How Can You Effectively End a Program in Python?
In the world of programming, knowing how to effectively manage your code is just as crucial as writing it. One common yet essential task every programmer encounters is the need to end a program gracefully. Whether you’re developing a simple script or a complex application, understanding the various methods to terminate your Python program can save you from unexpected behavior and potential data loss. This article will guide you through the different techniques available in Python for ending your program, ensuring that you can execute this task with confidence and precision.
When it comes to ending a Python program, there are several approaches you can take, each suited for different scenarios. From using built-in functions to implementing exception handling, the methods available allow for both immediate and controlled termination of your code. Understanding these options is vital, as they can influence how your program interacts with the environment and how it handles resources.
Moreover, the choice of termination method can also affect user experience and program reliability. Whether you need to exit a loop, terminate a script due to an error, or simply end the program after reaching a specific condition, knowing the right techniques will enhance your programming toolkit. In the following sections, we will delve into these methods, providing you with the knowledge to end your Python programs effectively and efficiently.
Using the `exit()` Function
The simplest way to terminate a Python program is by using the `exit()` function from the `sys` module. This function takes an optional argument that indicates the exit status. If no argument is provided, the program exits with a status code of `0`, indicating success.
python
import sys
# Exiting the program
sys.exit() # Successful termination
You can also specify a non-zero exit code to indicate an error or abnormal termination:
python
import sys
# Exiting with an error code
sys.exit(1) # Indicates an error occurred
Using the `quit()` Function
Similar to `exit()`, the `quit()` function is another built-in function that can be used to terminate a Python program. It serves the same purpose but is designed for use in interactive sessions. However, it is generally recommended to use `exit()` in scripts.
python
# Exiting the program
quit() # This will terminate the program
Using the `os._exit()` Function
The `os._exit()` function is a lower-level method that terminates the program immediately without calling cleanup handlers, flushing standard I/O buffers, etc. This function is useful in scenarios where you want to ensure that your program stops without any additional processing.
python
import os
# Forceful termination of the program
os._exit(0) # Exits immediately with a status of 0
Using the `raise SystemExit` Exception
Another approach to exit a program is by raising the `SystemExit` exception. This method allows you to exit the program at any point, and it can also be caught by exception handlers if needed.
python
# Raising SystemExit to terminate
raise SystemExit(“Exiting the program”)
Table of Exit Methods
Method | Description | Exit Status |
---|---|---|
sys.exit() | Exits the program gracefully. | 0 (default), or custom code. |
quit() | Similar to exit(), meant for interactive use. | 0 (default). |
os._exit() | Exits immediately without cleanup. | 0 (or custom code). |
raise SystemExit | Raises an exception to terminate the program. | Custom message or code. |
When deciding how to end a program in Python, consider the context and whether you need to perform cleanup operations. Each method has its specific use case, and understanding these can improve the robustness and predictability of your code.
Methods to End a Program in Python
In Python, there are several ways to terminate a program, each suited to different scenarios. The most common methods include using built-in functions, handling exceptions, or leveraging external libraries.
Using the `exit()` Function
The `exit()` function from the `sys` module is a straightforward way to end a Python program. This function can be called with an optional exit status code.
python
import sys
sys.exit() # Exits the program
sys.exit(0) # Exits with a status code of 0 (success)
sys.exit(1) # Exits with a status code of 1 (error)
- Status Codes:
- `0` indicates success.
- Non-zero values indicate different types of errors.
Using the `quit()` Function
Similar to `exit()`, the `quit()` function can also terminate the program. It is often used in interactive sessions.
python
quit() # Terminates the program
- Note: `quit()` is essentially an alias for `exit()` and should be used in the same manner.
Using the `raise SystemExit` Exception
The `SystemExit` exception can be raised directly to end a program. This method is useful when you want to perform cleanup operations before exiting.
python
raise SystemExit(“Exiting the program.”)
- Custom Messages: You can pass a message or status code to `SystemExit`, which can be useful for debugging.
Using `os._exit()` for Immediate Termination
The `os._exit()` function is a low-level method that terminates the program immediately without cleanup. This function is generally not recommended for use in normal circumstances.
python
import os
os._exit(0) # Terminates the program without cleanup
- Use Cases: This can be useful in multi-threaded applications where immediate termination of the process is required.
