How Can You Gracefully Exit a Python Program?
### Introduction
In the world of programming, knowing how to effectively manage the lifecycle of your applications is crucial for creating robust and user-friendly software. One fundamental aspect of this is understanding how to exit a Python program gracefully. Whether you’re developing a simple script or a complex application, there will come a time when you’ll need to terminate your program, either due to a completed task or an unexpected error. Mastering this skill not only enhances your coding efficiency but also improves the overall user experience.
Exiting a Python program might seem straightforward, but it involves more than just hitting the stop button. There are various methods to achieve this, each with its own implications and best use cases. From using built-in functions to handling exceptions, the way you choose to exit your program can affect how resources are managed and how user data is preserved. Understanding these methods will empower you to make informed decisions in your coding practices.
As we delve deeper into the topic, we will explore the different techniques available for exiting a Python program, the scenarios in which each method is most appropriate, and best practices to ensure a clean exit. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to handle program termination effectively. Get ready to enhance your Python programming skills and take control of your
Using the exit() Function
The `exit()` function is one of the simplest ways to terminate a Python program. This function is provided by the `sys` module, and it can be called without any arguments, which will exit the program with a default status code of zero. A non-zero value can be passed to indicate an error or abnormal termination.
- Example usage:
python
import sys
# Exit the program normally
sys.exit()
# Exit the program with an error status
sys.exit(1)
Using the quit() and exit() Functions
In addition to `sys.exit()`, Python provides two built-in functions: `quit()` and `exit()`. Both of these functions are essentially aliases for `sys.exit()` and behave in the same way. However, they are intended for use in the interactive interpreter and are not recommended for use in scripts.
- Example usage:
python
# This will terminate the program
quit()
Using raise SystemExit
Another method to exit a Python program is by raising the `SystemExit` exception. This approach provides a more explicit way of terminating the program and can be useful in larger applications where exceptions are managed more rigorously.
- Example usage:
python
raise SystemExit
Using os._exit() for Immediate Termination
If you require an immediate termination of the program, bypassing the cleanup process, the `os._exit()` function can be used. This function is a lower-level call and does not clean up resources such as flushing standard I/O buffers. It is typically used in multi-threaded programs where you want to stop a specific thread without affecting others.
- Example usage:
python
import os
os._exit(0) # Immediate exit with a success status
Exit Codes
When exiting a program, it is often useful to return an exit code that indicates the status of the program’s execution. By convention, an exit code of `0` indicates success, while any non-zero value indicates an error.
Exit Code | Description |
---|---|
0 | Success |
1 | General error |
2 | Misuse of shell builtins (according to Bash documentation) |
126 | Command invoked cannot execute |
127 | Command not found |
130 | Script terminated by Control-C |
Exiting Methods
Understanding the various methods for exiting a Python program allows developers to choose the most appropriate approach based on the context of their application. Whether through exceptions, built-in functions, or immediate termination, Python offers flexibility in handling program exits.
Methods to Exit a Python Program
Exiting a Python program can be achieved using several methods, each suitable for different scenarios. Understanding these methods will enhance control over program execution and ensure a clean exit.
Using the `exit()` Function
The `exit()` function, provided by the `sys` module, is a common way to terminate a program. It can be utilized in scripts where immediate termination is required.
python
import sys
sys.exit()
- Parameters:
- The optional argument allows you to specify an exit status code.
- A status code of `0` indicates a successful termination, while any non-zero value indicates an error.
Using the `quit()` Function
Similar to `exit()`, the `quit()` function is another built-in command that can be used to stop program execution.
python
quit()
- Usage:
- Primarily used in interactive sessions, but can also be used in scripts.
- Functionally similar to `exit()`.
Using the `os._exit()` Function
The `os` module provides the `_exit()` function, which can be used for terminating a program abruptly without cleanup.
python
import os
os._exit(0)
- Differences:
- Does not call cleanup handlers, flush stdio buffers, or execute `atexit` handlers, making it suitable for emergency exits in child processes.
Raising the `SystemExit` Exception
A more programmatic way to exit is by raising the `SystemExit` exception. This method allows for the handling of exit conditions within try-except blocks.
python
raise SystemExit
- Handling:
- Can be caught in an exception block for cleanup before exiting.
