How Can You Easily Add Numbers in Python?
In the world of programming, the ability to perform basic arithmetic operations is fundamental, and Python makes this task both intuitive and efficient. Whether you’re a beginner taking your first steps into coding or an experienced developer looking to refresh your skills, understanding how to add numbers in Python is essential. This simple yet powerful operation serves as the building block for more complex calculations and algorithms. In this article, we will explore the various ways to add numbers in Python, showcasing the language’s versatility and ease of use.
Adding numbers in Python is not just about the syntax; it encompasses a range of concepts from basic data types to more advanced structures. At its core, Python allows users to perform addition using straightforward operators, making it accessible for all skill levels. However, as you delve deeper, you’ll discover that Python’s capabilities extend beyond simple arithmetic, enabling you to manipulate and aggregate data in innovative ways.
Throughout this article, we will guide you through the different methods of adding numbers in Python, including using built-in functions and exploring how to handle various data types. By the end, you’ll not only be proficient in performing addition but also equipped with the knowledge to tackle more complex mathematical challenges in your programming journey. So, let’s dive in and unlock the power of addition in Python!
Basic Addition of Numbers
In Python, adding numbers can be performed using the `+` operator. This operator can be applied to integers, floats, and even complex numbers. For example, when you want to add two integers, you can simply write:
“`python
a = 5
b = 3
result = a + b
print(result) Output: 8
“`
Similarly, for floating-point numbers:
“`python
x = 2.5
y = 4.5
result = x + y
print(result) Output: 7.0
“`
Adding Multiple Numbers
To add multiple numbers, you can use a combination of the `+` operator or the built-in `sum()` function. The `sum()` function is particularly useful when working with a list or tuple of numbers, as it simplifies the process.
For example, to add a list of numbers:
“`python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) Output: 15
“`
You can also use `sum()` on a tuple:
“`python
numbers_tuple = (10, 20, 30)
total = sum(numbers_tuple)
print(total) Output: 60
“`
Using Functions for Addition
Creating a custom function for addition can enhance code readability and reusability. Here’s how you can define and use a function that adds two numbers:
“`python
def add_numbers(num1, num2):
return num1 + num2
result = add_numbers(10, 15)
print(result) Output: 25
“`
This function can be easily extended to handle more than two numbers by using the `*args` syntax:
“`python
def add_multiple_numbers(*args):
return sum(args)
result = add_multiple_numbers(1, 2, 3, 4)
print(result) Output: 10
“`
Handling Different Data Types
When adding numbers in Python, it’s important to be aware of data types. The addition operator can yield different results based on the types of the operands. Here are some considerations:
- Integers and Floats: Adding an integer and a float will result in a float.
- Strings: If you attempt to add strings, Python will concatenate them instead of performing arithmetic.
- Type Error: Attempting to add incompatible types, such as a string and an integer, will raise a `TypeError`.
For example:
“`python
a = 10 integer
b = 5.5 float
result = a + b
print(result) Output: 15.5
Concatenation
s1 = “Hello, ”
s2 = “world!”
greeting = s1 + s2
print(greeting) Output: Hello, world!
Type Error example
c = “10” + 5 This will raise a TypeError
“`
Table of Addition Examples
Type of Addition | Example | Output |
---|---|---|
Integer + Integer | 5 + 3 | 8 |
Float + Float | 2.5 + 4.5 | 7.0 |
Integer + Float | 5 + 2.5 | 7.5 |
String + String | “Hello” + ” World” | “Hello World” |
Adding Numbers Using the Addition Operator
In Python, the simplest way to add two or more numbers is to use the addition operator (`+`). This operator can be applied to integers, floats, and even complex numbers.
“`python
Adding integers
result_int = 5 + 10
print(result_int) Output: 15
Adding floats
result_float = 5.5 + 2.5
print(result_float) Output: 8.0
Adding complex numbers
result_complex = 1 + 2j + 2 + 3j
print(result_complex) Output: (3+5j)
“`
Adding Numbers in a List
To sum a list of numbers, Python provides the built-in `sum()` function. This function iterates through the elements of the list and returns their total.
“`python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) Output: 15
“`
For more complex calculations, such as summing only specific elements or applying conditions, a list comprehension or generator expression can be utilized.
“`python
Sum only even numbers
total_even = sum(num for num in numbers if num % 2 == 0)
print(total_even) Output: 6
“`
Adding Numbers with User Input
In scenarios where numbers are provided by the user, the `input()` function can be employed. It is important to convert the input from string format to numeric format (integer or float) before performing any addition.
