typically available in the menu or via a shortcut.
Implementing Key Features in Your Script
To enhance your script, you can implement various features:
- User Input: Use the `input()` function to gather data from users.
python
name = input(“Enter your name: “)
print(“Hello, ” + name)
- Error Handling: Use try-except blocks to handle exceptions gracefully.
python
try:
number = int(input(“Enter a number: “))
except ValueError:
print(“That’s not a valid number!”)
- Modules and Libraries: Import modules to extend functionality. For example, use `import math` to access mathematical functions.
Debugging and Testing Your Script
Debugging is crucial for ensuring your script runs as expected. Here are some techniques:
- Print Statements: Use print statements to inspect variable values.
- Python Debugger (pdb): Utilize the built-in debugger for more complex issues.
- Unit Testing: Write test cases using the `unittest` module to validate functionality.
Best Practices for Python Scripting
To maintain high-quality scripts, follow these best practices:
- Code Readability: Use meaningful variable names and proper indentation.
- Commenting: Add comments to explain complex sections of code.
- Version Control: Use systems like Git to track changes and collaborate with others.
- Documentation: Document your code using docstrings to help others understand how to use it.
Best Practice | Description |
---|---|
Code Readability | Write clear, understandable code. |
Commenting | Explain complex logic with comments. |
Version Control | Track changes and collaborate effectively. |
Documentation | Provide usage instructions through docstrings. |
Expanding Your Python Knowledge
To further develop your Python scripting skills, consider:
- Online Courses: Platforms such as Coursera, Udemy, or edX offer structured courses.
- Books: Titles like “Automate the Boring Stuff with Python” provide practical examples.
- Community Engagement: Join forums such as Stack Overflow or Reddit to discuss and troubleshoot with peers.
Expert Insights on Building a Script in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When building a script in Python, it is crucial to start with a clear understanding of the problem you are trying to solve. A well-defined objective will guide your coding process and help you structure your script effectively.”
Michael Chen (Python Development Consultant, CodeMaster Solutions). “Utilizing Python’s extensive libraries can significantly enhance your script’s functionality. Always explore available modules that can simplify tasks, such as requests for web scraping or pandas for data manipulation.”
Lisa Patel (Lead Data Scientist, Data Insights Corp.). “Testing and debugging are integral parts of building a Python script. Implementing unit tests during the development process can help identify issues early and ensure your script runs smoothly in production.”
Frequently Asked Questions (FAQs)
What are the basic steps to build a script in Python?
To build a script in Python, start by defining the purpose of the script. Next, set up your development environment by installing Python and an IDE or text editor. Write your code, ensuring to follow Python syntax and conventions. Test the script for errors, and finally, run the script to verify its functionality.
What tools do I need to write a Python script?
You need a text editor or an Integrated Development Environment (IDE) such as PyCharm, Visual Studio Code, or Jupyter Notebook. Additionally, ensure you have Python installed on your system to execute your scripts.
How do I execute a Python script?
To execute a Python script, open a terminal or command prompt, navigate to the directory containing your script, and run the command `python script_name.py`, replacing `script_name.py` with the actual name of your script.
What are some common errors to watch for when building a Python script?
Common errors include syntax errors, indentation errors, and runtime errors. Ensure proper indentation, correct use of variables, and validate data types to minimize these issues.
How can I improve my Python scripting skills?
Improving your Python scripting skills involves practicing regularly, working on projects, studying Python libraries, and engaging with the community through forums or coding challenges. Additionally, reviewing existing code can provide insights into best practices.
What resources are available for learning Python scripting?
Numerous resources are available, including online courses (Coursera, Udemy), documentation (Python.org), books (Automate the Boring Stuff with Python), and community forums (Stack Overflow, Reddit).
Building a script in Python involves several essential steps that guide the developer from concept to execution. First, it is crucial to define the purpose of the script, whether it is for automation, data analysis, web scraping, or another application. This foundational understanding informs the choice of libraries and frameworks that may be necessary for the task. Additionally, outlining the script’s structure and flow helps to organize the code logically, making it easier to develop and maintain.
Next, selecting the appropriate development environment is vital. Python scripts can be written in various Integrated Development Environments (IDEs) or text editors. Popular choices include PyCharm, Visual Studio Code, and Jupyter Notebook, each offering unique features that can enhance productivity. Once the environment is set up, writing the script involves utilizing Python’s syntax and libraries effectively. It is also important to incorporate error handling and testing to ensure the script runs smoothly and produces the desired output.
Finally, after the script is developed, it is essential to document the code thoroughly. Clear comments and documentation not only help others understand the script but also assist the original developer in recalling the script’s functionality in the future. Version control systems, such as Git, can also be employed to track changes and collaborate with others
Author Profile
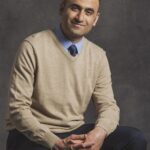
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?