How Can You Clear the Python Shell for a Fresh Start?
If you’ve ever found yourself working in the Python shell, you know how quickly your workspace can become cluttered with lines of code, outputs, and error messages. The Python interactive shell is a powerful tool for testing snippets of code, debugging, and learning the language, but it can also become overwhelming when you’re trying to focus on a specific task. Clearing the screen can significantly enhance your coding experience, providing a clean slate that allows for better concentration and organization. In this article, we will explore various methods to clear the Python shell, making your coding sessions more efficient and enjoyable.
When you’re deep in the throes of coding, the last thing you want is a crowded terminal filled with outdated information. Fortunately, Python offers several straightforward techniques to clear the shell, whether you’re using the command line, an integrated development environment (IDE), or even a Jupyter notebook. Each method has its own advantages, catering to different workflows and preferences. Understanding these options can streamline your coding process, allowing you to focus on what truly matters: writing clean, effective code.
As we delve into the various strategies for clearing the Python shell, we will also touch on the underlying principles that make these methods effective. Whether you’re a seasoned developer or a newcomer to the world of Python,
Clearing the Python Shell
When working in the Python shell, it’s common to want to clear the screen to declutter the view and focus on the current tasks. Clearing the shell can be done using different methods depending on the operating system being used. Here are the most effective ways to achieve this:
Clearing in Windows
To clear the Python shell on a Windows system, you can use the `cls` command. This command can be executed by importing the `os` module and utilizing the `system` function to call the command directly. Here’s how to do it:
“`python
import os
os.system(‘cls’)
“`
Alternatively, if you are using an Integrated Development Environment (IDE) or an interactive shell that supports keyboard shortcuts, you might also clear the output using:
- Ctrl + L (in some shells)
- Ctrl + Shift + L (in others)
Clearing in macOS and Linux
For users operating on macOS or Linux, the command to clear the Python shell is `clear`. Similar to the Windows method, you can use the following code snippet:
“`python
import os
os.system(‘clear’)
“`
Again, many IDEs or interactive shells support keyboard shortcuts for this function. You can typically use:
- Cmd + K (on macOS)
- Ctrl + L (on Linux)
Using Custom Functions
If you frequently need to clear the screen, you may consider creating a custom function that abstracts this functionality. Here’s a sample implementation that works across different operating systems:
“`python
import os
def clear_screen():
if os.name == ‘nt’: For Windows
os.system(‘cls’)
else: For macOS and Linux
os.system(‘clear’)
Call the function
clear_screen()
“`
This function checks the operating system and executes the appropriate command, allowing for a seamless experience irrespective of the environment.
Considerations for Interactive Environments
When working within interactive environments like Jupyter Notebooks or IPython, the method to clear the output differs slightly:
- In Jupyter Notebooks, you can clear the output of a cell by clicking on **Cell > All Output > Clear** from the menu.
- Alternatively, you can use the following command:
“`python
from IPython.display import clear_output
clear_output(wait=True)
“`
This command clears the output of the current cell, and the `wait=True` parameter allows for smoother transitions when re-executing cells.
Operating System | Command |
---|---|
Windows | cls |
macOS | clear |
Linux | clear |
By incorporating these methods and considerations, you can efficiently manage your workspace in the Python shell and enhance your productivity.
Methods to Clear the Python Shell
Clearing the Python shell can be beneficial for improving readability and managing output. Different methods can be employed depending on the environment in which the Python shell is running. Below are the primary techniques.
Using the Built-in Functions
While the Python shell does not have a built-in clear command, you can achieve a similar effect with the following methods:
- Using `os.system`:
You can utilize the `os` module to send a command to the operating system that clears the screen.
“`python
import os
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
This command checks the operating system type and runs the appropriate command (`cls` for Windows and `clear` for Unix/Linux systems).
Keyboard Shortcuts
Most integrated development environments (IDEs) and terminal interfaces offer keyboard shortcuts to clear the console. Common shortcuts include:
– **IDEs like PyCharm or VS Code**:
– **Clear Output**: Often `Ctrl + L` or `Cmd + K` (Mac).
– **Jupyter Notebooks**:
– **Clear Output**: Click on the “Cell” menu and select “All Output” > “Clear”.
