How Can You Create an Empty List in Python?
Creating an empty list in Python is one of the fundamental skills every programmer should master. Lists are versatile data structures that allow you to store collections of items, making them essential for a wide range of applications—from simple data storage to complex algorithms. Whether you’re a beginner taking your first steps in programming or an experienced coder looking to refresh your knowledge, understanding how to create and manipulate lists is crucial. In this article, we will explore the various methods to create an empty list in Python, highlighting their uses and best practices.
At its core, an empty list serves as a blank canvas for data organization. In Python, lists are dynamic, meaning you can add, remove, and modify elements as needed. This flexibility makes them perfect for tasks that require iterative processing or dynamic data handling. By learning how to create an empty list, you open the door to a myriad of programming possibilities, from data collection to algorithm implementation.
In this exploration, we will delve into the syntax and methods available for initializing an empty list. We will also touch upon the significance of lists in Python programming and how they can enhance your coding efficiency. Whether you’re preparing to tackle data analysis, web development, or any other field that utilizes Python, mastering the creation of empty lists is a stepping stone toward becoming a proficient programmer
Creating an Empty List
In Python, creating an empty list is a straightforward process. Lists are one of the most versatile data structures in Python, allowing you to store a collection of items. An empty list can be useful as a starting point for later additions or manipulations.
There are two primary ways to create an empty list in Python:
- Using square brackets
- Using the `list()` constructor
Both methods will yield the same result—a list that contains no elements.
Using Square Brackets
The most common way to create an empty list is by using square brackets. This method is concise and preferred for its simplicity.
python
empty_list = []
After executing this code, the variable `empty_list` will reference an empty list. You can confirm this by printing the variable:
python
print(empty_list) # Output: []
Using the list() Constructor
Another method to create an empty list is by using the built-in `list()` function. This method is equally effective and can be particularly useful for clarity in certain contexts.
python
empty_list = list()
This code also results in `empty_list` being an empty list. Again, you can check its contents:
python
print(empty_list) # Output: []
Comparison of Methods
Both methods of creating an empty list are valid; however, there are slight differences that may influence your choice based on context or coding style.
Method | Syntax | Use Case |
---|---|---|
Square Brackets | empty_list = [] |
Preferred for brevity and simplicity |
list() Constructor | empty_list = list() |
Useful in situations requiring clarity or when type conversion is involved |
In summary, both methods are efficient and can be used interchangeably to create an empty list in Python. Your choice may depend on personal or team coding standards, but either approach will effectively initialize an empty list for further use in your program.
Creating an Empty List in Python
In Python, an empty list can be created using two primary methods. Both approaches are widely accepted and serve the same purpose, allowing developers to initialize a list that can be populated later.
Method 1: Using Square Brackets
The most common way to create an empty list is by using square brackets. This approach is straightforward and easy to remember.
python
empty_list = []
This line of code initializes `empty_list` as an empty list that can later hold elements.
Method 2: Using the `list()` Constructor
Another method to create an empty list is by using the built-in `list()` function. This method is particularly useful for readability in certain contexts.
python
empty_list = list()
Both methods will yield the same result, and the choice between them often comes down to personal preference or stylistic guidelines within a project.
Verifying an Empty List
Once an empty list is created, you may want to verify that it is indeed empty. This can be done using the `len()` function or by directly evaluating the list in a conditional statement.
- Using `len()`:
python
print(len(empty_list)) # Output: 0
- Using a conditional:
python
if not empty_list:
print(“The list is empty.”)
This code checks if the list is empty and prints a message if true.
Adding Elements to an Empty List
After creating an empty list, you can populate it using various methods, such as `append()`, `extend()`, or `insert()`.
- Using `append()`:
python
empty_list.append(1) # Adds 1 to the list
- Using `extend()`:
python
empty_list.extend([2, 3]) # Adds multiple elements
- Using `insert()`:
python
empty_list.insert(0, 0) # Inserts 0 at the beginning
Each method provides a different way to manage how elements are added to the list, allowing for flexibility in data management.
Example of Creating and Using an Empty List
Here’s a complete example demonstrating the creation of an empty list and populating it with elements:
python
# Create an empty list
my_list = []
# Add elements to the list
my_list.append(“apple”)
my_list.append(“banana”)
my_list.extend([“cherry”, “date”])
# Output the list
print(my_list) # Output: [‘apple’, ‘banana’, ‘cherry’, ‘date’]
This example showcases the ease with which lists can be manipulated after initialization.
Expert Insights on Creating an Empty List in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). Creating an empty list in Python is straightforward and essential for initializing data structures. You can use either the list constructor, `list()`, or simply use empty square brackets `[]`. Both methods are efficient and widely accepted in the Python community.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). When starting with Python, understanding how to create an empty list is a fundamental skill. Using `my_list = []` is not only concise but also enhances code readability, making it easier for others to understand your intentions when initializing a list.
Sarah Lopez (Python Educator, LearnPythonNow). Teaching beginners how to create an empty list is crucial. I recommend demonstrating both methods: `my_list = []` and `my_list = list()`. This helps students grasp the flexibility of Python while reinforcing the concept that lists can be dynamically populated later in the program.
Frequently Asked Questions (FAQs)
How do I create an empty list in Python?
To create an empty list in Python, use either of the following methods: `empty_list = []` or `empty_list = list()`. Both will initialize an empty list.
Can I add elements to an empty list after creating it?
Yes, you can add elements to an empty list using methods such as `append()`, `extend()`, or `insert()`. For example, `empty_list.append(1)` will add the element `1` to the list.
What is the difference between a list and an empty list in Python?
A list in Python is a collection of items, while an empty list contains no items. An empty list is a valid list object that can be populated with elements later.
Is it possible to create an empty list with a specific size?
No, an empty list does not have a specific size. However, you can create a list with a predefined number of elements initialized to a default value, such as `empty_list = [None] * size`.
Can I create a nested empty list in Python?
Yes, you can create a nested empty list by initializing an empty list within another list, such as `nested_empty_list = [[]]`. This creates a list containing one empty list.
What are some common use cases for empty lists in Python?
Empty lists are commonly used for initializing collections that will be populated later, collecting results from loops, or storing temporary data during processing.
Creating an empty list in Python is a fundamental skill that is essential for effective programming. There are two primary methods to achieve this: using the list constructor `list()` and using square brackets `[]`. Both methods are widely accepted and yield the same result, allowing developers to choose based on their coding style or preference.
Utilizing the list constructor can be particularly useful in scenarios where clarity is paramount, as it explicitly indicates the intention to create a list. On the other hand, using square brackets is more concise and is often favored for its simplicity and ease of use. Regardless of the method chosen, the resulting empty list can be populated later with elements as needed, making it a versatile tool in data manipulation.
In summary, understanding how to create an empty list is a basic yet crucial aspect of Python programming. Mastery of this concept not only facilitates better data management but also enhances overall coding efficiency. By familiarizing oneself with these methods, programmers can build a solid foundation for more complex data structures and algorithms in their projects.
Author Profile
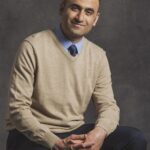
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?