How to Do It in Python: Your Essential Guide to Getting Started!
In the world of programming, mastering the art of logical operations is essential for creating efficient and effective code. Among these operations, the “or” operator stands out as a powerful tool that allows developers to combine conditions and make decisions based on multiple criteria. Whether you’re a seasoned coder or just starting your journey in Python, understanding how to utilize the “or” operator can significantly enhance your programming skills. In this article, we will delve into the nuances of using “or” in Python, exploring its syntax, practical applications, and the scenarios where it shines.
The “or” operator in Python serves as a fundamental component of conditional statements, enabling programmers to evaluate multiple expressions simultaneously. By leveraging this operator, you can create more flexible and dynamic code that responds intelligently to varying inputs. This functionality is particularly useful in situations where you need to check for multiple conditions before executing a block of code, allowing for cleaner and more readable logic.
As we explore the intricacies of the “or” operator, we will cover its role in Boolean logic, how it interacts with other operators, and common pitfalls to avoid. By the end of this article, you will have a solid understanding of how to effectively implement “or” in your Python projects, empowering you to write code
Using the `or` Operator in Python
The `or` operator in Python is a logical operator used to combine conditional statements. It evaluates two conditions and returns `True` if at least one of the conditions is true. If both conditions are , it returns “. This operator is particularly useful when implementing control flow in your programs.
Syntax of the `or` Operator
The syntax for using the `or` operator is straightforward:
“`python
condition1 or condition2
“`
Example Usage
Consider the following example where we check if a number is either positive or zero:
“`python
number = 5
if number >= 0 or number < 10: print("The number is either positive or less than 10.") ``` In this case, the output will confirm that at least one of the conditions is satisfied. Truth Table for the `or` Operator Understanding how the `or` operator evaluates conditions can be simplified through a truth table:
Condition 1 | Condition 2 | Result (Condition 1 or Condition 2) |
---|---|---|
True | True | True |
True | True | |
True | True | |
Practical Examples
The `or` operator can be utilized in various scenarios:
- User Input Validation: Checking if an input falls within acceptable ranges.
- Feature Flags: Enabling features based on various conditions.
- Access Control: Determining user permissions based on multiple conditions.
Here’s an example that checks user permissions:
“`python
is_admin =
is_moderator = True
if is_admin or is_moderator:
print(“Access granted.”)
else:
print(“Access denied.”)
“`
In this case, access is granted because one of the conditions (being a moderator) is true.
Short-Circuit Evaluation
Python’s `or` operator employs short-circuit evaluation. This means that if the first condition evaluates to `True`, Python does not evaluate the second condition, as the overall expression will already be true. This behavior can improve performance in certain situations.
Conclusion
The `or` operator is a powerful tool in Python that allows for flexible and efficient conditional checks. Understanding how to use it effectively can enhance your control flow and decision-making in programming.
Understanding Logical Operators in Python
In Python, the logical operator `or` is used to combine conditional statements. It evaluates conditions from left to right and returns `True` if at least one of the conditions is `True`. If both conditions are “, it returns “.
Basic Syntax of `or` Operator
The syntax for using the `or` operator is straightforward:
“`python
condition1 or condition2
“`
If `condition1` evaluates to `True`, Python will not evaluate `condition2`, optimizing performance through short-circuit evaluation.
Examples of Using `or` Operator
Here are a few examples illustrating how to use the `or` operator effectively:
“`python
Example 1
x = 10
y = 20
if x > 15 or y > 15:
print(“At least one condition is true.”)
Example 2
age = 17
if age < 18 or age > 65:
print(“Eligible for discount.”)
“`
In the first example, the output will be “At least one condition is true” because `y > 15` evaluates to `True`. In the second example, the condition checks if `age` falls under specific criteria for a discount.
Combining `or` with Other Logical Operators
The `or` operator can be combined with other logical operators like `and` and `not`. The order of evaluation follows standard precedence rules:
Operator | Description |
---|---|
`not` | Negates a condition |
`and` | Returns `True` if both conditions are `True` |
`or` | Returns `True` if at least one condition is `True` |
“`python
Combined Example
is_logged_in = True
has_permission =
if is_logged_in or not has_permission:
print(“Access granted.”)
“`
In this example, access is granted because `is_logged_in` is `True`, demonstrating how `or` interacts with `not`.
