How Can You Create a Timer in Python? A Step-by-Step Guide
In our fast-paced digital world, time management has become more crucial than ever. Whether you’re developing a productivity app, designing a game, or simply looking to enhance your coding skills, creating a timer in Python can be a valuable addition to your programming toolkit. With its simplicity and versatility, Python offers a range of libraries and techniques that make implementing a timer both straightforward and efficient. This article will guide you through the essentials of building a timer, empowering you to harness the power of time in your projects.
Timers are not just about counting down; they can be utilized in various applications, from scheduling tasks to managing events and even creating interactive user experiences. Python’s rich ecosystem provides several methods to create timers, each suited for different needs and levels of complexity. Whether you’re a beginner eager to learn the basics or an experienced developer looking for advanced features, understanding how to create a timer will enhance your programming capabilities and open up new possibilities for your projects.
As we delve into the world of Python timers, we’ll explore the fundamental concepts and techniques that will help you implement this essential feature. From simple countdowns to more complex implementations involving threading and asynchronous programming, you’ll discover how to effectively manage time in your applications. Get ready to unlock the potential of timers in Python
Using the time Module
To create a timer in Python, the simplest way is to utilize the built-in `time` module. This module provides various time-related functions, including the ability to pause execution for a specified duration. The `time.sleep(seconds)` function is particularly useful for creating timers.
Example of a simple countdown timer using the `time` module:
“`python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’) Overwrite the previous line
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
countdown_timer(10) 10-second timer
“`
This code snippet defines a `countdown_timer` function that counts down from a specified number of seconds. It uses `divmod` to convert seconds into minutes and seconds, formatting them for display.
Creating a Timer with the threading Module
For more advanced timing functionalities, such as non-blocking timers, the `threading` module can be beneficial. This approach allows you to run a timer in a separate thread, which means your main program can continue executing while the timer counts down.
Here’s an example of a timer that runs in a separate thread:
“`python
import threading
import time
def timer(seconds):
print(f’Timer started for {seconds} seconds.’)
time.sleep(seconds)
print(“Timer finished!”)
Create a thread for the timer
timer_thread = threading.Thread(target=timer, args=(5,))
timer_thread.start()
print(“You can continue doing other things while the timer runs.”)
“`
In this example, the `timer` function runs in a separate thread, allowing the main program to remain responsive.
Using the datetime Module
If you need to work with more complex timing scenarios, such as measuring elapsed time, the `datetime` module can be useful. This module allows you to capture the current time and calculate the difference between two time points.
Here is how you can implement a simple stopwatch:
“`python
from datetime import datetime
def stopwatch():
start_time = datetime.now()
input(“Press Enter to stop the stopwatch…”)
end_time = datetime.now()
elapsed_time = end_time – start_time
print(f’Elapsed time: {elapsed_time}’)
stopwatch()
“`
This code captures the start time when the function is called and calculates the elapsed time when the user presses Enter.
Comparison of Timer Approaches
The following table summarizes the different timer approaches discussed:
Method | Pros | Cons |
---|---|---|
time.sleep() | Simple to implement; straightforward | Blocking; halts the main program |
threading | Non-blocking; allows multitasking | More complex; requires thread management |
datetime | Flexible; great for measuring elapsed time | Less intuitive for countdowns |
By choosing the appropriate method based on the project requirements, developers can efficiently implement timers in their Python applications.
Creating a Simple Timer with Time Module
To create a basic timer in Python, you can utilize the built-in `time` module. This approach allows you to measure the elapsed time of a specific code block or event.
“`python
import time
start_time = time.time() Record the start time
Code block or task to measure goes here
time.sleep(5) Simulating a task with a 5-second sleep
end_time = time.time() Record the end time
elapsed_time = end_time – start_time Calculate elapsed time
print(f”Elapsed Time: {elapsed_time:.2f} seconds”)
“`
This code snippet captures the start time, executes a task (in this case, a 5-second pause), and then captures the end time. The difference gives the elapsed time in seconds.
Creating a Countdown Timer
For a countdown timer, you can implement a loop that decrements a value every second until it reaches zero. Here’s how to do it:
“`python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60) Convert seconds into minutes and seconds
timer = ‘{:02d}:{:02d}’.format(mins, secs) Format the timer
print(timer, end=’\r’) Print timer on the same line
time.sleep(1) Wait for one second
seconds -= 1 Decrement the seconds
print(“Time’s up!”)
countdown_timer(10) Start a countdown from 10 seconds
“`
This function takes the number of seconds as input and displays the countdown in a formatted manner.
