How Can You Create a Data Pipeline Using Python?
In today’s data-driven world, the ability to efficiently collect, process, and analyze information has become paramount for businesses and organizations. As data continues to grow exponentially, the need for robust data pipelines has never been greater. A data pipeline is essentially a series of processes that automate the movement and transformation of data from one system to another, enabling seamless data flow and real-time insights. For those looking to harness the power of data, learning how to create a data pipeline using Python can be a game-changer, providing both flexibility and scalability.
Building a data pipeline with Python involves a series of steps that encompass data extraction, transformation, and loading (ETL). Python’s rich ecosystem of libraries and frameworks, such as Pandas, Apache Airflow, and Dask, makes it an ideal choice for developers and data engineers alike. By leveraging these tools, you can streamline the process of gathering data from various sources, cleansing and transforming it into a usable format, and ultimately loading it into your desired storage or analysis platform.
As we delve deeper into the intricacies of creating a data pipeline using Python, we’ll explore the essential components, best practices, and common challenges you may encounter along the way. Whether you’re a seasoned data professional or a newcomer eager to learn, this guide will equip
Data Ingestion
Data ingestion is the first step in the data pipeline process, where data is collected from various sources. Python offers several libraries that facilitate this process, enabling seamless integration from diverse data sources such as databases, APIs, and flat files.
Common methods for data ingestion include:
- Database Connections: Using libraries like `SQLAlchemy` or `pyodbc` to connect to databases.
- APIs: Utilizing `requests` or `http.client` to retrieve data from web APIs.
- File Handling: Reading data from CSV, JSON, and Excel files using `pandas` or built-in file handling.
Data Transformation
Once the data is ingested, it typically requires transformation to ensure it is in the correct format for analysis. This may include cleaning, normalizing, and aggregating the data. Python’s `pandas` library is particularly effective for this purpose.
Key transformation tasks may involve:
- Data Cleaning: Removing duplicates and handling missing values.
- Data Normalization: Scaling data to fit within a specific range.
- Feature Engineering: Creating new variables from existing data that enhance model performance.
Here’s a simple code snippet for a transformation using `pandas`:
“`python
import pandas as pd
Load data
data = pd.read_csv(‘data.csv’)
Clean data
data.drop_duplicates(inplace=True)
data.fillna(method=’ffill’, inplace=True)
Normalize a column
data[‘normalized_column’] = (data[‘column’] – data[‘column’].mean()) / data[‘column’].std()
“`
Data Loading
After transforming the data, the next step is to load it into a destination where it can be analyzed or used for reporting. This could be a database, a data warehouse, or even a file system.
The following libraries and methods are commonly used for data loading:
- SQLAlchemy: For loading data into SQL databases.
- pandas: For writing data frames to CSV, Excel, or other formats.
- Apache Airflow: For orchestrating complex data loading tasks.
Orchestration
Orchestration is crucial in managing the workflow of a data pipeline. Python offers several tools for orchestrating tasks, ensuring that each step of the data pipeline executes in the correct order.
Popular orchestration tools include:
- Apache Airflow: A platform to programmatically author, schedule, and monitor workflows.
- Luigi: A Python package for building complex data pipelines.
- Prefect: A modern workflow orchestration tool that integrates seamlessly with Python.
These tools allow for defining tasks, setting dependencies, and scheduling executions.
Monitoring and Maintenance
Monitoring the performance of data pipelines is essential to ensure they run efficiently and without errors. Python libraries such as `logging` and `prometheus_client` can be utilized to track pipeline performance and capture metrics.
Monitoring strategies include:
- Logging: Capturing detailed logs of the data pipeline processes to troubleshoot issues.
- Alerts: Setting up notifications for failures or performance bottlenecks.
- Data Quality Checks: Regularly validating data to ensure accuracy and completeness.
Tool | Functionality |
---|---|
Apache Airflow | Workflow orchestration and scheduling |
Luigi | Task management and dependency resolution |
Prefect | Dynamic workflows with easy debugging |
Defining Your Data Pipeline Requirements
Establishing a clear understanding of your data pipeline requirements is crucial. This involves identifying the data sources, the transformations required, and the destination for the processed data.
- Data Sources: Determine where your data will come from. Common sources include:
- Databases (SQL, NoSQL)
- APIs
- Flat files (CSV, JSON)
- Streaming data (Kafka, MQTT)
- Transformations: Outline the necessary data transformations. This may include:
- Data cleaning (removing duplicates, handling missing values)
- Data enrichment (adding additional data points)
- Data aggregation (summarizing data)
- Destination: Specify where the processed data will reside. Options include:
- Data warehouses (Amazon Redshift, Google BigQuery)
- Data lakes (Amazon S3, Azure Data Lake)
- Analytics platforms (Tableau, Power BI)
Selecting the Right Tools and Libraries
Choosing the appropriate tools and libraries is essential for building your data pipeline. Python offers a variety of libraries that can facilitate different aspects of the pipeline.
Purpose | Libraries/Tools |
---|---|
Data extraction | `pandas`, `requests`, `BeautifulSoup` |
Data transformation | `pandas`, `numpy`, `Dask` |
Data loading | `SQLAlchemy`, `pyodbc`, `boto3` |
Workflow orchestration | `Apache Airflow`, `Luigi`, `Prefect` |
Building the Data Pipeline
Construct the data pipeline by integrating the selected libraries and defining the flow of data. This can be broken down into several steps:
- Data Extraction: Use appropriate libraries to fetch data from the defined sources.
