How Can You Create a New Line in Python?
In the world of programming, the ability to format output effectively is crucial for creating readable and user-friendly applications. One of the simplest yet most powerful formatting techniques is the use of new lines. In Python, this fundamental skill can enhance the clarity of your code and improve the overall user experience. Whether you’re crafting a console application, generating reports, or simply displaying data, knowing how to create a new line can make all the difference in presenting your information in a structured and engaging manner.
Creating a new line in Python is a straightforward process that can be achieved through various methods, each suited to different contexts and preferences. From using escape characters to leveraging built-in functions, Python offers multiple ways to break up text and enhance readability. Understanding these techniques not only helps in organizing output but also contributes to the aesthetic appeal of your programs, making them more professional and polished.
As we delve deeper into the topic, we will explore the various approaches to inserting new lines in Python, providing you with the tools you need to elevate your coding skills. Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine your output formatting, this guide will equip you with the knowledge to effectively manage line breaks and enhance your Python projects.
Using the Newline Character
In Python, the most common way to create a new line in a string is by using the newline character, represented as `\n`. This character can be included in strings to denote where a line break should occur. For example:
“`python
print(“Hello,\nWorld!”)
“`
This code will output:
“`
Hello,
World!
“`
The newline character can be utilized in various contexts, including string concatenation, formatted strings, or when writing to files.
Using Triple Quotes
Another method to create multi-line strings is by using triple quotes, either `”’` or `”””`. This allows you to write strings that span multiple lines without explicitly including newline characters. For instance:
“`python
multi_line_string = “””This is the first line.
This is the second line.
This is the third line.”””
print(multi_line_string)
“`
The output will be:
“`
This is the first line.
This is the second line.
This is the third line.
“`
This method is particularly useful for documentation strings (docstrings) or when you need to define a string that contains several lines of text.
Using the `print()` Function
The `print()` function in Python also has parameters that can help control how output is displayed. The `end` parameter can be set to a newline character to ensure each print statement starts on a new line. For example:
“`python
print(“Line 1″, end=”\n”)
print(“Line 2″, end=”\n”)
“`
You can also use `print()` to output multiple items separated by a newline:
“`python
print(“Line 1”, “Line 2″, sep=”\n”)
“`
This will produce:
“`
Line 1
Line 2
“`
Combining New Lines with Other Formatting
When formatting strings, you may want to combine new lines with other elements. Below is a table that summarizes various methods for creating new lines and their usage contexts:
Method | Usage Example | Description |
---|---|---|
Newline Character | `”Hello,\nWorld!”` | Inserts a new line within a string. |
Triple Quotes | “`”””Text here…”””“` | Creates multi-line strings easily. |
`print()` Function | `print(“Text”, end=”\n”)` | Controls line breaks when printing to console. |
Using `sep` Parameter | `print(“Line 1”, “Line 2″, sep=”\n”)` | Prints multiple arguments with new lines in between. |
Each of these methods can be applied depending on the specific requirements of your program and the desired output format.
Using the Print Function
In Python, the most common way to create a new line in output is by using the `print()` function. By default, `print()` adds a newline character at the end of its output. However, you can control the line breaks explicitly using the newline character `\n`.
Example:
“`python
print(“Hello, World!”)
print(“This is a new line.”)
“`
Output:
“`
Hello, World!
This is a new line.
“`
To include a newline character within a single `print()` statement:
“`python
print(“Hello,\nWorld!”)
“`
Output:
“`
Hello,
World!
“`
String Formatting Techniques
String formatting methods also allow for the insertion of new lines. You can utilize formatted strings (f-strings), the `format()` method, or the `%` operator.
Using f-strings:
“`python
name = “Alice”
message = f”Hello, {name}!\nWelcome to Python.”
print(message)
“`
Using the `format()` method:
“`python
message = “Hello, {}!\nWelcome to Python.”.format(name)
print(message)
“`
Using the `%` operator:
“`python
message = “Hello, %s!\nWelcome to Python.” % name
print(message)
“`
In each case, the `\n` character creates a new line in the output.
Multi-line Strings
Python supports multi-line strings, which are enclosed in triple quotes (`”’` or `”””`). This method inherently includes new lines based on how the string is formatted.
