How Can You Change the Background Color Using JavaScript?
### Introduction
In the vibrant world of web development, the ability to manipulate the visual elements of a webpage is a fundamental skill that can elevate user experience and engagement. One of the simplest yet most impactful changes you can make is altering the background color of a webpage using JavaScript. Whether you’re looking to create a dynamic interface that responds to user interactions or simply want to refresh the aesthetic of your site, understanding how to change background colors programmatically can open up a realm of creative possibilities.
In this article, we will explore the various methods available for changing background colors using JavaScript, from basic techniques to more advanced approaches that involve event handling and animations. You’ll discover how to easily implement these changes in your projects, allowing for a more interactive and visually appealing experience for your users. By the end of this guide, you’ll have a solid grasp of how to manipulate the Document Object Model (DOM) to achieve stunning visual effects with just a few lines of code.
Whether you’re a beginner eager to learn the ropes of JavaScript or an experienced developer looking to brush up on your skills, this article will provide you with the insights and practical examples you need to effectively change background colors in your web applications. Get ready to dive into the code and transform your web pages from ordinary to
Methods to Change Background Color
Changing the background color in JavaScript can be accomplished through various methods, primarily by manipulating the Document Object Model (DOM). Below are the most common approaches:
Using the `style` Property
The simplest way to change an element’s background color is by using the `style` property directly on the DOM element. Here’s how you can do it:
javascript
document.body.style.backgroundColor = “lightblue”;
This line of code sets the background color of the entire webpage to light blue. You can target specific elements as well:
javascript
document.getElementById(“myElement”).style.backgroundColor = “yellow”;
In this case, `myElement` would be the ID of the element whose background color you wish to change.
Using CSS Classes
Another efficient method is to define CSS classes and apply them using JavaScript. This approach is beneficial for managing multiple styles and maintaining cleaner code.
- First, define CSS classes in a stylesheet:
css
.bg-light {
background-color: lightblue;
}
.bg-dark {
background-color: darkblue;
}
- Then, use JavaScript to add or remove these classes:
javascript
document.body.classList.add(“bg-light”); // Adds the light background
document.body.classList.remove(“bg-dark”); // Removes the dark background
Changing Background Color on Events
JavaScript allows background colors to change dynamically based on user interactions, such as clicks or mouse movements. Here’s an example of changing the background color on a button click:
javascript
document.getElementById(“changeColorBtn”).onclick = function() {
document.body.style.backgroundColor = “lightgreen”;
};
This code changes the background color of the body to light green whenever the button is clicked.
Using Random Colors
For a more dynamic effect, you can generate random colors. Here’s an example that changes the background color to a random value:
javascript
function getRandomColor() {
const letters = ‘0123456789ABCDEF’;
let color = ‘#’;
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
document.getElementById("changeColorBtn").onclick = function() {
document.body.style.backgroundColor = getRandomColor();
};
This code defines a function that generates a random hex color and applies it to the background when the button is clicked.
Comparison Table of Background Color Methods
Method | Pros | Cons |
---|---|---|
Style Property | Simple and direct | Can become messy with multiple styles |
CSS Classes | Cleaner and reusable | Requires additional CSS management |
Event-Based | Interactive and dynamic | Can lead to complex logic |
Random Colors | Fun and engaging | Less control over color choices |
Changing Background Color Using JavaScript
To change the background color of a webpage or specific elements using JavaScript, you can utilize the `style` property of the DOM elements. Below are several methods to achieve this, ranging from simple inline changes to more dynamic approaches.
Changing Background Color of the Entire Document
To change the background color of the entire webpage, you can modify the `backgroundColor` property of the `document.body` object. Here is a straightforward example:
javascript
document.body.style.backgroundColor = “lightblue”;
This code snippet sets the background color of the entire document to light blue. You can replace `”lightblue”` with any valid CSS color value, such as hex codes, RGB, or named colors.
Changing Background Color of Specific Elements
To target a specific element, you first need to select it using methods like `getElementById`, `getElementsByClassName`, or `querySelector`. Here’s how to change the background color of a specific element:
javascript
document.getElementById(“myDiv”).style.backgroundColor = “yellow”;
In this example, the background color of the `
Dynamic Background Color Changes
JavaScript can also be used to create dynamic changes, such as changing the background color in response to user actions. Here’s an example using an event listener:
javascript
document.getElementById(“colorButton”).addEventListener(“click”, function() {
document.body.style.backgroundColor = “pink”;
});
In this case, clicking the button will change the background color of the entire document to pink.
