How Can You Easily Calculate the Mean in Python?
When it comes to data analysis and scientific computing, the ability to calculate the mean is a fundamental skill every Python programmer should master. The mean, or average, serves as a critical statistical measure that provides insight into the central tendency of a dataset. Whether you’re a seasoned data scientist or a beginner exploring the world of programming, understanding how to compute the mean in Python can enhance your analytical capabilities and empower you to draw meaningful conclusions from your data.
In Python, calculating the mean can be accomplished through various methods, each catering to different needs and preferences. From utilizing built-in functions to leveraging powerful libraries like NumPy and Pandas, the options are plentiful. Each approach offers unique advantages, whether it’s simplicity for quick calculations or advanced features for handling complex datasets. As you delve into the world of Python, you’ll discover how these tools can streamline your data analysis process and improve your overall efficiency.
Moreover, understanding the mean is just the tip of the iceberg. Once you grasp this concept, you can explore its implications in various fields, from finance to machine learning. With Python at your fingertips, you’ll be equipped to not only calculate the mean but also to interpret its significance in the context of your data. So, let’s embark on this journey to uncover the different ways to find
Using Built-in Functions to Calculate Mean
In Python, one of the simplest ways to calculate the mean of a dataset is by using the built-in `sum()` and `len()` functions. The mean, or average, is calculated by dividing the sum of all elements by the number of elements. Here’s how you can do it:
“`python
data = [10, 20, 30, 40, 50]
mean = sum(data) / len(data)
print(“Mean:”, mean)
“`
This code snippet initializes a list of numbers, computes the sum of the numbers using `sum(data)`, and then divides that sum by the total count of numbers using `len(data)`.
Using NumPy for Mean Calculation
For larger datasets or more complex numerical operations, the NumPy library is highly recommended due to its efficiency and additional functionalities. The `numpy.mean()` function can be used to compute the mean easily.
First, ensure you have NumPy installed:
“`bash
pip install numpy
“`
Then, you can compute the mean as follows:
“`python
import numpy as np
data = np.array([10, 20, 30, 40, 50])
mean = np.mean(data)
print(“Mean:”, mean)
“`
NumPy’s `mean()` function can handle multi-dimensional arrays and offers additional options for specifying the axis along which to compute the mean.
Using Pandas for Mean Calculation
Pandas is another powerful library for data manipulation and analysis. It provides the `DataFrame` object, which can be very useful for handling tabular data. To calculate the mean using Pandas, follow these steps:
First, install Pandas if it is not already installed:
“`bash
pip install pandas
“`
Here’s how to compute the mean using a Pandas DataFrame:
“`python
import pandas as pd
data = {‘values’: [10, 20, 30, 40, 50]}
df = pd.DataFrame(data)
mean = df[‘values’].mean()
print(“Mean:”, mean)
“`
Pandas’ `mean()` function will automatically ignore any NaN values, making it robust for real-world datasets.
Mean Calculation for Different Data Types
When dealing with different data types, such as lists, NumPy arrays, or Pandas DataFrames, the method to calculate the mean remains consistent but varies slightly based on the structure. Below is a comparison table summarizing the methods:
Data Type | Method | Code Example |
---|---|---|
List | sum() / len() | mean = sum(data) / len(data) |
NumPy Array | numpy.mean() | mean = np.mean(data) |
Pandas DataFrame | DataFrame.mean() | mean = df[‘column’].mean() |
Each of these methods offers a straightforward approach to calculating the mean, allowing users to choose based on their specific data structures and libraries in use.
Calculating the Mean Using Python’s Built-in Functions
Python provides several built-in functions and libraries that make it easy to calculate the mean of a dataset. The most common method is to use the built-in `sum()` and `len()` functions.
To compute the mean manually:
“`python
data = [10, 20, 30, 40, 50]
mean = sum(data) / len(data)
print(“Mean:”, mean)
“`
In this example, the `sum()` function calculates the total of the list, while `len()` gives the number of elements. The mean is then calculated by dividing the total by the count of elements.
Using NumPy for Mean Calculation
NumPy is a powerful library in Python that simplifies mathematical computations and is particularly efficient for array and matrix operations. To find the mean using NumPy, follow these steps:
- Install NumPy if it’s not already installed:
“`bash
pip install numpy
“`
- Use NumPy’s `mean()` function:
“`python
import numpy as np
data = np.array([10, 20, 30, 40, 50])
mean = np.mean(data)
print(“Mean:”, mean)
“`
The `mean()` function calculates the mean of the array quickly and efficiently, making it suitable for large datasets.
