How Can You Import a File into Python? A Step-by-Step Guide
In the world of programming, the ability to seamlessly integrate external data into your projects can be a game-changer. Whether you’re analyzing datasets, utilizing pre-written functions, or simply organizing your code for better readability, knowing how to import a file into Python is an essential skill for any aspiring developer. Python, with its rich ecosystem of libraries and straightforward syntax, makes the process of importing files not only efficient but also intuitive. In this article, we will explore the various methods of importing files into Python, empowering you to harness the full potential of your data and code.
Importing files in Python opens up a realm of possibilities, allowing you to work with different file formats such as CSV, JSON, and text files. Each format has its own unique characteristics and use cases, making it crucial to understand the best practices for importing them into your Python environment. From reading data for analysis to incorporating modules and packages, the ability to import files effectively can streamline your workflow and enhance your programming capabilities.
As we delve deeper into the topic, we will cover the fundamental techniques and libraries that facilitate file imports in Python. You’ll learn about built-in functions, popular libraries like Pandas and NumPy, and even how to handle potential errors during the import process. By the end of this
Using the Import Statement
To import a file into Python, the most common method is to utilize the `import` statement. This statement enables you to bring in modules, which can be either built-in or user-defined. For instance, if you have a module named `mymodule.py`, you can simply import it using:
“`python
import mymodule
“`
Once imported, you can access its functions and variables using the dot notation:
“`python
mymodule.my_function()
“`
If you only need specific components from a module, you can use the `from` keyword:
“`python
from mymodule import my_function
“`
This way, you can call `my_function()` directly without prefixing it with the module name.
Importing Specific File Types
When it comes to importing files, Python supports various formats such as CSV, JSON, and Excel files. Below are some popular libraries and methods to import these types of files:
- CSV Files: You can use the built-in `csv` module to read CSV files.
“`python
import csv
with open(‘data.csv’, mode=’r’) as file:
reader = csv.reader(file)
for row in reader:
print(row)
“`
- JSON Files: The `json` module allows you to work with JSON data easily.
“`python
import json
with open(‘data.json’, ‘r’) as file:
data = json.load(file)
print(data)
“`
- Excel Files: The `pandas` library is ideal for handling Excel files.
“`python
import pandas as pd
data = pd.read_excel(‘data.xlsx’)
print(data)
“`
File Paths and Working Directory
When importing files, it’s crucial to consider the file path. If the file is not in the current working directory, you need to provide an absolute or relative path. Here’s how to check and set your working directory in Python:
“`python
import os
Check current working directory
print(os.getcwd())
Change directory
os.chdir(‘/path/to/your/directory’)
“`
A common convention is to use relative paths for better portability. For example, if your script and data file are in the same directory, you can simply use the filename.
Handling Errors During Import
When importing files, it’s essential to handle potential errors gracefully. Common exceptions include `FileNotFoundError` and `PermissionError`. You can implement error handling using try-except blocks:
“`python
try:
with open(‘data.csv’, mode=’r’) as file:
reader = csv.reader(file)
for row in reader:
print(row)
except FileNotFoundError:
print(“The specified file was not found.”)
except PermissionError:
print(“You do not have permission to access this file.”)
“`
Table: Common File Import Methods
File Type | Library | Example Code |
---|---|---|
CSV | csv | import csv |
JSON | json | import json |
Excel | pandas | import pandas as pd |
Importing Modules in Python
To import files in Python, you typically use the `import` statement. This allows you to access functions, classes, and variables defined in another file or module.
- Basic Import:
Use the following syntax to import a module:
“`python
import module_name
“`
- Importing Specific Functions or Classes:
If you only need specific items from a module, you can import them directly:
“`python
from module_name import function_name
“`
- Importing with Aliases:
You can also assign an alias to a module for convenience:
“`python
import module_name as alias
“`
Importing Files from the Local Directory
To import files located in the same directory as your script, follow these steps:
- Ensure the target file is in the same folder as your main script.
- Use the `import` statement as shown above.
For example, if you have a file named `utilities.py`, you can import it as follows:
“`python
import utilities
“`
You can then access its functions by prefixing them with the module name:
“`python
utilities.function_name()
“`
Using the `importlib` Module
For dynamic imports or when you need to import a module whose name is not known until runtime, use the `importlib` module. This is particularly useful for plugins or dynamic loading of modules.
