How Can You Create an Empty Array in Python?
In the world of programming, arrays serve as fundamental building blocks for data organization and manipulation. Whether you’re a seasoned developer or just starting your coding journey, understanding how to create and work with arrays is essential. In Python, arrays can take various forms, and one of the most common tasks is creating an empty array. This seemingly simple operation opens the door to a myriad of possibilities, allowing you to dynamically store and manage data as your program evolves. Join us as we delve into the nuances of creating empty arrays in Python and explore their significance in efficient programming practices.
Creating an empty array in Python is a straightforward process, yet it lays the groundwork for more complex data structures and algorithms. Python offers multiple ways to define an empty array, each suited to different use cases and preferences. From using built-in data types to leveraging external libraries, the options are diverse, catering to both novice and expert programmers alike.
As we explore the methods for creating empty arrays, we will also touch upon the advantages of using arrays in Python, such as their flexibility and ease of use. Understanding these concepts will not only enhance your coding skills but also empower you to build more efficient and effective programs. So, let’s dive into the world of empty arrays and unlock the potential they hold
Creating an Empty Array in Python
To create an empty array in Python, you can utilize various approaches depending on the specific use case and the libraries you are employing. The most common methods include using the built-in list data type, the `array` module, and the NumPy library.
Using a List
The simplest way to create an empty array in Python is by using an empty list. Lists are dynamic arrays that can hold a collection of items. To create an empty list, you can use either of the following methods:
“`python
empty_list_1 = []
empty_list_2 = list()
“`
Both of these statements will create an empty list that can later be populated with elements.
Using the Array Module
If you require a more type-specific array, Python’s built-in `array` module can be utilized. This module provides a way to create an array that is constrained to a specific data type. Here’s how to create an empty array using the `array` module:
“`python
import array
empty_array = array.array(‘i’) ‘i’ indicates an array of integers
“`
In this example, an empty array of integers is created. You can specify different type codes to create arrays of other types, such as:
- `’f’` for float
- `’d’` for double
- `’b’` for signed char
Using NumPy
For more advanced array manipulations, the NumPy library is highly recommended. It provides a powerful array object that supports a wide range of mathematical operations. To create an empty array using NumPy, you first need to install the library (if you haven’t already):
“`bash
pip install numpy
“`
After installation, you can create an empty array as follows:
“`python
import numpy as np
empty_numpy_array = np.array([])
“`
This creates a NumPy array that is empty but can be resized and filled with data as needed.
Comparison of Methods
The choice of which method to use depends on the requirements of your project. Below is a comparison table summarizing the characteristics of each approach:
Method | Type | Use Case | Flexibility |
---|---|---|---|
List | Dynamic | General purpose, heterogeneous data | Highly flexible |
Array Module | Type-specific | Performance-sensitive applications | Less flexible than lists |
NumPy | Multidimensional | Scientific computing, large datasets | Very flexible with advanced features |
By understanding these methods and their characteristics, you can choose the most suitable approach for creating an empty array in Python based on your specific needs.
Methods to Create an Empty Array in Python
In Python, an array can be represented using different data structures, most commonly through lists or the array module. Here are the primary methods to create an empty array.
Using Lists
The most straightforward way to create an empty array is by using a list. Lists are versatile and allow for dynamic resizing.
“`python
empty_list = []
“`
Alternatively, you can use the `list()` constructor:
“`python
empty_list = list()
“`
Both methods result in an empty list that can be populated later.
Using the Array Module
For numerical data, Python provides an `array` module that offers a more efficient way to store elements of the same type. To create an empty array using this module, you need to import it first:
“`python
import array
empty_array = array.array(‘i’) ‘i’ indicates the type (integer)
“`
You can replace `’i’` with other type codes as needed:
Type Code | C Type | Python Type |
---|---|---|
‘b’ | signed char | int |
‘B’ | unsigned char | int |
‘u’ | Py_UNICODE | str |
‘h’ | signed short | int |
‘H’ | unsigned short | int |
‘i’ | signed int | int |
‘I’ | unsigned int | int |
‘l’ | signed long | int |
‘L’ | unsigned long | int |
‘f’ | float | float |
‘d’ | double | float |
Using NumPy Arrays
If you are working with scientific computing, you may prefer using NumPy, a powerful library for numerical operations. To create an empty array with NumPy, you can use the `numpy.empty()` function:
“`python
import numpy as np
empty_numpy_array = np.empty((0,))
“`
This creates an empty one-dimensional NumPy array. You can specify different shapes by changing the tuple passed to `np.empty()`.