“`python
User input for two numbers
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
result = num1 + num2
print(“The sum is:”, result)
“`
Adding Numbers in a Function
Creating a function can encapsulate the addition logic for reuse. This is particularly useful when adding multiple numbers or when the addition operation is part of a larger program.
“`python
def add_numbers(*args):
return sum(args)
Example usage
result_function = add_numbers(10, 20, 30)
print(result_function) Output: 60
“`
Adding Numbers with NumPy
For large datasets or numerical computations, the NumPy library offers efficient methods for adding numbers in arrays. The `numpy.add()` function can be used for element-wise addition of two arrays.
“`python
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result_numpy = np.add(array1, array2)
print(result_numpy) Output: [5 7 9]
“`
This method is particularly advantageous when working with large arrays due to its optimized performance.
Adding Numbers in Pandas DataFrames
When dealing with tabular data, the Pandas library allows for addition across DataFrame columns and rows. The `sum()` method can be applied to compute the total for a specific axis.
“`python
import pandas as pd
data = {‘A’: [1, 2, 3], ‘B’: [4, 5, 6]}
df = pd.DataFrame(data)
Sum across columns
column_sum = df.sum(axis=0)
print(column_sum) Output: A 6, B 15
Sum across rows
row_sum = df.sum(axis=1)
print(row_sum) Output: 0 5, 1 7, 2 9
“`
Expert Insights on Adding Numbers in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Adding numbers in Python is straightforward due to its dynamic typing and built-in arithmetic operators. The simplicity of using the ‘+’ operator allows for quick calculations, making it an excellent choice for both beginners and experienced developers.”
James Patel (Python Instructor, Code Academy). “To effectively add numbers in Python, one should understand the importance of data types. For instance, adding integers and floats requires awareness of type conversion, which can be done using the ‘float()’ function to ensure accurate results.”
Linda Xu (Data Scientist, Analytics Hub). “In data analysis, adding numbers can often involve aggregating data from various sources. Utilizing Python libraries such as NumPy can enhance performance and efficiency when performing large-scale arithmetic operations.”
Frequently Asked Questions (FAQs)
How do I add two numbers in Python?
You can add two numbers in Python using the `+` operator. For example, `result = a + b`, where `a` and `b` are the numbers you want to add.
Can I add more than two numbers in Python?
Yes, you can add multiple numbers by chaining the `+` operator. For instance, `result = a + b + c` will sum all three variables.
What data types can I add in Python?
In Python, you can add integers, floats, and even strings (if they represent numbers). However, adding incompatible types will raise a `TypeError`.
How do I add numbers from user input in Python?
You can use the `input()` function to get user input and convert it to a number using `int()` or `float()`. For example: `sum = int(input(“Enter first number: “)) + int(input(“Enter second number: “))`.
Is there a built-in function to add numbers in Python?
Python does not have a specific built-in function solely for addition, but you can use the `sum()` function to add elements of an iterable, such as a list. For example, `total = sum([a, b, c])`.
Can I add numbers in a list in Python?
Yes, you can use the `sum()` function to add all numbers in a list. For example, `total = sum(my_list)` will return the sum of all elements in `my_list`.
In Python, adding numbers is a fundamental operation that can be performed using the addition operator (+). This operator can be utilized with various numeric data types, including integers and floating-point numbers. Python’s dynamic typing allows for seamless addition between different numeric types, making it a versatile choice for mathematical operations. Furthermore, Python supports the addition of multiple numbers in a single expression, enhancing code efficiency and readability.
One of the key takeaways is the simplicity and clarity of Python’s syntax for arithmetic operations. Unlike some other programming languages, Python’s approach to adding numbers does not require complex syntax or additional libraries for basic operations. This accessibility makes it an excellent choice for beginners and experienced programmers alike. Additionally, Python’s built-in functions, such as sum(), can be utilized to add elements in a list or other iterable collections, providing further flexibility in handling numerical data.
Moreover, Python’s handling of numeric types allows for precise calculations without the need for explicit type conversion in most cases. However, it is essential to be aware of potential pitfalls, such as the differences in behavior when adding integers and floats, which can lead to unexpected results due to floating-point precision issues. Understanding these nuances is crucial for effective programming and ensures accurate results in numerical computations.
Author Profile
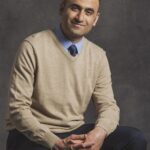
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?