How Can You Effectively Comment Out Code in JavaScript?
### Introduction
In the world of programming, clarity and organization are paramount, especially when it comes to writing and maintaining code. Among the various techniques that developers employ to enhance code readability, commenting stands out as a fundamental practice. For those venturing into JavaScript, understanding how to effectively comment out code can be a game-changer. Whether you’re debugging, collaborating with others, or simply trying to make sense of your own work, comments serve as invaluable guides that can illuminate your thought process and intentions.
Commenting in JavaScript is not just about leaving notes for yourself or others; it’s a powerful tool that can help streamline your coding workflow. By learning the different methods to comment out code, you can easily disable sections of your script without deleting them, making it easier to test and refine your code. This practice not only aids in debugging but also allows you to document your thought process and share insights with fellow developers, fostering better collaboration and understanding.
As we delve deeper into the nuances of commenting in JavaScript, we will explore various techniques and best practices that can elevate your coding experience. Whether you’re a novice programmer or a seasoned developer, mastering the art of commenting will undoubtedly enhance your ability to write clean, maintainable code. Get ready to unlock the secrets of effective commenting and
Single-Line Comments
In JavaScript, single-line comments can be created using two forward slashes (`//`). Everything following these slashes on that line will be ignored by the JavaScript interpreter. This is particularly useful for adding quick notes or disabling code temporarily.
Example:
javascript
// This is a single-line comment
console.log(“This will run.”); // This will also run
Multi-Line Comments
For comments that span multiple lines, you can use the `/*` and `*/` syntax. This method allows you to comment out blocks of code or provide detailed explanations without cluttering the code with multiple single-line comments.
Example:
javascript
/*
This is a multi-line comment.
It can span multiple lines.
*/
console.log(“This will also run.”);
Best Practices for Commenting
Effective commenting enhances code readability and maintainability. Here are some best practices to consider:
- Be Clear and Concise: Comments should explain the “why” behind the code, not just the “what.”
- Avoid Redundant Comments: Do not comment on every line; focus on complex logic or important decisions.
- Update Comments: Always ensure comments are updated as the code evolves to avoid misleading information.
Examples of Effective Comments
Consider the following examples that illustrate the proper use of comments in JavaScript code:
javascript
// Calculate the area of a circle
const radius = 5;
const area = Math.PI * radius * radius; // Area formula: πr²
console.log(area);
/*
The following function checks if a number is even or odd.
It returns true for even numbers and for odd numbers.
*/
function isEven(num) {
return num % 2 === 0;
}
Commenting Out Code During Development
During the development process, you may want to temporarily disable certain lines of code without deleting them. This can be accomplished by commenting them out. This practice allows for easier debugging and testing.
Example:
javascript
// console.log(“This line is temporarily disabled.”);
console.log(“This line will execute.”);
Table of Comment Usage
The following table summarizes when to use single-line versus multi-line comments:
Comment Type | Usage Scenario |
---|---|
Single-Line Comment (`//`) | Use for brief explanations or notes about a specific line of code. |
Multi-Line Comment (`/* … */`) | Use for detailed descriptions, documentation, or commenting out blocks of code. |
Commenting
Utilizing comments effectively can significantly improve the clarity and maintainability of JavaScript code. By adhering to best practices and understanding when to employ different types of comments, developers can create more readable and manageable codebases.
Commenting in JavaScript
In JavaScript, comments are used to annotate code, making it easier to understand for others (or for yourself at a later time). They can be single-line or multi-line, depending on the context and needs of your code.
Single-line Comments
Single-line comments start with two forward slashes (`//`). Everything following the `//` on that line will be ignored by the JavaScript engine. This is useful for brief notes or explanations.
Example:
javascript
// This is a single-line comment
let x = 5; // This initializes x to 5
Multi-line Comments
Multi-line comments are enclosed between `/*` and `*/`. This style is beneficial for longer explanations or when you need to comment out blocks of code. Everything between these markers will be ignored.
Example:
javascript
/*
This is a multi-line comment
that can span multiple lines
*/
let y = 10; /* This initializes y to 10 */
Best Practices for Using Comments
- Be Concise: Comments should be clear and to the point. Avoid verbose explanations; instead, focus on clarity.
