How Can You Concatenate Strings and Integers in Python?
In the world of programming, the ability to manipulate data types is crucial for creating efficient and effective code. One common task that developers encounter is the need to combine strings and integers—a fundamental operation that can seem straightforward but often leads to confusion for those new to Python. Whether you’re crafting user-friendly messages, generating dynamic content, or simply logging information, understanding how to concatenate strings and integers is an essential skill that can enhance your coding prowess.
Concatenation in Python allows you to merge different data types into a single, cohesive output. However, since strings and integers are inherently different types, directly combining them can result in errors. This is where Python’s flexibility shines, offering various methods to seamlessly join these types while maintaining the integrity of your data. From simple type conversion techniques to leveraging built-in functions, Python provides a toolkit for developers to navigate this common challenge.
As you delve deeper into the nuances of concatenating strings and integers, you’ll discover the best practices and techniques that can streamline your coding process. Whether you’re a beginner eager to learn the ropes or an experienced programmer looking to refine your skills, mastering this concept will empower you to write cleaner, more effective Python code. Get ready to unlock the secrets of string and integer concatenation, and elevate your programming capabilities to new heights!
Methods for Concatenating Strings and Integers in Python
In Python, concatenating a string with an integer requires converting the integer to a string first. This is necessary because Python does not allow direct concatenation of different data types. Below are several methods to achieve this:
Using the str() Function
The most straightforward way to concatenate a string and an integer is by using the built-in `str()` function. This function converts the integer to a string, allowing for seamless concatenation.
Example:
“`python
age = 25
greeting = “I am ” + str(age) + ” years old.”
print(greeting) Output: I am 25 years old.
“`
Using f-Strings
Introduced in Python 3.6, f-strings provide a concise and readable way to format strings. By prefixing the string with an ‘f’, you can directly include variables inside curly braces.
Example:
“`python
age = 25
greeting = f”I am {age} years old.”
print(greeting) Output: I am 25 years old.
“`
Using the format() Method
The `format()` method is another effective way to concatenate strings and integers. It allows for more complex string formatting.
Example:
“`python
age = 25
greeting = “I am {} years old.”.format(age)
print(greeting) Output: I am 25 years old.
“`
Using the % Operator
Although less common in modern Python, the `%` operator provides a way to concatenate strings and integers through old-style string formatting.
Example:
“`python
age = 25
greeting = “I am %d years old.” % age
print(greeting) Output: I am 25 years old.
“`
Comparison of Methods
The following table summarizes the different methods for concatenating strings and integers in Python, highlighting their syntax and ease of use:
Method | Syntax | Notes |
---|---|---|
str() | `”string” + str(int)` | Simple and clear |
f-Strings | `f”I am {int}”` | Most modern and preferred method |
format() | `”string {}”.format(int)` | Flexible formatting options |
% Operator | `”string %d” % int` | Older style, less recommended |
Each method has its advantages, and the choice depends on the specific use case and personal preference. For most modern applications, f-strings are recommended due to their readability and efficiency.
Concatenating Strings and Integers in Python
In Python, concatenating strings and integers requires explicit type conversion, as Python does not allow direct concatenation of different data types. There are several methods to achieve this, each with its own advantages.
Using the `str()` Function
The simplest way to concatenate a string and an integer is to convert the integer to a string using the `str()` function. This method is straightforward and highly readable.
“`python
number = 10
text = “The number is ”
result = text + str(number)
print(result) Output: The number is 10
“`
Using f-Strings (Formatted String Literals)
Introduced in Python 3.6, f-strings provide a concise and efficient way to format strings. This method allows embedding expressions inside string literals.
“`python
number = 10
result = f”The number is {number}”
print(result) Output: The number is 10
“`
Using the `format()` Method
The `format()` method is another flexible way to concatenate strings and integers. This method can handle multiple variables and formatting options.
