How Can You Easily Add Two Numbers in Python?
In the world of programming, mastering the basics is essential for building a strong foundation. One of the simplest yet most fundamental tasks in any programming language is the ability to perform arithmetic operations, such as adding two numbers. Whether you’re a complete beginner or someone looking to brush up on your Python skills, understanding how to add numbers in Python is a crucial stepping stone on your coding journey. This seemingly simple operation opens the door to more complex concepts and applications, making it an important skill to learn.
Adding two numbers in Python is not just about the mechanics; it’s about grasping the underlying principles of how programming languages handle data and operations. Python, known for its readability and simplicity, provides a straightforward approach to arithmetic that allows even novice programmers to quickly grasp the concept. In this article, we will explore the various methods to add numbers in Python, from basic operations to more advanced techniques, ensuring that you have a comprehensive understanding of this essential function.
As we delve into the topic, you will discover how Python’s syntax and built-in functions facilitate seamless calculations. We will also touch on the importance of data types and how they influence arithmetic operations. By the end of this exploration, you will not only know how to add two numbers in Python but also appreciate the elegance and efficiency
Using Basic Operators
To add two numbers in Python, you can simply use the `+` operator. This operator can be applied to both integers and floating-point numbers, making it versatile for various numeric operations. Here’s a simple example:
“`python
a = 5
b = 3
result = a + b
print(result) Output: 8
“`
In this code snippet, the variables `a` and `b` are assigned the values 5 and 3, respectively. The addition operation is performed, and the result is printed out.
Using Functions for Addition
Defining a function to handle addition can enhance code readability and reusability. This is particularly useful when you need to perform addition multiple times throughout your code. Here’s how you can create a function to add two numbers:
“`python
def add_numbers(x, y):
return x + y
result = add_numbers(10, 20)
print(result) Output: 30
“`
In this example, the `add_numbers` function takes two parameters and returns their sum. This method allows you to easily add different pairs of numbers without rewriting the addition logic.
Handling User Input
If you want to add numbers input by the user, you can use the `input()` function. This function captures user input as a string, so you will need to convert it to a numeric type before performing addition. Here’s a demonstration:
“`python
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
sum_result = num1 + num2
print(“The sum is:”, sum_result)
“`
This code prompts the user to enter two numbers, converts them to floats, and then adds them together.
Adding Multiple Numbers
When adding multiple numbers, you can use the `sum()` function along with a list of numbers. This approach is efficient and simplifies code when dealing with more than two values. Here’s an example:
“`python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(“The total sum is:”, total) Output: 15
“`
This snippet creates a list of numbers and computes the total sum using the `sum()` function.
Table of Addition Examples
To illustrate various scenarios of adding numbers in Python, consider the following table:
Example | Code Snippet | Output |
---|---|---|
Add Two Integers | a + b |
8 |
Add Two Floats | 5.5 + 4.5 |
10.0 |
Add User Input | input() |
Varies |
Add Multiple Numbers | sum([1, 2, 3]) |
6 |
This table encapsulates different methods to perform addition in Python, showcasing the flexibility of the language for handling numerical operations.
Basic Addition of Two Numbers
In Python, adding two numbers is straightforward. You can utilize the `+` operator to perform addition directly between numeric types. Here’s a simple example:
“`python
num1 = 5
num2 = 10
result = num1 + num2
print(result) Output: 15
“`
The above code snippet demonstrates how to add two integers. The `+` operator takes the values of `num1` and `num2`, computes their sum, and stores it in the variable `result`.
Adding Floating-Point Numbers
Adding floating-point numbers follows the same principle as integers. Python can handle decimal numbers seamlessly. Here’s an example:
“`python
float1 = 5.5
float2 = 10.2
result = float1 + float2
print(result) Output: 15.7
“`
In this case, `float1` and `float2` are added together, showcasing Python’s ability to work with different numeric types.
Using Functions for Addition
To improve code reusability and organization, defining a function for addition is beneficial. Here’s how to create a simple function:
“`python
def add_numbers(a, b):
return a + b
result = add_numbers(3, 7)
print(result) Output: 10
“`
The `add_numbers` function takes two parameters, `a` and `b`, and returns their sum. This function can be called with any numeric types, enhancing flexibility.
