How Does the Range Function Work in Python? A Comprehensive Guide
In the world of Python programming, the `range` function stands out as a fundamental tool that empowers developers to create efficient loops and manage sequences with ease. Whether you’re a novice coder or a seasoned programmer, understanding how `range` operates can significantly enhance your coding capabilities. This seemingly simple function opens the door to a multitude of possibilities, allowing you to generate lists of numbers, iterate through loops, and control the flow of your programs with precision.
At its core, the `range` function generates a sequence of numbers, which can be tailored to fit various programming needs. It provides flexibility in defining the start point, end point, and even the step size of the sequence. This versatility makes it an essential component of Python’s control structures, particularly in `for` loops where it can dictate how many times a block of code should execute.
Moreover, the `range` function is not just about generating numbers; it also plays a crucial role in optimizing performance. By utilizing `range`, Python can handle large datasets without consuming excessive memory, making it a favorite among developers who prioritize efficiency. As we delve deeper into the mechanics of the `range` function, you’ll discover its nuances and best practices that can elevate your programming skills to new heights.
Understanding the Range Function
The `range` function in Python is a built-in utility that generates a sequence of numbers. It is commonly used in for-loops, making it an essential part of programming in Python. The function can accept one to three parameters, allowing for flexibility in how the sequence is created.
Parameters of the Range Function
The `range` function can be called with the following parameters:
- start (optional): The beginning of the sequence. Default is 0.
- stop (required): The end of the sequence. The sequence will not include this value.
- step (optional): The increment (or decrement) between each number in the sequence. Default is 1.
Here is a concise representation of the parameters:
Parameter | Description | Default Value |
---|---|---|
start | Starting value of the sequence | 0 |
stop | Ending value (exclusive) of the sequence | N/A |
step | Difference between each number in the sequence | 1 |
Examples of Using Range
- Basic Usage:
“`python
for i in range(5):
print(i)
“`
This will output:
“`
0
1
2
3
4
“`
- Specifying Start and Stop:
“`python
for i in range(2, 6):
print(i)
“`
Output:
“`
2
3
4
5
“`
- Using Step:
“`python
for i in range(1, 10, 2):
print(i)
“`
Output:
“`
1
3
5
7
9
“`
Negative Steps
The `range` function can also generate sequences in reverse by using a negative step. This is particularly useful for iterating backwards through a list or performing countdowns.
Example:
“`python
for i in range(5, 0, -1):
print(i)
“`
Output:
“`
5
4
3
2
1
“`
Common Use Cases
The `range` function is frequently employed in various scenarios, including but not limited to:
- Iterating through lists or arrays by index
- Generating sequences for mathematical computations
- Creating countdowns or progressions in algorithms
In summary, the `range` function is a versatile tool in Python that simplifies the generation of numerical sequences, enhancing the efficiency and readability of code.
Understanding the Range Function in Python
The `range` function in Python is a built-in function primarily used for generating a sequence of numbers. It is often utilized in loops, particularly `for` loops, to iterate over a sequence of numbers without needing to manually create lists. The function can be called with one, two, or three arguments, allowing for flexible usage.
Syntax of the Range Function
The syntax of the `range` function is as follows:
“`python
range([start], stop[, step])
“`
- start: (Optional) The starting value of the sequence. Defaults to 0 if not specified.
- stop: The endpoint of the sequence, which is not included in the output.
- step: (Optional) The increment between each number in the sequence. Defaults to 1 if not specified.
Examples of Using the Range Function
Here are several examples demonstrating how to use the `range` function effectively:
- Single Argument:
Using only the `stop` argument generates numbers from 0 to `stop – 1`.
“`python
for i in range(5):
print(i)
“`
Output:
“`
0
1
2
3
4
“`
- Two Arguments:
Specifying both `start` and `stop` provides control over the starting point.
“`python
for i in range(2, 8):
print(i)
“`
Output:
“`
2
3
4
5
6
7
“`
- Three Arguments:
Using `step` modifies the increment between numbers.
