How Can You Configure Environment Variables in Docker Deployed on EC2?
In the ever-evolving landscape of cloud computing, Docker has emerged as a powerful tool for developers looking to streamline their application deployment processes. When paired with Amazon EC2, Docker not only enhances scalability but also simplifies the management of complex applications. However, configuring environment variables in a Docker container deployed on EC2 can be a daunting task for many. Understanding how to effectively manage these configurations is crucial for ensuring your applications run smoothly and securely in a cloud environment.
Configuring environment variables in Docker involves a series of strategic steps that allow you to customize your application’s behavior without altering the codebase. This flexibility is particularly beneficial when deploying applications across different environments, such as development, testing, and production. In the context of EC2, leveraging Docker’s capabilities can lead to more efficient resource utilization and easier updates, but it requires a solid grasp of both Docker commands and AWS infrastructure.
As we delve deeper into the intricacies of configuring your environment in a Docker container on EC2, we will explore best practices, common pitfalls, and practical tips to streamline your deployment process. Whether you are a seasoned developer or just starting your journey into cloud deployment, mastering this configuration will empower you to harness the full potential of Docker and EC2, paving the way for robust and resilient applications.
Setting Up Environment Variables in Docker
To configure environment variables in Docker, particularly when deploying on AWS EC2, there are several methods available. Utilizing environment variables allows you to manage configurations without hardcoding sensitive information into your Docker images. Here are the primary methods to set these variables:
- Using Dockerfile: You can define environment variables directly in your Dockerfile using the `ENV` instruction. This method is straightforward but can lead to less flexibility since these variables are baked into the image.
“`dockerfile
FROM ubuntu:latest
ENV APP_ENV=production
ENV DB_HOST=database.example.com
“`
- Using Docker Compose: If you are using Docker Compose to manage multi-container applications, you can specify environment variables in the `docker-compose.yml` file. This allows you to configure variables per service.
“`yaml
version: ‘3’
services:
web:
image: myapp:latest
environment:
- APP_ENV=production
- DB_HOST=database.example.com
“`
- Using `.env` Files: You can also use a `.env` file to manage your environment variables externally. Docker Compose automatically picks up these variables.
Example `.env` file:
“`
APP_ENV=production
DB_HOST=database.example.com
“`
Docker Compose reference:
“`yaml
version: ‘3’
services:
web:
image: myapp:latest
env_file:
- .env
“`
Passing Environment Variables at Runtime
Another method to configure environment variables is to pass them directly at runtime. This approach is useful for overriding default values without modifying your Dockerfile or Compose files.
- Using the `-e` flag with `docker run`:
“`bash
docker run -e APP_ENV=production -e DB_HOST=database.example.com myapp:latest
“`
- You can also use the `–env-file` option to specify a file containing environment variables:
“`bash
docker run –env-file .env myapp:latest
“`
Managing Secrets and Sensitive Information
When deploying applications, it is crucial to handle sensitive information securely. Here are some best practices:
- AWS Secrets Manager: Store sensitive environment variables in AWS Secrets Manager and retrieve them at runtime. This ensures your credentials are not hard-coded or stored in plaintext.
- Docker Secrets: For Docker Swarm deployments, use Docker secrets to manage sensitive data. Secrets are encrypted and only accessible by the services that need them.
- AWS Systems Manager Parameter Store: Store configuration data and secrets in AWS Systems Manager Parameter Store and access them securely.
Method | Description | Use Case |
---|---|---|
ENV in Dockerfile | Static variables baked into the image | Simple applications without sensitive info |
Docker Compose | Manage environment variables for multi-container apps | Complex applications requiring multiple services |
Runtime Variables | Pass variables during container run | Dynamic configurations or testing |
AWS Secrets Manager | Secure storage for sensitive information | Production applications needing secure secrets |
By employing these methods, you can effectively manage environment variables in Docker containers deployed on EC2, ensuring a secure and maintainable application environment.
Configuring Environment Variables in Docker on EC2
When deploying a Docker container on an EC2 instance, configuring environment variables is essential for managing application settings, secrets, and other configurations without hardcoding them. There are several methods to set these environment variables effectively.
Using Docker CLI
You can set environment variables directly when running a Docker container using the `-e` flag. This is a straightforward method for quick deployments.
“`bash
docker run -e VAR_NAME=value your_image
“`
For multiple variables, you can use multiple `-e` flags:
“`bash
docker run -e VAR1=value1 -e VAR2=value2 your_image
“`
Alternatively, you can use an environment file to define variables:
“`bash
docker run –env-file ./env.list your_image
“`
Where `env.list` contains:
“`
VAR1=value1
VAR2=value2
“`
Using Docker Compose
For more complex applications, Docker Compose provides a way to define and manage multi-container Docker applications. Environment variables can be specified in the `docker-compose.yml` file.
“`yaml
version: ‘3’
services:
app:
image: your_image
environment:
- VAR1=value1
- VAR2=value2
“`
You can also reference an external environment file:
“`yaml
version: ‘3’
services:
app:
image: your_image
env_file:
- ./env.list
“`
Using AWS Systems Manager Parameter Store
For enhanced security, consider using AWS Systems Manager Parameter Store to manage sensitive data such as API keys and database passwords. Here’s how to integrate it with Docker:
- Store Parameters: Use the AWS console or CLI to store parameters.
