How Can You Access Dictionary Values in Python Effectively?
In the world of programming, dictionaries are one of the most versatile and powerful data structures available in Python. They allow you to store and manage data in a way that mimics real-world associations, making them essential for tasks ranging from data analysis to web development. Whether you’re a beginner looking to grasp the fundamentals or an experienced coder seeking to refine your skills, understanding how to access dictionary values in Python is a crucial step on your journey.
Accessing values in a dictionary is not just about retrieving data; it’s about unlocking the potential of your code. With a simple key-value pairing, you can efficiently manage and manipulate complex datasets. From the straightforward retrieval of a single value to more intricate operations involving nested dictionaries, the methods available in Python empower you to handle data with ease and precision.
As we delve deeper into this topic, you’ll discover various techniques for accessing dictionary values, including the use of keys, methods for safe retrieval, and strategies for handling missing data. By mastering these concepts, you’ll enhance your programming toolkit and open up new avenues for creativity and problem-solving in your projects. So, let’s embark on this exploration of Python dictionaries and uncover the secrets to accessing their valuable contents!
Accessing Values Using Keys
In Python, dictionaries store data in key-value pairs. To access a value, you simply refer to its corresponding key within square brackets. For example:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
print(my_dict[‘name’]) Output: Alice
“`
If the key exists in the dictionary, it will return the associated value. However, if the key does not exist, this will raise a `KeyError`. To avoid errors, you can use the `get()` method, which returns `None` or a specified default value if the key is not found:
“`python
print(my_dict.get(‘name’)) Output: Alice
print(my_dict.get(‘gender’, ‘Not specified’)) Output: Not specified
“`
Accessing Nested Dictionary Values
Dictionaries can also contain other dictionaries, allowing for more complex data structures. To access a value in a nested dictionary, chain the keys together:
“`python
nested_dict = {‘user’: {‘name’: ‘Bob’, ‘age’: 25}}
print(nested_dict[‘user’][‘name’]) Output: Bob
“`
This approach requires that you know the structure of the dictionary in advance. Care should be taken to ensure each key exists at each level of the nesting to avoid `KeyError`.
Iterating Over Dictionary Values
You can iterate through all the values in a dictionary using a `for` loop. This can be particularly useful when you need to process or display all values in the dictionary:
“`python
for value in my_dict.values():
print(value)
“`
This will output:
“`
Alice
30
“`
You can also iterate over keys and values simultaneously using the `items()` method:
“`python
for key, value in my_dict.items():
print(f”{key}: {value}”)
“`
This will yield:
“`
name: Alice
age: 30
“`
Table of Dictionary Access Methods
Here is a comparison of different methods to access dictionary values:
Method | Syntax | Behavior on Missing Key |
---|---|---|
Square Bracket Notation | `dict[key]` | Raises KeyError |
get() Method | `dict.get(key)` | Returns None or default value |
setdefault() Method | `dict.setdefault(key, default)` | Returns value or sets default |
Using Default Values with setdefault()
The `setdefault()` method can be particularly useful when you want to ensure a key exists in the dictionary. It checks if the key is present and, if not, adds the key with a default value:
“`python
my_dict.setdefault(‘gender’, ‘Not specified’)
print(my_dict) Output: {‘name’: ‘Alice’, ‘age’: 30, ‘gender’: ‘Not specified’}
“`
This method allows you to initialize keys with default values easily while accessing their values simultaneously.
Summary of Access Techniques
To summarize, dictionary values in Python can be accessed through various methods, each suited to different scenarios. Understanding these techniques not only aids in writing efficient code but also in managing data effectively.
Accessing Dictionary Values Using Keys
In Python, dictionaries are collections of key-value pairs. To access the value associated with a specific key, you can use square brackets or the `get()` method.
- Using square brackets: This method raises a `KeyError` if the key does not exist in the dictionary.
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
age = my_dict[‘age’] Returns 30
“`
- Using the `get()` method: This method returns `None` (or a specified default value) if the key does not exist, preventing errors.
“`python
age = my_dict.get(‘age’) Returns 30
height = my_dict.get(‘height’, ‘Not available’) Returns ‘Not available’
“`
Accessing Values with Iteration
You can access all the values in a dictionary by iterating through it. This can be done using a `for` loop.
“`python
for value in my_dict.values():
print(value)
“`
This will print each value in the dictionary, allowing you to process them as needed.
Accessing Values with Keys in a List
If you have a list of keys and wish to access their corresponding values, you can use a list comprehension.
“`python
keys = [‘name’, ‘age’]
values = [my_dict[key] for key in keys if key in my_dict]
“`
This snippet creates a list of values for the specified keys, ensuring that it only includes keys that exist in the dictionary.
