How Can You Convert an Integer to a String in JavaScript?
In the world of programming, data types are the building blocks of our code, shaping how we manipulate and interact with information. Among these types, integers and strings serve distinct purposes, each with its own strengths. However, there are moments when we find ourselves needing to bridge the gap between these two types, particularly in JavaScript, a language renowned for its versatility and ease of use. Whether you’re displaying numbers on a webpage, formatting data for output, or simply working with user inputs, knowing how to convert an integer to a string is an essential skill for any developer.
Converting an integer to a string in JavaScript is a straightforward process, yet it can be approached in multiple ways, each with its own nuances. Understanding these methods not only enhances your coding toolkit but also improves the readability and maintainability of your code. From simple concatenation to built-in functions, JavaScript provides a variety of techniques that can be employed depending on the context of your application.
As you delve deeper into this topic, you’ll discover the advantages and potential pitfalls of each conversion method. Whether you’re a novice programmer or an experienced developer looking to refine your skills, mastering the art of type conversion in JavaScript will empower you to write more effective and efficient code. Get ready to unlock the secrets of
Using the String Constructor
The String constructor in JavaScript can be used to convert integers to strings. This method is straightforward and effective for type conversion. By passing an integer to the String constructor, you will receive a string representation of that integer.
javascript
let num = 123;
let str = String(num);
console.log(str); // “123”
Using the toString Method
Another method for converting an integer to a string is by utilizing the `toString()` method, which is available on number objects. This approach is particularly useful when you want to maintain the numeric object’s context while converting it to a string.
javascript
let num = 456;
let str = num.toString();
console.log(str); // “456”
Using Template Literals
Template literals, introduced in ES6, provide a modern way to handle string interpolation and can also be used for converting integers to strings. By embedding an integer within a template literal, JavaScript automatically converts the integer to a string.
javascript
let num = 789;
let str = `${num}`;
console.log(str); // “789”
Using String Concatenation
String concatenation is another simple method to convert an integer to a string. By concatenating an integer with an empty string, the integer is coerced into a string.
javascript
let num = 101112;
let str = num + ”;
console.log(str); // “101112”
Comparison of Methods
The following table summarizes the different methods for converting integers to strings, highlighting their syntax and use cases.
Method | Syntax | Use Case |
---|---|---|
String Constructor | String(num) | General conversion |
toString Method | num.toString() | When working with number objects |
Template Literals | `${num}` | Modern syntax with interpolation |
String Concatenation | num + ” | Quick and easy conversion |
These methods provide flexibility depending on the context and requirements of the code, allowing developers to choose the most suitable approach for their needs.
Conversion Methods
In JavaScript, converting an integer to a string can be accomplished using several methods, each offering distinct advantages depending on the context. Below are the most common approaches:
Using the `String()` Function
The `String()` function is a straightforward and versatile way to convert an integer to a string. It can handle various data types and will convert them to their string representation.
javascript
let num = 123;
let str = String(num);
console.log(str); // “123”
Using the `toString()` Method
Every number in JavaScript has access to the `toString()` method, which converts the number to a string. This method can also take a radix argument for converting numbers in different bases.
javascript
let num = 456;
let str = num.toString();
console.log(str); // “456”
// Example with radix
let binaryStr = (10).toString(2); // “1010”
Using Template Literals
Template literals provide a convenient way to embed expressions within string literals. By using backticks, you can easily convert an integer to a string.
javascript
let num = 789;
let str = `${num}`;
console.log(str); // “789”
Using String Concatenation
Concatenating an integer with an empty string is a quick method that effectively converts the integer to a string.
javascript
let num = 321;
let str = num + ”;
console.log(str); // “321”
Comparison of Methods
The following table summarizes the different methods for converting an integer to a string, including their syntax and characteristics.
Method | Syntax | Description |
---|---|---|
String() | `String(num)` | Converts any data type to a string. |
toString() | `num.toString()` | Converts the number to a string; accepts radix for bases. |
Template Literals | “ `${num}` “ | Uses backticks to embed the integer in a string context. |
String Concatenation | `num + ”` | Concatenates the integer with an empty string. |
Performance Considerations
While all methods effectively convert integers to strings, performance may vary based on the context in which they are used. The `String()` function and `toString()` method are generally efficient, but if performance is critical, benchmarking may be necessary for specific scenarios.
- String(): Slightly slower due to flexibility with various data types.
- toString(): More efficient for integers specifically.
- Template Literals: Offers readability but may be marginally less performant.
- Concatenation: Fast and straightforward for quick conversions.
Each method has its place in JavaScript development, so selecting the appropriate one depends on the specific requirements of your application.
Expert Insights on Converting Integers to Strings in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, converting an integer to a string can be accomplished using several methods, such as the `String()` function or the `toString()` method. Each approach has its advantages, but using `String()` is often more straightforward, especially for beginners.”
Michael Chen (JavaScript Developer Advocate, CodeCraft). “While both `String(value)` and `value.toString()` are effective, I recommend using template literals for conversion in modern JavaScript. This method not only converts the integer but also allows for easy concatenation with other strings.”
Laura Patel (Web Development Instructor, Code Academy). “It’s crucial to understand the context in which you are converting integers to strings. For instance, when dealing with user input or displaying data, ensuring the conversion is done correctly can prevent unexpected behavior in your applications.”
Frequently Asked Questions (FAQs)
How can I convert an integer to a string in JavaScript?
You can convert an integer to a string in JavaScript using the `String()` function or the `toString()` method. For example, `String(123)` or `(123).toString()` will both yield “123”.
Are there multiple ways to convert an integer to a string in JavaScript?
Yes, there are several methods to convert an integer to a string, including using the `String()` function, the `toString()` method, template literals (e.g., `${123}`), and string concatenation (e.g., `123 + ”`).
Is there a performance difference between the methods of conversion?
Generally, the performance difference among these methods is negligible for typical use cases. However, `String()` and `toString()` are commonly preferred for their clarity and directness.
What happens if I try to convert a non-integer value to a string?
When converting a non-integer value, JavaScript will still return a string representation of that value. For instance, `String(true)` will yield “true”, and `String(null)` will yield “null”.
Can I convert an array of integers to strings in JavaScript?
Yes, you can convert an array of integers to strings using the `map()` method. For example, `[1, 2, 3].map(String)` will return `[“1”, “2”, “3”]`.
Is it necessary to convert integers to strings in JavaScript?
It is not always necessary, but converting integers to strings can be useful when concatenating with other strings or when formatting output for display purposes.
Converting an integer to a string in JavaScript is a straightforward process that can be accomplished using several methods. The most common approaches include using the `String()` function, the `toString()` method, and template literals. Each of these methods effectively transforms an integer into a string format, allowing for greater flexibility in data manipulation and presentation.
The `String()` function is a versatile option that can convert any data type to a string, making it a reliable choice for integer conversion. Alternatively, the `toString()` method is specifically designed for numbers, providing a clear and direct way to achieve the conversion. Template literals, introduced in ES6, offer a modern syntax that can also be utilized for this purpose, particularly when concatenating strings and variables.
In summary, understanding the various methods available for converting integers to strings in JavaScript enhances a developer’s ability to handle data types effectively. Each method has its unique advantages, and the choice of which to use may depend on the specific context of the task at hand. By mastering these techniques, developers can ensure their code is both efficient and easy to read.
Author Profile
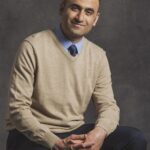
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?