How Can You Create a Queue in Python? A Step-by-Step Guide
In the world of programming, managing data efficiently is crucial for building responsive and robust applications. One fundamental structure that plays a pivotal role in this realm is the queue. Imagine a line of people waiting for their turn—this simple analogy captures the essence of a queue in computer science. In Python, creating and managing queues is not only straightforward but also essential for tasks like scheduling, handling asynchronous operations, and ensuring that data is processed in the order it arrives. Whether you’re developing a web application, a game, or a data processing system, understanding how to create and manipulate queues can significantly enhance your coding toolkit.
Queues are a type of data structure that follows the First In, First Out (FIFO) principle, meaning that elements are added to the back of the queue and removed from the front. This behavior is particularly useful in scenarios where order matters, such as task scheduling or managing requests in a server environment. Python offers several ways to implement queues, each with its unique features and benefits, allowing developers to choose the best approach for their specific needs.
From utilizing built-in libraries to crafting custom implementations, the process of creating a queue in Python is both versatile and accessible. As we delve deeper into the topic, you will discover various techniques, including the use of lists,
Using Python’s Built-in Queue Module
Python provides a built-in module called `queue` that allows for the creation of queue data structures. This module includes several types of queues, such as FIFO (First In, First Out), LIFO (Last In, First Out), and priority queues. To use the `queue` module, you first need to import it.
“`python
import queue
“`
The most common type of queue is the FIFO queue, which can be created using the `Queue` class. Below is an example of how to create and use a FIFO queue:
“`python
Creating a FIFO queue
fifo_queue = queue.Queue()
Adding items to the queue
fifo_queue.put(‘item1’)
fifo_queue.put(‘item2’)
Removing an item from the queue
item = fifo_queue.get()
print(item) Outputs: item1
“`
The `put()` method is used to add items to the queue, while the `get()` method retrieves and removes the item at the front of the queue.
Implementing a LIFO Queue with the queue Module
For scenarios where you need a Last In, First Out (LIFO) structure, the `LifoQueue` class can be utilized. This behaves like a stack. Here’s how to implement it:
“`python
Creating a LIFO queue
lifo_queue = queue.LifoQueue()
Adding items to the queue
lifo_queue.put(‘item1’)
lifo_queue.put(‘item2’)
Removing an item from the queue
item = lifo_queue.get()
print(item) Outputs: item2
“`
In this case, the last item added to the queue is the first one to be removed.
Creating a Priority Queue
A priority queue is another variant offered by the `queue` module, which allows items to be retrieved based on priority rather than order of addition. Here is how to create a priority queue:
“`python
Creating a priority queue
priority_queue = queue.PriorityQueue()
Adding items with priority
priority_queue.put((1, ‘item1’)) Lower number indicates higher priority
priority_queue.put((3, ‘item3’))
priority_queue.put((2, ‘item2’))
Removing items based on priority
while not priority_queue.empty():
item = priority_queue.get()
print(item) Outputs: (1, ‘item1’), (2, ‘item2’), (3, ‘item3’)
“`
In this example, the tuple’s first element is the priority, and the second element is the value. The items are retrieved in order of priority.
Custom Queue Implementation
While the `queue` module is useful, you may also implement your own queue using a list. Below is a simple example of how to create a basic queue class:
“`python
class CustomQueue:
def __init__(self):
self.items = []
def is_empty(self):
return not self.items
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.pop(0)
raise IndexError(“Dequeue from an empty queue”)
Usage
custom_queue = CustomQueue()
custom_queue.enqueue(‘item1’)
custom_queue.enqueue(‘item2’)
print(custom_queue.dequeue()) Outputs: item1
“`
This implementation contains methods to check if the queue is empty, add items, and remove items.
Comparison of Queue Types
The following table summarizes the differences between the various queue types in Python:
Queue Type | Order | Class |
---|---|---|
FIFO Queue | First In, First Out | queue.Queue |
LIFO Queue | Last In, First Out | queue.LifoQueue |
Priority Queue | Based on priority | queue.PriorityQueue |
These diverse implementations allow for flexibility depending on the specific needs of your application.
Using the Queue Module
The `queue` module in Python provides a thread-safe FIFO (First In, First Out) implementation. It is particularly useful in multi-threaded programming for managing tasks.
To create a queue using this module, follow the steps below:
“`python
import queue
Create a FIFO queue
my_queue = queue.Queue()
“`
You can perform several operations with a queue:
- Adding items: Use `put()` to add items to the queue.
- Removing items: Use `get()` to remove and return an item from the queue.
- Check if empty: Use `empty()` to check if the queue is empty.