Accessing Values in Nested Dictionaries
Dictionaries can contain other dictionaries. To access values in nested dictionaries, you can chain the keys.
“`python
nested_dict = {‘person’: {‘name’: ‘Alice’, ‘age’: 30}, ‘city’: ‘Wonderland’}
name = nested_dict[‘person’][‘name’] Returns ‘Alice’
“`
In this example, the value of `name` is accessed through two keys.
Using Dictionary Comprehensions for Value Access
Dictionary comprehensions can be used for accessing and transforming values. For instance, if you want to create a new dictionary with modified values, you can do so easily.
“`python
squared_ages = {key: value ** 2 for key, value in my_dict.items() if isinstance(value, int)}
“`
This creates a new dictionary where the values are the squares of the original ages, assuming the values are integers.
Accessing Values with Conditionals
Sometimes, you may need to access values based on certain conditions. Using conditional statements can help filter the keys you’re interested in.
“`python
for key in my_dict:
if my_dict[key] > 25: Example condition
print(f”{key}: {my_dict[key]}”)
“`
This code prints only those keys and values where the value exceeds 25.
Using the `items()` Method
The `items()` method returns a view object that displays a list of a dictionary’s key-value tuple pairs. This can be useful for accessing both keys and values simultaneously.
“`python
for key, value in my_dict.items():
print(f”Key: {key}, Value: {value}”)
“`
This method allows for comprehensive access to all elements within the dictionary, facilitating operations that require both keys and values.
Expert Insights on Accessing Dictionary Values in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Accessing dictionary values in Python is straightforward, utilizing the key associated with the desired value. The syntax is simple: you can retrieve a value using `dict[key]`, which raises a KeyError if the key does not exist. For safer access, the `get()` method is recommended, as it allows you to specify a default value if the key is absent.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “In Python, dictionaries are versatile data structures that allow for efficient value retrieval. When accessing values, it is crucial to ensure that the key exists to avoid exceptions. Using the `in` keyword to check for key existence before access can prevent runtime errors and enhance code reliability.”
Sarah Thompson (Data Scientist, Analytics Hub). “Dictionaries in Python are not only convenient for storing key-value pairs but also offer various methods for accessing values. The `items()` method can be particularly useful when you need to iterate over both keys and values simultaneously, providing a more comprehensive approach to data manipulation.”
Frequently Asked Questions (FAQs)
How do I access a value in a dictionary using a key?
You can access a value in a dictionary by using square brackets with the key inside, like this: `value = my_dict[key]`.
What happens if I try to access a key that does not exist in the dictionary?
If you attempt to access a key that does not exist, Python will raise a `KeyError`. To avoid this, you can use the `get()` method, which returns `None` or a specified default value if the key is missing.
Can I access dictionary values using a variable as the key?
Yes, you can use a variable to access dictionary values. For example, if `key` is a variable containing the key name, you can access the value with `value = my_dict[key]`.
Is there a way to access all values in a dictionary without specifying keys?
Yes, you can access all values in a dictionary using the `values()` method. This returns a view object containing all the values: `all_values = my_dict.values()`.
How can I check if a key exists in a dictionary before accessing its value?
You can check for a key’s existence using the `in` keyword. For example: `if key in my_dict:` allows you to safely access the value without encountering a `KeyError`.
Can I access nested dictionary values in Python?
Yes, you can access nested dictionary values by chaining keys. For example, if `my_dict` contains another dictionary under the key `nested`, you can access a value with `value = my_dict[‘nested’][‘key’]`.
Accessing dictionary values in Python is a fundamental skill that is essential for effective programming. Dictionaries in Python are collections of key-value pairs, allowing for efficient data retrieval. To access a value, one simply uses the corresponding key within square brackets or the `get()` method. The square bracket method raises a KeyError if the key does not exist, while `get()` allows for a default value to be returned if the key is absent, enhancing code robustness.
Additionally, understanding the different methods available for accessing dictionary values can significantly improve code readability and maintainability. For instance, using the `items()` method allows for iteration over both keys and values, while `keys()` and `values()` provide access to just the keys or values, respectively. This versatility enables developers to handle dictionaries in a way that best suits their specific needs.
In summary, mastering how to access dictionary values in Python not only streamlines data manipulation but also fosters better coding practices. By leveraging the various methods available, programmers can write more efficient and error-resistant code. As dictionaries are a core data structure in Python, proficiency in their use is invaluable for anyone looking to excel in the language.
Author Profile
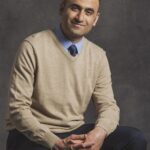
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?