How Can You Implement Waterfall Testing in Selenium with Python?
Introduction
In the world of web automation and testing, Selenium has emerged as a powerful tool for developers and testers alike. One of the most visually striking and useful features of Selenium is the ability to create waterfall charts, which can help visualize the performance of web applications over time. Whether you are monitoring load times, analyzing user interactions, or assessing the efficiency of your web pages, understanding how to generate and interpret waterfall charts using Selenium in Python can significantly enhance your testing strategies. In this article, we will explore the essential steps and techniques to harness this capability, enabling you to gain deeper insights into your web applications.
Waterfall charts are an invaluable resource for anyone involved in web development or testing. They provide a clear visual representation of how various elements of a webpage load and interact, allowing teams to pinpoint bottlenecks and optimize performance. By integrating Selenium with Python, you can automate the process of gathering data for these charts, making it easier to track changes over time and ensure that your web applications are running smoothly. This synergy not only improves efficiency but also empowers developers to make data-driven decisions based on real-time performance metrics.
As we delve into the specifics of creating waterfall charts with Selenium in Python, we will cover the fundamental concepts and tools required for successful implementation. From setting up
Understanding Waterfall Testing in Selenium
Waterfall testing is a linear and sequential approach to software development, where each phase must be completed before the next begins. This methodology can be effectively implemented in Selenium for automated testing. Selenium is a widely-used framework for testing web applications, and it supports various programming languages, including Python.
To implement waterfall testing using Selenium in Python, follow these steps:
- Define the project requirements and testing objectives.
- Design the test cases based on the specifications.
- Implement the Selenium scripts for each test case.
- Execute the tests in a controlled environment.
- Validate the results and document the findings.
Setting Up Selenium for Python
Before diving into waterfall testing, ensure that your environment is ready. Here are the steps to set up Selenium with Python:
- Install Python: Ensure you have Python installed on your machine. You can download it from the official Python website.
- Install Selenium: Use pip to install the Selenium package:
bash
pip install selenium
- Download a WebDriver: Depending on the browser you wish to automate (e.g., Chrome, Firefox), download the corresponding WebDriver. For Chrome, download ChromeDriver and ensure it matches your browser version.
- Set Up Your Script: Create a Python script to initialize Selenium. Below is a simple example to get started:
python
from selenium import webdriver
# Initialize the Chrome driver
driver = webdriver.Chrome(executable_path=’path/to/chromedriver’)
# Navigate to a webpage
driver.get(‘https://www.example.com’)
# Close the browser
driver.quit()
Creating a Waterfall Testing Framework
To build a waterfall testing framework using Selenium in Python, follow these structured steps:
- Requirements Gathering: Clearly outline the features to be tested.
- Test Case Design: Write test cases that describe the input, execution, and expected output.
- Test Script Development: Develop test scripts using Selenium, ensuring they are modular and reusable.
- Test Execution: Run the scripts in a designated environment, tracking any issues.
- Results Analysis: Analyze the output and compare it against expected results.
Example of a Test Case
Below is a sample structure for a test case that can be implemented in Selenium using Python:
Test Case ID | Description | Steps | Expected Result |
---|---|---|---|
TC001 | Verify login functionality |
|
User should be redirected to the dashboard |
By adhering to this structured approach, you can effectively implement waterfall testing in Selenium with Python, ensuring that your tests are thorough and reliable.
Understanding Waterfall Testing in Selenium
Waterfall testing is a traditional software development methodology where each phase must be completed before the next one begins. In the context of Selenium, this approach can be applied to ensure that each step of the testing process is thoroughly validated. This is particularly useful for complex web applications where multiple features interact with each other.
Setting Up Selenium for Waterfall Testing
To effectively implement waterfall testing using Selenium with Python, follow these steps:
- Install Required Packages
Ensure you have the necessary libraries installed. Use pip for installation:
bash
pip install selenium
- Download WebDriver
Depending on the browser you are testing, download the appropriate WebDriver. For example:
- Chrome: [ChromeDriver](https://sites.google.com/chromium.org/driver/)
- Firefox: [GeckoDriver](https://github.com/mozilla/geckodriver/releases)
- Setup Your Test Environment
Create a Python script and import the required modules:
python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
Implementing Waterfall Testing Steps
The waterfall testing approach can be structured into distinct phases, often resembling the following:
Phase | Description | Selenium Implementation Example |
---|---|---|
Requirements | Define the requirements for each feature | Document expected behaviors |
Design | Create a test plan detailing the test cases | Outline test scripts |
Implementation | Write test scripts for each feature | Use Selenium to navigate and test UI |
Verification | Execute tests and verify outcomes | Use assertions to validate results |
Maintenance | Update tests as application evolves | Refactor scripts to accommodate changes |
Example of Waterfall Testing Script
Here’s a simplified example of how to structure a waterfall testing script using Selenium:
python
# Importing libraries
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Step 1: Initialize WebDriver
driver = webdriver.Chrome(executable_path=’path/to/chromedriver’)
driver.get(‘https://example.com’)
try:
# Step 2: Requirements – Wait for element
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, ‘requiredElementId’))
)
# Step 3: Implementation – Perform actions
element.click()
# Step 4: Verification – Check outcomes
assert “Expected Title” in driver.title
finally:
# Step 5: Maintenance – Close the driver
driver.quit()
Best Practices for Waterfall Testing with Selenium
To maximize the effectiveness of waterfall testing in Selenium, consider the following best practices:
- Maintain Clear Documentation: Ensure every test case is well-documented, outlining expected behaviors and outcomes.
