How Can You Effectively Compare Two Strings in JavaScript?
In the world of programming, string manipulation is a fundamental skill that every developer must master. Among the various tasks that involve strings, comparing them is one of the most common yet crucial operations. Whether you’re validating user input, checking for duplicates, or implementing search functionalities, knowing how to compare two strings in JavaScript can significantly enhance your coding efficiency and accuracy. This article will guide you through the essential techniques and best practices for string comparison, ensuring you have the tools you need to tackle any challenge that comes your way.
When it comes to comparing strings in JavaScript, there are multiple approaches you can take, each suited to different scenarios. The simplest method involves using the equality operators, which allow you to check if two strings are identical. However, the nuances of string comparison extend beyond mere equality; factors such as case sensitivity and locale can affect how strings are evaluated. Understanding these elements is crucial for writing robust code that behaves as expected under various conditions.
Additionally, JavaScript provides built-in methods that can facilitate more complex comparisons, such as determining the order of strings or finding substring occurrences. By leveraging these methods, developers can create more dynamic and responsive applications. As we delve deeper into this topic, you will discover the various techniques available for string comparison, along with practical examples that illustrate
String Comparison Methods
In JavaScript, comparing two strings can be accomplished in several ways, each suitable for different use cases. The most common methods include using the equality operators, the locale-specific comparison, and string methods that provide more control over the comparison process.
Using Equality Operators
The simplest way to compare two strings is by using the equality (`==`) or strict equality (`===`) operators. The distinction between these operators is crucial:
- The `==` operator checks for value equality, performing type coercion if necessary.
- The `===` operator checks for both value and type equality, making it the preferred method for most use cases.
Example:
javascript
let string1 = “hello”;
let string2 = “hello”;
let string3 = “Hello”;
console.log(string1 === string2); // true
console.log(string1 === string3); //
Using String Methods
JavaScript provides several built-in string methods that facilitate comparison based on specific criteria.
- `localeCompare()`: This method compares two strings in the current locale and returns a number indicating whether the reference string comes before, after, or is the same as the compared string.
Example:
javascript
let str1 = ‘apple’;
let str2 = ‘banana’;
console.log(str1.localeCompare(str2)); // -1 (str1 comes before str2)
- `startsWith()` and `endsWith()`: These methods check if a string starts or ends with a specified substring, respectively.
Example:
javascript
let str = “Hello, world!”;
console.log(str.startsWith(“Hello”)); // true
console.log(str.endsWith(“world!”)); // true
Case Sensitivity in Comparisons
When comparing strings, it is essential to consider case sensitivity. The equality operators are case-sensitive, which can lead to unexpected results if not handled correctly. To perform case-insensitive comparisons, convert both strings to the same case using `toLowerCase()` or `toUpperCase()`.
Example:
javascript
let strA = “JavaScript”;
let strB = “javascript”;
console.log(strA.toLowerCase() === strB.toLowerCase()); // true
Comparison Table
The following table summarizes the various string comparison methods in JavaScript:
Method | Type | Case Sensitive | Returns |
---|---|---|---|
== | Operator | No | Boolean |
=== | Operator | Yes | Boolean |
localeCompare() | Method | Yes | Number |
startsWith() | Method | Yes | Boolean |
endsWith() | Method | Yes | Boolean |
String Comparison
Understanding the nuances of string comparison in JavaScript is vital for ensuring accurate results in your code. By utilizing the appropriate methods and operators, you can effectively compare strings according to your specific requirements.
String Comparison Methods in JavaScript
JavaScript offers several methods to compare strings, each suited for different scenarios. Below are the most commonly used techniques:
Using the Equality Operator (== and ===)
The simplest way to compare two strings is through the equality operators:
- `==` (Loose Equality): Checks for equality after performing type coercion.
- `===` (Strict Equality): Checks for equality without type coercion, meaning both the value and the type must be the same.
Example:
javascript
let str1 = “Hello”;
let str2 = “Hello”;
let str3 = new String(“Hello”);
console.log(str1 == str2); // true
console.log(str1 === str2); // true
console.log(str1 == str3); // true
console.log(str1 === str3); //
Using the `localeCompare` Method
The `localeCompare` method is beneficial for comparing strings in a locale-sensitive manner. It returns:
- A negative number if the reference string is sorted before the compared string.
- Zero if both strings are equivalent.
- A positive number if the reference string is sorted after the compared string.
Example:
javascript
let str1 = “apple”;
let str2 = “banana”;
console.log(str1.localeCompare(str2)); // -1
console.log(str2.localeCompare(str1)); // 1
console.log(str1.localeCompare(“apple”)); // 0
Using String Length Comparison
In certain cases, comparing the length of the strings can quickly determine their relationship. If one string is longer than another, it is considered greater.
