How Can You Code a Website Using Python?
Creating a website can seem like a daunting task, especially if you’re venturing into the world of coding for the first time. However, with the right tools and knowledge, you can harness the power of Python to build a dynamic and engaging web application. Python, known for its simplicity and versatility, has become a popular choice among developers for web development. Whether you’re looking to create a personal blog, a portfolio site, or a complex web application, understanding how to code a website in Python can open up a world of possibilities.
In this article, we’ll explore the fundamental concepts and frameworks that make Python an excellent choice for web development. From setting up your environment to understanding the core principles of web frameworks like Flask and Django, we will guide you through the essential steps to get your website up and running. You’ll learn how Python’s readability and extensive libraries can streamline the development process, allowing you to focus on creativity and functionality.
As we delve deeper, you will discover how to structure your project, handle user input, and manage databases—all crucial components of a successful web application. By the end of this journey, you’ll not only have the skills to code a website in Python but also the confidence to explore more advanced features and functionalities. Get ready to unlock your potential and bring your web ideas
Choosing a Web Framework
When coding a website in Python, selecting an appropriate web framework is crucial. Python offers several frameworks that simplify the process of building web applications. The most popular include:
- Flask: A lightweight and flexible micro-framework ideal for small applications.
- Django: A high-level framework that promotes rapid development and clean, pragmatic design.
- FastAPI: Designed for building APIs quickly with automatic interactive documentation.
Each framework has unique features that cater to different project requirements. Below is a comparison table of these frameworks:
Framework | Use Case | Features | Learning Curve |
---|---|---|---|
Flask | Small to medium apps | Minimalist, highly customizable | Low |
Django | Large applications | Built-in admin, ORM, security features | Medium |
FastAPI | APIs | Asynchronous support, auto-generated docs | Medium |
Setting Up Your Environment
Before you start coding, you need to set up your development environment. This typically involves installing Python and the chosen framework. Here are the steps:
- Install Python: Download the latest version of Python from the official website and follow the installation instructions for your operating system.
- Set Up a Virtual Environment: This helps manage dependencies for your project without conflicts.
- Run `python -m venv venv` to create a virtual environment.
- Activate it using `source venv/bin/activate` on macOS/Linux or `venv\Scripts\activate` on Windows.
- Install the Framework: Use pip to install your chosen framework. For example:
- For Flask: `pip install Flask`
- For Django: `pip install Django`
- For FastAPI: `pip install fastapi[all]`
Creating Your First Web Application
After setting up your environment, you can create a simple web application. Here’s a brief example using Flask:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
In this code snippet, you define a basic Flask application with one route, which returns “Hello, World!” when accessed. Running this code will start a local development server.
Routing and Views
Routing is essential in web development as it determines how URLs correspond to functions in your application. In Flask, routes are defined using the `@app.route` decorator, mapping a URL to a specific function. For example:
“`python
@app.route(‘/about’)
def about():
return “This is the About page.”
“`
In Django, routing is handled through URLconf. You define URL patterns in the `urls.py` file, mapping URLs to views. An example is shown below:
“`python
from django.urls import path
from . import views
urlpatterns = [
path(”, views.home, name=’home’),
path(‘about/’, views.about, name=’about’),
]
“`
By organizing your routes effectively, you ensure that users can navigate your application intuitively.
Templates and Static Files
Templates allow you to separate your application’s logic from the presentation. Both Flask and Django use templating engines (Jinja2 for Flask and Django’s built-in template system). This enables dynamic content rendering in your HTML files.
For static files such as CSS and JavaScript, ensure they are stored in designated directories. In Flask, you typically have a `/static` folder, while Django uses `/static` as well, with settings to manage static files.
Overall, understanding these fundamental concepts will aid in creating a robust web application using Python.
Choosing a Web Framework
Selecting the appropriate web framework is crucial for developing a website in Python. The most popular frameworks include:
- Flask: A micro-framework that is lightweight and easy to use for small applications.
- Django: A high-level framework that encourages rapid development and clean, pragmatic design for larger applications.
- FastAPI: Designed for building APIs quickly with automatic interactive documentation.
Framework | Pros | Cons |
---|---|---|
Flask | Simple, flexible, and minimal overhead | Requires more setup for larger applications |
Django | Built-in features, ORM, and admin panel | Can be overly complex for small projects |
FastAPI | High performance and easy to use for APIs | Less mature than Flask and Django |
Setting Up Your Development Environment
To begin coding your website, you need to set up your development environment. Follow these steps:
- Install Python: Ensure you have Python installed. Use the command:
“`bash
python –version
“`
- Create a Virtual Environment: This isolates your project dependencies.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows: myenv\Scripts\activate
“`
- Install the Framework: Use pip to install your chosen framework.
“`bash
pip install Flask For Flask
pip install Django For Django
pip install fastapi For FastAPI
“`
Building the Basic Structure
Once your environment is set up, create the basic structure of your web application. This typically includes:
- Main Application File: Entry point for your application (e.g., `app.py` for Flask).