- Version Control: Utilize version control systems like Git to manage changes in your test scripts.
- Regular Review: Periodically review and update test cases as application features change.
- Modular Scripts: Design test scripts in a modular fashion to facilitate easier maintenance and updates.
By adhering to these practices, you can enhance the reliability and effectiveness of your waterfall testing strategy using Selenium in Python.
Expert Insights on Implementing Waterfall in Selenium with Python
Dr. Emily Carter (Senior Software Engineer, Automation Solutions Inc.). “To effectively implement a waterfall model using Selenium in Python, it is crucial to define clear phases for requirements gathering, design, implementation, testing, and maintenance. Each phase should be meticulously documented to ensure that the transition between stages is smooth and that all stakeholders are aligned.”
James Liu (Lead QA Analyst, Tech Innovations Group). “Incorporating the waterfall approach in Selenium testing requires a structured test plan that outlines all the test cases upfront. This ensures that all functionalities are tested in a sequential manner, which is essential for projects with fixed requirements and timelines.”
Sarah Thompson (Automation Testing Consultant, Quality First Consulting). “When using Selenium with Python in a waterfall framework, it is important to focus on comprehensive documentation and regular reviews at each stage. This will help identify potential issues early and ensure that the final product meets the initial specifications without significant deviations.”
Frequently Asked Questions (FAQs)
What is a waterfall model in Selenium testing?
The waterfall model in Selenium testing is a sequential design process where each phase must be completed before the next begins. It emphasizes thorough documentation and planning, making it easier to manage testing phases.
How do I implement a waterfall approach in Selenium with Python?
To implement a waterfall approach in Selenium with Python, define clear phases such as requirements gathering, test planning, test case development, test execution, and maintenance. Ensure each phase is completed and reviewed before proceeding to the next.
What are the advantages of using a waterfall model in Selenium testing?
The advantages include structured phases, clear documentation, and easier management of testing processes. It also allows for better tracking of progress and requirements, which can enhance communication among team members.
Are there any disadvantages to the waterfall model in Selenium testing?
Yes, disadvantages include inflexibility to changes, potential for late discovery of defects, and challenges in accommodating evolving requirements. This model may not be suitable for projects requiring frequent updates or iterative development.
Can I combine the waterfall model with other testing methodologies in Selenium?
Yes, combining the waterfall model with agile or iterative methodologies can provide flexibility while maintaining structure. This hybrid approach allows for adjustments during the testing process while adhering to the waterfall’s sequential nature.
What tools can assist in waterfall model implementation with Selenium in Python?
Tools such as JIRA for project management, TestRail for test case management, and Jenkins for continuous integration can assist in implementing the waterfall model with Selenium in Python, ensuring effective tracking and execution of testing phases.
obtaining a waterfall effect in Selenium using Python involves implementing a series of automated actions that simulate a cascading or sequential flow of events. This technique can be particularly useful for testing web applications where multiple interactions occur in a specific order, such as filling out forms, clicking buttons, or navigating through pages. By utilizing Selenium’s capabilities, developers can create scripts that mimic user behavior effectively, ensuring that the application responds correctly under various conditions.
Key takeaways include the importance of structuring your Selenium scripts to handle timing and synchronization issues, which are critical for achieving a smooth waterfall effect. Utilizing explicit waits can help manage the timing of interactions, ensuring that each action occurs only after the previous one has completed successfully. Additionally, organizing your code into functions can enhance readability and maintainability, making it easier to manage complex sequences of actions.
Furthermore, it is essential to consider the use of appropriate selectors when interacting with web elements. This ensures that your script can reliably locate and interact with the intended elements. By following best practices in Selenium scripting, developers can create robust and efficient automation scripts that effectively demonstrate the waterfall effect in their web applications.
Author Profile
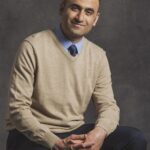
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?