Using List Comprehensions
Another method to generate an empty array is through list comprehensions, although this is less common for just creating an empty array:
“`python
empty_array = [x for x in []]
“`
This approach is generally unnecessary for creating an empty array but can be useful in more complex situations.
To summarize, you can create an empty array in Python using lists, the array module, or NumPy, depending on your specific needs and the type of data you intend to store. Each method has its own advantages, making Python a flexible language for handling arrays.
Expert Insights on Creating Empty Arrays in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Creating an empty array in Python can be efficiently achieved using the NumPy library. By utilizing `numpy.array([])`, you can initialize an empty array that can later be populated with data, which is crucial for data manipulation and analysis.”
Michael Chen (Software Engineer, Python Development Group). “For beginners, the simplest way to create an empty array in Python is by using a list. By declaring `empty_list = []`, you establish an empty list that can serve as an array for various operations, making it a versatile choice in many programming scenarios.”
Sarah Patel (Python Instructor, Code Academy). “It’s important to understand the distinction between lists and arrays in Python. While lists are created with `[]`, arrays can be created using the `array` module with `array(‘d’, [])` for a double-precision floating-point array. This distinction is essential for optimizing performance in numerical computations.”
Frequently Asked Questions (FAQs)
How do I create an empty array in Python?
You can create an empty array in Python using the `array` module by initializing it with `array(‘typecode’)`, where `typecode` specifies the type of elements. Alternatively, you can use a list by simply assigning an empty list `[]`.
What is the difference between an array and a list in Python?
An array in Python is a data structure that stores elements of the same type, while a list can store elements of different types. Arrays are more efficient for numerical operations, whereas lists offer more flexibility.
Can I create an empty array with a specific data type in Python?
Yes, you can create an empty array with a specific data type by using the `array` module. For example, `array(‘i’)` creates an empty array of integers.
What are the common use cases for arrays in Python?
Common use cases for arrays in Python include numerical computations, data manipulation, and situations where performance is critical, such as in scientific computing or when working with large datasets.
Are there any libraries that enhance array functionality in Python?
Yes, libraries such as NumPy provide enhanced functionality for arrays, including multi-dimensional arrays, mathematical operations, and advanced indexing techniques, making them suitable for complex data analysis.
How do I check if an array is empty in Python?
You can check if an array is empty by using the `len()` function. For example, `len(my_array) == 0` returns `True` if the array is empty.
Creating an empty array in Python can be accomplished using several methods, depending on the specific requirements of the application. The most common way to create an empty array is by using the built-in list data structure, which can be initialized simply by assigning an empty pair of square brackets, like so: `empty_array = []`. This approach is straightforward and leverages Python’s dynamic typing and flexibility.
For those who require more specialized array functionality, the NumPy library offers an alternative. By importing NumPy, one can create an empty array using the `numpy.empty()` function, which allows for the specification of the desired shape of the array. For example, `import numpy as np; empty_array = np.empty((0,))` creates an empty one-dimensional NumPy array. This method is particularly useful for numerical computations and operations that require multi-dimensional arrays.
In summary, Python provides multiple options for creating empty arrays, catering to different needs and use cases. Whether using native lists for general-purpose programming or leveraging NumPy for scientific computing, users can choose the method that best fits their requirements. Understanding these options allows for greater flexibility and efficiency in Python programming.
Author Profile
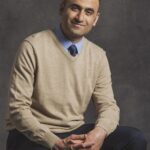
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?