“`python
number = 10
result = “The number is {}”.format(number)
print(result) Output: The number is 10
“`
Using the Percent Formatting
This is an older method of string formatting in Python that uses the `%` operator. While it is less common in modern Python code, it is still valid.
“`python
number = 10
result = “The number is %d” % number
print(result) Output: The number is 10
“`
Comparison of Methods
Method | Readability | Conciseness | Flexibility |
---|---|---|---|
`str()` | High | Medium | Low |
f-Strings | Very High | High | High |
`format()` | High | Medium | High |
Percent Formatting | Medium | Low | Medium |
Choosing the Right Method
- Use `str()` when simplicity is key, and you are working with a small number of variables.
- Use f-strings for clean, readable code, especially when dealing with multiple variables or expressions.
- Use `format()` when you need more control over formatting options, such as decimal places or padding.
- Use percent formatting primarily for legacy code or when working with very simple concatenations.
By employing the appropriate method, you can efficiently concatenate strings and integers in your Python applications.
Expert Insights on Concatenating Strings and Integers in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Corp.). “In Python, concatenating strings and integers requires explicit conversion of the integer to a string using the `str()` function. This ensures that the types are compatible, allowing for seamless integration of different data types into a single output.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “A common approach to concatenate a string and an integer in Python is to use formatted strings, such as f-strings or the `format()` method. This not only enhances readability but also maintains the integrity of the data types involved.”
Sarah Thompson (Python Educator, LearnPython.org). “When teaching beginners about string and integer concatenation in Python, I emphasize the importance of understanding type conversion. The `+` operator will raise a TypeError if you attempt to concatenate a string and an integer directly, making it crucial to convert the integer first.”
Frequently Asked Questions (FAQs)
How can I concatenate a string and an integer in Python?
To concatenate a string and an integer in Python, you must first convert the integer to a string using the `str()` function. For example: `result = “The number is ” + str(5)`.
What happens if I try to concatenate a string and an integer directly?
If you attempt to concatenate a string and an integer directly, Python will raise a `TypeError` indicating that you cannot concatenate a string and an integer.
Is there a way to concatenate strings and integers using formatted strings?
Yes, you can use formatted strings (f-strings) to concatenate strings and integers. For example: `number = 5; result = f”The number is {number}”` achieves this effectively.
Can I use the `format()` method to concatenate a string and an integer?
Yes, the `format()` method allows for concatenation. For example: `result = “The number is {}”.format(5)` will produce the desired output.
Are there any performance differences between using f-strings and the `str()` method for concatenation?
F-strings are generally faster and more readable than using the `str()` method for concatenation, especially when dealing with multiple variables.
What is the best practice for concatenating multiple strings and integers in Python?
The best practice is to use f-strings for their clarity and efficiency, especially when concatenating multiple variables. For example: `result = f”Values: {a}, {b}, and {c}”` where `a`, `b`, and `c` can be strings or integers.
In Python, concatenating strings and integers requires converting the integer to a string format before combining them. This is essential because Python does not allow direct concatenation of different data types, such as strings and integers. The most common methods for achieving this conversion include using the built-in `str()` function or employing formatted string literals (f-strings), which were introduced in Python 3.6. Both methods ensure that the integer is appropriately transformed into a string for seamless concatenation.
Using the `str()` function is straightforward; simply pass the integer as an argument to convert it. For example, `result = “The number is ” + str(number)` effectively combines a string with an integer. Alternatively, f-strings provide a more concise and readable approach, allowing you to embed expressions directly within string literals. For instance, `result = f”The number is {number}”` achieves the same result with improved clarity and efficiency.
In summary, understanding how to concatenate strings and integers in Python is fundamental for effective programming. By utilizing the `str()` function or f-strings, developers can easily manage type conversions and create dynamic strings. This knowledge not only enhances code readability but also prevents type errors, ensuring that string manipulation tasks are
Author Profile
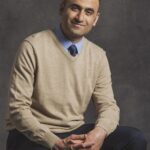
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?