Handling Exceptions to Exit Gracefully
In many cases, you might want to end a program due to an error condition. Using exception handling allows for graceful termination.
python
try:
# Code that may raise an exception
risky_operation()
except Exception as e:
print(f”An error occurred: {e}”)
sys.exit(1) # Exits with an error status
- Best Practices: Always log the error or clean up resources before exiting to avoid data loss or corruption.
Using `KeyboardInterrupt` to Exit Programs
When running a program in an interactive environment, pressing `Ctrl+C` raises a `KeyboardInterrupt` exception, which can be caught to perform cleanup before exiting.
python
try:
while True:
pass # Simulating a long-running process
except KeyboardInterrupt:
print(“Program was interrupted. Exiting…”)
sys.exit(0)
- User Control: This method gives users the ability to terminate the program cleanly.
Summary of Methods
Method | Description | Use Cases |
---|---|---|
`sys.exit()` | Standard way to exit program with optional status | General use |
`quit()` | Similar to `exit()`, mainly for interactive sessions | Interactive environments |
`raise SystemExit` | Raise an exception to exit, allowing cleanup | When needing to handle cleanup |
`os._exit()` | Immediate termination without cleanup | Multi-threaded applications |
Exception Handling | Graceful exit on errors | Handling errors during execution |
`KeyboardInterrupt` | Catch user interruption for clean exit | User-initiated termination |
These methods provide flexibility in managing program termination based on specific requirements and contexts.
Effective Methods to Terminate a Python Program
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “To gracefully end a program in Python, one can use the built-in function `sys.exit()`. This method allows you to specify an exit status, which is particularly useful for signaling success or failure to the operating system.”
Michael Chen (Lead Python Instructor, Code Academy). “In interactive environments, such as Jupyter notebooks, using the command `exit()` or `quit()` is a straightforward way to terminate the program. These commands are user-friendly and provide a clear indication that the session has ended.”
Linda Patel (Software Architect, Tech Innovations Inc.). “For more complex applications, especially those involving threads or subprocesses, it is crucial to implement proper exception handling and cleanup routines before calling `sys.exit()`. This ensures that all resources are released appropriately, preventing potential memory leaks.”
Frequently Asked Questions (FAQs)
How can I terminate a Python program using the exit command?
You can terminate a Python program by using the `exit()` function, which raises the `SystemExit` exception, effectively stopping the program.
What is the purpose of the sys.exit() function in Python?
The `sys.exit()` function is used to exit from Python. It can take an optional argument that can be an integer or a string, indicating the exit status or message.
Can I stop a Python program with a keyboard interrupt?
Yes, you can stop a Python program by pressing `Ctrl + C` in the terminal, which raises a `KeyboardInterrupt` exception, terminating the program.
What happens if I use the quit() function in Python?
The `quit()` function behaves similarly to `exit()`, terminating the program and raising the `SystemExit` exception. It is often used in interactive sessions.
Is there a way to exit a Python script based on a condition?
Yes, you can use conditional statements along with `exit()`, `sys.exit()`, or `quit()` to exit the script based on specific conditions being met.
How do I ensure a clean exit from a Python program?
To ensure a clean exit, you can use a `try…finally` block, placing cleanup code in the `finally` section, which guarantees execution before the program exits.
In Python, there are several methods to end a program gracefully, each suited to different scenarios. The most common approach is to use the `exit()` function from the `sys` module, which allows for an immediate termination of the program. Another method is to raise the `SystemExit` exception, which can also be caught in a try-except block if necessary. Additionally, the `quit()` and `exit()` functions, although not recommended for production code, provide user-friendly ways to terminate scripts during interactive sessions.
It is essential to consider the context in which the program is being terminated. For instance, if the program is running in an interactive environment, using `exit()` or `quit()` may be more appropriate. Conversely, in a script meant for production, a clean exit using `sys.exit()` or handling exceptions ensures that any necessary cleanup operations are performed before termination. Understanding these nuances is crucial for writing robust Python applications.
effectively ending a Python program involves selecting the appropriate method based on the program’s context and requirements. Whether using `sys.exit()`, raising `SystemExit`, or employing interactive commands like `quit()`, developers should prioritize clarity and maintainability in their code. By doing so, they
Author Profile
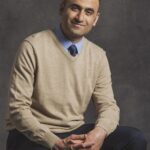
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?