How Can You Call a Function Stored in a Dictionary in Python?
In the dynamic world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow developers to store and manage data in key-value pairs, making it easy to access and manipulate information. But did you know that dictionaries can also hold functions? This unique capability opens up a realm of possibilities for organizing and executing code in a more structured and efficient manner. If you’ve ever wondered how to leverage this feature to call functions stored within a dictionary, you’re in the right place.
Understanding how to call a function in a dictionary is not just about syntax; it’s about enhancing your programming toolkit. By embedding functions within dictionaries, you can create dynamic and flexible code that adapts to different scenarios with ease. This approach can lead to cleaner code and improved readability, as it allows for a more organized way to manage related functionalities. Whether you’re looking to streamline your code or explore advanced programming techniques, grasping this concept is essential.
As we delve deeper into this topic, we’ll explore the mechanics of defining and invoking functions stored in dictionaries, along with practical examples that illustrate their utility. You’ll learn how to harness this feature to create more efficient and maintainable code, ultimately elevating your Python programming skills to new heights. Get ready to unlock the potential of dictionaries and functions in
Storing Functions in a Dictionary
In Python, a dictionary can hold various data types, including functions. This feature allows for dynamic function calls based on keys. To store a function in a dictionary, you can simply assign the function name (without parentheses) to a key.
Example:
python
def greet():
return “Hello!”
def farewell():
return “Goodbye!”
functions_dict = {
“greet”: greet,
“farewell”: farewell
}
In this example, we have two functions, `greet` and `farewell`, stored in the `functions_dict` dictionary.
Calling Functions from a Dictionary
To call a function stored in a dictionary, access it using its key and then invoke it with parentheses. This allows for flexible function execution based on runtime conditions.
Example:
python
# Calling the greet function
print(functions_dict[“greet”]()) # Output: Hello!
# Calling the farewell function
print(functions_dict[“farewell”]()) # Output: Goodbye!
Dynamic Function Calls with Arguments
If your functions require arguments, you can still utilize a dictionary for dynamic calling. You would pass the required arguments when invoking the function.
Example:
python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
math_operations = {
“add”: add,
“subtract”: subtract
}
# Calling the add function with arguments
print(math_operations[“add”](5, 3)) # Output: 8
# Calling the subtract function with arguments
print(math_operations[“subtract”](5, 3)) # Output: 2
Table of Function Calls in a Dictionary
The following table summarizes the function names, their keys in the dictionary, and their outputs when called:
Function Name | Dictionary Key | Output |
---|---|---|
greet | greet | Hello! |
farewell | farewell | Goodbye! |
add | add | 8 (for inputs 5, 3) |
subtract | subtract | 2 (for inputs 5, 3) |
This approach of using dictionaries to store and call functions enhances code organization and allows for more dynamic programming styles.
Calling Functions Stored in a Dictionary
In Python, dictionaries can store not only data but also functions as values. This capability allows for dynamic function execution based on keys, enhancing code flexibility and organization. Here’s how to call a function stored in a dictionary.
Defining Functions and Storing Them
First, define functions that you want to store in a dictionary. For example:
python
def greet(name):
return f”Hello, {name}!”
def farewell(name):
return f”Goodbye, {name}!”
Next, create a dictionary to hold these functions:
python
function_dict = {
‘greet’: greet,
‘farewell’: farewell
}
Calling Functions from the Dictionary
To call a function from the dictionary, use the corresponding key and parentheses for any required arguments. The syntax is straightforward:
python
name = “Alice”
result_greet = function_dict[‘greet’](name)
result_farewell = function_dict[‘farewell’](name)
print(result_greet) # Output: Hello, Alice!
print(result_farewell) # Output: Goodbye, Alice!
Handling Missing Keys
When accessing functions via keys, it is essential to handle potential missing keys to avoid runtime errors. This can be managed using the `get` method, which returns `None` (or a specified default value) if the key does not exist:
python
func = function_dict.get(‘welcome’, None)
if func:
print(func(name))
else:
print(“Function not found.”)
