How Can You Clear the Screen in Python Effectively?
In the world of programming, clarity and organization are paramount, especially when it comes to visual output. Whether you’re developing a simple command-line application or a complex interactive program, the ability to manage the display of information can significantly enhance user experience. One common task that many Python developers encounter is the need to clear the screen. This seemingly simple action can make a world of difference, allowing users to focus on the most relevant information without the distraction of previous outputs. In this article, we’ll explore various methods to achieve this in Python, catering to different operating systems and use cases.
Clearing the screen in Python can be accomplished through a variety of techniques, each suited to different environments and preferences. From utilizing built-in libraries to executing system commands, there are multiple pathways to achieve a clean slate. Understanding these methods not only helps in maintaining a tidy output but also empowers developers to create more dynamic and user-friendly applications.
As we delve deeper into the topic, we will examine the most effective ways to clear the console screen in Python, considering both cross-platform solutions and those tailored for specific operating systems. Whether you are a beginner looking to enhance your coding skills or an experienced developer seeking to refine your output management, this guide will provide you with the insights and tools necessary to master this
Using System Calls to Clear the Screen
One of the most common methods to clear the console screen in Python is by utilizing system calls. This approach varies depending on the operating system you are using. The `os` module provides a way to execute shell commands directly from Python.
To clear the screen, you can use the following code snippet:
python
import os
def clear_screen():
# Check the operating system
if os.name == ‘nt’: # For Windows
os.system(‘cls’)
else: # For Linux and Mac
os.system(‘clear’)
This function checks whether the operating system is Windows (`nt`) or Unix-based (Linux/Mac) and executes the appropriate command to clear the console.
Using ANSI Escape Sequences
Another method for clearing the screen is by using ANSI escape sequences, which are supported by many terminal emulators. This method does not require any external module and can be implemented as follows:
python
def clear_screen():
print(“\033[H\033[J”)
This command uses the escape sequence to move the cursor to the home position and clear the screen.
Using a Third-Party Library
For more complex applications, you might consider using a third-party library, such as `curses`, which provides an interface for creating text-based user interfaces. Here’s a simple example of clearing the screen using `curses`:
python
import curses
def clear_screen():
stdscr = curses.initscr() # Initialize the window
stdscr.clear() # Clear the window
stdscr.refresh() # Refresh to show changes
curses.endwin() # End the curses application
Comparison of Methods
The following table summarizes the advantages and disadvantages of each method to clear the screen in Python:
Method | Advantages | Disadvantages |
---|---|---|
System Calls | Simple to implement, works across platforms | Dependent on the OS, can be slower |
ANSI Escape Sequences | Fast, no dependencies | May not work in all terminal emulators |
Third-Party Libraries (curses) | Powerful for UI applications | More complex, requires understanding of library |
Choosing the appropriate method to clear the screen in Python largely depends on the specific requirements of your application and the environment in which it is running.
Methods to Clear the Screen in Python
Clearing the screen in Python can be achieved through various methods depending on the operating system and the context in which your Python script runs. Below are the most common approaches:
Using OS Module
The `os` module provides a straightforward way to clear the console screen. You can utilize the `system` function to issue a command to the underlying operating system.
python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
- Explanation:
- The function checks the OS name; if it is ‘nt’ (Windows), it uses the `cls` command. For other operating systems (like UNIX, Linux, or macOS), it uses the `clear` command.
Using ANSI Escape Sequences
For terminal environments that support ANSI escape sequences, you can clear the screen using a simple print statement.
python
def clear_screen():
print(“\033[H\033[J”)
- Explanation:
- `\033[H` moves the cursor to the top-left corner.
- `\033[J` clears the screen from the cursor down.
In Interactive Environments
If you are working within an interactive Python shell, such as IPython or Jupyter Notebooks, you can clear the output using specific functions.
- IPython:
python
from IPython.display import clear_output
clear_output(wait=True)
- Jupyter Notebook:
python
from IPython.display import clear_output
clear_output()
- Explanation:
- The `clear_output` function removes the output from the cell, and the `wait=True` parameter ensures that only the latest output is displayed.
Using a Custom Function
If you prefer a more reusable approach, creating a custom function can be beneficial. Here’s an example that combines the methods mentioned:
python
def clear_screen():
try:
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
except Exception as e:
print(“Failed to clear screen:”, e)
- Explanation:
- This function attempts to clear the screen and handles any exceptions that might arise, providing a safer execution.
Choosing the appropriate method to clear the screen in Python depends on your development environment and the specific requirements of your application. Each of these methods is effective in its context, providing flexibility for developers.
Expert Insights on Clearing the Screen in Python
Dr. Emily Carter (Senior Software Engineer, CodeTech Solutions). “Clearing the screen in Python can be accomplished using different methods depending on the operating system. The most common approach is to use the ‘os’ module to execute system commands like ‘cls’ for Windows or ‘clear’ for Unix-based systems. This ensures that the screen is cleared effectively in a cross-platform manner.”
Michael Chen (Python Developer Advocate, Tech Innovations Inc.). “While many developers rely on simple system calls to clear the console, I recommend encapsulating this functionality within a function. This not only promotes code reusability but also enhances readability. A well-structured function can handle various environments and provide a cleaner interface for screen management.”
Sarah Patel (Lead Educator, Python Programming Academy). “For beginners, understanding how to clear the screen in Python is essential for creating interactive applications. I often suggest using libraries like ‘curses’ for more advanced terminal manipulation, as it provides a robust way to manage the screen and user input, allowing for a more dynamic user experience.”
Frequently Asked Questions (FAQs)
How can I clear the console screen in Python on Windows?
To clear the console screen in Python on Windows, you can use the command `os.system(‘cls’)`. Ensure to import the `os` module before executing this command.
What command should I use to clear the screen in Python on macOS or Linux?
On macOS or Linux, you can clear the console screen by using `os.system(‘clear’)`. Again, make sure to import the `os` module prior to using this command.
Is there a cross-platform way to clear the screen in Python?
Yes, you can use the following code snippet to clear the screen in a cross-platform manner:
python
import os
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
This checks the operating system and executes the appropriate command.
Can I create a function to clear the screen in Python?
Yes, you can define a function to encapsulate the screen-clearing logic. For example:
python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
You can then call `clear_screen()` whenever needed.
Does clearing the screen affect the program’s performance?
Clearing the screen does not significantly impact the performance of a Python program. However, frequent screen clearing may affect user experience by making it difficult to track output history.
Are there any libraries that can help with clearing the screen in Python?
While the built-in `os` module is commonly used, libraries like `curses` can also manage screen clearing and more complex terminal manipulations. However, `curses` is primarily for Unix-like systems.
Clearing the screen in Python can be accomplished using various methods, depending on the operating system being used. For Windows users, the command `cls` can be executed through the `os` module, while Unix-based systems, including Linux and macOS, utilize the command `clear`. These commands can be invoked using the `os.system()` function, which allows Python to interface with the underlying command line.
Additionally, there are alternative approaches for clearing the console that do not rely on external commands. For instance, printing multiple new lines can effectively push previous content out of view, although this method does not truly clear the screen. For more interactive environments, such as Jupyter notebooks, specific commands or functions tailored to those platforms may be required to achieve a similar effect.
In summary, understanding how to clear the screen in Python is essential for creating user-friendly console applications. By leveraging the appropriate commands for the user’s operating system or utilizing alternative methods, developers can enhance the readability of their output and improve the overall user experience. Mastery of these techniques is a valuable skill for any Python programmer.
Author Profile
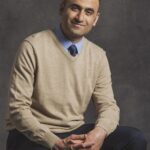
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?