How Can You Compile C Programs on Linux?
Compiling C code on Linux is a fundamental skill for programmers, whether you’re a seasoned developer or just starting your coding journey. The Linux environment offers a powerful and flexible platform for software development, and understanding how to compile C programs is essential for bringing your ideas to life. With a multitude of tools and options available, mastering the compilation process can significantly enhance your programming efficiency and effectiveness. In this article, we will explore the ins and outs of compiling C on Linux, demystifying the process and empowering you to create robust applications.
To compile C code on Linux, you’ll primarily rely on the GNU Compiler Collection (GCC), a versatile and widely-used compiler that supports various programming languages. The compilation process transforms your human-readable C source code into machine code that the computer can execute. This involves several steps, including preprocessing, compiling, assembling, and linking, each of which plays a crucial role in producing a functional executable file. Understanding these stages not only helps you troubleshoot errors but also enables you to optimize your code for better performance.
In addition to GCC, Linux offers a variety of integrated development environments (IDEs) and text editors that can streamline the compilation process, making it easier to write, debug, and run your programs. Whether you prefer a command-line
Installing a C Compiler
Before you can compile C programs on a Linux system, you need to ensure that a C compiler is installed. The most commonly used compiler is GCC (GNU Compiler Collection). To install GCC, you can use your distribution’s package manager. Here are commands for some popular Linux distributions:
- Debian/Ubuntu:
“`bash
sudo apt update
sudo apt install build-essential
“`
- Fedora:
“`bash
sudo dnf install gcc gcc-c++
“`
- Arch Linux:
“`bash
sudo pacman -S gcc
“`
Once installed, verify the installation by checking the version of GCC:
“`bash
gcc –version
“`
Writing a Simple C Program
To compile a C program, you first need to write the code. You can use any text editor to create a simple C program. Here’s an example of a basic C program saved as `hello.c`:
“`c
include
int main() {
printf(“Hello, World!\n”);
return 0;
}
“`
Compiling the C Program
To compile the C program, navigate to the directory where the `hello.c` file is located using the terminal. The compilation is done using the `gcc` command followed by the name of the source file and the output option.
The basic syntax is:
“`bash
gcc -o output_file source_file.c
“`
For example, to compile the `hello.c` file, you would run:
“`bash
gcc -o hello hello.c
“`
This command generates an executable file named `hello`.
Running the Compiled Program
After successfully compiling the program, you can run it by executing the following command in your terminal:
“`bash
./hello
“`
This should output:
“`
Hello, World!
“`
Common Compilation Options
GCC provides various options that can be used during the compilation process. Here are some commonly used options:
Option | Description |
---|---|
-Wall | Enables all compiler’s warning messages. |
-g | Generates debugging information to be used by GDB. |
-O2 | Enables a set of optimizations for better performance. |
-std=c11 | Specifies the C standard to be used (C11 in this case). |
To use these options, you can include them in your compilation command. For example:
“`bash
gcc -Wall -g -o hello hello.c
“`
This command enables warnings and includes debugging information in the output file.
Debugging Your C Program
If your program encounters errors or crashes, you can use a debugger like GDB (GNU Debugger). To debug your program, compile it with the `-g` option to include debugging information:
“`bash
gcc -g -o hello hello.c
“`
Then, start GDB with the executable:
“`bash
gdb ./hello
“`
Within GDB, you can set breakpoints, inspect variables, and step through your code to find issues.
Installing a C Compiler
To compile C programs on Linux, you need to have a C compiler installed. The most common compiler is GCC (GNU Compiler Collection). Follow these steps to install GCC:
- Open your terminal.
- Update your package manager:
“`bash
sudo apt update
“`
- Install GCC:
“`bash
sudo apt install build-essential
“`
- Verify the installation:
“`bash
gcc –version
“`
Writing a C Program
Create a simple C program using a text editor of your choice. Here’s a basic example that prints “Hello, World!” to the console.
“`c
include
int main() {
printf(“Hello, World!\n”);
return 0;
}
“`
Save this file as `hello.c`.
Compiling the C Program
Once you have written your C program, you can compile it using GCC. Use the following command in the terminal:
“`bash
gcc hello.c -o hello
“`
This command does the following:
- `gcc`: Invokes the GCC compiler.
- `hello.c`: Specifies the source file to compile.
- `-o hello`: Indicates the name of the output file (in this case, `hello`).
Running the Compiled Program
To run your compiled program, execute the following command in your terminal:
“`bash
./hello
“`
You should see the output:
“`
Hello, World!
