How Can You Effectively Debug Cobra in Go? A Comprehensive Guide
Debugging is an essential skill for any developer, and when it comes to building command-line applications in Go using the Cobra library, it can be particularly challenging. Cobra is a powerful tool that simplifies the creation of powerful CLI applications, but like any complex system, it can present its own set of debugging challenges. Whether you’re encountering unexpected behavior, errors, or simply trying to understand how your commands interact, knowing how to effectively debug your Cobra applications can save you time and frustration. In this article, we will explore practical strategies and techniques to help you troubleshoot and refine your Cobra-based projects.
At its core, debugging Cobra applications involves a blend of understanding both the Go programming language and the specific intricacies of the Cobra library. Developers often face issues ranging from command parsing errors to unexpected output, which can stem from a variety of sources, including misconfigured flags, incorrect command hierarchies, or even logical errors in the application’s flow. By leveraging Go’s built-in debugging tools, as well as some best practices specific to Cobra, you can systematically identify and resolve these issues.
Additionally, effective debugging requires a mindset geared towards problem-solving and experimentation. As you delve into the nuances of your Cobra application, you’ll learn to utilize logging, breakpoints, and other debugging techniques to gain
Understanding Cobra’s Structure
Cobra is a powerful library in Go for creating command-line applications. To effectively debug Cobra applications, it is essential to understand its structure, including commands, flags, and arguments. Each command in Cobra is represented as a separate object, which can have its own set of flags and subcommands.
When debugging, keep the following in mind:
- Command Hierarchy: Commands can have subcommands, which means understanding the parent-child relationship is crucial.
- Flag Definitions: Ensure that flags are defined correctly and are accessible in the command’s run function.
- Argument Parsing: Verify how arguments are parsed and whether they align with expected formats.
Setting Up Logging
One of the simplest ways to debug Cobra applications is by implementing logging. Using Go’s built-in `log` package or a more advanced logging library can help track the flow of execution and capture errors. Here’s a basic setup:
“`go
import (
“log”
“os”
)
func init() {
log.SetOutput(os.Stdout)
log.SetFlags(log.Ldate | log.Ltime | log.Lshortfile)
}
“`
With this setup, you can insert log statements throughout your code to monitor the command execution paths and identify any issues.
Using Debug Flags
Adding a debug flag to your Cobra commands can provide insights during execution. Here’s how you can implement it:
- Define a boolean flag in your command.
- Use this flag to toggle verbose logging.
“`go
var debug bool
var rootCmd = &cobra.Command{
Use: “app”,
Short: “An application”,
Run: func(cmd *cobra.Command, args []string) {
if debug {
log.Println(“Debug mode enabled”)
}
// Command logic goes here
},
}
func init() {
rootCmd.Flags().BoolVarP(&debug, “debug”, “d”, , “Enable debug mode”)
}
“`
This allows you to run your application with increased verbosity by simply appending `–debug` or `-d` when invoking the command.
Testing Commands Interactively
For more complex debugging, consider testing commands interactively. This can be achieved by creating mock inputs and simulating command execution. Here’s a simple example of how to set up tests for a Cobra command:
“`go
func TestCommandExecution(t *testing.T) {
cmd := &cobra.Command{Use: “test”}
cmd.SetArgs([]string{“–flag”, “value”})
err := cmd.Execute()
if err != nil {
t.Fatalf(“Command execution failed: %v”, err)
}
}
“`
This approach allows you to verify that your commands behave as expected under various conditions.
Common Debugging Techniques
When debugging Cobra applications, consider the following techniques:
- Print Statements: Use `fmt.Println` to output variable states at critical points in your commands.
- Error Handling: Always check for errors after command execution and log them appropriately.
- Unit Tests: Write unit tests for your commands to ensure functionality remains intact after changes.
Technique | Description |
---|---|
Print Statements | Insert print statements to track variable values. |
Error Handling | Check and log errors after executing commands. |
Unit Tests | Automate testing of commands to catch issues early. |
By applying these strategies, you can streamline the debugging process in your Cobra applications, making it easier to identify and resolve issues efficiently.
Understanding Cobra Debugging Techniques
Debugging Cobra applications in Go requires familiarity with both the Cobra library and general debugging techniques in Go. Here are some effective strategies to troubleshoot issues within your Cobra commands:
Setting Up Debugging Environment
To effectively debug your Cobra application, ensure your development environment is set up correctly:
- Install Go Debugger: Use tools like `Delve`, which is a powerful debugger for the Go programming language.
- Integrated Development Environment (IDE): Utilize an IDE like GoLand or Visual Studio Code, which has built-in support for debugging Go applications.
- Cobra Version: Ensure you’re using the latest version of Cobra to take advantage of improvements and bug fixes.
Using Print Statements
A straightforward yet effective debugging approach is to use print statements. This can help you trace the flow of execution and examine variable values:
- Insert `fmt.Println()` statements at critical points in your command’s execution flow.
- Log the values of command-line arguments and flags.
- Output error messages where applicable.
Example:
“`go
cmd.Flags().StringVarP(&myFlag, “myflag”, “m”, “”, “Description of my flag”)
fmt.Println(“Flag value:”, myFlag)
“`
Utilizing Debugging Tools
Integrating debugging tools can provide deeper insights into your application:
- Delve: This tool allows you to set breakpoints, inspect variables, and step through your code.
