How Can You Easily Copy Files in Python?
In the digital age, the ability to manipulate files efficiently is a crucial skill for programmers and data enthusiasts alike. Whether you’re automating mundane tasks, organizing data, or developing applications, knowing how to copy files in Python can save you time and enhance your productivity. Python, with its simple syntax and powerful libraries, makes file handling intuitive and straightforward, allowing you to focus on what truly matters: your project.
Copying files in Python is a fundamental operation that can be accomplished using various methods and libraries, each catering to different needs and scenarios. From the built-in `shutil` module, which provides a high-level interface for file operations, to more specialized libraries that handle specific file types or conditions, Python offers a plethora of options. Understanding these methods not only equips you with the tools to duplicate files seamlessly but also deepens your grasp of file management in programming.
As you delve deeper into the world of file copying in Python, you’ll discover best practices, error handling techniques, and performance considerations that can elevate your coding skills. Whether you’re a beginner looking to learn the ropes or an experienced developer seeking to refine your approach, mastering file copying in Python is an essential step on your journey to becoming a proficient programmer. Get ready to unlock the potential of Python’s file handling capabilities and streamline
Using the `shutil` Module
The `shutil` module in Python is a powerful utility for file operations, including copying files. It provides a straightforward interface for both shallow and deep copies of files.
To copy a file, you can use the `shutil.copy()` function. This method copies the content and the permissions of the file to the destination path. Here’s a basic example:
“`python
import shutil
shutil.copy(‘source.txt’, ‘destination.txt’)
“`
If you want to copy a file along with its metadata (like the creation and modification times), you can use `shutil.copy2()` instead:
“`python
shutil.copy2(‘source.txt’, ‘destination.txt’)
“`
Copying Directories
When it comes to copying directories, `shutil` provides additional functions such as `shutil.copytree()`. This function recursively copies an entire directory tree to a new location. Here’s how you can use it:
“`python
shutil.copytree(‘source_dir’, ‘destination_dir’)
“`
If the destination directory already exists, `copytree()` will raise an error. To overwrite the destination directory, you can use the `dirs_exist_ok` parameter:
“`python
shutil.copytree(‘source_dir’, ‘destination_dir’, dirs_exist_ok=True)
“`
File Copying Options
When copying files in Python, you may want to consider various options and functionalities provided by the `shutil` module:
- `shutil.copy()`: Copies the content and permissions of a file.
- `shutil.copy2()`: Similar to `copy()`, but also preserves metadata.
- `shutil.copytree()`: Recursively copies an entire directory.
Function | Description |
---|---|
`shutil.copy(src, dst)` | Copies a file from `src` to `dst`, preserving file permissions. |
`shutil.copy2(src, dst)` | Copies a file and preserves all metadata, including timestamps. |
`shutil.copytree(src, dst)` | Recursively copies an entire directory tree. |
`shutil.move(src, dst)` | Moves a file or directory to a new location, effectively performing a copy followed by a delete. |
Error Handling
When copying files, it is essential to implement error handling to manage potential issues, such as missing files or permissions errors. You can use try-except blocks around your copy operations to handle exceptions gracefully.
“`python
try:
shutil.copy(‘source.txt’, ‘destination.txt’)
except FileNotFoundError:
print(“Source file not found.”)
except PermissionError:
print(“Permission denied.”)
“`
This approach ensures that your program can continue running smoothly, even if a file operation fails.
Using the `shutil` Module
The most common way to copy files in Python is through the `shutil` module. This module provides a high-level interface for file operations, including copying files.
- `shutil.copy(src, dst)`: Copies the file from `src` to `dst`. The destination can be a directory or a file path. If the destination is a directory, the file will be copied into that directory with the same name.
- `shutil.copy2(src, dst)`: Similar to `copy`, but it also attempts to preserve metadata such as timestamps.
“`python
import shutil
Copying a file
shutil.copy(‘source.txt’, ‘destination.txt’)
Copying a file with metadata
shutil.copy2(‘source.txt’, ‘destination.txt’)
“`
Copying Directories
To copy an entire directory, `shutil` also provides functions specifically designed for this purpose.
- `shutil.copytree(src, dst)`: Recursively copies an entire directory tree rooted at `src` to the `dst` directory. The destination directory must not already exist.
