How Can You Cube a Number in Python? A Step-by-Step Guide
In the world of programming, mastering the fundamental operations on numbers is crucial for building more complex algorithms and applications. One such operation that often comes up in mathematical computations is cubing a number. Whether you’re developing a game, analyzing data, or working on a scientific project, knowing how to cube a number in Python can be a handy skill to have in your toolkit. This article will guide you through the various methods to achieve this, ensuring you have a solid grasp of both the concept and its practical implementation in Python.
Cubing a number is simply raising it to the power of three, which means multiplying the number by itself twice. In Python, this can be accomplished in several ways, each with its own advantages depending on the context in which you’re working. From using the exponentiation operator to leveraging built-in functions, Python offers a range of tools that make this operation straightforward and efficient.
As we delve deeper into the topic, you’ll discover not only the syntax and methods for cubing numbers but also some practical examples that illustrate how this operation can be applied in real-world scenarios. Whether you’re a novice programmer looking to enhance your skills or an experienced developer seeking a refresher, understanding how to cube a number in Python is an essential step in your coding journey.
Methods to Cube a Number in Python
Cubing a number in Python can be accomplished through various methods, allowing flexibility based on the specific needs of your program. Here are some of the most common approaches:
Using the Exponentiation Operator
The simplest way to cube a number in Python is to use the exponentiation operator `**`. This operator raises a number to the power of another number. For cubing, you would raise the number to the power of 3.
“`python
number = 5
cubed = number ** 3
print(cubed) Output: 125
“`
Using the Built-in `pow()` Function
Another method is to utilize the built-in `pow()` function, which can also raise a number to a specified power. The syntax for this function is `pow(base, exp)` where `base` is the number you want to cube and `exp` is the exponent.
“`python
number = 4
cubed = pow(number, 3)
print(cubed) Output: 64
“`
Defining a Custom Function
For better code organization and reusability, you may want to define a custom function that takes a number as an argument and returns its cube. This is particularly useful in larger programs where you need to cube numbers in multiple places.
“`python
def cube(num):
return num ** 3
print(cube(3)) Output: 27
“`
Using NumPy for Array Operations
If you are working with arrays of numbers, using the NumPy library can be highly efficient. NumPy allows you to perform operations on entire arrays at once, including cubing each element.
“`python
import numpy as np
array = np.array([1, 2, 3, 4])
cubed_array = np.power(array, 3)
print(cubed_array) Output: [ 1 8 27 64]
“`
Comparison Table of Methods
Method | Code Example | Use Case |
---|---|---|
Exponentiation Operator | `number ** 3` | Simple cubing of a single number |
pow() Function | `pow(number, 3)` | Flexible for different exponents |
Custom Function | `def cube(num): return num ** 3` | Reusable across multiple parts of the program |
NumPy | `np.power(array, 3)` | Efficient for array operations |
Each of these methods has its advantages and can be chosen based on the context in which you are working. Whether you are performing quick calculations or developing more complex applications, Python provides the tools necessary to efficiently cube numbers.
Cubing a Number Using the Exponentiation Operator
In Python, the simplest way to cube a number is by using the exponentiation operator `**`. This operator allows you to raise a number to any power, making it straightforward to compute cubes.
“`python
number = 3
cubed = number ** 3
print(cubed) Output: 27
“`
This method is efficient and readable, suitable for both beginners and experienced programmers.
Cubing a Number with the `pow()` Function
Another approach to cube a number in Python is by utilizing the built-in `pow()` function. This function can take two arguments: the base and the exponent.
“`python
number = 4
cubed = pow(number, 3)
print(cubed) Output: 64
“`
This method is particularly useful when working with more complex calculations or when you need to compute powers dynamically.
Creating a Custom Function to Cube a Number
For scenarios where you may need to cube numbers repeatedly, defining a custom function can enhance code organization and reuse.
“`python
def cube(n):
return n ** 3
result = cube(5)
print(result) Output: 125
“`
This function encapsulates the cubing logic, making your code cleaner and easier to maintain.