- Check size: Use `qsize()` to get the approximate size of the queue.
Example
“`python
my_queue.put(1) Add an item
my_queue.put(2)
print(my_queue.get()) Returns 1
print(my_queue.empty()) Returns
print(my_queue.qsize()) Returns 1
“`
Using Collections.deque
For scenarios where you may need a more flexible queue implementation, the `collections` module provides `deque`, which can be used as both a stack and a queue.
To create a deque as a queue:
“`python
from collections import deque
Create a deque
my_deque = deque()
“`
Operations for a deque include:
- Adding items: Use `append()` to add items to the right end, or `appendleft()` to add items to the left end.
- Removing items: Use `pop()` to remove items from the right end, or `popleft()` to remove items from the left end.
Example
“`python
my_deque.append(1) Add to the right
my_deque.append(2)
print(my_deque.popleft()) Returns 1
print(my_deque) deque([2])
“`
Using List as a Queue
Although using a list as a queue is possible, it is not recommended due to performance issues. Adding and removing elements from the front of a list has a time complexity of O(n).
Example
“`python
my_list = []
Adding items
my_list.append(1) Add to the end
my_list.append(2)
Removing items
first_item = my_list.pop(0) Returns 1
“`
While this method works, it is advisable to use `queue.Queue` or `collections.deque` for efficient queue management.
In Python, there are multiple methods to create a queue, each with its advantages depending on the specific requirements of your application. The `queue` module offers a robust, thread-safe option, while `collections.deque` provides flexibility. Using lists as queues is generally less efficient and should be avoided in performance-critical applications.
Expert Insights on Creating Queues in Python
Dr. Emily Carter (Software Engineer, Python Software Foundation). “When creating a queue in Python, utilizing the `queue` module is essential for thread-safe operations. This module provides a simple way to implement FIFO (First In, First Out) queues, which can be crucial for managing tasks in concurrent programming.”
Michael Chen (Data Scientist, Tech Innovations Inc.). “For data processing tasks, I often recommend using the `collections.deque` for queue implementation in Python. It offers O(1) time complexity for appends and pops from both ends, making it highly efficient for large datasets.”
Sarah Patel (Senior Python Developer, CodeCraft Solutions). “In scenarios where you need a priority queue, the `heapq` module is invaluable. It allows you to maintain a sorted order of elements, which is particularly useful for scheduling tasks based on priority.”
Frequently Asked Questions (FAQs)
How can I create a queue in Python?
You can create a queue in Python using the `queue` module, which provides a `Queue` class. You can instantiate it with `queue.Queue()` and use methods like `put()` to add items and `get()` to remove them.
What is the difference between a list and a queue in Python?
A list allows random access and can be modified at any position, while a queue follows the FIFO (First In, First Out) principle, ensuring that elements are processed in the order they were added.
Are there any built-in libraries for implementing queues in Python?
Yes, the `queue` module is a built-in library that provides thread-safe queues. Additionally, the `collections` module offers `deque`, which can also be used to implement queues efficiently.
Can I implement a queue using a list in Python?
Yes, you can use a list to implement a queue by using `append()` to enqueue and `pop(0)` to dequeue. However, this approach is less efficient for large queues due to the time complexity of removing the first element.
What are the common methods used with Python’s Queue class?
Common methods include `put(item)` to add an item to the queue, `get()` to remove and return an item, `qsize()` to check the number of items, and `empty()` to check if the queue is empty.
Is it possible to create a priority queue in Python?
Yes, you can create a priority queue using the `queue.PriorityQueue` class from the `queue` module, which allows you to prioritize items based on their assigned priority levels.
Creating a queue in Python can be accomplished using several approaches, depending on the specific requirements of your application. The most common methods include utilizing the built-in `list` data structure, the `collections.deque` class, and the `queue.Queue` class from the standard library. Each of these methods offers unique advantages, such as performance efficiency and thread safety, making them suitable for different scenarios.
When using a list, operations like appending and popping elements can be straightforward; however, they can lead to inefficiencies due to the time complexity of inserting or removing elements from the front of the list. On the other hand, `collections.deque` provides a more efficient way to implement a queue with O(1) time complexity for both appending and popping elements from either end. This makes it an ideal choice for scenarios where performance is critical.
For applications that require thread-safe operations, the `queue.Queue` class is recommended. It is designed to be used in multi-threaded programming and provides built-in locking mechanisms to ensure that the queue can be accessed safely by multiple threads. This class also includes additional features like task tracking, which can be beneficial in concurrent programming contexts.
In summary, the choice of
Author Profile
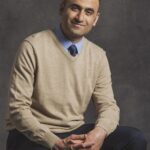
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?