Using Terminal Commands in Interactive Mode
When working in an interactive terminal or command line interface, you can use the following commands:
- For Unix/Linux/Mac:
- Simply type `clear` and press Enter.
- For Windows:
- Type `cls` and press Enter.
These commands will clear the visible output from the terminal without affecting your Python session.
Clear Function in IPython and Jupyter Notebooks
In IPython or Jupyter Notebook environments, you can use:
- Magic Commands:
- `%clear` can be used within an IPython shell to clear the console.
- Jupyter Notebook:
- Use `from IPython.display import clear_output` followed by `clear_output(wait=True)` to clear the output of the cell.
Integrating Clear Functionality into Scripts
You can create a reusable function within your Python scripts to clear the shell whenever necessary. Here’s an example:
“`python
import os
def clear_shell():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
You can call `clear_shell()` anytime within your script to clear the output.
Considerations for Usage
While clearing the shell can enhance clarity, consider the following:
- Impact on Debugging: Clearing the output can remove valuable context when debugging.
- Environment Compatibility: Ensure that the method used is compatible with the environment to avoid errors.
- User Experience: Frequent clearing may disrupt the workflow of users who prefer to see command history.
By employing these techniques, you can effectively manage the output in your Python shell, enhancing both functionality and user experience.
Expert Insights on Clearing the Python Shell
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To clear the Python shell, you can use the command `import os` followed by `os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)`. This command effectively clears the terminal screen across different operating systems, providing a clean slate for your coding tasks.”
Michael Chen (Python Instructor, Code Academy). “In interactive Python environments like IDLE, you can simply use the shortcut Ctrl + L to clear the shell. This is a quick and efficient way to declutter your workspace without needing to type any commands.”
Sarah Thompson (Lead Developer, Open Source Projects). “For those using Jupyter Notebooks, clearing the output of a cell can be done by selecting ‘Clear All Outputs’ from the ‘Cell’ menu. This feature helps maintain focus on the code without distractions from previous outputs.”
Frequently Asked Questions (FAQs)
How can I clear the Python shell screen?
You can clear the Python shell screen by using the `os` module. Import the module and call `os.system(‘cls’)` for Windows or `os.system(‘clear’)` for Unix/Linux systems.
Is there a shortcut to clear the Python shell?
There is no built-in keyboard shortcut specifically for clearing the Python shell. However, you can use the commands mentioned above in your code to achieve the same effect.
Can I clear the shell using a command in IDLE?
Yes, in IDLE, you can clear the shell by selecting “Shell” from the menu and then clicking “Restart Shell.” This will clear the current session and reset the shell.
Does clearing the shell affect the variables in memory?
Clearing the shell does not affect the variables in memory. The variables remain accessible until the Python interpreter is restarted or the session is terminated.
Are there any third-party libraries that can help clear the shell?
While there are no specific third-party libraries for clearing the shell, you can use libraries like `curses` for more advanced terminal control, including clearing the screen.
Can I automate the clearing of the shell in my scripts?
Yes, you can automate the clearing of the shell by including the appropriate `os.system` command at the beginning of your script to clear the screen each time the script runs.
In summary, clearing the Python shell can be achieved through various methods depending on the environment in which the shell is being used. For instance, in the standard Python interactive shell, the command `import os; os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)` can be executed to clear the screen. This command checks the operating system type and executes the appropriate command to clear the terminal screen, enhancing the user experience by providing a clean workspace.
Additionally, Integrated Development Environments (IDEs) like PyCharm or Jupyter Notebooks offer built-in functionalities to clear the console or output cells. In Jupyter, for example, users can clear the output by selecting the appropriate option from the menu or using keyboard shortcuts. Understanding these methods is crucial for maintaining an organized coding environment, especially during extensive coding sessions.
Moreover, it is important to note that clearing the shell does not affect the state of the variables or the code that has been executed. The variables remain in memory, allowing users to continue working without losing their progress. This distinction is vital for users who may be concerned about losing their work when clearing the display.
knowing how to effectively clear the Python shell enhances productivity
Author Profile
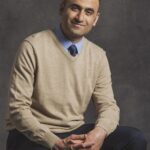
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?