Adding Numbers from User Input
To add numbers provided by a user, you can utilize the `input()` function. Note that input values are captured as strings, so conversion to a numeric type is necessary:
“`python
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
result = num1 + num2
print(f”The sum is: {result}”)
“`
This code allows users to input their values, which are then converted to floats before performing the addition.
Error Handling in Addition
When adding numbers, it is prudent to implement error handling to manage potential input errors. Using a `try` and `except` block helps catch exceptions effectively:
“`python
try:
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
result = num1 + num2
print(f”The sum is: {result}”)
except ValueError:
print(“Invalid input. Please enter numeric values.”)
“`
This approach ensures that if a user enters non-numeric data, the program will not crash and will provide a helpful message instead.
Advanced Addition: Using NumPy
For more complex scenarios, especially involving arrays or matrices, the NumPy library is a powerful tool. Here’s how to use NumPy for addition:
“`python
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = array1 + array2
print(result) Output: [5 7 9]
“`
NumPy facilitates element-wise addition, making it particularly useful for operations on large datasets.
Following these methods, you can effectively add two numbers in Python, whether they are integers, floating-point numbers, or elements of arrays.
Expert Insights on Adding Two Numbers in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Adding two numbers in Python is a straightforward task that can be accomplished using the ‘+’ operator. This simplicity is one of the reasons Python is favored for educational purposes, as it allows beginners to grasp fundamental programming concepts without unnecessary complexity.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “When adding two numbers in Python, it is important to ensure that both variables are of compatible types, such as integers or floats. This avoids type errors and ensures that the arithmetic operation behaves as expected, especially when dealing with user input or data from external sources.”
Sarah Johnson (Computer Science Educator, Future Coders Academy). “In teaching how to add two numbers in Python, I emphasize the importance of using clear variable names and comments in the code. This not only aids in readability but also helps students understand the logic behind their calculations, fostering better coding practices from the start.”
Frequently Asked Questions (FAQs)
How do you add two numbers in Python?
To add two numbers in Python, you can use the `+` operator. For example, `result = a + b`, where `a` and `b` are the numbers you want to add.
What data types can be added in Python?
In Python, you can add integers, floats, and even strings (if they are compatible). For instance, you can add two integers or two floats directly, while adding strings concatenates them.
Can I add more than two numbers in Python?
Yes, you can add more than two numbers by using the `+` operator multiple times, or by using the `sum()` function, which can take an iterable of numbers as an argument.
What happens if I try to add incompatible data types?
If you attempt to add incompatible data types, such as an integer and a string, Python will raise a `TypeError`, indicating that the operation is not supported for the given types.
Is there a built-in function for adding numbers in Python?
Python does not have a specific built-in function solely for addition, but the `sum()` function can be used to add elements in an iterable, such as a list or a tuple.
Can I add numbers from user input in Python?
Yes, you can add numbers from user input by using the `input()` function to capture the input and then converting it to the appropriate numeric type (e.g., `int()` or `float()`) before performing the addition.
In Python, adding two numbers is a straightforward process that can be accomplished using the addition operator (+). This operator can be applied to various numeric data types, including integers and floats, allowing for flexibility in handling different numerical inputs. The syntax is simple, as one only needs to specify the two numbers to be added, either directly or through variables that store the values.
To illustrate this, consider the following example: if you have two variables, `a` and `b`, representing the numbers you wish to add, you can perform the addition by writing `result = a + b`. This will store the sum in the variable `result`, which can then be used for further calculations or output. Additionally, Python’s dynamic typing means that you do not need to declare the data type of the variables beforehand, making the process even more intuitive.
In summary, adding two numbers in Python is an essential skill that forms the foundation for more complex programming tasks. The simplicity of the syntax and the flexibility of data types make it accessible for beginners while remaining powerful for experienced developers. Understanding this basic operation is crucial for anyone looking to enhance their programming capabilities in Python.
Author Profile
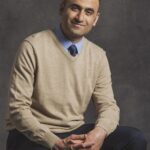
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?