Using CSS Classes for Background Color Changes
Another efficient way to manage background colors is through CSS classes. This method is particularly useful if you want to apply multiple styles easily. First, define CSS classes:
css
.bg-red {
background-color: red;
}
.bg-green {
background-color: green;
}
.bg-blue {
background-color: blue;
}
Then, you can toggle these classes using JavaScript:
javascript
const button = document.getElementById(“colorButton”);
button.addEventListener(“click”, function() {
document.body.classList.toggle(“bg-blue”);
});
This code toggles the `bg-blue` class on the body, changing its background color each time the button is clicked.
Table of Background Color Methods
Method | Description | Example |
---|---|---|
Inline Style | Directly sets the background color. | `document.body.style.backgroundColor = “red”;` |
Element Selector | Targets specific elements for background changes. | `document.getElementById(“myDiv”).style.backgroundColor = “green”;` |
Event Listener | Changes color based on user actions. | `button.addEventListener(“click”, function() {…});` |
CSS Class Toggle | Uses CSS classes for managing styles. | `document.body.classList.toggle(“bg-red”);` |
By employing these techniques, you can effectively change and manage background colors on your webpage using JavaScript, enhancing both aesthetics and user interactivity.
Expert Insights on Changing Background Color in JavaScript
Emily Chen (Senior Front-End Developer, Tech Innovations Inc.). “Changing the background color in JavaScript can be achieved using the `style` property of an HTML element. For example, `document.body.style.backgroundColor = ‘blue’;` will effectively set the background color of the entire page to blue. It is essential to ensure that the color values are correctly formatted, whether using named colors, HEX, or RGB values.”
Michael Patel (JavaScript Framework Specialist, CodeCraft Academy). “Utilizing JavaScript to change background colors dynamically enhances user experience significantly. I recommend using event listeners to trigger background color changes based on user interactions, such as clicks or hovers. This approach not only makes the interface more engaging but also allows for a more interactive design.”
Sarah Thompson (Web Development Instructor, Digital Learning Hub). “One effective method to change background colors in JavaScript is to apply CSS classes dynamically. By defining CSS classes with desired background colors and toggling them using `classList.add()` or `classList.remove()`, developers can maintain cleaner code and leverage CSS transitions for smoother visual effects.”
Frequently Asked Questions (FAQs)
How can I change the background color of a webpage using JavaScript?
You can change the background color of a webpage by modifying the `document.body.style.backgroundColor` property. For example: `document.body.style.backgroundColor = ‘blue’;`.
Is it possible to change the background color of a specific element using JavaScript?
Yes, you can change the background color of a specific element by selecting it with `document.getElementById` or other DOM selection methods, and then setting its `style.backgroundColor` property. For example: `document.getElementById(‘myElement’).style.backgroundColor = ‘red’;`.
Can I use RGB or HEX values to set the background color in JavaScript?
Absolutely. You can use both RGB and HEX color values to set the background color. For example: `document.body.style.backgroundColor = ‘rgb(255, 0, 0)’;` or `document.body.style.backgroundColor = ‘#ff0000’;`.
How do I change the background color dynamically based on user input?
You can use an event listener to capture user input, such as from a color picker, and then apply the selected color to the background. For example:
javascript
document.getElementById(‘colorPicker’).addEventListener(‘input’, function() {
document.body.style.backgroundColor = this.value;
});
What is the difference between inline styles and CSS classes when changing background color?
Inline styles directly modify an element’s style property in JavaScript, while CSS classes allow for reusable styles defined in a stylesheet. Using classes can enhance maintainability and separation of concerns.
Can I animate the background color change using JavaScript?
Yes, you can animate background color changes using CSS transitions in combination with JavaScript. By adding a class with a transition property, you can smoothly change the background color over a specified duration.
In summary, changing the background color in JavaScript can be achieved through various methods, primarily by manipulating the Document Object Model (DOM). The most common approach involves accessing the `style` property of an HTML element, such as the `document.body`, and setting its `backgroundColor` attribute to the desired color value. This can be done using either inline styles or by applying CSS classes dynamically.
Additionally, JavaScript offers flexibility in how colors can be specified. Developers can use color names, hexadecimal values, RGB, or RGBA formats, allowing for a wide range of visual effects. Understanding these options enables developers to create more dynamic and visually appealing web applications.
Moreover, event-driven programming can enhance user interaction by allowing background color changes in response to user actions, such as clicks or mouse movements. This interactivity can significantly improve user experience and engagement on a website.
mastering the techniques for changing background colors in JavaScript not only enriches a developer’s toolkit but also contributes to creating more engaging and responsive web designs. By leveraging the power of JavaScript and the flexibility of CSS, developers can effectively enhance the aesthetic appeal of their web applications.
Author Profile
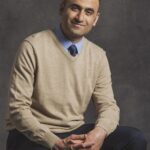
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?