“`python
for i in range(1, 10, 2):
print(i)
“`
Output:
“`
1
3
5
7
9
“`
Behavior of the Range Function
The `range` function exhibits specific behaviors based on the arguments provided:
Argument Type | Behavior |
---|---|
`start` not specified | Defaults to 0 |
`stop` required | The sequence ends at `stop – 1` |
`step` positive | Increments numbers upwards |
`step` negative | Generates a descending sequence |
For example, to generate numbers in reverse order:
“`python
for i in range(10, 0, -1):
print(i)
“`
Output:
“`
10
9
8
7
6
5
4
3
2
1
“`
Range Object Characteristics
The output of the `range` function is not a list but a `range` object, which is more memory efficient. This object generates numbers on demand. To convert it into a list, use the `list()` function:
“`python
numbers = list(range(5))
print(numbers)
“`
Output:
“`
[0, 1, 2, 3, 4]
“`
Common Use Cases
The `range` function is widely used in Python programming for various purposes:
- Looping through indices: Useful when you need to access items in a list by their index.
- Generating sequences: Ideal for creating sequences of numbers for calculations or iterations.
- Controlling iterations: Employed to limit the number of iterations in loops.
the `range` function is a versatile tool for generating numeric sequences, making it an essential component in Python programming.
Understanding the Range Function in Python: Insights from Experts
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The range function in Python is a built-in function that generates a sequence of numbers, which is particularly useful for iterating over with loops. It allows for customization of the start, stop, and step parameters, providing flexibility in how sequences are generated.”
Michael Chen (Data Scientist, Analytics Pro). “In Python, the range function is often utilized in for loops to control the number of iterations. It can produce a list of integers, making it an essential tool for tasks that require repetition or indexing.”
Laura Simmons (Computer Science Educator, Code Academy). “Understanding how the range function operates is fundamental for anyone learning Python. It not only helps in creating loops but also enhances performance by generating numbers on-the-fly rather than storing them in memory, especially when using range with large datasets.”
Frequently Asked Questions (FAQs)
What is the purpose of the range function in Python?
The range function generates a sequence of numbers, which is commonly used for looping a specific number of times in for loops.
How do you use the range function with a single argument?
When called with a single argument, range(n) generates numbers from 0 to n-1. For example, range(5) produces the sequence 0, 1, 2, 3, 4.
Can you specify a starting point in the range function?
Yes, you can specify a starting point by using two arguments: range(start, stop). This generates numbers from ‘start’ to ‘stop – 1’.
Is it possible to set a step value in the range function?
Yes, the range function can take a third argument to define the step value: range(start, stop, step). This allows you to skip numbers in the generated sequence.
What type of object does the range function return?
The range function returns a range object, which is an immutable sequence type that generates numbers on demand, rather than storing them in memory.
How can you convert a range object to a list?
You can convert a range object to a list by passing it to the list constructor: list(range(start, stop)). This will create a list containing all the numbers in the specified range.
The `range` function in Python is a versatile tool used primarily for generating sequences of numbers. It is commonly utilized in loops, particularly `for` loops, to iterate over a sequence of numbers efficiently. The function can take one, two, or three arguments, allowing for flexibility in defining the start, stop, and step values of the generated sequence. When only one argument is provided, it generates numbers starting from zero up to, but not including, the specified number. With two arguments, the first specifies the starting point, while the second defines the endpoint. The optional third argument allows for specifying the increment, enabling the creation of sequences with custom step sizes.
One of the key advantages of using the `range` function is its ability to generate large sequences without consuming significant memory. This is achieved because `range` returns an iterable object rather than a list, which means that numbers are generated on-the-fly as needed. This feature is particularly beneficial when working with large datasets or when performance is a concern. Additionally, the `range` function supports negative step values, allowing for the creation of decreasing sequences, which further enhances its utility in various programming scenarios.
In summary, the `range` function is an essential component of Python
Author Profile
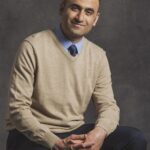
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?