How Can You Easily Cube Numbers in Python?
In the world of programming, Python stands out as a versatile language that empowers developers to tackle a wide array of tasks with ease and efficiency. Whether you’re a seasoned coder or just starting your journey, understanding how to manipulate numbers and perform mathematical operations is fundamental. One common operation that often arises in various applications is cubing a number. This seemingly simple task can be the gateway to more complex mathematical computations and data manipulations. In this article, we’ll explore the various methods to cube numbers in Python, providing you with the tools to enhance your programming skills and tackle challenges with confidence.
Cubing a number in Python can be achieved through multiple approaches, each with its own advantages and use cases. From straightforward arithmetic operations to leveraging built-in functions and libraries, Python offers flexibility that caters to different programming styles and preferences. Whether you’re working on a small script or a larger application, knowing how to efficiently cube numbers can streamline your code and improve performance.
As we delve deeper into the topic, we will examine practical examples and best practices for cubing numbers in Python. You’ll discover how to implement these methods in real-world scenarios, enhancing your understanding of both Python syntax and mathematical concepts. So, let’s embark on this mathematical journey and unlock the potential of cubing in Python!
Using the Power Operator
One of the simplest methods to cube a number in Python is by utilizing the power operator `**`. This operator allows you to raise a number to the power of another number. To cube a number, you would raise it to the power of 3.
“`python
def cube_using_power_operator(number):
return number ** 3
result = cube_using_power_operator(3)
print(result) Output: 27
“`
This method is straightforward and works seamlessly for both integers and floats.
Using the `pow()` Function
Another efficient way to perform exponentiation in Python is by using the built-in `pow()` function. The `pow()` function can take two or three arguments, but for cubing, only two are necessary.
“`python
def cube_using_pow_function(number):
return pow(number, 3)
result = cube_using_pow_function(3)
print(result) Output: 27
“`
This function provides the same results as the power operator and is equally effective.
Creating a Custom Function
For more clarity and encapsulation, you may want to define your own function that specifically cubes a number. This can enhance code readability, especially in larger projects.
“`python
def cube(number):
return number * number * number
result = cube(3)
print(result) Output: 27
“`
This method explicitly shows the multiplication process, which can be beneficial for those who prefer more transparency in their code.
Using List Comprehension for Multiple Values
If you need to cube multiple numbers, list comprehensions provide a concise way to achieve this. You can create a list of cubed values from an existing list of numbers.
“`python
numbers = [1, 2, 3, 4, 5]
cubed_numbers = [cube(num) for num in numbers]
print(cubed_numbers) Output: [1, 8, 27, 64, 125]
“`
This approach is efficient and leverages Python’s powerful list handling capabilities.
Comparison of Methods
The following table summarizes the different methods for cubing a number in Python:
Method | Code Example | Notes |
---|---|---|
Power Operator | `number ** 3` | Simple and readable |
`pow()` Function | `pow(number, 3)` | Built-in function, versatile |
Custom Function | `def cube(number): …` | Explicit and clear |
List Comprehension | `[cube(num) for num in numbers]` | Efficient for multiple values |
Each method has its own advantages, and the choice depends on the specific requirements of your project or personal coding style.
Methods to Cube a Number in Python
Cubing a number in Python can be achieved through various methods, each suitable for different scenarios. Here are the most common approaches:
Using the Exponentiation Operator
The simplest way to cube a number is by using the exponentiation operator `**`. This operator raises a number to the power of another number.
“`python
number = 3
cubed = number ** 3
print(cubed) Output: 27
“`
Using the Built-in `pow()` Function
Python’s built-in `pow()` function can also be utilized to cube a number. This function takes two arguments: the base and the exponent.
“`python
number = 4
cubed = pow(number, 3)
print(cubed) Output: 64
“`
Defining a Custom Function
Creating a custom function for cubing a number can enhance code readability, especially in larger scripts.