To install Delve:
“`bash
go install github.com/go-delve/delve/cmd/dlv@latest
“`
- Running Delve:
“`bash
dlv debug path/to/your/app
“`
- Set breakpoints:
“`go
b main.main
“`
- Start debugging:
“`go
run
“`
Examining Command Structure
Inspect the command structure for potential issues:
- Verify that each command is registered properly.
- Check for command dependencies and their configurations.
- Ensure that flags are defined correctly and match the expected types.
Handling Errors Gracefully
Incorporating error handling helps identify issues during command execution:
- Use `errors.Wrap()` from the `github.com/pkg/errors` package for better error context.
- Log errors with context to understand where they occur.
Example:
“`go
if err := cmd.Execute(); err != nil {
log.Fatalf(“Command execution failed: %v”, err)
}
“`
Testing Cobra Commands
Unit tests can be invaluable in identifying issues early:
- Write tests for each Cobra command using Go’s testing framework.
- Utilize test flags to simulate command-line inputs.
Example of a simple test:
“`go
func TestMyCommand(t *testing.T) {
cmd := NewMyCommand()
cmd.SetArgs([]string{“–myflag”, “test”})
err := cmd.Execute()
if err != nil {
t.Errorf(“Command execution failed: %v”, err)
}
}
“`
Visualizing Command Flow
Visualizing the command flow can help identify logical errors:
- Use flowcharts or sequence diagrams to map out command execution paths.
- Identify potential dead ends or unhandled cases in the command flow.
Leveraging Community Resources
Engage with the Go and Cobra community for support:
- GitHub Issues: Check for similar issues or raise new ones.
- Forums/Discussion Groups: Participate in discussions on platforms like Reddit or Stack Overflow.
By employing these techniques, you can effectively debug your Cobra applications in Go, leading to more robust command-line tools.
Expert Insights on Debugging Cobra in Golang
Dr. Emily Carter (Senior Software Engineer, GoLang Innovations). “When debugging Cobra applications in Go, it is essential to leverage the built-in logging capabilities. Utilizing the `log` package effectively can provide insights into command execution flow and error handling, which is crucial for identifying issues.”
Michael Zhang (Lead Developer, CodeCraft Solutions). “I recommend using the `–verbose` flag when running Cobra commands. This will give you a detailed output of the command execution process, making it easier to track down where things might be going wrong.”
Sarah Thompson (Technical Consultant, DevOps Insights). “Integrating a debugger like Delve can significantly enhance your debugging process with Cobra. It allows you to set breakpoints and inspect variables at runtime, which is invaluable for troubleshooting complex command interactions.”
Frequently Asked Questions (FAQs)
How can I enable debug logging in a Cobra application?
To enable debug logging in a Cobra application, you can use the built-in logging package to set the log level to debug. This can be done by configuring the logger at the start of your main function, allowing you to capture detailed output during execution.
What tools can I use to debug Cobra commands in Go?
You can use Go’s built-in debugging tools such as Delve, which allows you to set breakpoints, inspect variables, and step through your code. Additionally, using logging statements throughout your Cobra commands can help trace execution flow and identify issues.
How do I handle errors in Cobra commands effectively?
To handle errors effectively in Cobra commands, always check for errors returned by functions and use the `cmd.PrintErrln()` method to output error messages. This ensures that users receive clear feedback when something goes wrong.
Can I test Cobra commands without running the entire application?
Yes, you can test Cobra commands in isolation by invoking the command’s `Execute()` method directly in your test cases. This allows you to simulate different inputs and verify the command’s behavior without starting the entire application.
What are common pitfalls when debugging Cobra applications?
Common pitfalls include not properly initializing flags, neglecting to check for required flags, and failing to handle command errors appropriately. Additionally, overlooking the command hierarchy can lead to confusion when debugging nested commands.
How can I improve the readability of my Cobra command code during debugging?
To improve readability, structure your Cobra command code by separating logic into smaller functions, use descriptive variable names, and include comments to explain complex sections. This makes it easier to follow the flow of execution during debugging.
Debugging Cobra in Golang involves a systematic approach to identify and resolve issues within command-line applications built using the Cobra library. Key strategies include utilizing logging to trace execution flow, employing the built-in debugging tools of the Go programming language, and leveraging the Cobra framework’s features to inspect command behavior. By understanding the structure of commands and flags, developers can effectively isolate problems and enhance their applications’ reliability.
One of the most effective methods for debugging is to incorporate verbose logging throughout the application. This allows developers to capture the state of the application at various points, making it easier to track down where things may be going wrong. Additionally, using Go’s built-in debugging tools, such as the `delve` debugger, provides a powerful means to step through code execution, inspect variables, and evaluate expressions in real-time.
Another valuable insight is the importance of writing comprehensive tests for Cobra commands. Unit tests can help ensure that each command behaves as expected under different conditions. This proactive approach not only aids in debugging but also contributes to the overall quality and maintainability of the codebase. Furthermore, understanding the command lifecycle and how Cobra handles flags and arguments can significantly improve the debugging process.
Author Profile
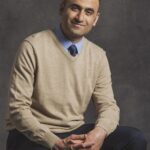
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?