Using Keyboard Interrupts
In a running program, you can interrupt execution using a keyboard command (usually Ctrl+C). This generates a `KeyboardInterrupt` exception, which can be caught and handled gracefully.
python
try:
# Program logic here
except KeyboardInterrupt:
print(“Program terminated by user.”)
- Behavior:
- Allows for a clean exit with the possibility of executing cleanup code.
Exiting from a Loop or Function
In scenarios where you want to exit from a loop or a specific function instead of the entire program, you can use the `break` statement or return from the function.
python
for i in range(10):
if i == 5:
break # Exits the loop
python
def my_function():
return # Exits the function
- Use Cases:
- Useful for controlling flow without terminating the entire program.
Exit Codes and Status
When exiting a program, understanding exit codes is crucial for signaling the success or failure of execution to the operating system or parent processes. Below is a summary of commonly used exit codes:
Exit Code | Meaning |
---|---|
0 | Successful termination |
1 | General error |
2 | Misuse of shell builtins |
126 | Command invoked cannot execute |
127 | Command not found |
130 | Script terminated by Control-C |
Utilizing the appropriate exit method and status code will improve program robustness and clarity in handling termination.
Expert Insights on Exiting a Python Program
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Exiting a Python program can be efficiently handled using the `sys.exit()` function, which allows for a clean termination of the script. This method is particularly useful when you need to exit from deep within nested function calls.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “In addition to `sys.exit()`, using the `exit()` function is a straightforward way to terminate a Python program. However, it’s essential to remember that `exit()` is intended for interactive use and may not be suitable for production code.”
Linda Garcia (Python Instructor, Advanced Coding Academy). “For graceful exits, especially in larger applications, consider implementing exception handling. By raising a custom exception, you can control the exit process and ensure that necessary cleanup operations are performed before the program terminates.”
Frequently Asked Questions (FAQs)
How can I exit a Python program using the exit() function?
You can exit a Python program by calling the `exit()` function, which raises a `SystemExit` exception. This can be done by simply including `exit()` in your code.
Is there a difference between exit() and sys.exit() in Python?
Yes, `exit()` is a convenience function provided by the built-in Python module, while `sys.exit()` is part of the `sys` module. `sys.exit()` is generally preferred in scripts for more explicit control over exit status.
Can I use the quit() function to exit a Python program?
Yes, `quit()` is similar to `exit()` and can be used to terminate a Python program. However, it is primarily intended for use in interactive sessions.
What happens if I use return in the main function of a Python script?
Using `return` in the main function will exit the function and, if the function is the main entry point, it will effectively terminate the program. However, it is less common than using `exit()` or `sys.exit()`.
How do I exit a Python program with a specific exit code?
You can exit a Python program with a specific exit code by using `sys.exit(code)`, where `code` is an integer representing the exit status. A code of zero typically indicates success, while any non-zero code indicates an error.
Are there any exceptions to consider when exiting a Python program?
Yes, when using `exit()` or `sys.exit()`, any `try` blocks that are still executing will be exited, and any cleanup code in `finally` blocks will run. Be cautious of leaving resources unclosed or incomplete operations.
Exiting a Python program can be accomplished through several methods, each suited to different scenarios. The most common way to terminate a program is by using the built-in `exit()` function or `sys.exit()` from the `sys` module. These functions allow developers to exit the program gracefully, providing an optional exit status that can indicate success or failure. It is important to note that using `exit()` is typically intended for use in the interactive interpreter, while `sys.exit()` is more appropriate for scripts and applications.
Another method to exit a Python program is through the use of exceptions. By raising a `SystemExit` exception, developers can control the exit process, allowing for cleanup operations or the execution of specific code before termination. This approach is particularly useful in larger applications where resource management and error handling are crucial. Additionally, using `os._exit()` can forcefully terminate a program without executing cleanup handlers, which may be necessary in certain low-level scenarios.
In summary, understanding how to exit a Python program effectively is essential for developers. By utilizing the appropriate exit methods, they can ensure that their applications terminate correctly and manage resources efficiently. Whether through standard exit functions, exception handling, or low-level termination, the choice of method
Author Profile
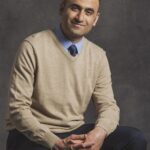
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?