How Can You Effectively End a Program in Python?
In the world of programming, knowing how to effectively manage the lifecycle of your applications is crucial. Whether you’re developing a simple script or a complex software system, understanding how to end a program in Python is an essential skill that can enhance your coding efficiency and improve your debugging process. As you dive into Python, you’ll discover that gracefully terminating your programs not only helps maintain system resources but also contributes to a more polished user experience.
Ending a Python program can be straightforward, but there are several methods and best practices to consider. From using built-in functions to handling exceptions and signals, the approach you choose can depend on the context of your application. For instance, you might want to terminate a program based on user input or as a response to an error, each requiring a different technique. Moreover, understanding how to manage program termination can help you avoid common pitfalls, such as leaving resources open or failing to save user data.
As we explore the various ways to end a Python program, you’ll gain insights into the importance of proper termination. This knowledge will not only empower you to write cleaner code but also enhance your overall programming capabilities. So, whether you’re a beginner looking to solidify your foundation or an experienced coder seeking to refine your skills, mastering the art of program termination in Python is
Using `exit()` or `sys.exit()`
One of the most straightforward methods to terminate a Python program is by using the `exit()` function from the built-in library or `sys.exit()` from the `sys` module. Both functions effectively end the program execution, but there are subtle differences in their usage.
- exit(): This function is primarily intended for use in interactive sessions. It raises a `SystemExit` exception, which can be caught if needed.
- sys.exit(): This method is more commonly used in scripts and applications. It allows the passing of an exit status code to the operating system, where `0` typically indicates success, while any non-zero value indicates an error.
Here’s how to implement these methods:
python
# Using exit()
exit()
# Using sys.exit()
import sys
sys.exit(0)
Using `os._exit()`
In some cases, you might need to terminate a program without raising an exception. The `os._exit()` function does this by terminating the process immediately without cleanup. This is particularly useful in multi-threaded applications where you want to exit without executing the cleanup handlers.
Example usage:
python
import os
# Exiting immediately without cleanup
os._exit(0)
Handling Exceptions to End Programs
You can gracefully end a program by handling exceptions. This allows you to clean up resources or display error messages before exiting. Use a try-except block to catch exceptions and call `sys.exit()`.
Example:
python
import sys
try:
# Your code that might raise an exception
risky_operation()
except Exception as e:
print(f”An error occurred: {e}”)
sys.exit(1) # Exit with an error code
Using `KeyboardInterrupt`
When running a Python script from the command line, you can interrupt its execution by sending a `KeyboardInterrupt` signal (usually via Ctrl+C). This can be captured in your script to perform cleanup actions before terminating.
Example:
python
try:
while True:
pass # Your long-running operation
except KeyboardInterrupt:
print(“Program terminated by user.”)
sys.exit(0)
Exit Codes
When ending a Python program, it is a good practice to return an exit code that indicates the outcome of the program’s execution. Below is a table illustrating common exit codes:
Exit Code | Description |
---|---|
0 | Success |
1 | General error |
2 | Misuse of shell builtins |
126 | Command invoked cannot execute |
127 | Command not found |
130 | Script terminated by Ctrl+C |
By using appropriate exit codes, you provide valuable information about the program’s execution outcome, which can be especially useful when the script is part of a larger automated process.
Using the `exit()` Function
The `exit()` function is part of the `sys` module and is commonly used to terminate a Python program. It allows you to specify an exit status, which can be useful for indicating success or failure to the operating system.
python
import sys
# Terminating the program with a success status
sys.exit(0)
# Terminating the program with an error status
sys.exit(1)
- `0`: Indicates successful termination.
- Non-zero values: Indicate an error or abnormal termination.
Using the `quit()` Function
The `quit()` function serves a similar purpose as `exit()`. It is often used in interactive sessions and scripts to terminate the program cleanly.
python
quit()
While `quit()` is user-friendly, it is recommended to use `exit()` in production code for better clarity.
Using the `break` Statement in Loops
To exit a loop prematurely in Python, the `break` statement can be employed. This is particularly useful when you need to stop processing based on a specific condition.
python
for i in range(10):
if i == 5:
break # Exits the loop when i is 5
print(i)
- The loop terminates when the condition is met, allowing for controlled exits.
Using the `return` Statement in Functions
Inside a function, you can use the `return` statement to exit the function and optionally return a value. If the function is called in the main program, it will effectively end that part of the program.
python
def my_function():
return “Exiting function”
result = my_function()
print(result)
- This statement can also be used to exit from nested functions or to handle exceptions.
