How Can You Create a Dynamic Menu in Python?
Creating a menu in Python can be an exciting journey into the world of programming, where functionality meets user experience. Whether you’re building a simple console application or a more complex graphical user interface, having a well-structured menu is essential for guiding users through your software. A menu serves as the backbone of navigation, allowing users to access various features and functions with ease. In this article, we will explore the different methods and techniques to create dynamic and interactive menus in Python, empowering you to enhance your applications and engage your users effectively.
At its core, a menu in Python can be as simple or as intricate as your project demands. From basic text-based menus that utilize the command line to sophisticated GUI menus that leverage libraries like Tkinter or PyQt, the possibilities are vast. Understanding how to implement these menus involves grasping fundamental programming concepts such as loops, conditionals, and functions. As you delve into the world of menu creation, you’ll discover how to structure your code for clarity and efficiency, ensuring a smooth user experience.
Moreover, creating a menu is not just about aesthetics; it’s about functionality and usability. A well-designed menu can significantly enhance the user interface of your application, making it intuitive and easy to navigate. In the following sections, we will break down the various approaches
Creating a Simple Text-Based Menu
To create a simple text-based menu in Python, you can utilize basic input/output functions along with loops and conditional statements. This approach allows users to interact with the program by selecting options from a displayed menu. Below is an example of how to implement a straightforward menu system:
“`python
def display_menu():
print(“Menu:”)
print(“1. Option 1”)
print(“2. Option 2”)
print(“3. Option 3”)
print(“4. Exit”)
def main():
while True:
display_menu()
choice = input(“Please choose an option (1-4): “)
if choice == ‘1’:
print(“You selected Option 1.”)
elif choice == ‘2’:
print(“You selected Option 2.”)
elif choice == ‘3’:
print(“You selected Option 3.”)
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid choice. Please try again.”)
if __name__ == “__main__”:
main()
“`
In this example, the `display_menu` function prints the available options, while the `main` function handles user input and executes the corresponding actions based on the user’s choice.
Enhancing the Menu with Functions
To build a more complex menu that performs specific tasks, you can define functions for each menu option. This approach enhances modularity and makes the code easier to maintain. Here’s an enhanced version:
“`python
def option_one():
print(“Executing Option 1…”)
def option_two():
print(“Executing Option 2…”)
def option_three():
print(“Executing Option 3…”)
def display_menu():
print(“Menu:”)
print(“1. Option 1”)
print(“2. Option 2”)
print(“3. Option 3”)
print(“4. Exit”)
def main():
while True:
display_menu()
choice = input(“Please choose an option (1-4): “)
if choice == ‘1’:
option_one()
elif choice == ‘2’:
option_two()
elif choice == ‘3’:
option_three()
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid choice. Please try again.”)
if __name__ == “__main__”:
main()
“`
This structured approach allows each option to be handled by its own function, making it easier to expand the menu’s capabilities.
Using a Dictionary for Menu Options
For more advanced use cases, consider using a dictionary to map menu choices to their corresponding functions. This technique reduces the number of conditional statements and enhances readability:
“`python
def option_one():
print(“Executing Option 1…”)
def option_two():
print(“Executing Option 2…”)
def option_three():
print(“Executing Option 3…”)
def display_menu():
print(“Menu:”)
print(“1. Option 1”)
print(“2. Option 2”)
print(“3. Option 3”)
print(“4. Exit”)
def main():
options = {
‘1’: option_one,
‘2’: option_two,
‘3’: option_three,
‘4’: exit
}
while True:
display_menu()
choice = input(“Please choose an option (1-4): “)
action = options.get(choice)
if action:
action()
else:
print(“Invalid choice. Please try again.”)
if __name__ == “__main__”:
main()
“`
This method allows for easy addition of new options by simply updating the dictionary without modifying the control flow logic.
Table of Menu Options
For better organization, you can present the menu options in a tabular format. Here’s how to create a simple table within a Python script:
Option Number | Description |
---|---|
1 | Option 1 Description |
2 | Option 2 Description |
3 | Option 3 Description |
4 | Exit |
Utilizing such tables can help clarify the menu options for users, especially in more complex applications.
Creating a Simple Text-Based Menu in Python
To create a simple text-based menu in Python, you can utilize basic control flow statements such as loops and conditionals. Below is a straightforward approach to implement such a menu.
“`python
def display_menu():
print(“1. Option One”)
print(“2. Option Two”)
print(“3. Option Three”)
print(“4. Exit”)
def main():
while True:
display_menu()
choice = input(“Please select an option: “)
if choice == ‘1’:
print(“You selected Option One.”)
elif choice == ‘2’:
print(“You selected Option Two.”)
elif choice == ‘3’:
print(“You selected Option Three.”)
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid choice. Please try again.”)
if __name__ == “__main__”:
main()
“`
Enhancing the Menu with Functions
For better organization and maintainability, you can define separate functions for each menu option. This modular approach allows for easier modifications and debugging.