Using Threading for Timer Functionality
If you want to create a timer that runs in the background while the main program continues executing, using the `threading` module is effective. Here’s a sample implementation:
“`python
import threading
import time
def timer(seconds):
time.sleep(seconds)
print(“Timer finished!”)
Set a timer for 5 seconds
timer_thread = threading.Thread(target=timer, args=(5,))
timer_thread.start()
print(“Timer started…”)
Main program can continue running here
“`
This approach allows the timer to execute concurrently with other code, making it useful for applications that require multitasking.
Utilizing the Timer Class from the threading Module
Python’s `threading` module also provides a `Timer` class, which can be used to create a timer that executes a function after a specified interval.
“`python
from threading import Timer
def hello():
print(“Hello, World!”)
Set a timer to call the hello function after 3 seconds
t = Timer(3.0, hello)
t.start() Start the timer
print(“Timer started, will call hello in 3 seconds.”)
“`
In this example, the `hello` function is called after a 3-second delay, allowing for easy scheduling of tasks.
Using Asyncio for Non-blocking Timers
For asynchronous programming in Python, you can use the `asyncio` module to create non-blocking timers. This is particularly useful for I/O-bound tasks.
“`python
import asyncio
async def timer(seconds):
print(f”Timer started for {seconds} seconds.”)
await asyncio.sleep(seconds) Non-blocking sleep
print(“Timer finished!”)
async def main():
await timer(5) Start a timer for 5 seconds
asyncio.run(main())
“`
This code snippet demonstrates how to define an asynchronous timer that does not block the execution of other asynchronous tasks.
Expert Insights on Creating a Timer in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When creating a timer in Python, utilizing the built-in `time` module is essential. It provides a straightforward way to measure elapsed time and can be easily integrated into various applications.”
Michael Chen (Python Developer, CodeCraft Solutions). “For more complex timing functionalities, consider using the `threading` module. This allows you to run timers in the background without blocking the main program, which is crucial for maintaining responsiveness in applications.”
Sarah Lopez (Data Scientist, Analytics Hub). “In data-driven projects, implementing a timer can help track performance metrics. Using decorators to measure function execution time can provide insights into optimization opportunities within your code.”
Frequently Asked Questions (FAQs)
How do I create a simple countdown timer in Python?
To create a simple countdown timer in Python, you can use the `time` module. Utilize a loop to decrement the timer value and `time.sleep()` to pause execution for one second between updates.
Can I create a timer that counts up instead of down?
Yes, you can create a timer that counts up by using a loop that increments a variable. Again, use the `time.sleep()` function to control the timing interval.
What libraries can I use to create a more advanced timer in Python?
For more advanced timers, consider using libraries such as `threading` for concurrent execution or `tkinter` for graphical user interface timers. The `datetime` module can also be helpful for more complex time manipulations.
How can I format the output of my timer?
You can format the output of your timer using string formatting techniques. For example, you can use `str.format()` or f-strings to display hours, minutes, and seconds in a user-friendly format.
Is it possible to pause and resume a timer in Python?
Yes, you can implement pause and resume functionality by maintaining the current state of the timer and using flags to control the execution flow. You can store the elapsed time and continue from that point when resuming.
Can I set a timer to trigger a function after a specific duration?
Yes, you can use the `threading.Timer` class to set a timer that executes a function after a specified duration. This allows you to run code asynchronously after the timer expires.
Creating a timer in Python can be accomplished using various methods, depending on the specific requirements of the application. The most common approaches involve utilizing the built-in `time` module or leveraging the `threading` module for more advanced functionalities. A basic timer can be implemented using a simple loop that counts down from a specified duration, while more sophisticated implementations can include features such as user input, countdown displays, and the ability to run timers in the background.
Key insights into timer creation in Python include understanding the importance of time management in programming and the versatility of Python’s libraries. The `time.sleep()` function is essential for pausing execution, while threading allows for concurrent operations, enabling timers to run alongside other processes. Additionally, Python’s ability to handle exceptions ensures that timers can be made robust against user errors or unexpected inputs.
Overall, Python provides a flexible environment for creating timers, making it suitable for a wide range of applications from simple scripts to complex software systems. By mastering the techniques for timer creation, developers can enhance their productivity and create more interactive and user-friendly applications. Understanding the foundational concepts of timing mechanisms in Python is crucial for any programmer looking to optimize their code and improve user experience.
Author Profile
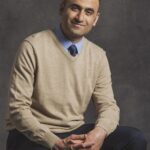
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?