How Can I Retrieve the Value of an Input Field in JavaScript?
In the dynamic world of web development, understanding how to interact with user inputs is crucial for creating engaging and responsive applications. Whether you’re building a simple form or a complex web application, capturing the value of input fields is a fundamental skill that every developer should master. JavaScript, the backbone of interactivity on the web, provides powerful tools to access and manipulate these values, allowing you to enhance user experience and streamline data handling.
At its core, retrieving the value of an input in JavaScript involves understanding the Document Object Model (DOM) and how it represents the structure of your web page. By leveraging various methods and properties, you can easily access user inputs, whether they come from text fields, checkboxes, or dropdown menus. This capability not only empowers you to validate user data but also enables you to perform actions based on user interactions, making your applications more dynamic and user-friendly.
As we delve deeper into this topic, we’ll explore the various techniques and best practices for capturing input values in JavaScript. From using event listeners to accessing input properties, you’ll gain insights that will elevate your coding skills and enhance your web projects. Get ready to unlock the potential of user input and transform the way you build interactive web experiences!
Accessing Input Values
To retrieve the value of an input field in JavaScript, you typically use the Document Object Model (DOM) to access the input element and then extract its value. The most common methods for accessing input values are through the `getElementById` and `querySelector` methods.
Using getElementById
The `getElementById` method is straightforward and effective when you know the ID of the input element. Here’s how to use it:
javascript
function getInputValue() {
var inputValue = document.getElementById(“myInput”).value;
console.log(inputValue);
}
In this example, clicking the button will log the value of the input field to the console.
Using querySelector
The `querySelector` method is more versatile as it allows you to select elements using CSS selectors. This can be particularly useful when dealing with classes or more complex selectors.
javascript
function getInputValue() {
var inputValue = document.querySelector(“.myInput”).value;
console.log(inputValue);
}
This method works similarly, but it provides more flexibility in selecting elements.
Handling Different Input Types
It’s essential to note that input elements can have various types (e.g., text, checkbox, radio). Accessing their values may require slightly different approaches:
- Text Input: Use `.value` as shown in the previous examples.
- Checkbox: Use `.checked` to determine if the checkbox is checked or not.
- Radio Button: Use a loop or `querySelector` to find the selected radio button.
Here’s a brief code snippet demonstrating how to handle checkboxes and radio buttons:
javascript
function getValues() {
var checkboxValue = document.getElementById(“myCheckbox”).checked;
var selectedRadio = document.querySelector(‘input[name=”myRadio”]:checked’).value;
console.log(“Checkbox: ” + checkboxValue);
console.log(“Selected Radio: ” + selectedRadio);
}
Table of Input Types and Methods
Input Type | Method to Access Value |
---|---|
Text | .value |
Checkbox | .checked |
Radio | querySelector(‘input[name=”name”]:checked’).value |
Dropdown (Select) | .value |
Understanding these methods and how to manipulate input values is crucial for effective form handling in JavaScript.
Accessing Input Values
To retrieve the value of an input field in JavaScript, you typically use the `value` property of the input element. This can be accomplished in various contexts, such as when a user submits a form or when they interact with the input directly.
Using Document Object Model (DOM) Methods
You can access input values using various DOM methods. Here are common approaches:
- By Element ID: If the input element has an `id` attribute, you can access it directly.
javascript
const inputValue = document.getElementById(‘inputId’).value;
- By Class Name: If the input has a class, you can use `getElementsByClassName`, which returns a live HTMLCollection.
javascript
const inputValue = document.getElementsByClassName(‘inputClass’)[0].value;
- Using Query Selectors: The `querySelector` method allows for more complex selections using CSS selectors.
javascript
const inputValue = document.querySelector(‘.inputClass’).value;
Handling Events to Capture Input Values
Often, you may want to capture the input value on specific events. Here are common events and their implementations:
- On Input Change: Capture the value when the input changes.
javascript
const inputElement = document.getElementById(‘inputId’);
inputElement.addEventListener(‘input’, function() {
console.log(inputElement.value);
});
- On Form Submission: Retrieve input values when a form is submitted.
javascript
const formElement = document.getElementById(‘formId’);
formElement.addEventListener(‘submit’, function(event) {
event.preventDefault(); // Prevents the form from submitting
const inputValue = document.getElementById(‘inputId’).value;
console.log(inputValue);
});
Examples of Input Types
Different input types may require specific handling. Below is a table outlining how to access values for various input types.