“`python
def option_one():
print(“You are now in Option One.”)
def option_two():
print(“You are now in Option Two.”)
def option_three():
print(“You are now in Option Three.”)
def display_menu():
print(“1. Option One”)
print(“2. Option Two”)
print(“3. Option Three”)
print(“4. Exit”)
def main():
while True:
display_menu()
choice = input(“Please select an option: “)
if choice == ‘1’:
option_one()
elif choice == ‘2’:
option_two()
elif choice == ‘3’:
option_three()
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid choice. Please try again.”)
if __name__ == “__main__”:
main()
“`
Creating a Graphical User Interface (GUI) Menu
For a more sophisticated application, consider using a GUI library such as Tkinter. This allows you to create a visually appealing menu.
“`python
import tkinter as tk
from tkinter import messagebox
def option_one():
messagebox.showinfo(“Option One”, “You selected Option One.”)
def option_two():
messagebox.showinfo(“Option Two”, “You selected Option Two.”)
def option_three():
messagebox.showinfo(“Option Three”, “You selected Option Three.”)
def create_menu(root):
menu = tk.Menu(root)
root.config(menu=menu)
submenu = tk.Menu(menu)
menu.add_cascade(label=”Options”, menu=submenu)
submenu.add_command(label=”Option One”, command=option_one)
submenu.add_command(label=”Option Two”, command=option_two)
submenu.add_command(label=”Option Three”, command=option_three)
submenu.add_separator()
submenu.add_command(label=”Exit”, command=root.quit)
def main():
root = tk.Tk()
root.title(“Python Menu Example”)
create_menu(root)
root.mainloop()
if __name__ == “__main__”:
main()
“`
Using External Libraries for More Complex Menus
For applications requiring more advanced menu features, consider using libraries like `PyQt` or `Kivy`. These libraries provide extensive options for customizing and designing menus.
Library | Description | Suitable For |
---|---|---|
PyQt | A set of Python bindings for Qt libraries | Advanced desktop applications |
Kivy | An open-source Python library for developing multitouch applications | Mobile applications and games |
Utilizing these libraries often involves a steeper learning curve but results in more professional and polished user interfaces.
Expert Insights on Creating Menus in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a menu in Python can be accomplished effectively using various methods, including lists and dictionaries. A well-structured menu improves user interaction and enhances the overall experience of the application.”
Michael Chen (Python Developer, CodeCraft Solutions). “Utilizing libraries such as Tkinter for GUI applications allows for the creation of visually appealing menus. This not only streamlines functionality but also engages users more effectively.”
Sarah Patel (Educational Technologist, LearnPython.org). “When teaching how to create menus in Python, it is essential to emphasize the importance of user input handling. Implementing error-checking mechanisms ensures that the program remains robust and user-friendly.”
Frequently Asked Questions (FAQs)
How do I create a simple text menu in Python?
To create a simple text menu in Python, use a loop to display options and input from the user. Utilize conditional statements to execute different functions based on the user’s choice.
What libraries can I use to create a graphical menu in Python?
You can use libraries such as Tkinter, PyQt, or Kivy to create graphical menus in Python. These libraries provide tools for building user interfaces with buttons, labels, and other widgets.
How can I handle user input in a Python menu?
You can handle user input using the `input()` function for text-based menus. For graphical menus, event handling methods specific to the library you are using will allow you to capture user interactions.
Is it possible to create a dynamic menu in Python?
Yes, you can create a dynamic menu in Python by using data structures like lists or dictionaries to store menu options. This allows you to modify the menu at runtime based on user input or other conditions.
Can I implement submenus in a Python menu system?
Yes, you can implement submenus in a Python menu system by nesting functions or using additional loops to display options when a user selects a specific item from the main menu.
What are some best practices for designing a menu in Python?
Best practices include keeping the menu simple and intuitive, providing clear instructions, validating user input, and ensuring that the menu is responsive to different user choices to enhance the overall user experience.
Creating a menu in Python can be achieved through various methods, depending on the complexity and requirements of the application. A simple text-based menu can be implemented using basic control structures such as loops and conditionals. For more advanced applications, graphical user interfaces (GUIs) can be developed using libraries like Tkinter or PyQt, which provide a more interactive and user-friendly experience.
When designing a menu, it is essential to consider the user experience. The menu should be intuitive and easy to navigate, allowing users to access different functionalities with minimal effort. Clear labeling of menu options and providing feedback for user actions are crucial elements that enhance usability. Additionally, implementing error handling and validation can prevent issues that may arise from user input.
In summary, whether you opt for a simple command-line interface or a sophisticated GUI, the principles of clarity, usability, and functionality remain paramount. By focusing on these aspects, developers can create effective menus that improve the overall user experience in their Python applications.
Author Profile
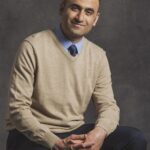
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?