How Can You Concatenate Strings in JavaScript? A Comprehensive Guide
### Introduction
In the world of programming, the ability to manipulate and combine data is crucial, and string concatenation is one of the most fundamental operations you will encounter. Whether you’re building a dynamic web application, crafting user-friendly interfaces, or simply handling text data, knowing how to effectively concatenate strings in JavaScript can significantly enhance your coding toolkit. This essential skill not only streamlines your code but also opens up a realm of possibilities for creating engaging and interactive user experiences.
String concatenation in JavaScript allows developers to merge multiple strings into a single cohesive unit, making it easier to display messages, format data, and construct dynamic content. From the classic method of using the `+` operator to the more modern approach with template literals, JavaScript provides various techniques to achieve this task. Understanding these methods will not only improve your coding efficiency but also help you write cleaner, more readable code.
As we delve deeper into the nuances of string concatenation, we will explore the different techniques available in JavaScript, highlighting their unique advantages and potential use cases. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, this guide will equip you with the knowledge you need to master string concatenation in JavaScript. Get ready to enhance your programming prowess
Using the + Operator
The simplest and most common method for concatenating strings in JavaScript is by using the `+` operator. This operator allows you to join two or more strings together seamlessly.
Example:
javascript
let firstName = “John”;
let lastName = “Doe”;
let fullName = firstName + ” ” + lastName; // “John Doe”
This method is straightforward and works effectively for most use cases. However, it can become cumbersome when concatenating multiple strings or including variables.
Utilizing Template Literals
Introduced in ES6 (ECMAScript 2015), template literals provide a more powerful and flexible way to concatenate strings. They are enclosed by backticks (“ ` “) and allow for embedded expressions, making them particularly useful for creating strings that include variables or expressions.
Example:
javascript
let firstName = “John”;
let lastName = “Doe”;
let age = 30;
let greeting = `Hello, my name is ${firstName} ${lastName} and I am ${age} years old.`;
// “Hello, my name is John Doe and I am 30 years old.”
Template literals also support multi-line strings and can enhance readability, especially when dealing with longer text.
Array Join Method
Another effective way to concatenate strings in JavaScript is by using the `join()` method on arrays. This method is particularly useful when you have an array of strings that you want to combine into a single string.
Example:
javascript
let words = [“Hello”, “world”, “!”];
let sentence = words.join(” “); // “Hello world !”
This method allows you to specify a separator, making it flexible for different output formats.
Comparison of String Concatenation Methods
To better understand the different methods of string concatenation in JavaScript, here is a comparison table:
Method | Syntax | Use Case | Multi-line Support |
---|---|---|---|
+ Operator | `string1 + string2` | Simple concatenation | No |
Template Literals | “ `string ${variable}` “ | Complex strings with expressions | Yes |
Array Join Method | `array.join(separator)` | Concatenating multiple strings from an array | No |
Each method has its advantages and can be chosen based on the specific requirements of the task at hand. Understanding these options allows developers to write cleaner and more efficient code when handling string data in JavaScript.
Methods to Concatenate Strings in JavaScript
In JavaScript, there are several methods available for concatenating strings. The most common approaches are using the `+` operator, the `concat()` method, and template literals. Each method has its own use cases and benefits.
Using the `+` Operator
The simplest way to concatenate strings is by using the `+` operator. This method allows you to join two or more strings easily.
javascript
let str1 = “Hello, “;
let str2 = “World!”;
let result = str1 + str2;
console.log(result); // Outputs: Hello, World!
You can also concatenate multiple strings in a single expression:
javascript
let greeting = str1 + str2 + ” How are you?”;
console.log(greeting); // Outputs: Hello, World! How are you?
The `concat()` Method
The `concat()` method is a built-in function of the String object, which can be used to join two or more strings. It is less commonly used than the `+` operator but can be beneficial in certain scenarios.
javascript
let str1 = “Hello, “;
let str2 = “World!”;
let result = str1.concat(str2);
console.log(result); // Outputs: Hello, World!
This method can also take multiple string arguments:
javascript
let result = str1.concat(str2, ” How are you?”);
console.log(result); // Outputs: Hello, World! How are you?
Template Literals
Template literals, introduced in ES6 (ECMAScript 2015), offer a modern way to concatenate strings. They allow for embedding expressions and are enclosed by backticks (“ ` “).
javascript
let name = “World”;
let greeting = `Hello, ${name}!`;
console.log(greeting); // Outputs: Hello, World!