Handling Exceptions with `raise`
The `raise` statement can be used to trigger exceptions that may terminate a program. This is particularly useful for error handling.
python
def check_value(x):
if x < 0:
raise ValueError("Negative value encountered")
try:
check_value(-1)
except ValueError as e:
print(e)
- This approach allows for graceful termination when unexpected conditions arise.
Using Signals to Terminate Processes
In more advanced scenarios, you may want to terminate a program using signals. This is particularly relevant when dealing with multi-threaded applications or processes.
python
import signal
import os
# Function to handle the termination
def handler(signum, frame):
print(“Program terminated”)
sys.exit(0)
# Register the signal handler
signal.signal(signal.SIGINT, handler)
# Keep the program running
while True:
pass
- This code snippet demonstrates how to handle a termination signal like `SIGINT` (usually triggered by Ctrl+C).
Graceful Shutdown with Context Managers
Using context managers can help ensure that resources are released properly when a program ends. By defining a `__exit__` method, you can manage cleanup actions.
python
class MyContext:
def __enter__(self):
print(“Entering context”)
def __exit__(self, exc_type, exc_value, traceback):
print(“Exiting context”)
with MyContext():
print(“Inside the context”)
- This provides a clean and controlled way to end a program.
Using the `os._exit()` Function
The `os._exit()` function can be used for an immediate termination of a program, bypassing cleanup handlers. This is generally used in child processes.
python
import os
os._exit(0) # Terminates immediately
- This should be used with caution, as it does not allow for any cleanup actions.
Expert Insights on Terminating Python Programs
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To end a Python program effectively, one can utilize the built-in `sys.exit()` function, which allows for a graceful termination of the program. This method is particularly useful for closing applications that may need to return an exit status.”
James Liu (Lead Python Developer, CodeCraft Solutions). “In scenarios where a program needs to terminate due to an error or unexpected condition, employing exception handling with `try` and `except` blocks can provide a controlled exit point. This ensures that resources are properly released before the program ends.”
Sarah Thompson (Python Instructor, Advanced Coding Academy). “For beginners, understanding the use of the `exit()` function from the `os` module can be beneficial. It offers a straightforward way to terminate a script while allowing for optional exit codes, which can be useful for debugging and process management.”
Frequently Asked Questions (FAQs)
How can I terminate a Python program gracefully?
You can terminate a Python program gracefully by using the `sys.exit()` function from the `sys` module. This allows you to exit the program and optionally return an exit status.
What is the purpose of the `exit()` function in Python?
The `exit()` function is a built-in function that raises the `SystemExit` exception, which terminates the program. It can be used in scripts and interactive sessions to exit without an error.
Can I stop a Python program using keyboard shortcuts?
Yes, you can stop a running Python program in the terminal or command prompt by pressing `Ctrl + C`. This sends a keyboard interrupt signal to the program.
What happens if I use `quit()` in a Python script?
The `quit()` function behaves similarly to `exit()`, raising a `SystemExit` exception. It is intended for use in interactive sessions and is generally not recommended for scripts.
Is there a way to terminate a Python program from within a loop?
Yes, you can use a conditional statement within the loop to call `sys.exit()` or `break` to exit the loop and subsequently terminate the program.
How can I handle exceptions before ending a Python program?
You can use a `try-except` block to catch exceptions and handle them appropriately before calling `sys.exit()` or another termination method. This ensures that resources are released and cleanup is performed.
In Python, ending a program can be accomplished through various methods depending on the context and requirements of the application. The most straightforward way to terminate a program is by using the built-in function `exit()`, `quit()`, or `sys.exit()`. Each of these functions serves the purpose of stopping the execution of the script, but they may have different implications in terms of cleanup and exit status.
Another approach to ending a Python program is by allowing it to reach the end of the script naturally. When the interpreter processes the last line of code, it will automatically terminate the program. Additionally, handling exceptions with try-except blocks can provide a graceful exit when errors occur, ensuring that resources are properly released and any necessary cleanup is performed.
It is important to consider the context in which the program is running. For instance, in interactive environments or applications with a graphical user interface, the termination process may involve more complex considerations, such as closing windows or saving user data. Understanding these nuances can lead to a more polished and user-friendly application.
In summary, Python offers several methods to end a program effectively. The choice of method should align with the specific needs of the application, ensuring a clean and efficient termination process
Author Profile
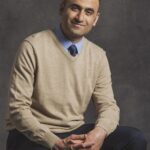
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?