Dynamic Function Calls
You can also use variables to dynamically select which function to call. For example:
python
action = ‘greet’ # This could be determined at runtime
if action in function_dict:
print(function_dict[action](name)) # Calls the greet function
else:
print(“Action not recognized.”)
Example with Parameters
If the functions require multiple parameters, ensure that you pass them correctly. Here’s an example with additional parameters:
python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
math_operations = {
‘add’: add,
‘subtract’: subtract
}
result_add = math_operations[‘add’](5, 3) # Output: 8
result_subtract = math_operations[‘subtract’](5, 3) # Output: 2
Table of Function Usage
The following table summarizes how to define, store, and call functions from a dictionary:
Action | Code Example |
---|---|
Define Function | `def function_name(args): …` |
Store in Dictionary | `my_dict = {‘key’: function_name}` |
Call Function | `my_dict[‘key’](args)` |
Handle Missing Key | `my_dict.get(‘key’, default_value)` |
Utilizing dictionaries to store and call functions can significantly streamline your code by promoting modularity and simplifying function management.
Understanding Function Calls in Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, calling a function stored in a dictionary is straightforward. You simply access the function by its key and use parentheses to invoke it. This allows for dynamic function execution based on user input or other conditions.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “Utilizing dictionaries to store functions enhances code modularity and readability. By mapping function names to their respective implementations, developers can easily manage and call functions dynamically, which is particularly useful in event-driven programming.”
Linda Zhao (Python Educator, LearnPython Academy). “When teaching how to call a function in a dictionary, I emphasize the importance of ensuring that the stored values are callable. This means checking if the value associated with a key is indeed a function, which can be done using the callable() function in Python.”
Frequently Asked Questions (FAQs)
How can I store functions in a dictionary in Python?
You can store functions in a dictionary by assigning them as values to keys. For example:
python
def greet():
return “Hello!”
functions_dict = {‘greet’: greet}
How do I call a function stored in a dictionary?
To call a function stored in a dictionary, access it using its key and then invoke it with parentheses. For example:
python
result = functions_dict[‘greet’]()
Can I pass arguments to a function stored in a dictionary?
Yes, you can pass arguments by including them in the function call. For instance:
python
def add(x, y):
return x + y
functions_dict = {‘add’: add}
result = functions_dict[‘add’](5, 3)
What happens if I try to call a non-existent function in a dictionary?
If you try to call a non-existent function using a key that does not exist, Python will raise a `KeyError`. Ensure the key exists before calling the function.
Can I store lambda functions in a dictionary?
Yes, lambda functions can also be stored in a dictionary just like regular functions. For example:
python
functions_dict = {‘square’: lambda x: x ** 2}
result = functions_dict[‘square’](4)
Is it possible to store methods in a dictionary?
Yes, methods can be stored in dictionaries in the same way as functions. Ensure you reference the method correctly, especially if it belongs to a class instance.
In Python, calling a function stored within a dictionary is a straightforward process that leverages the dynamic nature of the language. By associating functions with keys in a dictionary, you can create a flexible and organized way to manage and invoke these functions based on specific conditions or inputs. To call a function from a dictionary, you simply access the function using its corresponding key and then use parentheses to execute it, allowing for both clarity and efficiency in your code.
One of the key advantages of using dictionaries to store functions is the ability to easily modify or extend the functionality of your program. By adding new functions to the dictionary or changing existing mappings, you can adapt your application without significant rewrites. This approach also enhances readability, as it groups related functionalities together, making the code easier to maintain and understand.
Additionally, this method supports the implementation of various design patterns, such as the command pattern, where you can map commands to functions. This flexibility allows for more dynamic and interactive applications, as you can execute different functions based on user input or other runtime conditions. Overall, utilizing dictionaries for function calls in Python is a powerful technique that promotes modularity and scalability in programming.
Author Profile
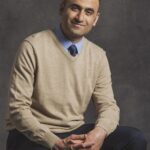
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?