How Can You Effectively Fix JavaScript Errors?
### Introduction
JavaScript is the backbone of modern web development, powering interactive elements and dynamic features that enhance user experience. However, even the most seasoned developers encounter errors that can disrupt their carefully crafted code. Whether you’re a beginner just starting out or a professional troubleshooting a complex application, understanding how to fix JavaScript errors is essential for maintaining a smooth, functional website. In this article, we’ll explore effective strategies and tools that can help you diagnose and resolve common JavaScript issues, ensuring your code runs seamlessly.
### Overview
When faced with JavaScript errors, the first step is to identify the problem. Errors can range from simple syntax mistakes to more complex issues related to logic or asynchronous behavior. By leveraging browser developer tools, you can gain valuable insights into the nature of the error, including line numbers and error messages that guide you toward a solution. Understanding the different types of errors, such as runtime errors, syntax errors, and logical errors, is crucial in developing a systematic approach to debugging.
Once you’ve pinpointed the error, the next phase involves applying various debugging techniques. These may include using console logging to track variable values, stepping through code with breakpoints, or employing error handling practices to gracefully manage unexpected situations. The journey of fixing JavaScript errors not only enhances your coding
Identifying JavaScript Errors
To effectively fix JavaScript errors, the first step is to accurately identify them. JavaScript errors can manifest in several ways, including syntax errors, runtime errors, and logical errors. Each type of error requires a different approach for resolution.
- Syntax Errors: These occur when the code violates the grammar rules of JavaScript. For example, missing parentheses or semicolons.
- Runtime Errors: These happen when the code is syntactically correct but fails during execution, often due to referencing undefined variables or functions.
- Logical Errors: These are errors in the algorithm or logic that produce incorrect results despite the code running without crashing.
Using the browser’s developer tools can significantly aid in identifying errors. Most browsers offer a console that displays error messages along with the line numbers where the errors occur.
Debugging Tools and Techniques
Utilizing debugging tools is crucial for effectively resolving JavaScript errors. The following techniques can streamline the debugging process:
- Console Logging: Use `console.log()` to print variable values and execution flow to the console. This helps trace the state of your application at various points.
- Breakpoints: Setting breakpoints in your code allows you to pause execution and inspect variable values at specific moments.
- Error Stack Traces: Review the stack trace provided in the console when an error occurs. It outlines the sequence of function calls leading to the error.
Tool | Purpose | Usage |
---|---|---|
Chrome DevTools | Inspect and debug web applications | Open via F12 or right-click -> Inspect |
Linting Tools | Identify syntax errors before execution | Integrate with code editors (e.g., ESLint) |
Remote Debugging | Debug code running on remote devices | Use Chrome or Firefox remote debugging features |
Common Error Types and Solutions
Understanding common JavaScript errors and their solutions can expedite the debugging process. Below are some frequently encountered errors and their resolutions:
- Uncaught ReferenceError: This error indicates that a variable is being used before it is defined. To resolve this, ensure variables are declared before use.
- TypeError: Occurs when a value is not of the expected type. For instance, trying to call a method on `undefined`. Check the data types and use conditional checks.
- SyntaxError: Typically occurs due to incorrect syntax. Review the code for missing brackets, commas, or incorrect variable declarations.
- RangeError: This error arises when a value is not within the allowed range, such as passing an invalid number to a function expecting a specific range. Verify the input parameters.
- Timeout Error: This can happen when a script takes too long to execute, especially in asynchronous code. Optimize the code or check for infinite loops.
By systematically following these steps and leveraging the appropriate tools, developers can effectively troubleshoot and fix JavaScript errors, leading to a smoother development process and improved application performance.
Identifying JavaScript Errors
To effectively fix JavaScript errors, the first step is identification. Errors can typically be found through:
- Browser Developer Tools: Most modern browsers have built-in developer tools accessible via right-clicking on the page and selecting “Inspect” or pressing `F12`. The Console tab will display error messages.
- Error Messages: Pay attention to the specific error messages provided. They often include a line number and a brief description of the problem.
- Code Comments: Using comments in your code to isolate sections can help determine where an error occurs.
Common Types of JavaScript Errors
JavaScript errors can be classified into several common types:
Error Type | Description |
---|---|
Syntax Error | Occurs when the code syntax is incorrect (e.g., missing parentheses). |
Reference Error | Happens when a reference is made to a variable that does not exist. |
Type Error | Arises when an operation is performed on an incompatible type. |
Range Error | Occurs when a value is not within the allowable range. |
Eval Error | Specific to the eval() function when the string passed cannot be evaluated. |
Debugging Techniques
Employing effective debugging techniques can streamline the error-fixing process:
- Console Logging: Use `console.log()` to output variable values at various points in your code. This helps track down unexpected values.
- Breakpoints: Set breakpoints in the developer tools to pause execution and inspect the current state of variables and the call stack.