“`python
import shutil
Copying a directory
shutil.copytree(‘source_directory’, ‘destination_directory’)
“`
Handling Exceptions
When copying files, it is essential to handle potential exceptions that may arise, such as file not found or permission errors. Using a try-except block can help manage these errors gracefully.
“`python
import shutil
import os
try:
shutil.copy(‘source.txt’, ‘destination.txt’)
except FileNotFoundError:
print(“The source file was not found.”)
except PermissionError:
print(“Permission denied.”)
except Exception as e:
print(f”An unexpected error occurred: {e}”)
“`
Using `os` Module for File Copying
Though less common for simple file copying, the `os` module can be utilized in combination with low-level file handling functions.
- `os.rename(src, dst)`: While primarily used for moving files, it can also be used to copy files if combined with file reading and writing operations.
“`python
import os
Copying a file using os
with open(‘source.txt’, ‘rb’) as src_file:
with open(‘destination.txt’, ‘wb’) as dst_file:
dst_file.write(src_file.read())
“`
File Types and Permissions
When copying files, keep in mind that certain file types may require specific handling, especially with permissions and executables.
File Type | Handling Recommendations |
---|---|
Text Files | Standard copy methods work fine. |
Binary Files | Use binary mode when reading/writing. |
Executables | Use `shutil.copy2` to preserve metadata. |
Symbolic Links | Use `shutil.copy` or `shutil.copytree` to maintain symlink structure. |
Conclusion on Best Practices
Utilizing the `shutil` module is generally the most efficient and straightforward method for file copying in Python. Always ensure to handle exceptions properly and consider the implications of file permissions and types during the copying process.
Expert Insights on Copying Files in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “When copying files in Python, utilizing the built-in `shutil` module is highly recommended. It provides a simple and efficient way to handle file operations, including copying files and directories, which is essential for maintaining data integrity in applications.”
Mark Thompson (Python Developer, Tech Innovations). “For beginners, the `shutil.copy()` function is a straightforward approach to copy files. It allows you to specify both the source and destination, making it an excellent choice for quick file management tasks in scripts.”
Linda Zhang (Data Scientist, AI Analytics Group). “In scenarios where you need to copy large datasets, consider using `shutil.copyfileobj()` for better performance. This method efficiently copies file-like objects and is particularly useful when working with large files or streams.”
Frequently Asked Questions (FAQs)
How can I copy a file in Python?
You can copy a file in Python using the `shutil` module, specifically the `shutil.copy()` function. This function takes the source file path and the destination file path as arguments.
What is the difference between shutil.copy() and shutil.copy2()?
The `shutil.copy()` function copies the contents and permissions of a file, while `shutil.copy2()` also copies the metadata, such as the last access and modification times, making it a more complete copy.
Can I copy an entire directory in Python?
Yes, you can copy an entire directory using `shutil.copytree()`. This function recursively copies all files and subdirectories from the source directory to the destination directory.
What happens if the destination file already exists when using shutil.copy()?
If the destination file already exists, `shutil.copy()` will overwrite it without any warning. To avoid this, you can check if the file exists before copying.
Is it possible to copy files while preserving their original permissions?
Yes, you can preserve the original permissions by using `shutil.copy2()`, which copies both the file’s contents and its metadata, including permissions.
Can I copy files across different file systems in Python?
Yes, you can copy files across different file systems using the `shutil` module. The functions like `shutil.copy()` and `shutil.copy2()` handle this seamlessly, as they work with file paths rather than the underlying file system.
Copying files in Python is a straightforward process that can be accomplished using various methods provided by the standard library. The most commonly used module for this task is `shutil`, which offers a simple and efficient way to copy files and directories. The `shutil.copy()` function allows users to copy the contents of a file from one location to another, while `shutil.copytree()` is useful for copying entire directories, preserving the structure and contents.
In addition to `shutil`, Python also provides the `os` module, which can be utilized for file operations. However, `os` is more suited for lower-level operations, such as renaming or removing files, rather than copying. For more advanced file operations, including file permissions and metadata preservation, `shutil` is the preferred choice. It is essential to handle exceptions properly when copying files to ensure that the program can manage errors such as file not found or permission denied gracefully.
mastering file copying in Python is an essential skill for developers. Utilizing the `shutil` module not only simplifies the process but also enhances code readability and maintainability. By understanding the various functions available and implementing proper error handling, developers can efficiently manage file operations in their applications
Author Profile
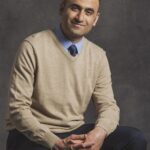
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?