- Update Comments: Regularly review and update comments to reflect any changes in the code. Outdated comments can lead to confusion.
- Avoid Obvious Comments: Comments should add value. For instance, commenting that `let x = 5; // Set x to 5` is redundant and unnecessary.
- Use Comments to Explain Why: Instead of just stating what the code does, explain why certain decisions were made, which can be more beneficial for future developers.
Commenting Out Code
In addition to providing explanations, comments can be used to temporarily disable code without deleting it. This practice is particularly useful during debugging or testing.
Example of commenting out code:
javascript
// let z = x + y; // This line is commented out and won’t execute
let result = x * y; // This line will execute
Limitations and Considerations
Aspect | Single-line Comments | Multi-line Comments |
---|---|---|
Syntax | `//` | `/* … */` |
Line Breaks | Can only comment on one line | Can span multiple lines |
Nesting | Cannot be nested | Can be nested, but with caution |
Usage Scenario | Quick notes or short explanations | Longer explanations or multiple lines of code |
Using comments effectively enhances code readability and maintainability, creating a more collaborative environment for developers. Proper commenting can significantly ease the onboarding of new team members and facilitate smoother code reviews.
Understanding Commenting Techniques in JavaScript
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). “Commenting in JavaScript is essential for maintaining code readability. Developers can use single-line comments with ‘//’ for brief notes or multi-line comments enclosed within ‘/* */’ for more extensive explanations. This practice not only aids in collaboration but also enhances code maintenance.”
Michael Chen (Lead JavaScript Developer, Tech Solutions Group). “Effective commenting is a hallmark of professional coding. In JavaScript, utilizing comments strategically can clarify complex logic and document the purpose of functions. It is vital to strike a balance; excessive commenting can clutter the code, while insufficient comments can lead to misunderstandings.”
Sarah Thompson (JavaScript Educator, Web Development Academy). “Teaching newcomers how to comment out code in JavaScript is fundamental. I emphasize the importance of using comments to disable code during debugging. This allows developers to isolate issues without deleting code, fostering a better understanding of the programming process.”
Frequently Asked Questions (FAQs)
How do I comment out a single line in JavaScript?
You can comment out a single line in JavaScript by using two forward slashes `//` at the beginning of the line. For example: `// This is a comment`.
What is the syntax for multi-line comments in JavaScript?
Multi-line comments in JavaScript are enclosed between `/*` and `*/`. For instance: `/* This is a multi-line comment */`.
Can I comment out code in JavaScript that is inside a function?
Yes, you can comment out any code inside a function using either single-line or multi-line comment syntax. The comments will not affect the execution of the code.
Are comments in JavaScript ignored during execution?
Yes, comments are completely ignored by the JavaScript interpreter and do not affect the execution of the code.
Is there a difference between single-line and multi-line comments in JavaScript?
Yes, single-line comments are suitable for brief annotations, while multi-line comments are used for longer explanations or to comment out blocks of code.
Can I use comments to temporarily disable code in JavaScript?
Absolutely. Comments are often used to temporarily disable code during development or debugging without deleting it.
Commenting in JavaScript is an essential practice for developers, as it enhances code readability and maintainability. There are two primary methods for adding comments: single-line comments and multi-line comments. Single-line comments are initiated with two forward slashes (//), allowing developers to annotate specific lines of code succinctly. Multi-line comments, on the other hand, are enclosed between /* and */, making them suitable for longer explanations or temporarily disabling blocks of code.
Utilizing comments effectively can significantly improve collaboration among team members and facilitate easier debugging. By providing context and explanations, comments serve as a guide for anyone reviewing the code, whether they are the original author or a new team member. This practice is particularly valuable in complex projects where understanding the logic behind certain implementations is crucial.
In summary, mastering the art of commenting in JavaScript is a fundamental skill that contributes to better coding practices. Developers should strive to use comments judiciously, ensuring they add value without cluttering the code. By doing so, they foster a more efficient development process and create a more accessible codebase for future reference.
Author Profile
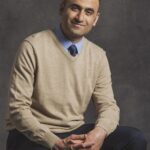
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?