- Templates Folder: Contains HTML files for rendering views.
- Static Folder: Stores CSS, JavaScript, and images.
For a Flask application, your directory structure may look like this:
“`
/my_flask_app
/static
/templates
app.py
“`
Creating Routes
In web development, routes are essential for defining how URLs map to functions. Here is how to set up routes in Flask and Django.
Flask Example:
“`python
from flask import Flask, render_template
app = Flask(__name__)
@app.route(‘/’)
def home():
return render_template(‘index.html’)
if __name__ == ‘__main__’:
app.run(debug=True)
“`
Django Example:
- Create a new Django project and app:
“`bash
django-admin startproject myproject
cd myproject
django-admin startapp myapp
“`
- Add a view in `myapp/views.py`:
“`python
from django.http import HttpResponse
def home(request):
return HttpResponse(“Hello, World!”)
“`
- Map the view in `myproject/urls.py`:
“`python
from django.urls import path
from myapp import views
urlpatterns = [
path(”, views.home),
]
“`
Implementing Templates and Static Files
Templates allow you to separate your HTML from Python code. In Flask, you can use Jinja2 templating.
Flask Template Example (`index.html`):
“`html
Welcome to My Flask App
“`
In Django, you would use the Django template language similarly, placing your templates in the `templates` directory and static files in the `static` directory.
Running Your Application
To see your website in action, you need to run the application.
Flask:
“`bash
python app.py
“`
Django:
“`bash
python manage.py runserver
“`
Your application will typically be accessible at `http://127.0.0.1:5000` for Flask and `http://127.0.0.1:8000` for Django.
Expert Insights on Coding Websites with Python
Dr. Emily Carter (Senior Software Engineer, Web Innovations Inc.). “When coding a website in Python, leveraging frameworks such as Django or Flask is essential. These frameworks provide robust tools and libraries that streamline the development process and enhance security, making it easier to build scalable web applications.”
Michael Thompson (Lead Developer, Tech Solutions Group). “Understanding the basics of HTML, CSS, and JavaScript is crucial for any Python web developer. While Python handles the backend, a solid grasp of frontend technologies ensures a seamless integration and a better user experience.”
Sarah Lin (Web Development Instructor, Code Academy). “For beginners, I recommend starting with Flask due to its simplicity and flexibility. It allows new developers to grasp the core concepts of web development without overwhelming them with too many features at once.”
Frequently Asked Questions (FAQs)
What frameworks can I use to code a website in Python?
You can use frameworks such as Django, Flask, and FastAPI. Django is ideal for large applications due to its built-in features, while Flask is suitable for smaller projects and offers more flexibility. FastAPI is great for building APIs quickly.
Do I need to know HTML and CSS to code a website in Python?
Yes, knowledge of HTML and CSS is essential for creating the front-end of a website. Python handles the back-end logic, while HTML and CSS are used for structuring and styling the user interface.
Can I create a dynamic website using Python?
Absolutely. Python frameworks like Django and Flask enable the development of dynamic websites that can interact with databases and provide user-specific content.
What database options are available when coding a website in Python?
Popular database options include PostgreSQL, MySQL, SQLite, and MongoDB. The choice depends on your project requirements, such as scalability and data structure.
How do I deploy a Python web application?
You can deploy a Python web application using platforms like Heroku, AWS, or DigitalOcean. Each platform provides documentation and tools to help you get your application online.
Are there any resources for learning how to code a website in Python?
Yes, numerous resources are available, including online courses on platforms like Coursera and Udemy, as well as documentation from the respective Python frameworks. Books and tutorials are also widely accessible.
coding a website in Python involves understanding several key frameworks and tools that facilitate web development. Popular frameworks such as Flask and Django provide robust environments for building web applications, each catering to different project requirements. Flask is ideal for smaller applications and offers flexibility, while Django is suited for larger projects that require a more structured approach with built-in features like authentication and an ORM.
Additionally, it is essential to grasp the fundamentals of HTML, CSS, and JavaScript, as these technologies complement Python in creating dynamic and visually appealing web pages. Python can handle the backend logic, while HTML and CSS manage the frontend presentation. Integrating these technologies allows developers to create seamless user experiences.
Moreover, leveraging tools such as virtual environments and version control systems like Git enhances the development process. These tools help manage dependencies and track changes in the code, ensuring a more organized workflow. Testing frameworks and deployment strategies, including using cloud services, are also critical components of the web development lifecycle in Python.
Ultimately, mastering website coding in Python requires a blend of technical skills, familiarity with various frameworks, and an understanding of web technologies. By focusing on these areas, developers can create efficient, scalable, and maintainable web applications that meet user
Author Profile
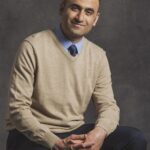
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?