Template literals are particularly useful when dealing with multi-line strings or when you need to include variables directly within the string.
Performance Considerations
While all methods of string concatenation are valid, performance can vary depending on the context:
- `+` Operator: Generally the fastest and most readable for simple concatenations.
- `concat()` Method: May be slightly slower than the `+` operator, especially when concatenating multiple strings.
- Template Literals: Offer clarity and ease of use, particularly in complex strings, but may introduce slight overhead in performance.
Examples of Concatenation in Different Scenarios
Here are some examples demonstrating different scenarios of string concatenation:
Scenario | Example Code | Output |
---|---|---|
Simple Concatenation | `let result = “Hello” + ” ” + “World”;` | Hello World |
Using `concat()` | `let result = “Hello”.concat(” “, “World”);` | Hello World |
With Variables | `let name = “Alice”; let greeting = “Hi, ” + name;` | Hi, Alice |
Multi-line String | “let message = `Hello, ${name}.\nWelcome!`;“ | Hello, Alice. Welcome! |
By understanding these methods, developers can choose the most appropriate one based on their specific needs and coding style.
Expert Insights on Concatenating Strings in JavaScript
Julia Thompson (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, concatenating strings can be achieved using the `+` operator or the `concat()` method. However, for modern applications, template literals using backticks are often preferred for their readability and flexibility in embedding expressions.”
Michael Chen (JavaScript Framework Specialist, CodeCraft Academy). “While traditional concatenation methods work, I recommend utilizing the `join()` method on arrays for concatenating multiple strings. This approach enhances performance and simplifies code, especially when dealing with dynamic string collections.”
Linda Martinez (Full-Stack Developer, Web Solutions Group). “It’s crucial to consider the implications of concatenating strings in JavaScript, particularly regarding type coercion. Always ensure that the variables being concatenated are indeed strings to avoid unexpected results.”
Frequently Asked Questions (FAQs)
How can I concatenate strings in JavaScript?
You can concatenate strings in JavaScript using the `+` operator or the `concat()` method. For example, `let result = “Hello” + ” ” + “World”;` or `let result = “Hello”.concat(” “, “World”);`.
What is the difference between using `+` and `concat()` for string concatenation?
The `+` operator is more commonly used for concatenation due to its simplicity and readability. The `concat()` method, while functional, is less frequently used and can be less intuitive for those unfamiliar with it.
Can I concatenate more than two strings at once in JavaScript?
Yes, you can concatenate multiple strings at once using either the `+` operator or the `concat()` method. For example, `let result = “Hello” + ” ” + “World” + ” ” + “2023”;` or `let result = “Hello”.concat(” “, “World”, ” “, “2023”);`.
Is there a modern way to concatenate strings in JavaScript?
Yes, template literals provide a modern approach to string concatenation. You can use backticks (“ ` “) and `${}` syntax to embed expressions. For example, “let result = `Hello ${name}`;“.
What happens if I concatenate a string with a number in JavaScript?
When concatenating a string with a number using the `+` operator, JavaScript converts the number to a string and performs concatenation. For example, `let result = “The number is ” + 5;` results in `”The number is 5″`.
Are there performance differences between concatenation methods in JavaScript?
In general, using the `+` operator is more efficient for concatenating a small number of strings. However, for concatenating many strings, using an array and the `join()` method can be more performant, as it minimizes intermediate string creation.
In JavaScript, concatenating strings can be accomplished through various methods, each with its own advantages and use cases. The most common approaches include using the `+` operator, the `concat()` method, and template literals. The `+` operator is straightforward and widely used for its simplicity, allowing developers to join two or more strings seamlessly. The `concat()` method, while less common, provides a functional approach to string concatenation and can be useful in specific scenarios where method chaining is desired.
Template literals, introduced in ES6, offer a modern and flexible way to concatenate strings. They allow for embedding expressions and multi-line strings, making them particularly useful for more complex string constructions. This method enhances readability and maintainability, especially when dealing with dynamic content or variables. Understanding these different methods equips developers with the tools needed to choose the most appropriate approach based on their specific requirements.
In summary, string concatenation in JavaScript can be efficiently achieved through multiple techniques. Each method has its unique features and applications, making it essential for developers to be familiar with them. By leveraging the strengths of these various approaches, developers can write cleaner, more efficient code that meets the needs of their applications.
Author Profile
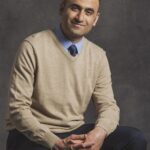
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?