Input Type | Example Code |
---|---|
Text | document.getElementById('textInput').value; |
Checkbox | document.getElementById('checkboxInput').checked; |
Radio | document.querySelector('input[name="radioName"]:checked').value; |
Select (Dropdown) | document.getElementById('selectInput').value; |
Debugging Input Value Retrieval
When debugging issues related to input value retrieval, consider the following tips:
- Console Logging: Use `console.log()` to output values for verification.
- Inspect Element: Check the structure of the HTML to ensure the correct IDs or classes are being targeted.
- Check Event Listeners: Ensure that event listeners are properly set up and that they are firing as expected.
By understanding these methods and practices, you can effectively retrieve input values in JavaScript and handle user interactions seamlessly.
Expert Insights on Retrieving Input Values in JavaScript
Emily Chen (Senior Web Developer, Tech Innovations Inc.). “To effectively retrieve the value of an input field in JavaScript, one should utilize the `document.getElementById()` method or query selectors. This allows for precise targeting of the input element, ensuring that the correct value is accessed.”
Michael Thompson (JavaScript Educator, Code Academy). “Using event listeners is crucial when capturing input values dynamically. Implementing the `input` event allows developers to respond to user interactions in real-time, making the application more interactive and user-friendly.”
Sarah Patel (Frontend Engineer, Digital Solutions Group). “For modern applications, leveraging frameworks like React or Vue can simplify the process of managing input values. These frameworks provide built-in state management, which streamlines data handling and enhances performance.”
Frequently Asked Questions (FAQs)
How do I access the value of an input field in JavaScript?
You can access the value of an input field by using the `value` property of the input element. For example, if you have an input with the id `myInput`, you can retrieve its value using `document.getElementById(‘myInput’).value`.
What is the difference between `getElementById` and `querySelector` for getting input values?
`getElementById` selects an element based on its unique ID, while `querySelector` can select elements using CSS selectors, allowing for more complex selections. Both can be used to retrieve input values, but `querySelector` offers greater flexibility.
Can I get the value of an input field on a button click?
Yes, you can retrieve the value of an input field when a button is clicked by adding an event listener to the button. Inside the event handler, access the input value using the appropriate method, such as `document.getElementById(‘myInput’).value`.
How do I handle input values in a form submission?
To handle input values during form submission, you can attach an event listener to the form’s `submit` event. Within the event handler, prevent the default submission behavior and access the input values as needed.
Is it possible to get the value of multiple input fields at once?
Yes, you can retrieve the values of multiple input fields by selecting them using a common class or attribute. You can iterate through the selected elements and access their `value` properties to gather all input values.
What should I do if the input field is empty?
You can check if the input field is empty by comparing its value to an empty string. If the value is empty, you can handle it accordingly, such as displaying an error message or preventing form submission.
In JavaScript, obtaining the value of an input element is a fundamental task that is essential for interactive web applications. The most common method to achieve this is by using the `document.getElementById()` or `document.querySelector()` functions to select the input element. Once the element is selected, the value can be accessed through the `value` property of the input element. This straightforward approach allows developers to easily retrieve user input for processing or validation purposes.
It is also important to consider the context in which the input value is being retrieved. For example, handling events such as ‘input’, ‘change’, or ‘submit’ can significantly affect how and when the value is accessed. By attaching event listeners to input elements, developers can capture user input in real-time or upon specific actions, enhancing the user experience. Additionally, proper handling of input types, such as text, number, or checkbox, is crucial for ensuring that the retrieved values are accurate and usable.
Moreover, utilizing modern frameworks and libraries, such as React or Vue.js, can streamline the process of managing input values through state management. These tools provide built-in mechanisms for binding input values to state variables, simplifying the data flow within applications. This not only improves code
Author Profile
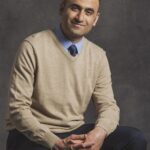
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?