Calculating Mean with Pandas
Pandas is another powerful library specifically designed for data manipulation and analysis. To compute the mean using Pandas, follow these steps:
- Install Pandas if necessary:
“`bash
pip install pandas
“`
- Use Pandas to calculate the mean:
“`python
import pandas as pd
data = pd.Series([10, 20, 30, 40, 50])
mean = data.mean()
print(“Mean:”, mean)
“`
Pandas automatically handles missing values, making it a robust choice for data analysis.
Mean Calculation for Multi-Dimensional Data
When dealing with multi-dimensional data, both NumPy and Pandas can compute the mean along specific axes.
Using NumPy:
“`python
import numpy as np
data = np.array([[10, 20, 30], [40, 50, 60]])
mean_axis0 = np.mean(data, axis=0) Mean across rows
mean_axis1 = np.mean(data, axis=1) Mean across columns
print(“Mean across rows:”, mean_axis0)
print(“Mean across columns:”, mean_axis1)
“`
Using Pandas:
“`python
import pandas as pd
data = pd.DataFrame([[10, 20, 30], [40, 50, 60]])
mean_columns = data.mean(axis=0) Mean of each column
mean_rows = data.mean(axis=1) Mean of each row
print(“Mean of each column:\n”, mean_columns)
print(“Mean of each row:\n”, mean_rows)
“`
Both methods allow for flexible computation based on the structure of your data.
Considerations When Calculating Mean
When calculating the mean, keep in mind the following:
- Handling Missing Values: Ensure that missing values in your dataset are addressed, as they can skew the results.
- Data Type Compatibility: Confirm that the data types in your dataset are numeric; otherwise, you may encounter errors.
- Outliers: The mean can be significantly affected by outliers. Consider using median or trimmed mean for datasets with extreme values.
By understanding and utilizing these methods, you can effectively calculate the mean in Python across various types of data structures.
Expert Insights on Calculating Mean in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “To find the mean in Python, utilizing the NumPy library is highly efficient. The function `numpy.mean()` can compute the mean of an array or list with minimal code, making it an ideal choice for data analysis tasks.”
Michael Thompson (Senior Software Engineer, CodeCraft Solutions). “For beginners, using the built-in `statistics` module is a straightforward approach. The `statistics.mean()` function is intuitive and provides a clear understanding of how to calculate the mean without the need for additional libraries.”
Sarah Patel (Python Educator, LearnPython Academy). “When working with pandas DataFrames, the `mean()` method is incredibly useful. It allows users to calculate the mean across different axes, which is particularly advantageous for handling large datasets in data science projects.”
Frequently Asked Questions (FAQs)
How do I calculate the mean of a list in Python?
You can calculate the mean of a list in Python using the built-in `sum()` function divided by the length of the list. For example: `mean = sum(my_list) / len(my_list)`.
Which library is best for calculating the mean in Python?
The `numpy` library is widely used for numerical calculations, including finding the mean. You can use `numpy.mean(my_array)` for efficient computation.
Can I find the mean of a DataFrame column in Python?
Yes, you can find the mean of a DataFrame column using the `mean()` method. For example: `df[‘column_name’].mean()` will return the mean of the specified column.
What if my data contains NaN values when calculating the mean?
You can handle NaN values by using the `mean()` method with the parameter `skipna=True`, which is the default behavior. This will ignore NaN values in the calculation.
Is there a way to calculate the mean using Python’s statistics module?
Yes, you can use the `statistics` module. Import it and use `statistics.mean(my_list)` to calculate the mean of a list or other iterable.
How can I visualize the mean of a dataset in Python?
You can visualize the mean using libraries like `matplotlib` or `seaborn`. For example, you can create a bar plot to show the mean value alongside the data distribution.
Finding the mean in Python is a straightforward process that can be accomplished using various methods, depending on the specific requirements of the task at hand. The most common approaches include utilizing built-in functions, leveraging libraries such as NumPy and Pandas, or implementing custom functions. Each method has its advantages, and the choice largely depends on the complexity of the data and the user’s familiarity with these libraries.
Using the built-in `sum()` and `len()` functions is a simple way to calculate the mean for a list of numbers. However, for larger datasets or more complex data structures, libraries like NumPy and Pandas provide optimized functions that not only simplify the calculation but also enhance performance. For instance, NumPy’s `mean()` function can handle multi-dimensional arrays efficiently, while Pandas offers a convenient way to calculate the mean of DataFrame columns.
In summary, Python provides multiple avenues for calculating the mean, catering to both novice and experienced programmers. Understanding the context in which you are working and the structure of your data will guide you in selecting the most appropriate method. By leveraging Python’s capabilities, users can efficiently compute the mean and derive valuable insights from their datasets.
Author Profile
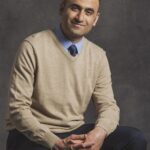
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?