Using `or` with Boolean Values
The `or` operator is often used directly with Boolean values, which simplifies condition checks:
“`python
a = True
b =
result = a or b
print(result) Output: True
“`
This example shows that if either value is `True`, the result will be `True`.
Common Use Cases
The `or` operator is widely used in various scenarios:
- Input validation: Checking if user input meets certain criteria.
- Feature toggles: Enabling features based on multiple conditions.
- Default values: Providing fallback options.
“`python
Input validation example
username = input(“Enter your username: “)
if username == “” or username is None:
print(“Username cannot be empty.”)
“`
Best Practices
When using the `or` operator in Python, consider the following best practices:
- Clarity: Ensure conditions are clear and easy to understand.
- Short-circuit evaluation: Be mindful of performance implications when using complex conditions.
- Parentheses for clarity: Use parentheses to group conditions, enhancing readability.
“`python
Clear and readable condition
if (age < 18 or age > 65) and not is_logged_in:
print(“Access denied.”)
“`
By adhering to these guidelines, you can write more efficient and understandable code that leverages the `or` operator effectively.
Expert Insights on Performing Operations in Python
Dr. Emily Chen (Senior Python Developer, Tech Innovations Inc.). “When working with Python, utilizing built-in functions such as `map`, `filter`, and list comprehensions can significantly enhance code efficiency and readability. These tools allow for concise operations on collections without the need for verbose loops.”
Michael Thompson (Data Scientist, Analytics World). “For data manipulation in Python, leveraging libraries like Pandas is essential. Operations such as merging, grouping, and aggregating data can be performed seamlessly, making it easier to handle large datasets and perform complex analyses.”
Sarah Patel (Software Engineer, CodeCrafters). “Understanding the principles of object-oriented programming in Python is crucial for performing operations effectively. By defining classes and methods, developers can create reusable code structures that simplify complex tasks and enhance maintainability.”
Frequently Asked Questions (FAQs)
How to do file handling in Python?
File handling in Python can be accomplished using built-in functions such as `open()`, `read()`, `write()`, and `close()`. You can open a file in various modes (e.g., read, write, append) and perform operations on the file content accordingly.
How to do web scraping in Python?
Web scraping in Python can be performed using libraries such as Beautiful Soup and Scrapy. These libraries allow you to parse HTML and XML documents, extract data from web pages, and automate the data collection process.
How to do data visualization in Python?
Data visualization in Python can be achieved using libraries like Matplotlib, Seaborn, and Plotly. These libraries provide functions to create a wide range of static, animated, and interactive visualizations to represent data effectively.
How to do unit testing in Python?
Unit testing in Python can be implemented using the built-in `unittest` framework. You can create test cases by subclassing `unittest.TestCase`, define test methods, and use assertions to verify that your code behaves as expected.
How to do object-oriented programming in Python?
Object-oriented programming in Python involves defining classes and creating objects. You can encapsulate data and functions within classes, utilize inheritance to create subclasses, and implement polymorphism to allow methods to use objects of different classes interchangeably.
How to do database operations in Python?
Database operations in Python can be conducted using libraries such as SQLite3 for SQLite databases or SQLAlchemy for various database systems. These libraries enable you to connect to databases, execute SQL queries, and manage data efficiently.
In summary, the use of the logical operator “or” in Python plays a crucial role in controlling the flow of programs through conditional statements. The “or” operator allows for the evaluation of multiple conditions, returning True if at least one of the conditions is met. This functionality is essential for implementing decision-making processes in code, enabling developers to create more dynamic and responsive applications.
Furthermore, understanding the nuances of how “or” interacts with other logical operators, such as “and” and “not,” is vital for writing efficient Python code. The precedence of these operators can affect the outcome of complex logical expressions, making it important for programmers to be mindful of the order in which conditions are evaluated. Proper use of parentheses can help clarify the intended logic, ensuring that the code behaves as expected.
Key takeaways include the importance of mastering logical operators to enhance programming skills and the necessity of thorough testing to validate that conditional statements work as intended. Additionally, leveraging the “or” operator effectively can lead to cleaner, more readable code, which is an essential aspect of professional software development. By incorporating these practices, developers can improve both the functionality and maintainability of their Python applications.
Author Profile
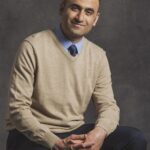
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?