“`python
import pandas as pd
Example of extracting data from a CSV file
data = pd.read_csv(‘source_data.csv’)
“`
- Data Transformation: Apply necessary transformations to clean and prepare the data.
“`python
Example of cleaning data
data.drop_duplicates(inplace=True)
data.fillna(method=’ffill’, inplace=True)
“`
- Data Loading: Load the transformed data into the destination.
“`python
from sqlalchemy import create_engine
Example of loading data into a SQL database
engine = create_engine(‘sqlite:///mydatabase.db’)
data.to_sql(‘table_name’, con=engine, if_exists=’replace’, index=)
“`
Implementing Workflow Orchestration
To ensure the pipeline runs smoothly, implement orchestration tools that can automate and monitor the workflow.
– **Apache Airflow**: Provides a platform to programmatically author, schedule, and monitor workflows.
– **Luigi**: A Python package that helps build complex data pipelines by defining tasks and dependencies.
– **Prefect**: A modern data workflow orchestration tool that simplifies data pipeline development.
Example of a simple Airflow DAG:
“`python
from airflow import DAG
from airflow.operators.python_operator import PythonOperator
from datetime import datetime
def extract():
Extraction logic here
def transform():
Transformation logic here
def load():
Loading logic here
dag = DAG(‘data_pipeline’, start_date=datetime(2023, 1, 1), schedule_interval=’@daily’)
extract_task = PythonOperator(task_id=’extract’, python_callable=extract, dag=dag)
transform_task = PythonOperator(task_id=’transform’, python_callable=transform, dag=dag)
load_task = PythonOperator(task_id=’load’, python_callable=load, dag=dag)
extract_task >> transform_task >> load_task
“`
Testing and Monitoring the Pipeline
Establish a robust testing and monitoring process to ensure the reliability of your pipeline.
- Unit Tests: Write tests for each component of the pipeline to validate functionality.
- Integration Tests: Test the pipeline as a whole to verify that all components work together seamlessly.
- Monitoring: Utilize monitoring tools like Prometheus, Grafana, or built-in features of orchestration tools to track performance and alert on failures.
- Key Metrics to Monitor:
- Data throughput
- Failure rates
- Execution times
Implementing these practices will enhance the reliability and efficiency of your data pipeline using Python.
Expert Insights on Creating Data Pipelines with Python
Dr. Emily Chen (Data Engineer, Tech Innovations Inc.). “To create a robust data pipeline using Python, it is essential to leverage libraries such as Pandas for data manipulation and Apache Airflow for orchestration. These tools provide a solid foundation for building scalable and maintainable pipelines.”
Michael Thompson (Senior Data Scientist, Analytics Solutions Group). “The key to an effective data pipeline is to ensure that data quality is maintained throughout the process. Implementing validation checks and using Python’s built-in logging module can help track issues and enhance reliability.”
Sarah Patel (Lead Software Engineer, Cloud Data Services). “When designing a data pipeline, consider using modular programming practices in Python. This approach allows for easier testing and debugging, making your pipeline more resilient to changes and easier to extend.”
Frequently Asked Questions (FAQs)
What is a data pipeline?
A data pipeline is a series of data processing steps that involve the collection, transformation, and storage of data from various sources to a destination, enabling efficient data analysis and reporting.
How do I start creating a data pipeline using Python?
Begin by identifying the data sources and the desired output. Utilize libraries such as Pandas for data manipulation, Requests for API calls, and SQLAlchemy for database interactions. Structure your pipeline into stages: extraction, transformation, and loading (ETL).
What libraries are commonly used for building data pipelines in Python?
Common libraries include Pandas for data manipulation, NumPy for numerical operations, Requests for HTTP requests, SQLAlchemy for database connections, and Apache Airflow or Luigi for workflow management.
How can I ensure data quality in my pipeline?
Implement data validation checks at each stage of the pipeline. Use assertions, logging, and exception handling to catch errors. Additionally, consider using libraries like Great Expectations for automated data quality checks.
What are some best practices for maintaining a data pipeline?
Best practices include modularizing your code for reusability, documenting each step of the pipeline, implementing version control, and regularly monitoring performance metrics to identify bottlenecks or failures.
Can I automate my data pipeline in Python?
Yes, you can automate your data pipeline by scheduling tasks using libraries like Apache Airflow, Prefect, or by using cron jobs. Automation ensures timely data processing and minimizes manual intervention.
Creating a data pipeline using Python involves several key steps that facilitate the efficient movement and transformation of data from one system to another. The process typically begins with data ingestion, where data is collected from various sources such as databases, APIs, or files. Python libraries such as Pandas, NumPy, and requests can be employed to streamline this process, ensuring that data is gathered in a structured format suitable for further processing.
Once data is ingested, the next phase is data transformation, which includes cleaning, filtering, and aggregating the data as needed. Python’s versatile data manipulation libraries, such as Pandas, provide powerful tools to perform these operations effectively. Additionally, the use of frameworks like Apache Airflow or Luigi can help orchestrate these tasks, allowing for the automation of workflows and ensuring that data is processed in a timely manner.
Finally, the data pipeline culminates in data storage or visualization, where the processed data is saved to a database or made available for analysis. Python supports various database connectors, enabling seamless integration with systems like PostgreSQL, MySQL, or NoSQL databases. Moreover, libraries such as Matplotlib and Seaborn can be utilized for visualizing the data, providing insights that drive decision-making. Overall
Author Profile
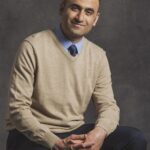
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?