“`python
def cube(num):
return num ** 3
result = cube(5)
print(result) Output: 125
“`
Using NumPy for Arrays
When working with arrays or lists of numbers, the NumPy library offers an efficient way to cube each element.
“`python
import numpy as np
array = np.array([1, 2, 3, 4])
cubed_array = np.power(array, 3)
print(cubed_array) Output: [ 1 8 27 64]
“`
Using List Comprehensions
For a more Pythonic approach, list comprehensions can be employed to cube each element in a list.
“`python
numbers = [1, 2, 3, 4]
cubed_numbers = [x ** 3 for x in numbers]
print(cubed_numbers) Output: [1, 8, 27, 64]
“`
Performance Considerations
When choosing a method for cubing, consider the context and efficiency:
Method | Complexity | Use Case |
---|---|---|
Exponentiation Operator | O(1) | Simple calculations |
`pow()` Function | O(1) | Readable for mathematical operations |
Custom Function | O(1) | Enhanced code clarity |
NumPy | O(n) | Bulk operations on arrays |
List Comprehensions | O(n) | Functional programming style |
Selecting the appropriate method depends on the specific needs of your application, including readability, performance, and the size of data being processed.
Expert Insights on Cubing in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Cubing in Python can be efficiently achieved using the NumPy library, which provides powerful tools for numerical operations. By leveraging NumPy’s array capabilities, one can easily create a cubed function that applies to each element in an array, enhancing both performance and readability.”
Mark Thompson (Software Engineer, CodeCraft Solutions). “When cubing numbers in Python, it’s essential to consider both the mathematical approach and the computational efficiency. Utilizing list comprehensions can yield concise and readable code, while also optimizing performance for larger datasets.”
Sarah Lee (Python Developer, Open Source Community). “For those looking to cube values in Python, I recommend exploring the use of the built-in `map` function in conjunction with a lambda expression. This method not only simplifies the code but also makes it easier to apply the cubing operation to any iterable.”
Frequently Asked Questions (FAQs)
How do I cube a number in Python?
To cube a number in Python, you can use the exponentiation operator ``. For example, to cube the variable `x`, you would write `x 3`.
Can I create a function to cube a number in Python?
Yes, you can define a function to cube a number. For instance:
“`python
def cube(num):
return num ** 3
“`
What is the difference between cubing and squaring a number in Python?
Cubing a number raises it to the power of three, while squaring raises it to the power of two. In Python, this is represented by `num 3` for cubing and `num 2` for squaring.
Is there a built-in function in Python to cube a number?
Python does not have a built-in function specifically for cubing numbers. However, you can easily use the exponentiation operator or define your own function as shown earlier.
Can I cube a list of numbers in Python?
Yes, you can cube each number in a list using a list comprehension. For example:
“`python
cubed_numbers = [x ** 3 for x in my_list]
“`
What libraries can I use to perform mathematical operations in Python?
You can use libraries like NumPy and math for advanced mathematical operations. For cubing, NumPy allows you to cube arrays efficiently using `numpy.power(array, 3)`.
In Python, cubing a number can be accomplished through various methods, each catering to different programming styles and preferences. The most straightforward approach involves using the exponentiation operator (), which allows for a clear and concise expression of the cube operation. For example, to cube a number `x`, one can simply write `x 3`. This method is not only easy to read but also efficient for basic numerical operations.
Another common technique is to utilize the built-in `pow()` function, which serves a similar purpose. The syntax `pow(x, 3)` achieves the same result as the exponentiation operator. This method can be particularly useful when working with larger expressions or when the exponent is stored in a variable, enhancing flexibility in the code.
For those who prefer a more functional programming style, defining a custom function to cube a number can be beneficial. By creating a function such as `def cube(n): return n ** 3`, one can encapsulate the cubing logic, making it reusable throughout the code. This approach promotes better organization and readability, especially in larger projects where the cubing operation may be needed multiple times.
In summary, cubing a number in Python can be efficiently achieved through
Author Profile
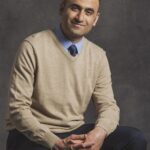
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?