How Can You Easily Retrieve the Current Directory in Python?
When diving into the world of Python programming, understanding your environment is crucial for efficient coding. One fundamental aspect of this environment is the current working directory, which is the folder where your Python script is running. Whether you’re managing files, reading data, or saving outputs, knowing how to access the current directory can streamline your workflow and enhance your productivity. In this article, we’ll explore the various methods to retrieve the current directory in Python, ensuring you have the tools you need to navigate your projects with ease.
The current working directory serves as the default location for file operations in Python. It’s the starting point from which relative paths are resolved, making it essential for tasks such as file reading and writing. Python provides several built-in functions and modules to help you identify this directory, each with its unique advantages. From the simple and straightforward to more advanced techniques, you’ll discover how to effectively manage your file paths and ensure your scripts run smoothly.
As we delve deeper into the topic, we’ll cover the most common methods for obtaining the current directory, highlighting their use cases and best practices. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, understanding how to get the current directory in Python is a valuable addition to your programming toolkit. Get ready
Using the `os` Module
The most common way to retrieve the current working directory in Python is by utilizing the `os` module, which provides a way to interact with the operating system. The function `os.getcwd()` returns the path of the current working directory as a string.
To use this method, follow these simple steps:
“`python
import os
current_directory = os.getcwd()
print(“Current Directory:”, current_directory)
“`
This code snippet imports the `os` module, calls the `getcwd()` function, and prints the current directory to the console.
Using the `pathlib` Module
With the of Python 3.4, the `pathlib` module offers an object-oriented approach to filesystem paths. This module allows for more intuitive manipulation of paths compared to the `os` module.
To obtain the current directory using `pathlib`, you can use the following code:
“`python
from pathlib import Path
current_directory = Path.cwd()
print(“Current Directory:”, current_directory)
“`
In this example, `Path.cwd()` returns a `Path` object representing the current working directory, which can also be easily manipulated or converted to a string if needed.
Comparison of Methods
While both methods achieve the same result, the choice between `os` and `pathlib` may depend on your specific use case and coding style preference. Below is a comparison of the two approaches:
Feature | os Module | pathlib Module |
---|---|---|
Type of Return | String | Path object |
Usability | Procedural | Object-oriented |
Python Version | Available in all versions | Python 3.4 and above |
Path Manipulation | Requires string methods | Native methods available |
Both methods are effective, but `pathlib` provides enhanced functionality for handling and manipulating file paths, making it a better choice for complex tasks involving filesystem paths.
Considerations for Different Environments
When working in various environments, such as integrated development environments (IDEs), command line, or web applications, the current working directory may differ. It is essential to understand the context in which your Python script is running, as this can affect the output of `os.getcwd()` and `Path.cwd()`.
- In IDEs, the current directory might be set to the project root.
- In scripts executed from the command line, it will be the directory from which the script is run.
- In web applications, the working directory could vary based on the server configuration.
Understanding these nuances helps in correctly managing file paths and avoiding common pitfalls related to relative and absolute path usage.
Methods to Get the Current Directory in Python
In Python, several methods can be employed to retrieve the current working directory. Below are the primary approaches, each suited for different use cases.
Using `os` Module
The `os` module provides a straightforward way to interact with the operating system. To get the current directory, the `os.getcwd()` function is commonly used.
“`python
import os
current_directory = os.getcwd()
print(“Current Directory:”, current_directory)
“`
- Function: `os.getcwd()`
- Returns: A string representing the current working directory.
Using `pathlib` Module
The `pathlib` module, introduced in Python 3.4, offers an object-oriented approach to handling filesystem paths. You can obtain the current directory using the `Path` class.
“`python
from pathlib import Path
current_directory = Path.cwd()
print(“Current Directory:”, current_directory)
“`
- Method: `Path.cwd()`
- Returns: A `Path` object representing the current directory.
Using `os` and `sys` Modules
Another method involves using the `sys` module in conjunction with `os`. This approach can be particularly useful if you need to access the directory from the script’s file location.
“`python
import os
import sys
current_directory = os.path.dirname(os.path.abspath(sys.argv[0]))
print(“Current Directory of the Script:”, current_directory)
“`
- Functionality:
- `sys.argv[0]`: Retrieves the script’s filename.
- `os.path.abspath()`: Converts it to an absolute path.
- `os.path.dirname()`: Extracts the directory portion.
Comparison of Methods
Method | Module | Returns | Use Case |
---|---|---|---|
`os.getcwd()` | os | String | Simple retrieval of current directory. |
`Path.cwd()` | pathlib | Path object | Object-oriented handling of paths. |
`os.path` | os + sys | String | Current directory of the running script. |
Each method has its advantages, depending on whether you prefer a functional or object-oriented approach, or if you need the directory related to the script rather than the process.
Understanding How to Retrieve the Current Directory in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “To effectively retrieve the current directory in Python, one can utilize the `os` module, specifically the `os.getcwd()` function. This approach is not only straightforward but also ensures compatibility across different operating systems, making it a reliable choice for developers.”
Michael Chen (Lead Python Instructor, Code Academy). “Using `os.getcwd()` is a fundamental skill for any Python programmer. It allows for dynamic file handling and directory management, which are crucial for building robust applications. I always emphasize this in my courses as it lays the groundwork for more advanced file operations.”
Sarah Thompson (Data Scientist, Tech Innovations Inc.). “In data science projects, knowing how to get the current directory can streamline data loading processes. Using `os.getcwd()` not only helps in locating files but also in organizing project structures efficiently, which is essential for maintaining clarity in complex analyses.”
Frequently Asked Questions (FAQs)
How can I get the current working directory in Python?
You can obtain the current working directory in Python using the `os` module. By calling `os.getcwd()`, you will receive the path of the current directory as a string.
Is there an alternative method to get the current directory in Python?
Yes, you can also use the `pathlib` module. By creating a `Path` object with `Path.cwd()`, you will retrieve the current working directory as a `Path` object.
What is the difference between os.getcwd() and Path.cwd()?
`os.getcwd()` returns the current directory as a string, while `Path.cwd()` returns it as a `Path` object, which provides additional functionality for path manipulations.
Can I change the current working directory in Python?
Yes, you can change the current working directory using `os.chdir(path)`, where `path` is the new directory you want to set as the current working directory.
How can I verify the current directory after changing it?
After changing the directory with `os.chdir(path)`, you can verify the current directory by calling `os.getcwd()` or `Path.cwd()` to ensure the change was successful.
Are there any exceptions I should be aware of when changing directories?
Yes, attempting to change to a non-existent directory will raise a `FileNotFoundError`. Always ensure the path exists before calling `os.chdir()`.
In Python, obtaining the current working directory is a straightforward process that can be accomplished using the built-in `os` module or the `pathlib` module. The most common method involves using `os.getcwd()`, which returns the path of the current working directory as a string. Alternatively, the `pathlib.Path` class provides a more modern approach with the `Path.cwd()` method, which also yields the current directory in a `Path` object format. Both methods are efficient and widely used in various applications.
It is important to understand the context in which the current directory is relevant. The current working directory is the directory from which a Python script is executed, and it can influence file operations such as reading from or writing to files. Therefore, knowing how to retrieve this information is crucial for effective file management and ensuring that scripts function correctly in different environments.
Moreover, developers should be aware that the current directory can change during the execution of a program, especially if the script includes commands that alter the working directory. As a best practice, it is advisable to use absolute paths when working with files to avoid potential issues related to changes in the current directory. This approach enhances the robustness of the code and minimizes errors associated with file handling
Author Profile
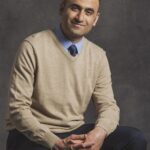
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?