Example:
javascript
let str1 = “Hello”;
let str2 = “Hi”;
if (str1.length > str2.length) {
console.log(`${str1} is longer than ${str2}`);
} else if (str1.length < str2.length) {
console.log(`${str2} is longer than ${str1}`);
} else {
console.log(`${str1} and ${str2} are of equal length`);
}
Case Sensitivity in String Comparison
JavaScript string comparisons are case-sensitive. For example, “hello” is not equal to “Hello”. To perform a case-insensitive comparison, convert both strings to the same case using `toLowerCase()` or `toUpperCase()`.
Example:
javascript
let str1 = “Hello”;
let str2 = “hello”;
if (str1.toLowerCase() === str2.toLowerCase()) {
console.log(“Strings are equal (case-insensitive)”);
} else {
console.log(“Strings are not equal”);
}
Using Regular Expressions for Pattern Matching
Regular expressions can also be employed for string comparisons, particularly when you need to check for patterns rather than direct equality.
Example:
javascript
let str = “Hello, World!”;
let pattern = /hello/i; // ‘i’ for case-insensitive
if (pattern.test(str)) {
console.log(“Pattern matches the string”);
} else {
console.log(“Pattern does not match”);
}
Performance Considerations
When comparing strings, especially within loops or large datasets, consider the following:
- Strict Equality (`===`) is generally faster than loose equality (`==`) because it does not involve type coercion.
- `localeCompare` may have performance implications depending on the locale and complexity of the comparison.
Choosing the appropriate method depends on the specific requirements of the comparison, such as sensitivity to case, locale, and performance constraints.
Expert Insights on Comparing Strings in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When comparing two strings in JavaScript, it is crucial to utilize the strict equality operator (===) to ensure both value and type are compared. This method prevents unexpected results that can arise from type coercion.”
Michael Chen (Lead Developer, CodeCraft Solutions). “For case-insensitive comparisons, transforming both strings to a common case using .toLowerCase() or .toUpperCase() is essential. This approach enhances accuracy when comparing user inputs, especially in search functionalities.”
Sarah Thompson (JavaScript Specialist, WebDev Academy). “Utilizing the localeCompare() method is particularly beneficial when comparing strings that may contain special characters or different alphabets. This method provides a more culturally aware comparison, which is vital for international applications.”
Frequently Asked Questions (FAQs)
How can I compare two strings for equality in JavaScript?
You can compare two strings for equality using the equality operator (`==`) or the strict equality operator (`===`). The strict equality operator checks both value and type, making it the preferred method for string comparison.
What method can I use to check if one string contains another string?
You can use the `includes()` method to check if one string contains another. For example, `string1.includes(string2)` returns `true` if `string2` is found within `string1`.
How do I compare strings in a case-insensitive manner?
To compare strings in a case-insensitive manner, convert both strings to the same case using `toLowerCase()` or `toUpperCase()` before comparison. For example, `string1.toLowerCase() === string2.toLowerCase()`.
What is the difference between `localeCompare()` and standard string comparison?
The `localeCompare()` method compares two strings in a locale-sensitive manner, taking into account language-specific rules. Standard comparison (`string1 < string2`) does not consider locale and is based on Unicode values.
Can I compare strings for order in JavaScript?
Yes, you can compare strings for order using relational operators like `<`, `>`, `<=`, and `>=`. These operators compare strings based on their Unicode values, determining their lexicographical order.
What should I do if I need to compare strings with special characters?
When comparing strings with special characters, ensure you use `localeCompare()` for accurate results based on locale-specific rules. This method handles special characters appropriately, providing a more reliable comparison.
In JavaScript, comparing two strings can be accomplished using several methods, each suitable for different scenarios. The most straightforward approach is to use the equality operators, such as `==` for loose comparison and `===` for strict comparison. The strict equality operator checks both the value and the type of the strings, making it the preferred choice for most cases where precise comparison is required.
Another method involves using the `localeCompare()` function, which provides a way to compare strings in a locale-sensitive manner. This is particularly useful when dealing with strings that may contain characters from different languages or when sorting strings according to specific cultural norms. Additionally, string methods like `includes()`, `startsWith()`, and `endsWith()` can be employed to check for the presence of substrings or specific patterns within strings.
When comparing strings, it is essential to consider case sensitivity. By default, string comparisons in JavaScript are case-sensitive, meaning that ‘apple’ and ‘Apple’ would be considered different. To perform case-insensitive comparisons, one can convert both strings to the same case using methods like `toLowerCase()` or `toUpperCase()` before making the comparison. This ensures that the comparison is based solely on the string content
Author Profile
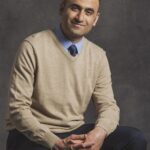
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?