- Step Through the Code: Step through your code line-by-line using the debugger to observe where things may be going awry.
Common Fixes for JavaScript Errors
Once errors are identified, the following common fixes can be applied:
- Correct Syntax Errors:
- Ensure all brackets, parentheses, and quotes are correctly matched.
- Look for missing or extra commas and semicolons.
- Resolve Reference Errors:
- Verify that variables are declared before use.
- Check for typos in variable names or function calls.
- Address Type Errors:
- Ensure that operations are performed on compatible types (e.g., do not add a number to a string without conversion).
- Use type-checking functions like `typeof` to debug types.
- Fix Range Errors:
- Check any arrays or collections being accessed for out-of-bound indices.
- Ensure that the values being processed fall within the expected range.
Best Practices for Preventing JavaScript Errors
To minimize future errors, consider the following best practices:
- Use Strict Mode: Enable strict mode by adding `”use strict”;` at the beginning of your scripts to enforce better coding standards.
- Linting Tools: Utilize tools like ESLint to analyze your code for potential errors and enforce consistent coding styles.
- Modular Code: Break your code into smaller, reusable modules. This makes it easier to isolate and fix issues.
- Unit Testing: Implement unit tests to catch errors early in the development process.
Resources for Further Learning
Consider exploring the following resources to deepen your understanding of JavaScript error handling:
- MDN Web Docs: Comprehensive documentation and guides on JavaScript.
- JavaScript.info: A modern tutorial that covers everything from basic to advanced JavaScript concepts.
- Stack Overflow: A community-driven Q&A platform where you can find solutions to specific JavaScript errors.
Strategies for Resolving JavaScript Errors
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively fix JavaScript errors, it is crucial to utilize debugging tools such as Chrome DevTools. These tools allow developers to inspect code, set breakpoints, and monitor variable states, which can significantly streamline the error identification process.”
Michael Chen (Lead Frontend Developer, Creative Solutions LLC). “A systematic approach to fixing JavaScript errors involves isolating the problematic code. By commenting out sections and reintroducing them gradually, developers can pinpoint the exact line causing the issue, making it easier to implement a solution.”
Sarah Johnson (JavaScript Specialist, CodeMaster Academy). “Understanding the error messages provided by the JavaScript engine is essential. These messages often include line numbers and descriptions that can guide developers toward the root cause of the error, enabling them to address it more efficiently.”
Frequently Asked Questions (FAQs)
How do you identify JavaScript errors?
JavaScript errors can be identified using browser developer tools, which typically include a console that logs errors. Look for red error messages that indicate the type of error and the line number in your code.
What are common types of JavaScript errors?
Common types of JavaScript errors include syntax errors, reference errors, type errors, and range errors. Each type indicates a different issue, such as incorrect syntax, accessing undefined variables, or performing invalid operations.
How can you debug JavaScript code effectively?
Effective debugging can be achieved by using breakpoints in the browser’s developer tools, logging variable values with `console.log()`, and stepping through code execution to observe behavior and identify issues.
What tools can assist in fixing JavaScript errors?
Tools such as browser developer tools (Chrome DevTools, Firefox Developer Edition), linters (ESLint, JSHint), and debugging libraries (Sentry, Rollbar) can assist in identifying and fixing JavaScript errors.
How do you handle asynchronous errors in JavaScript?
Asynchronous errors can be handled using `try…catch` blocks in conjunction with `async/await` syntax or by using `.catch()` method with Promises. This ensures that errors in asynchronous code are properly caught and managed.
What is the role of error handling in JavaScript?
Error handling in JavaScript is crucial for maintaining application stability and user experience. It allows developers to gracefully manage unexpected issues, providing informative feedback to users and preventing application crashes.
Fixing JavaScript errors requires a systematic approach that begins with identifying the nature of the error. Common types of errors include syntax errors, runtime errors, and logical errors. Utilizing browser developer tools can significantly aid in pinpointing these issues, as they provide detailed error messages and stack traces that help developers understand where the problem lies in the code. Additionally, leveraging debugging techniques such as breakpoints and console logging can further facilitate the troubleshooting process.
Once the error is identified, the next step is to analyze the code to determine the root cause. This may involve reviewing variable declarations, function calls, and control structures to ensure they are implemented correctly. It is also beneficial to consult documentation or online resources for clarification on specific JavaScript functions or methods that may be causing the error. Collaboration with peers or seeking assistance from online forums can provide fresh perspectives and solutions.
Finally, after making the necessary corrections, thorough testing is essential to ensure that the fix resolves the issue without introducing new errors. Writing unit tests can help verify that the code behaves as expected. Continuous learning and staying updated with JavaScript best practices will also enhance a developer’s ability to prevent and fix errors effectively in the future.
Author Profile
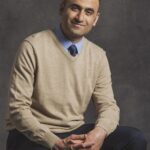
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?