“`bash
aws ssm put-parameter –name “/app/db_password” –value “your_password” –type “SecureString”
“`
- Retrieve Parameters: Modify your Docker entrypoint or application code to retrieve these parameters at runtime. For example, using AWS CLI within your container:
“`bash
aws ssm get-parameters –names “/app/db_password” –with-decryption –query “Parameters[0].Value” –output text
“`
- IAM Role: Ensure your EC2 instance has an IAM role with permissions to access the Parameter Store.
Best Practices for Managing Environment Variables
- Use `.env` files: Keep environment variables in `.env` files for local development, avoiding sensitive data in version control.
- Keep secrets secure: Use tools like AWS Secrets Manager for sensitive data.
- Document variables: Clearly document each environment variable and its purpose in your application to facilitate onboarding and maintenance.
- Version control: Keep your environment variable configurations in version control systems but avoid committing sensitive information.
Monitoring Environment Variables
To ensure that your environment variables are set correctly and monitored during runtime, consider using logging or monitoring tools:
Tool | Description |
---|---|
Prometheus | For monitoring and alerting on application metrics. |
ELK Stack | For logging and visualizing application logs. |
Datadog | For comprehensive monitoring and alerting solutions. |
Implementing these practices will streamline the configuration of environment variables in your Docker containers deployed on EC2, enhancing both security and maintainability.
Expert Insights on Configuring Environment Variables in Docker on EC2
Dr. Emily Chen (Cloud Solutions Architect, Tech Innovations Inc.). “To effectively configure environment variables in a Docker container deployed on EC2, I recommend using the `-e` flag with the `docker run` command. This allows you to set environment variables directly at runtime, ensuring that your application can access the necessary configurations without hardcoding sensitive information into your images.”
Mark Thompson (DevOps Engineer, CloudOps Solutions). “Utilizing AWS Secrets Manager in conjunction with Docker on EC2 is a best practice for managing sensitive environment variables. By storing your secrets securely and injecting them into your containers at runtime, you enhance the security of your application while maintaining flexibility in your deployment process.”
Sarah Patel (Containerization Specialist, Modern DevOps). “For a more streamlined approach, consider using a `.env` file along with Docker Compose. This method allows you to define all your environment variables in one place, making it easier to manage configurations across multiple containers deployed on EC2 instances. Just ensure to keep your `.env` file out of version control to protect sensitive data.”
Frequently Asked Questions (FAQs)
How do I set environment variables in a Docker container?
You can set environment variables in a Docker container using the `-e` flag with the `docker run` command. For example, `docker run -e MY_ENV_VAR=value my_image` sets the environment variable `MY_ENV_VAR` to `value` within the container.
Can I use a .env file to configure environment variables in Docker?
Yes, you can use a `.env` file to define environment variables. When using Docker Compose, the variables in the `.env` file are automatically loaded. For standalone Docker, you can specify the file with the `–env-file` option.
How do I pass environment variables to Docker containers deployed on EC2?
You can pass environment variables to Docker containers on EC2 by using the `-e` flag in your `docker run` command or by defining them in your Docker Compose file. Ensure that your EC2 instance has the necessary IAM roles and permissions to access any required resources.
Is it possible to set environment variables in a Dockerfile?
Yes, you can set environment variables in a Dockerfile using the `ENV` instruction. For example, `ENV MY_ENV_VAR=value` sets the environment variable `MY_ENV_VAR` to `value` for all subsequent instructions in the Dockerfile.
How can I verify the environment variables in a running Docker container?
You can verify the environment variables in a running Docker container by executing the command `docker exec
What are the security considerations for using environment variables in Docker?
Environment variables can expose sensitive information. It is advisable to avoid hardcoding secrets in Dockerfiles or Docker Compose files. Instead, consider using Docker secrets or a secure vault service to manage sensitive data securely.
Configuring environment variables in a Docker container deployed on Amazon EC2 is a crucial step for ensuring that your applications run smoothly and securely. This process typically involves defining the necessary environment variables in your Dockerfile or using a Docker Compose file. By doing so, you can manage configurations such as database connections, API keys, and other sensitive information without hardcoding them into your application code.
One effective approach is to utilize the `.env` file in conjunction with Docker Compose. This allows you to store environment variables in a separate file, which can be easily referenced in your `docker-compose.yml`. Additionally, using the `environment` section within the Compose file enables you to specify environment variables directly, providing flexibility in how you manage configurations across different environments, such as development, testing, and production.
Another important consideration is the security of your environment variables. It is advisable to avoid committing sensitive information to version control. Instead, consider using AWS Secrets Manager or Parameter Store to manage sensitive data securely. This practice not only enhances security but also simplifies the management of environment variables across multiple deployments.
In summary, configuring environment variables in Docker on EC2 involves careful planning and implementation. By leveraging Docker Compose and external secret management tools, you
Author Profile
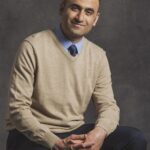
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?