Example usage:
“`python
import importlib
module_name = ‘utilities’
utilities = importlib.import_module(module_name)
utilities.function_name()
“`
Importing from Subdirectories
When working with files in subdirectories, ensure that the subdirectory contains an `__init__.py` file (this can be empty). This file makes Python treat the directory as a package.
To import a module from a subdirectory:
“`python
from subdirectory import module_name
“`
Handling Import Errors
When importing modules, you may encounter errors. Here are common scenarios and their resolutions:
Error Type | Description | Solution |
---|---|---|
ModuleNotFoundError | The specified module cannot be found. | Verify the module name and path. |
ImportError | The module is found, but an import fails. | Check for spelling errors or circular imports. |
AttributeError | The module is found, but the specified function/class does not exist. | Check if the function/class is defined in the module. |
To handle these errors gracefully, consider using try-except blocks:
“`python
try:
import utilities
except ModuleNotFoundError:
print(“Module not found. Please check the file path.”)
“`
Best Practices for Importing
- Keep Imports at the Top: Always place your import statements at the beginning of your script to improve readability.
- Avoid Wildcard Imports: Using `from module_name import *` can clutter the namespace and lead to conflicts.
- Organize Imports: Group standard library imports, third-party imports, and local imports separately for clarity.
Expert Insights on Importing Files into Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Importing files into Python is a fundamental skill for data manipulation. Utilizing libraries such as Pandas and NumPy can streamline the process significantly, allowing for efficient data handling and analysis.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “When importing files in Python, it is crucial to understand the file format you are dealing with. Different formats, such as CSV, JSON, or Excel, require specific libraries and methods, which can greatly impact your workflow.”
Sarah Lee (Python Instructor, LearnPython Academy). “For beginners, I recommend starting with the built-in open() function for simple text files. As you progress, exploring libraries like Pandas for structured data will enhance your capabilities in data analysis.”
Frequently Asked Questions (FAQs)
How do I import a CSV file into Python?
To import a CSV file into Python, use the `pandas` library. First, install it using `pip install pandas`. Then, use the following code:
“`python
import pandas as pd
data = pd.read_csv(‘filename.csv’)
“`
What is the purpose of the `import` statement in Python?
The `import` statement in Python is used to include external modules or libraries into your script, allowing you to access their functions, classes, and variables.
Can I import files from a different directory in Python?
Yes, you can import files from a different directory by modifying the system path or using relative or absolute paths. For example:
“`python
import sys
sys.path.append(‘/path/to/directory’)
import module_name
“`
How do I import a text file and read its contents in Python?
To import and read a text file, use the built-in `open()` function. For example:
“`python
with open(‘filename.txt’, ‘r’) as file:
content = file.read()
“`
What libraries are commonly used to import Excel files into Python?
The `pandas` library is commonly used to import Excel files using the `read_excel()` function. Additionally, `openpyxl` and `xlrd` can also be used for reading Excel files.
Is it possible to import JSON files into Python?
Yes, you can import JSON files using the built-in `json` module. Use the following code:
“`python
import json
with open(‘filename.json’) as file:
data = json.load(file)
“`
Importing a file into Python is a fundamental skill that enables users to work with external data efficiently. The process typically involves using built-in functions or libraries tailored for specific file types, such as text files, CSV files, JSON files, and more. For instance, the `open()` function is commonly used for text files, while the `pandas` library offers powerful tools for handling CSV and Excel files. Understanding the appropriate methods for different file formats is crucial for effective data manipulation and analysis.
Moreover, error handling is an essential aspect of file importation in Python. Users should be aware of potential issues such as file not found errors, permission errors, and encoding problems. Implementing try-except blocks can help manage these exceptions gracefully, ensuring that the program can handle errors without crashing. This approach not only improves the robustness of the code but also enhances user experience by providing informative feedback.
In summary, mastering the techniques for importing files into Python is vital for anyone looking to leverage the language for data analysis or software development. By familiarizing oneself with various file types and employing best practices for error handling, users can streamline their workflows and maximize the utility of their data. This foundational knowledge serves as a stepping stone for more advanced data
Author Profile
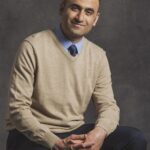
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?