Example:
“`python
multi_line_string = “””This is the first line.
This is the second line.
This is the third line.”””
print(multi_line_string)
“`
Output:
“`
This is the first line.
This is the second line.
This is the third line.
“`
Joining Strings with New Lines
You can create a new line by joining strings with the newline character using the `join()` method.
Example:
“`python
lines = [“This is line one.”, “This is line two.”, “This is line three.”]
output = “\n”.join(lines)
print(output)
“`
Output:
“`
This is line one.
This is line two.
This is line three.
“`
Writing to Files with New Lines
When writing to files, you can also include new lines by using the same `\n` character.
Example:
“`python
with open(“output.txt”, “w”) as file:
file.write(“First line.\nSecond line.\nThird line.”)
“`
This will create a file named `output.txt` containing:
“`
First line.
Second line.
Third line.
“`
Summary of Methods
Method | Description |
---|---|
`print()` | Basic output with automatic newline. |
`\n` in strings | Explicitly define new lines in strings. |
Multi-line strings | Use triple quotes for multi-line text. |
`join()` method | Combine multiple strings with new lines. |
File writing | Include `\n` when writing strings to files. |
Expert Insights on Creating New Lines in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “In Python, creating a new line is typically achieved using the newline character, represented as ‘\\n’. This character can be included in strings to format output, making it essential for enhancing readability in text processing.”
Michael Thompson (Lead Python Instructor, Code Academy). “When teaching beginners how to create a new line in Python, I emphasize the importance of understanding escape sequences. The ‘\\n’ character is a fundamental tool that allows programmers to control text flow in console applications and file outputs.”
Sarah Jenkins (Data Scientist, Analytics Solutions). “For data visualization and reporting in Python, using the newline character is crucial for formatting multi-line outputs. It helps in structuring data presentation, especially when generating reports or logs that require clear separation of information.”
Frequently Asked Questions (FAQs)
How do I create a new line in Python using the print function?
To create a new line in Python using the print function, you can use the newline character `\n` within the string. For example, `print(“Hello\nWorld”)` will output “Hello” on one line and “World” on the next line.
Is there a way to create multiple new lines in Python?
Yes, you can create multiple new lines by using multiple newline characters. For instance, `print(“Hello\n\nWorld”)` will insert an empty line between “Hello” and “World”.
Can I use triple quotes to create new lines in Python?
Yes, triple quotes (either `”’` or `”””`) allow you to create multi-line strings in Python. For example, `print(“””Hello\nWorld”””)` will print “Hello” and “World” on separate lines.
What is the difference between using `\n` and triple quotes for new lines?
The `\n` character is used within a single-line string to indicate a new line, while triple quotes allow you to define a string that spans multiple lines without needing to include `\n` explicitly.
Can I create a new line in Python without using print?
Yes, you can create a new line in Python in other contexts, such as writing to a file. For example, when using `file.write(“Hello\n”)`, it will create a new line in the file.
How can I ensure compatibility across different operating systems when creating new lines?
To ensure compatibility, use the `os.linesep` constant from the `os` module, which provides the appropriate newline character(s) for the operating system. For example, `import os; print(“Hello” + os.linesep + “World”)` will work correctly on any OS.
Creating a new line in Python is a fundamental aspect of formatting output and enhancing the readability of text. The most common method to achieve this is by using the newline character, represented as `\n`. When included in a string, this character instructs Python to break the line at that point, effectively moving any subsequent text to the next line. This method can be utilized in various contexts, including print statements and string concatenation.
Another approach to creating new lines is by using the `print()` function with the `end` parameter. By default, the `print()` function ends with a newline, but you can customize this behavior. For instance, setting `end` to an empty string or a specific character allows for more control over how output is formatted. This flexibility can be particularly useful when you need to format output in a specific manner or when dealing with multiple print statements in succession.
Additionally, Python’s triple quotes enable multi-line strings, allowing developers to create strings that span multiple lines without needing explicit newline characters. This feature is particularly advantageous when working with longer text blocks or when embedding formatted text directly into code. Understanding these various methods equips Python developers with the tools necessary to effectively manage and format text output in their applications.
Author Profile
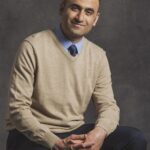
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?