Using List Comprehensions for Multiple Values
When you need to cube multiple numbers, list comprehensions provide a concise way to achieve this efficiently.
“`python
numbers = [1, 2, 3, 4, 5]
cubed_numbers = [x ** 3 for x in numbers]
print(cubed_numbers) Output: [1, 8, 27, 64, 125]
“`
This method is both elegant and Pythonic, allowing you to operate on collections of data seamlessly.
Using NumPy for Vectorized Operations
For applications involving large datasets or matrices, the NumPy library can significantly enhance performance through vectorized operations. NumPy allows you to cube an entire array in one command.
“`python
import numpy as np
array = np.array([1, 2, 3, 4, 5])
cubed_array = np.power(array, 3)
print(cubed_array) Output: [ 1 8 27 64 125]
“`
This method is highly efficient and is recommended for numerical computing tasks.
Performance Considerations
When selecting a method to cube a number in Python, consider the following aspects:
Method | Performance | Readability | Best Use Case |
---|---|---|---|
Exponentiation Operator | Fast | High | Simple calculations |
`pow()` Function | Fast | High | Dynamic power calculations |
Custom Function | Moderate | High | Repetitive calculations |
List Comprehension | Moderate | High | Bulk processing of lists |
NumPy Vectorization | Very Fast | Moderate | Large datasets and arrays |
Understanding these factors helps choose the most appropriate method based on the specific requirements of your project.
Expert Insights on Cubing Numbers in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Cubing a number in Python is straightforward, and I recommend using the exponentiation operator `**`. This method is not only concise but also enhances code readability, making it easier for others to understand the intent of the operation.”
Michael Chen (Python Software Engineer, CodeCraft Solutions). “For those looking to cube numbers in Python, leveraging a simple function can streamline the process. Defining a function like `def cube(x): return x ** 3` allows for reusable code, which is a best practice in software development.”
Laura Patel (Computer Science Educator, Future Coders Academy). “Understanding how to cube a number in Python is fundamental for beginners. Utilizing built-in functions or custom functions not only teaches the concept of cubing but also reinforces the importance of function definitions in programming.”
Frequently Asked Questions (FAQs)
How do I cube a number in Python?
To cube a number in Python, you can use the exponentiation operator ``. For example, `number 3` will return the cube of `number`.
Can I use the `pow()` function to cube a number in Python?
Yes, the `pow()` function can be used to cube a number. For instance, `pow(number, 3)` will yield the same result as `number ** 3`.
Is there a built-in function in Python specifically for cubing a number?
Python does not have a built-in function specifically for cubing. However, you can define your own function, such as `def cube(x): return x ** 3`.
What data types can be cubed in Python?
In Python, you can cube integers and floats. Both types support the exponentiation operation.
How can I cube a number in a list in Python?
You can use a list comprehension to cube each element in a list. For example, `cubed_list = [x ** 3 for x in original_list]` will create a new list with the cubed values.
Are there any performance considerations when cubing large numbers in Python?
Cubing large numbers can lead to performance issues due to increased computation time and memory usage. It is advisable to use efficient algorithms or libraries like NumPy for handling large datasets.
Cubing a number in Python is a straightforward process that can be accomplished using various methods. The most common approach is to use the exponentiation operator (), which allows you to raise a number to a specified power. For instance, to cube a number, you can simply write `number 3`. This method is both intuitive and efficient, making it a preferred choice for many programmers.
Alternatively, you can achieve the same result by utilizing the built-in `pow()` function, which also supports exponentiation. By calling `pow(number, 3)`, you can obtain the cube of the number. This function is particularly useful when you need to compute powers in a more general context, as it can handle both integers and floating-point numbers seamlessly.
Moreover, for those who prefer a more explicit approach, you can define a custom function to cube a number. This method enhances code readability and reusability, especially in larger projects. A simple function could look like this: `def cube(x): return x * x * x`. This encapsulates the logic of cubing a number and can be easily called whenever needed.
In summary, cubing a number in Python can be efficiently performed using the exponentiation
Author Profile
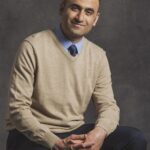
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?