“`
Common Compilation Options
GCC offers various options to control the compilation process. Here are some frequently used flags:
Option | Description |
---|---|
`-Wall` | Enable all compiler’s warning messages. |
`-g` | Generate debug information for debugging. |
`-O2` | Optimize the code for speed. |
`-std=c11` | Specify the C standard to use (e.g., C11). |
Example of using options:
“`bash
gcc -Wall -g -O2 hello.c -o hello
“`
Debugging with GDB
To debug your C program, you can use GDB (GNU Debugger). First, compile your program with the `-g` flag:
“`bash
gcc -g hello.c -o hello
“`
Start GDB with the following command:
“`bash
gdb ./hello
“`
Within GDB, you can use commands like:
- `run`: Start the program.
- `break
`: Set a breakpoint at a specific line. - `next`: Execute the next line of code.
- `print
`: Display the value of a variable.
Cleaning Up Compiled Files
After compiling and testing your program, you may want to remove the compiled output files. You can do this using the `rm` command:
“`bash
rm hello
“`
This command deletes the executable file `hello`. If you also want to remove object files (if any), you can add those to the command as well.
Expert Insights on Compiling C Code in Linux
Dr. Emily Chen (Senior Software Engineer, Open Source Initiative). “Compiling C code on Linux is a straightforward process, primarily utilizing the GCC (GNU Compiler Collection). It is essential to ensure that GCC is installed on your system, which can typically be done through your package manager. Once installed, you can compile your C program by using the command ‘gcc filename.c -o outputfile’, where ‘filename.c’ is your source code and ‘outputfile’ is the name of the executable you wish to create.”
Mark Thompson (Lead Developer, Linux Development Group). “Understanding the compilation process is crucial for effective programming in C on Linux. The use of flags with GCC, such as ‘-Wall’ for warnings and ‘-g’ for debugging information, can significantly enhance your development experience. Additionally, familiarizing yourself with Makefiles can streamline the compilation of larger projects, allowing for easier management of dependencies and build processes.”
Lisa Patel (Technical Writer, Linux Journal). “When compiling C code on Linux, it is important to consider the environment in which you are working. Using an Integrated Development Environment (IDE) like Code::Blocks or Eclipse can simplify the process for beginners. However, mastering the command line compilation not only builds confidence but also provides a deeper understanding of how the compilation process works, which is invaluable for troubleshooting and optimization.”
Frequently Asked Questions (FAQs)
How do I install a C compiler on Linux?
To install a C compiler on Linux, you can use the package manager specific to your distribution. For example, on Ubuntu or Debian, run `sudo apt install build-essential`. For Fedora, use `sudo dnf groupinstall “Development Tools”`.
What command is used to compile a C program?
The command to compile a C program is `gcc filename.c -o outputfile`. Replace `filename.c` with your source file name and `outputfile` with the desired name for the compiled executable.
How can I compile a C program with debugging information?
To include debugging information in your compiled C program, use the `-g` flag with the gcc command: `gcc -g filename.c -o outputfile`. This allows you to use debugging tools like gdb.
What does the `-o` option do in the gcc command?
The `-o` option specifies the name of the output file. If omitted, gcc will create an executable named `a.out` by default.
How do I compile multiple C files together?
To compile multiple C files, list them all in the gcc command: `gcc file1.c file2.c -o outputfile`. This will link the files together into a single executable.
What are common errors when compiling C code on Linux?
Common errors include syntax errors, missing header files, and linking errors. The compiler will provide messages indicating the nature of the errors, which should guide you in troubleshooting.
Compiling C programs on Linux is a straightforward process that primarily involves the use of the GNU Compiler Collection (GCC). To begin, users must ensure that GCC is installed on their system, which can typically be done through the package manager associated with their Linux distribution. Once GCC is installed, compiling a C program can be achieved with a simple command in the terminal, such as `gcc filename.c -o outputname`, where `filename.c` is the source file and `outputname` is the desired name of the compiled executable.
Understanding the various options available with GCC can enhance the compilation process. For instance, using the `-Wall` flag enables all compiler’s warning messages, which is crucial for identifying potential issues in the code. Additionally, the `-g` flag can be employed to include debugging information, making it easier to troubleshoot any errors during execution. Users can also explore optimization flags like `-O2` to improve the performance of the compiled program.
Furthermore, it is essential to be aware of the linking process, especially when dealing with multiple source files or external libraries. The linking stage combines different object files into a single executable, and users can specify additional libraries using the `-l` flag. For
Author Profile
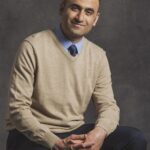
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?